41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
Summary
TLDRIn this educational video, part of a programming series, the focus is on fundamental programming concepts such as variables, constants, inputs, outputs, and assignments. Using Python, the tutorial explains how variables act as memory address pointers with user-friendly labels, and contrasts them with constants, which remain unchanged during program execution. The video also covers data types, the significance of assignment with the '=' operator, and the use of arithmetic operators. It demonstrates these concepts through a simple dice game for children, illustrating how to handle user input, process it, and output results. The script emphasizes the importance of understanding these core programming ideas, which are applicable across different programming languages.
Takeaways
- 💡 A variable is a pointer to a memory address that can be given a user-friendly name.
- 🎯 The CPU's memory address register is used to store the address of data to be read or written, similar to how variables work in programming.
- 📊 Variables can change or vary during the program's execution, hence the name 'variable'.
- 🔢 In Python, data types are not declared explicitly; the type is inferred from the initial value assigned to the variable.
- 🛑 Constants are values that remain fixed and do not change during program execution, but Python does not natively support constants.
- 📝 Assignment is the act of giving a value to a variable or constant, typically done using the equal sign.
- 🧮 Operators are used to perform operations on variables and constants, such as addition, which is demonstrated in the script with 'total = total + roll_value'.
- 🔄 Casting is the process of converting data from one type to another, like converting a string input to an integer.
- 📋 Input is gathered from the user, often via the keyboard, and can be assigned to variables for use in the program.
- 📢 Output statements are used to display information to the user, such as printing the results of a calculation to the screen.
- 🔑 The script emphasizes the importance of understanding programming concepts over specific language syntax, as the concepts are universal across languages.
Q & A
What is a variable in programming?
-A variable is a pointer to a memory address that can be given a user-friendly label or name, allowing the programmer to store and manipulate data during the execution of a program.
How does a variable differ from a constant in programming?
-A variable is a value that can change or vary during the execution of a program, while a constant is a value that remains fixed and does not change once it is set.
What is the role of the 'int' command in the context of the script?
-The 'int' command is used to cast or convert a value from a string to an integer, which is necessary when storing user input as a numerical value in the program.
What is the purpose of the 'input()' function in Python as described in the script?
-The 'input()' function in Python is used to get input from the user, typically from the keyboard, and it can also cast the input into a specific data type such as an integer.
What is the significance of the 'print' statement in Python?
-The 'print' statement in Python is used to output data to the screen, allowing the program to communicate results or information back to the user.
How does the script handle the rolling of dice in the dice game example?
-In the dice game example, the script simulates rolling dice by generating random numbers, and it ensures that doubles are rolled again by not counting them and requiring a re-roll.
What is the rule for combining the highest and lowest dice in the dice game described in the script?
-In the dice game, the player's score is determined by combining the highest and lowest dice values into a two-digit number, with the highest die value placed first.
Why is it important to understand the difference between variables and constants in programming?
-Understanding the difference between variables and constants is important because it helps programmers manage data that changes during program execution and data that remains constant, which can affect program logic and memory usage.
What is the role of data types in programming as mentioned in the script?
-Data types in programming define the kind of data a variable can hold, such as integers, strings, or floats. They are important because they determine how data is stored and manipulated within a program.
How does the script illustrate the concept of casting in programming?
-The script illustrates casting by showing how values are converted from one data type to another, such as converting user input from a string to an integer, or dice values from integers to strings.
What is the advice given to students regarding language syntax in the script?
-The script advises students to learn the underlying programming concepts rather than memorizing specific syntax, as the concepts can be applied to any programming language.
