COS 333: Chapter 15, Part 2
Summary
TLDRThis lecture delves into functional programming with Scheme, focusing on numeric predicate functions, control flow, and list operations. It explains how predicate functions return boolean values and introduces control structures like 'if' and 'cond' in Scheme. The lecture also covers list manipulation functions such as 'quote', 'car', 'cdr', and 'cons', highlighting their importance in functional programming. Additionally, it discusses recursion as the method for repetition in Scheme, due to its lack of loop support, and provides an example of implementing the 'member' function recursively to check for an element within a list.
Takeaways
- ๐ The lecture continues the discussion on functional programming with a focus on the Scheme programming language, building upon concepts introduced in the previous lecture.
- ๐ข Introduction to numeric predicate functions in Scheme, which are functions that return a boolean result and operate only on numeric literal values, not symbolic literals.
- ๐ Scheme's approach to control flow is explored, highlighting the absence of traditional loops due to its pure functional nature and the use of recursion for repetition.
- ๐ The 'if' function in Scheme is detailed, emphasizing its role as a selector function akin to 'if' statements in imperative languages, with a unique application to three parameters based on a predicate result.
- ๐ The 'cond' function is presented as Scheme's method for multiple selections, similar to a 'switch' statement, evaluating predicates in sequence until one is found to be true.
- ๐ The importance of list operations in Scheme is underscored, with functions like 'quote', 'list', 'car', 'cdr', and 'cons' being fundamental for list manipulation.
- ๐ The 'quote' function is explained as a means to prevent evaluation of list parameters, allowing them to be treated as literal data rather than function applications.
- ๐ Predicate functions for lists, such as 'list?' and 'null?', are introduced as essential for determining the nature and state of list structures within Scheme programs.
- ๐ The 'cons' function is described as a critical tool for constructing lists, allowing for the creation of new lists with elements added in a specific order.
- โ๏ธ The 'eqv?' and 'eq?' functions are contrasted, with 'eqv?' performing value comparisons and 'eq?' performing pointer comparisons, affecting how equivalence is determined.
- ๐ ๏ธ The 'let' function is introduced as a way to create local bindings within Scheme, allowing for the evaluation of expressions in the context of these bindings.
Q & A
What is the main focus of the second lecture on Chapter 15?
-The main focus of the second lecture is to continue the discussion on Scheme programming language within the context of functional programming, specifically covering numeric predicate functions, control flow, and list operations.
What is a predicate function in Scheme?
-A predicate function in Scheme is a function that produces a boolean result, either true or false.
How does Scheme represent true and false values?
-In Scheme, 't' represents a true value, '#f' represents a false value, and an empty list is also interpreted as false, while a non-empty list is interpreted as true.
What are some of the numeric comparison functions provided by Scheme?
-Scheme provides comparison functions such as 'equals', 'less than', 'greater than', 'not equals', 'greater than or equal to', and 'less than or equal to'.
How does Scheme handle control flow for selection and loops?
-Scheme handles control flow using functions like 'if' for two-way selection and 'cond' for multiple selections. It does not provide loop structures because it is a pure functional programming language without support for variables, so repetition is handled through recursion.
What is the purpose of the 'quote' function in Scheme?
-The 'quote' function in Scheme is used to avoid parameter evaluation, ensuring that lists are treated as literal data rather than function applications.
What are the 'car' and 'cdr' functions in Scheme used for?
-The 'car' function is used to access the first element of a list, and the 'cdr' function is used to access the rest of the list after the first element.
What is the 'cons' function in Scheme and how is it used?
-The 'cons' function in Scheme is used to construct a new list by placing its first parameter as the first element and appending the elements of the second parameter list to it.
What is the 'let' function in Scheme and how does it work?
-The 'let' function in Scheme is used to create local bindings for names with associated expressions. It evaluates the expressions, binds the values to the names, and then evaluates the body using these bindings.
Can you provide an example of a recursive function in Scheme?
-The 'member' function is an example of a recursive function in Scheme. It checks if an atom is contained within a list by recursively comparing the atom to each element of the list and eliminating one element at a time until a match is found or the list is empty.
What is the importance of the order of base cases and recursive case in a 'cond' expression in Scheme?
