Python Programming #3 - Arithmetic Operators and Strings
Summary
TLDRIn this Python Programming series video, Avi introduces arithmetic operators and strings, demonstrating their use with variables and real-world applications. The tutorial covers addition, subtraction, multiplication, division, and modulus with integers, and string operations like concatenation and repetition. Avi also explains string slicing, an essential feature for manipulating text in Python, providing a foundational understanding for further programming endeavors.
Takeaways
- 🔢 Arithmetic operators in Python include addition, subtraction, multiplication, division, and modulus, which are similar to those used in mathematics.
- 👫 Variables can be used to represent real-world entities, such as the ages of two friends, and manipulated using arithmetic operators.
- 📊 Addition is used to calculate the sum of values, as demonstrated by adding the ages of two friends to get a total.
- ➖ Subtraction can determine the difference between two values, like subtracting one friend's age from another's.
- 🔄 Multiplication with the asterisk (*) symbol can be used to scale numbers, such as calculating the product of two ages.
- 📉 Division in Python provides a quotient, which can be approximated to a decimal value, as shown with dividing one age by another.
- 🔄 Modulus gives the remainder of a division, useful for scenarios where only the leftover part after division is needed.
- 💡 Arithmetic operations are essential for real-world applications, such as calculating totals or averages.
- 📝 Strings are sequences of characters enclosed in quotation marks and can be manipulated using some arithmetic operators.
- 🔗 Concatenation of strings can be achieved using the addition operator, combining first and last names to form a full name.
- 🔁 String multiplication can repeat a string a specified number of times, as shown by repeating 'Hi' 10 times.
- 📚 Slicing is a feature in Python that allows accessing parts of a string using indices, which starts from zero.
- 👀 Slicing can be used to extract a substring, like getting the name 'Avi' from a sentence by specifying the start and end indices.
Q & A
What are the basic arithmetic operators discussed in the video?
-The basic arithmetic operators discussed in the video are addition, subtraction, multiplication, division, and modulus.
How are the ages of two friends represented in the script using variables?
-The ages of the two friends are represented using two variables: 'age1' set to 12 and 'Age2' set to 18.
What is the result of adding the ages of the two friends using Python's arithmetic operators?
-The result of adding the ages of the two friends is 30, which is obtained by using the expression 'age1 + Age2'.
What does the modulus operator do in Python?
-The modulus operator in Python provides the remainder of a division operation. For example, 'age2 % age1' gives the remainder 6 when 18 is divided by 12.
Can subtraction be applied to strings in Python like it is with integers?
-No, subtraction cannot be applied to strings in Python. Attempting to subtract one string from another will result in an error.
How can you concatenate two strings to form a full name in Python?
-You can concatenate two strings to form a full name by using the addition operator and including a space between them, like 'first_name + ' ' + last_name'.
What is the result of multiplying a string by an integer in Python?
-Multiplying a string by an integer in Python results in the repetition of the string that many times. For example, 'High' * 10 will repeat the string 'High' ten times.
What is slicing in Python and how is it used in the script?
-Slicing in Python is a way to extract a part of a string. In the script, it is used to extract the name 'Avi' from the sentence by using the slice 'sentence[0:3]'.
Why is the last index in a Python slice exclusive?
-The last index in a Python slice is exclusive because it allows you to specify the range up to, but not including, the character at that index.
How can arithmetic operators be useful in real-world applications according to the video?
-Arithmetic operators can be useful in real-world applications for tasks such as calculating the total amount to pay after adding various costs or determining the average rating from different user scores.
What is the importance of understanding arithmetic operators and strings in Python as mentioned in the video?
-Understanding arithmetic operators and strings is fundamental in Python and incredibly handy for various programming tasks, as emphasized in the video.
