COS 333: Chapter 6, Part 2
Summary
TLDRThis lecture delves into various data types, focusing on arrays and their operations across different programming languages. It discusses heterogeneous arrays, array types like jagged and rectangular, and introduces associative arrays. The lecture also explores record types, tuple types, and list types, highlighting their uses and differences. It touches on performance implications of dynamic versus static data access and concludes with a look at list comprehensions and their applications in functional programming languages.
Takeaways
- π The lecture delves into various data types, continuing from the previous lecture, focusing on array types and their advanced operations.
- π It differentiates between standard arrays and special types like associative arrays, which use keys for indexing instead of numerical indices.
- π The concept of heterogeneous arrays is introduced, where elements can be of different data types, in contrast to the homogeneous nature of standard arrays.
- π€ Programming languages like Perl, Python, JavaScript, and Ruby natively support heterogeneous arrays, while others can simulate them through object-oriented techniques.
- π§© The lecture explains array operations across different programming languages, including APL, Ada, Python, Java, and Ruby, highlighting unique features like elemental operations.
- π A distinction is made between rectangular and jagged arrays, with the latter allowing rows of varying lengths, a feature supported by languages like C, C++, and Java.
- π The concept of array slices is explored, which allows for the extraction of substructures from an array, applicable mainly in languages that support rectangular arrays.
- π Associative arrays are described as unordered collections indexed by keys, often implemented using hash tables for efficient data retrieval.
- π·οΈ Records, or structs, are introduced as named fields aggregates, fixed in structure unlike the flexible key-value pairs in associative arrays.
- π Records can be manipulated with operations like assignment, comparison, and initialization, with some languages like Ada supporting complex nested record structures.
- π The lecture concludes with a discussion on list types, central to languages like Lisp, Scheme, ML, and F#, and the use of list comprehensions for sophisticated list initialization.
Q & A
What are the main topics covered in the second lecture on chapter 2 about data types?
-The lecture continues the discussion on data types, focusing on special kinds of arrays, advanced array operations, associative arrays, record types, tuple types, and list data types.
What is a heterogeneous array and how can it be simulated in languages that do not natively support them?
-A heterogeneous array is an array that can store elements of different data types. It can be simulated in other languages by using arrays that contain a generic data value, often through the use of polymorphism and object inheritance.
Can you explain the difference between rectangular and jagged arrays?
-Rectangular arrays are two-dimensional structures where all rows and columns have the same number of elements, while jagged arrays allow rows to have varying numbers of elements, often represented as an array of arrays.
What is an array slice and in which types of arrays does it make sense to use it?
-An array slice is a substructure of an array that retrieves part of the array as a single unit. It is applicable to rectangular arrays but not to jagged arrays due to their different internal representation.
How are associative arrays different from regular arrays?
-Associative arrays are unordered collections of data elements indexed by keys, rather than having fixed numerical indices like regular arrays. They often use more complex data structures like hash tables for efficient data retrieval.
What are the two main design issues associated with associative arrays?
-The two main design issues are the syntactic form of references to elements (how to specify the key for the value to be retrieved) and whether associative arrays have dynamic or static sizes.
What is a record type and how does it differ from an array?
-A record type is a possibly heterogeneous aggregate of data elements referred to as fields, identified by names. Unlike arrays, records do not require elements to be of the same type, and access to fields is by name rather than by numerical index.
What is the purpose of the 'move corresponding' operation in the Ada programming language?
-The 'move corresponding' operation is used to copy all fields from a source record to the corresponding fields in a target record. It does not require the two records to be of the same type, allowing for partial copying of data where only corresponding fields are copied.
Why is accessing fields within a record generally faster than subscripting in an array?
-Accessing fields within a record is faster because field names are static and their memory locations are determined at compile time. In contrast, array subscripts are dynamic and require runtime computation to determine the memory location.
What are tuples and how do they differ from records?
-Tuples are similar to records in that they store a number of fields, potentially of different types. However, the fields in a tuple do not have names associated with them, making tuples suitable for temporary storage of grouped values without the need for individual field access.
Can you describe the use of list comprehensions in Python and their advantage over regular list initialization?