Outlines
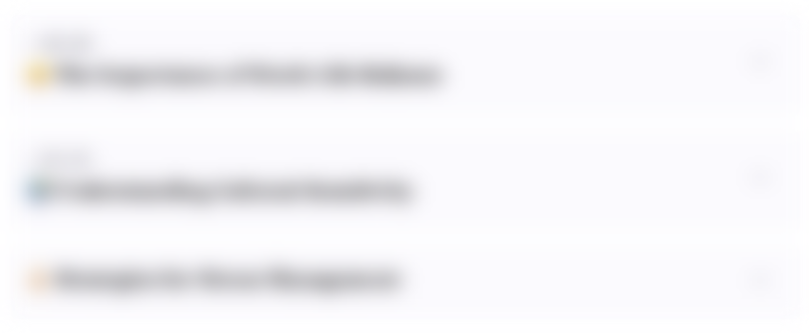
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
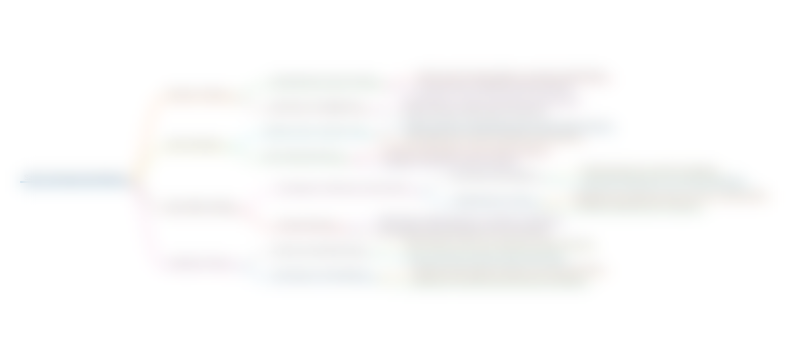
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
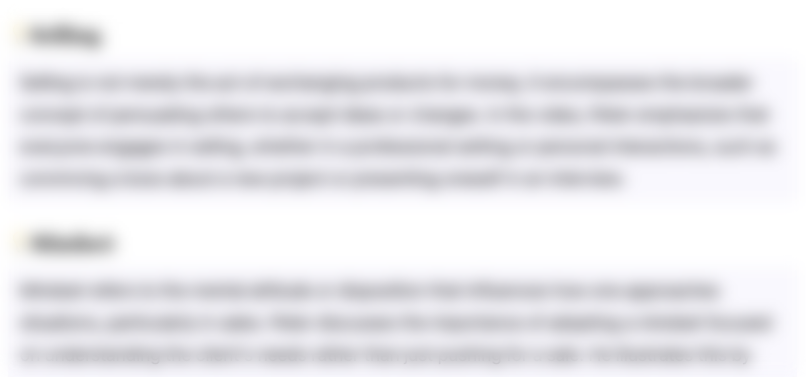
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