Outlines
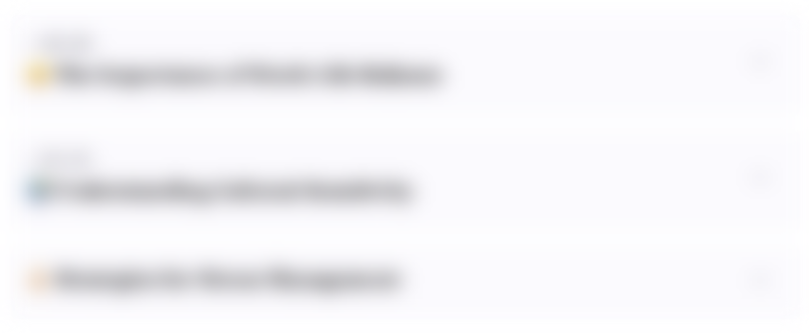
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
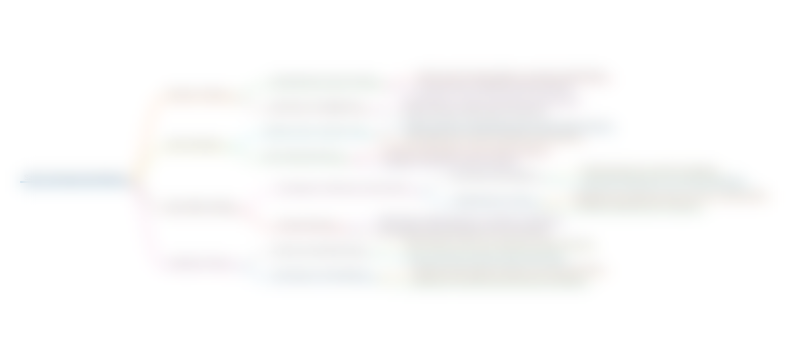
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
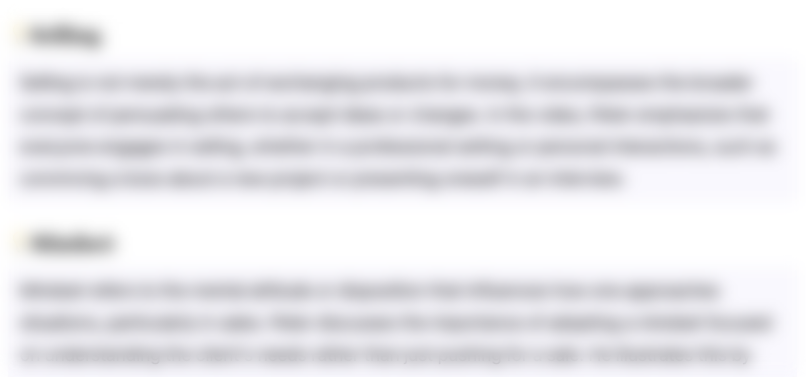