-List comprehensions in Python allow for sophisticated list initialization, including the ability to add conditions for the values to be placed into the list. This is more flexible and concise compared to regular list initialization, which often requires multiple steps and separate assignments.
Outlines
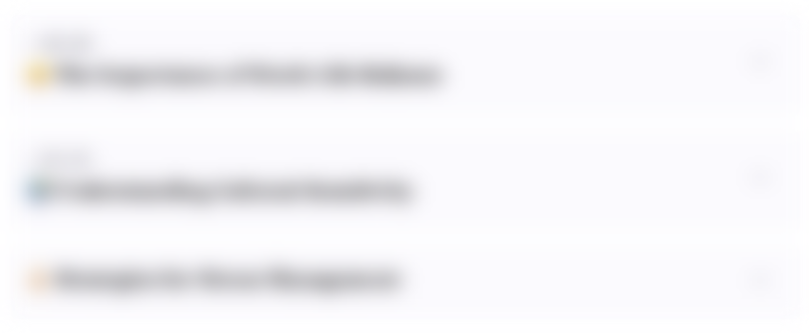
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
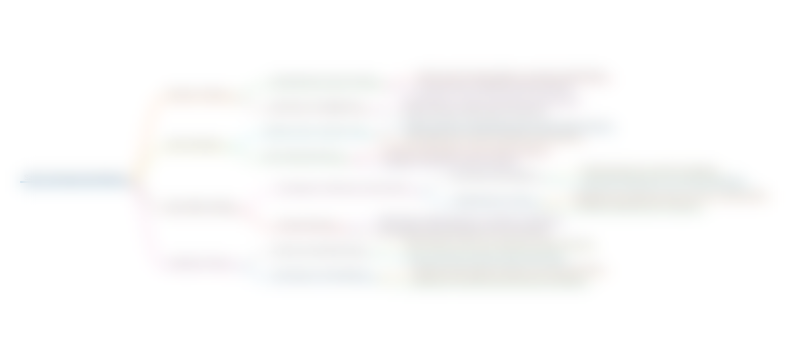
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
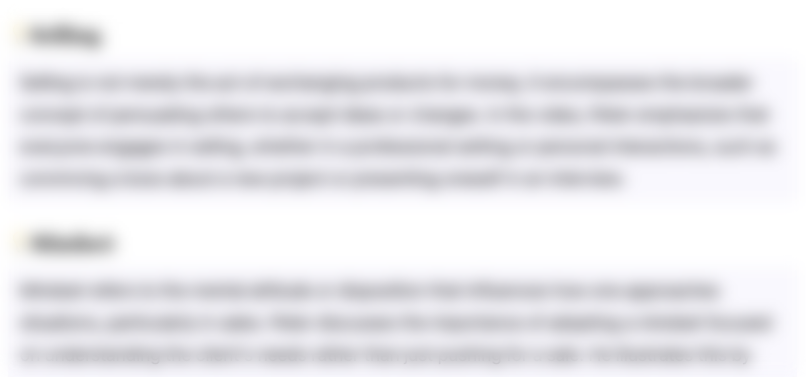
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
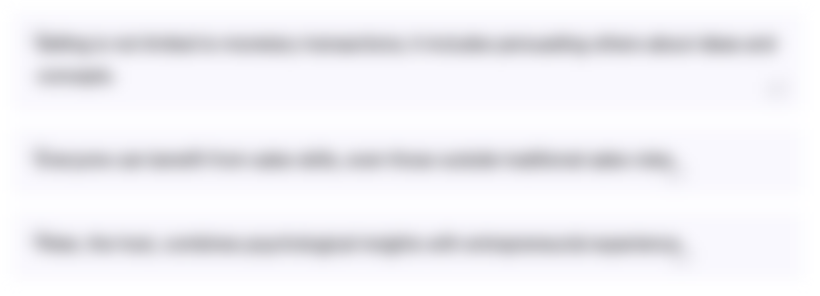
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
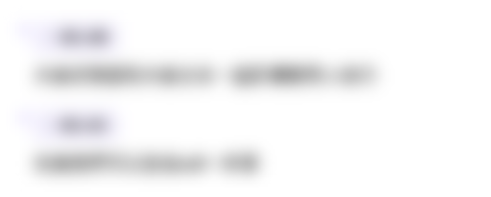
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)