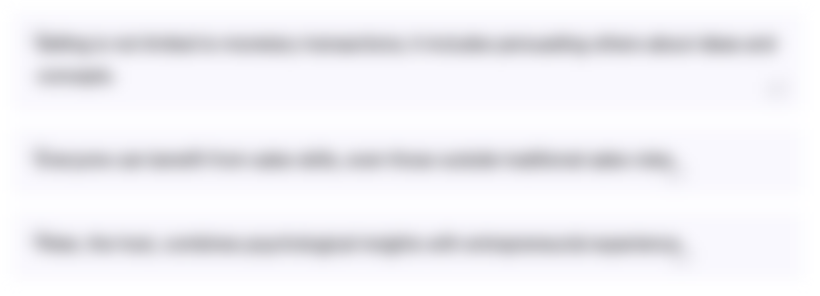
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
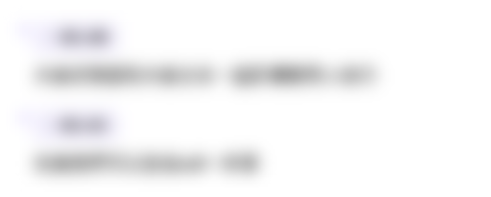
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
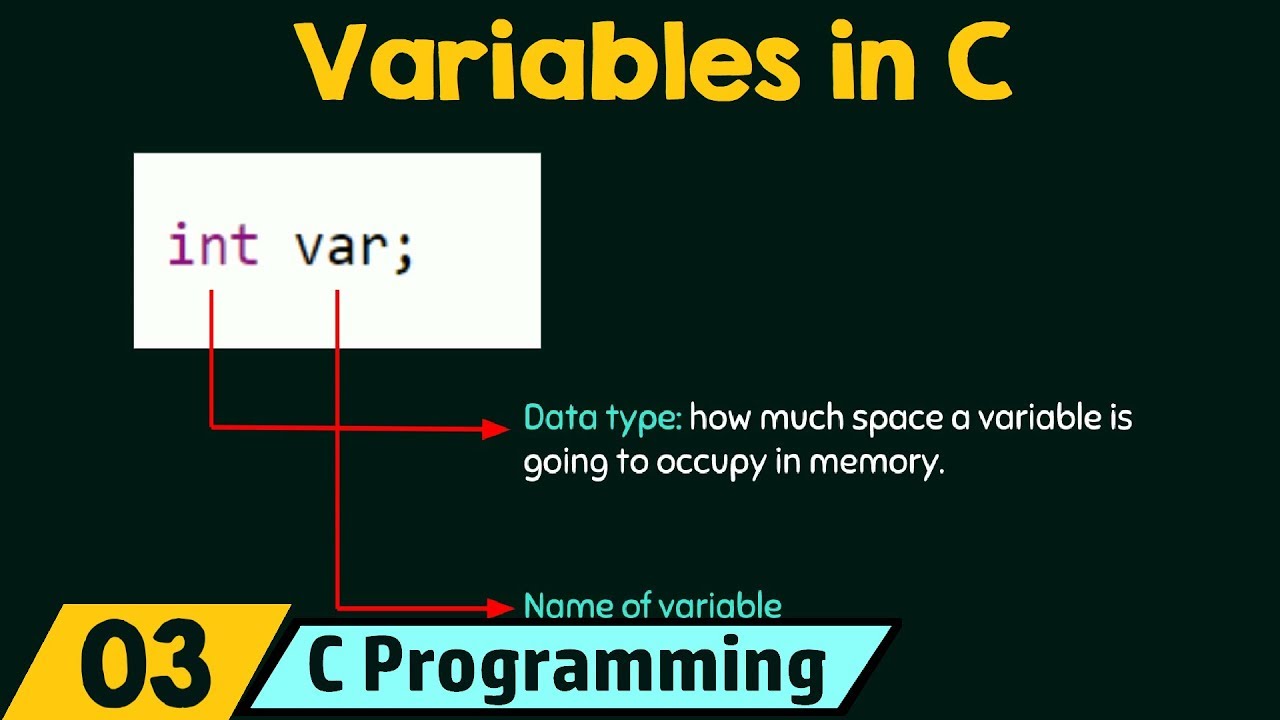
Introduction to Variables
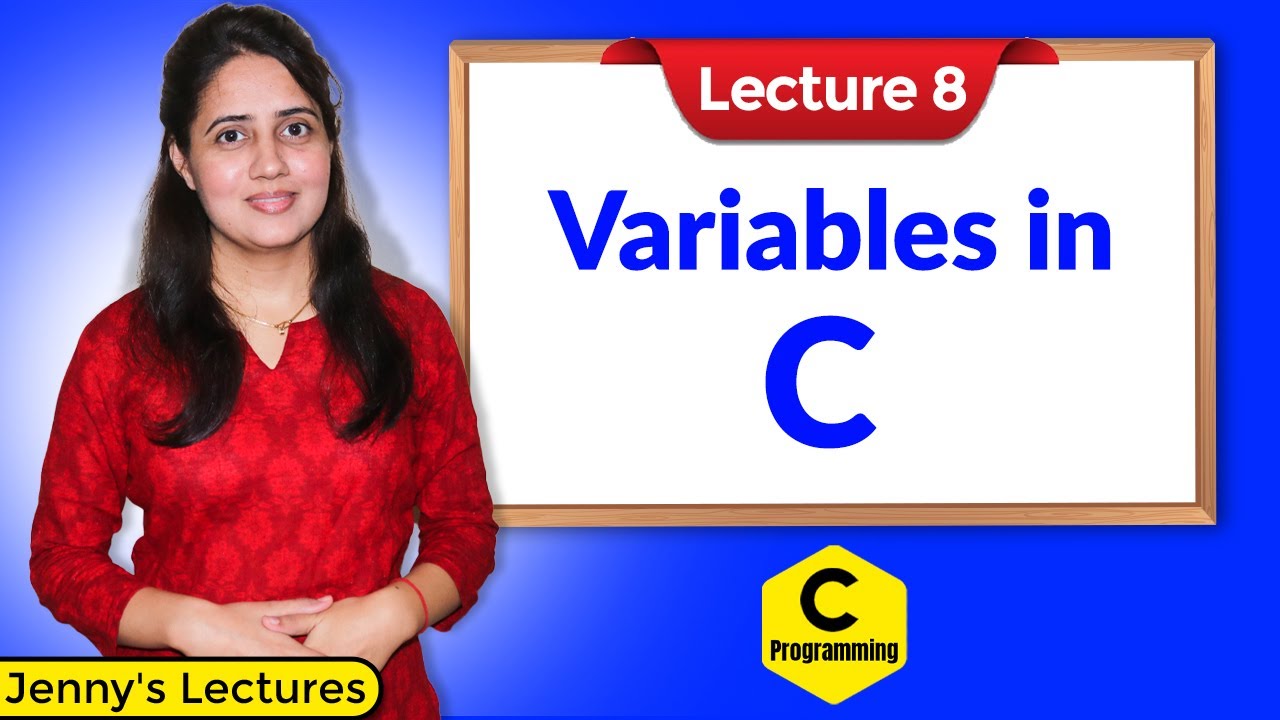