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
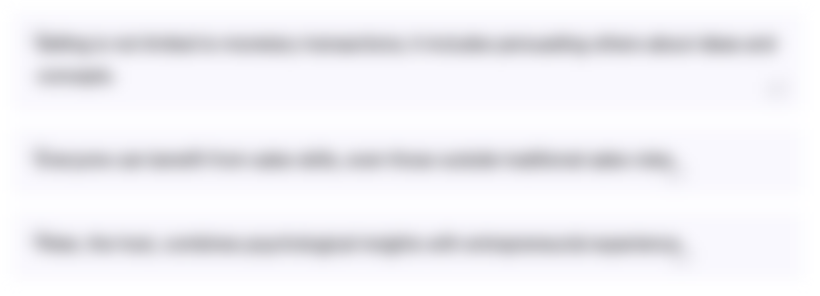
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
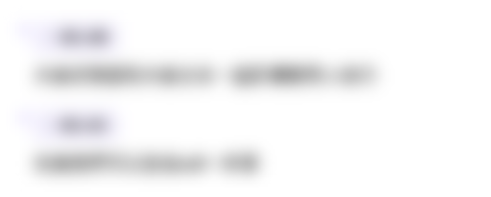
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
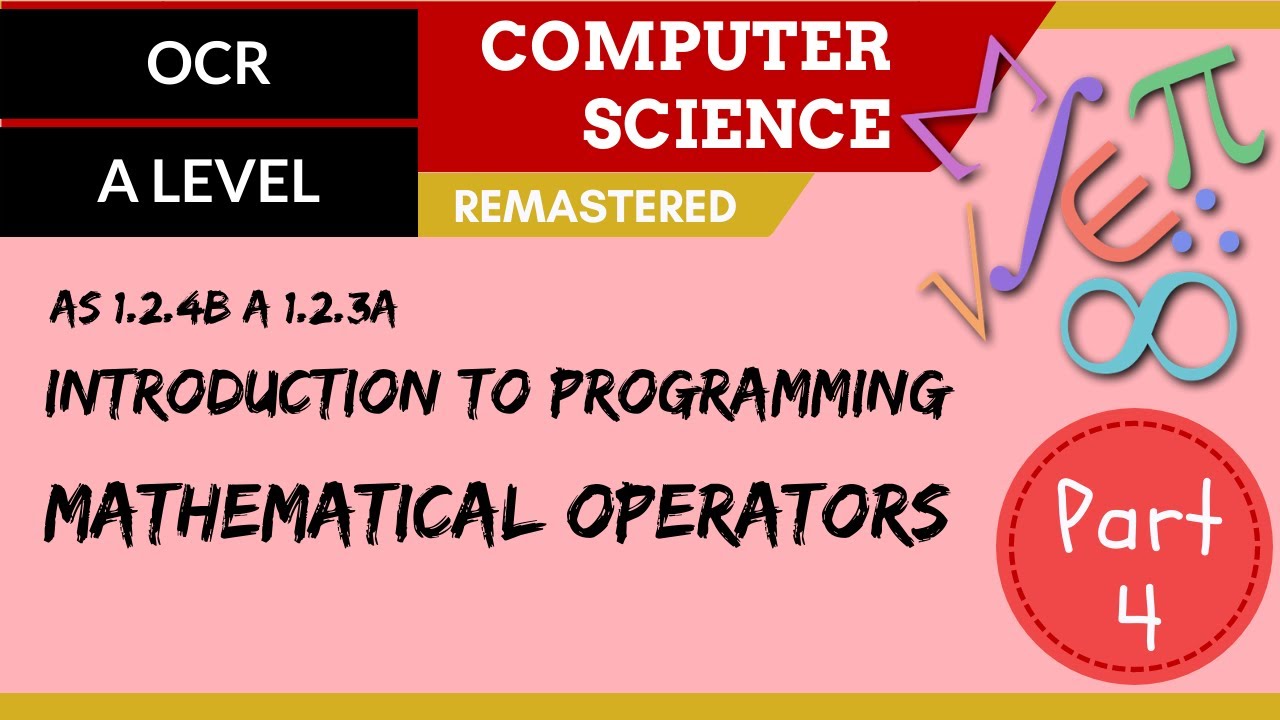
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators
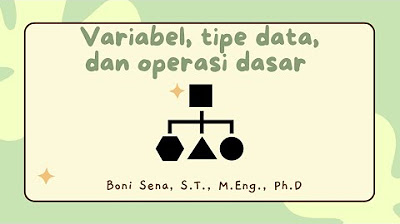
Variabel, tipe data dan operasi dasar

BAID MK1 P3
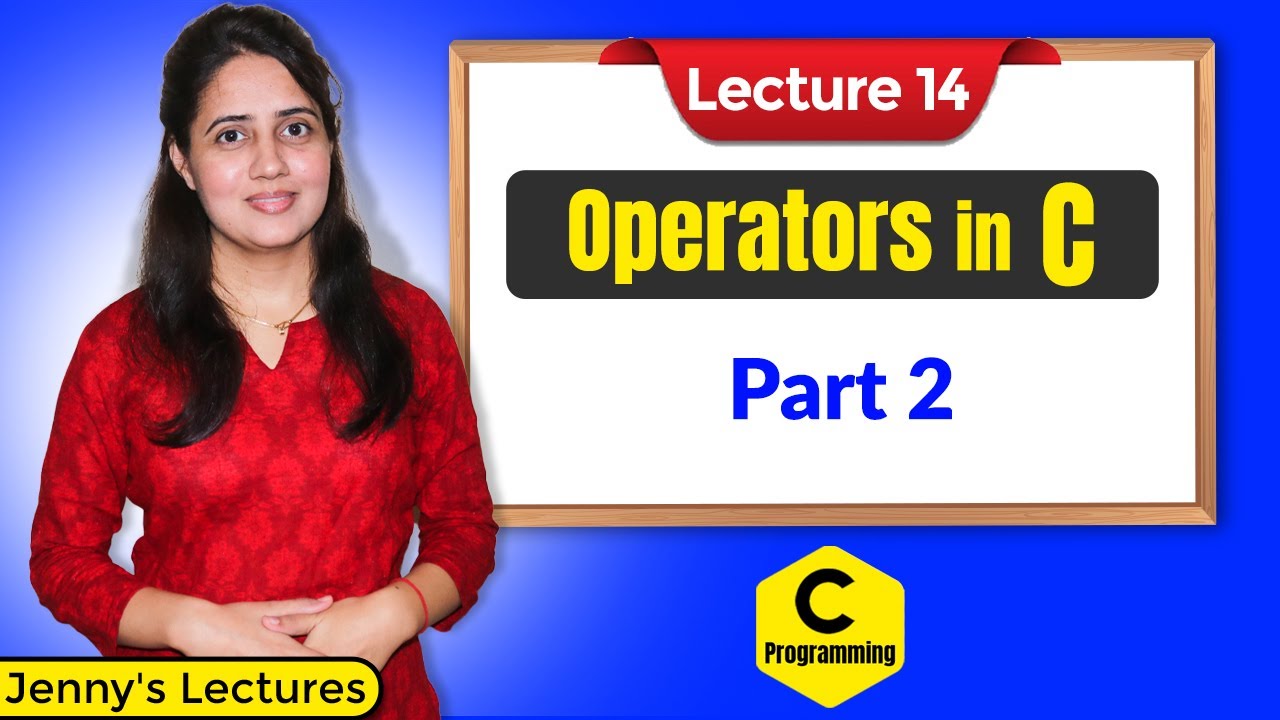
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