-The order in a 'cond' expression is important to ensure that base cases are checked first before the recursive case to avoid non-terminating recursion. Additionally, checking for an empty list with 'null' should come before checking for equality to prevent unnecessary evaluations.
Outlines
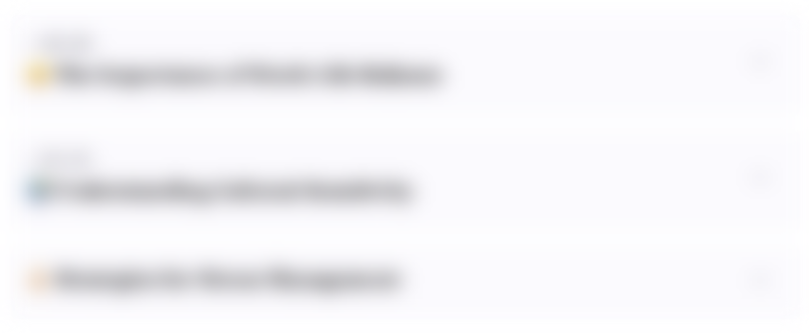
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
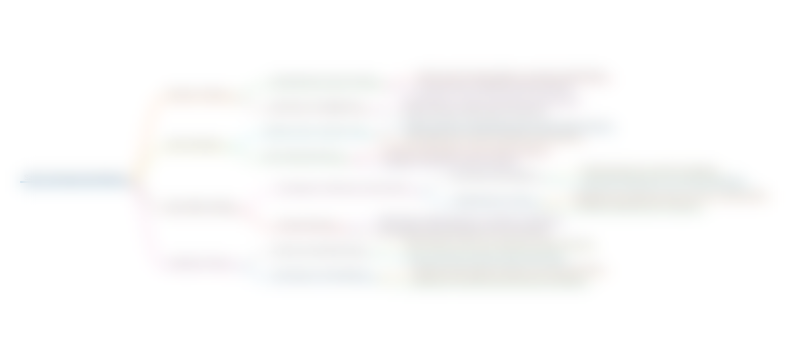
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
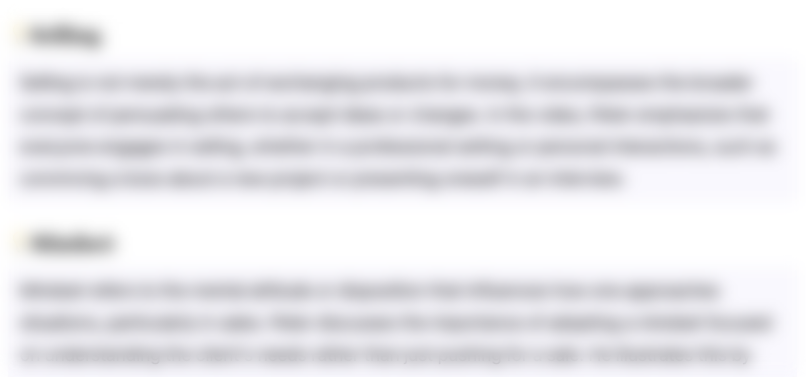
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
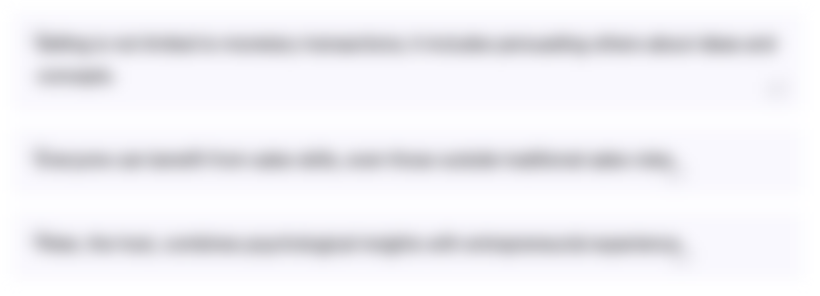
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
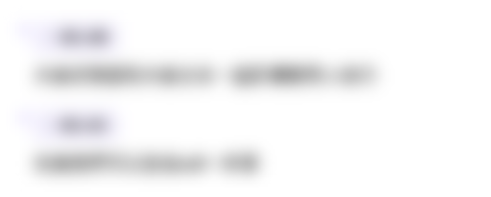
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)