C_08 Variables in C Programming | C Programming Tutorials

Introdução à Programação - Aula 02 - Problemas e Algoritmos

#2 Tipe Data, Variabel, dan Operator
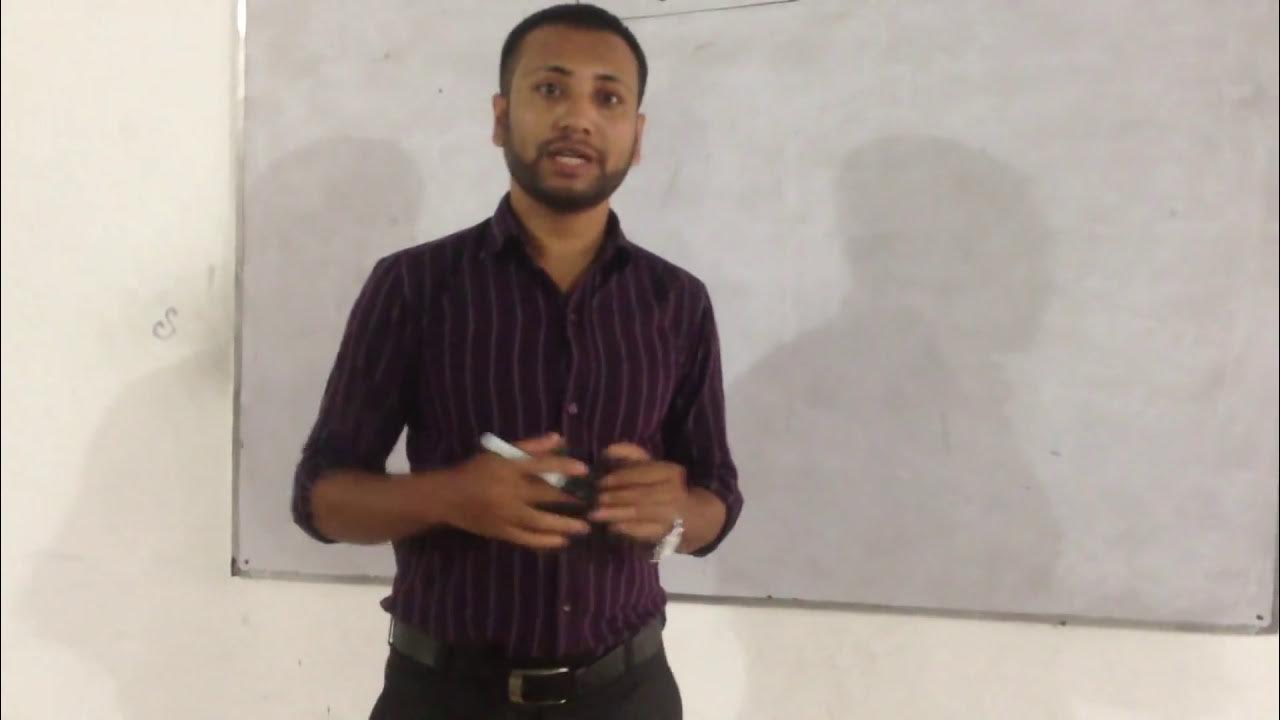
C programming Bangla Tutorial 5.2 : কিভাবে সি প্রোগ্রামিং পড়বেন / পড়াবেন ?