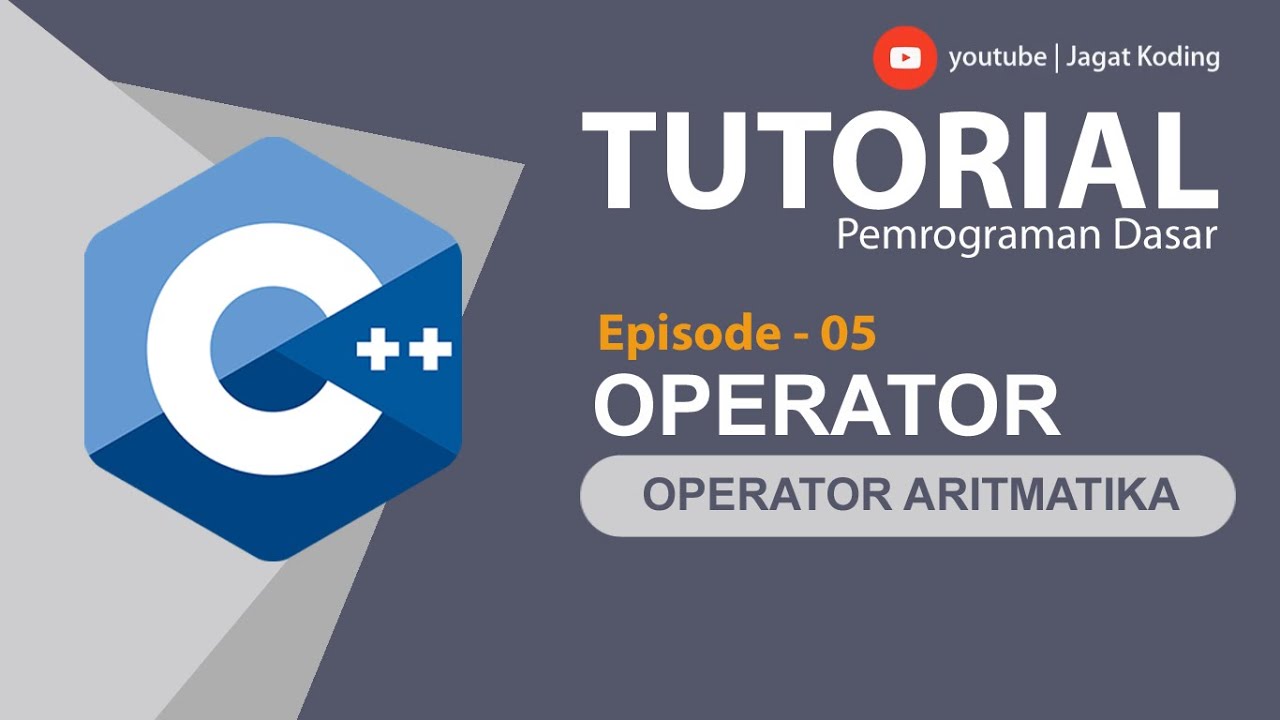
C++ 05 | Operator Aritmatika | Tutorial Dev C++ Indonesia
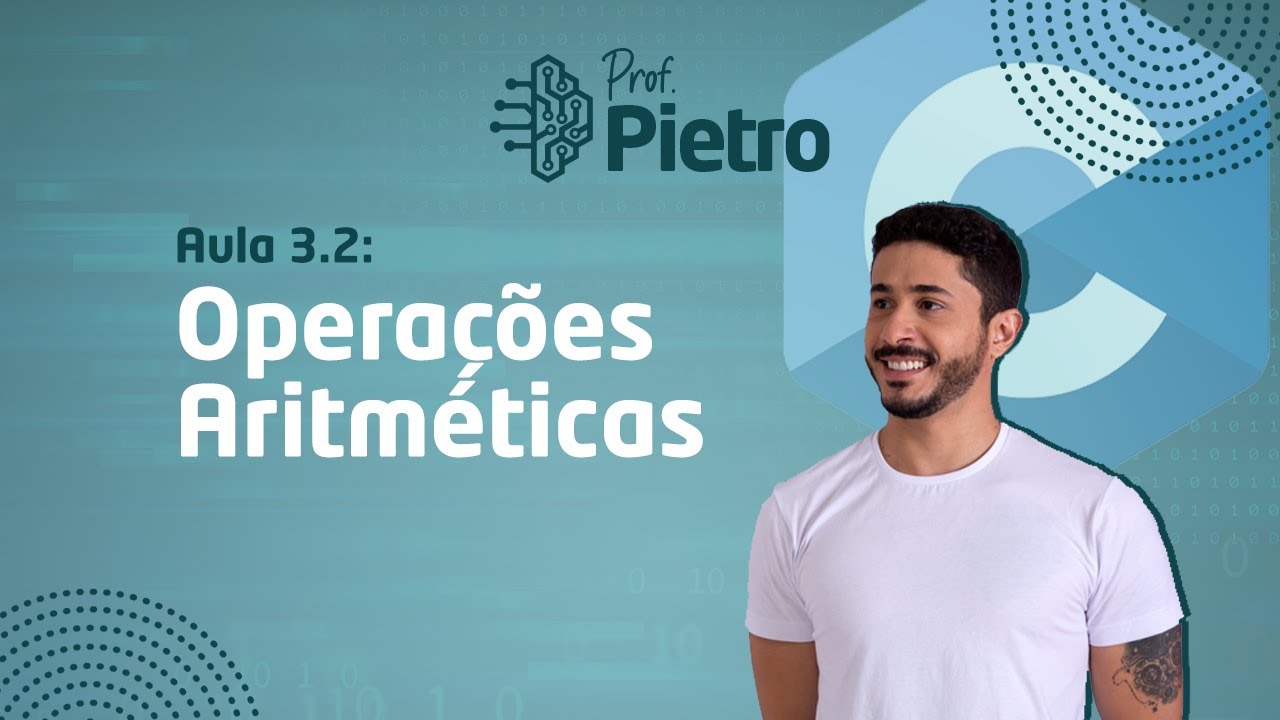
Linguagem C - Aula 3.2 - Aprenda a realizar cálculos em C (2022)
5.0 / 5 (0 votes)