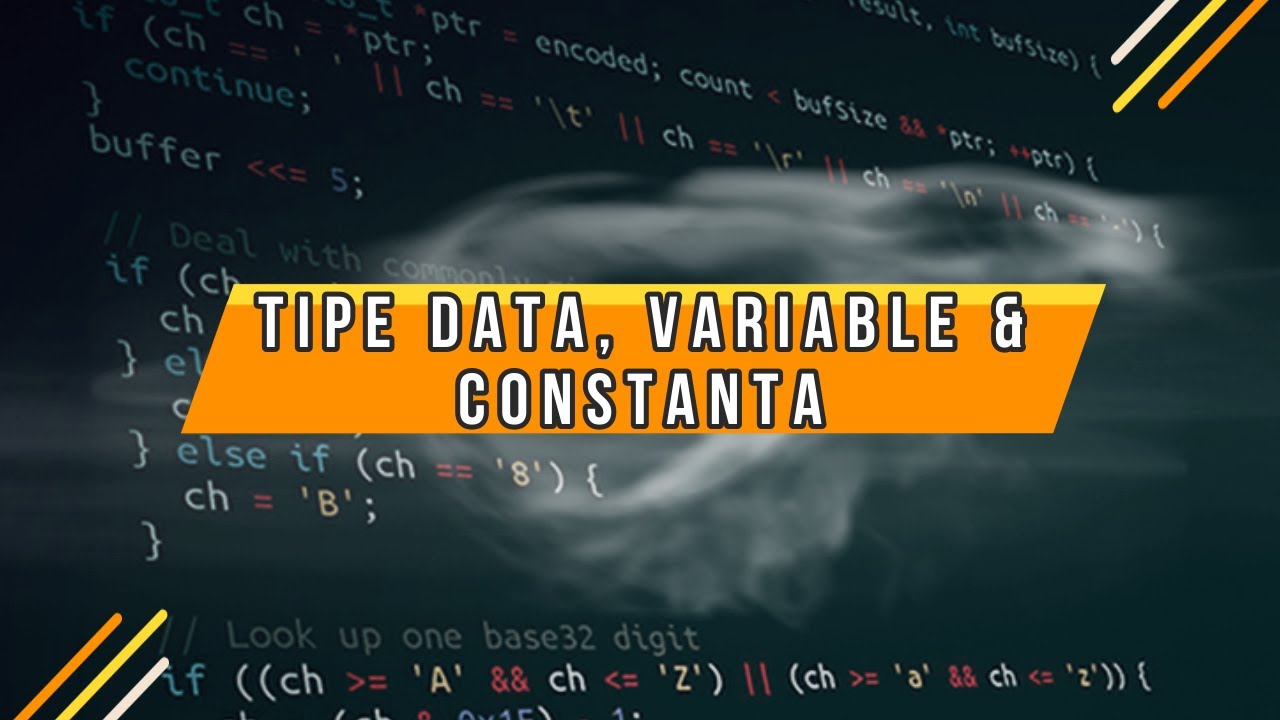
Pemrograman Dasar - Tipe Data, Variable & Constanta
5.0 / 5 (0 votes)