Learn JavaScript Programming Basics: Else If in JavaScript
Summary
TLDRThis video provides an introduction to the 'else if' logic structure in JavaScript, explaining how it is used to evaluate multiple conditions. The script demonstrates how to assign grades based on specific value ranges, such as an 'A' for scores above 90, a 'B' for scores above 80, and a 'C' for scores above 70. It also covers the use of the 'else' condition for values outside these ranges. The tutorial is aimed at beginners and shows how to implement logical conditions in JavaScript effectively.
Takeaways
- 😀 The 'else if' structure in JavaScript is used to evaluate multiple conditions and execute a specific block of code based on which condition is true.
- 😀 The first condition in an 'if' statement is evaluated using a comparison operator. For example, checking if a value is greater than or equal to 90.
- 😀 If the first condition is not met, you can use 'else if' to check additional conditions sequentially.
- 😀 Each 'else if' block can evaluate different ranges or specific conditions (e.g., if a value is between 80 and 89).
- 😀 The 'else' statement is used to handle any case where none of the previous conditions are true.
- 😀 The 'else if' structure is useful for grading systems, where different scores correspond to different grades (A, B, C, etc.).
- 😀 You can use the 'document.write' method in JavaScript to display results in the browser.
- 😀 If the value doesn't meet any of the specified conditions, the 'else' block will execute to handle unexpected or default cases.
- 😀 The example provided uses a grading system where values of 90 or more receive an 'A', 80-89 receive a 'B', and 70-79 receive a 'C'.
- 😀 The example demonstrates how to change the variable's value and refresh the browser to test different conditions and their outputs.
Q & A
What is the purpose of the 'else if' statement in JavaScript?
-The 'else if' statement in JavaScript is used to test multiple conditions. It checks if a specific condition is true and executes a block of code if it is.
How do we define a condition in JavaScript using 'else if'?
-A condition in 'else if' is defined by specifying a comparison operator like '>=' (greater than or equal to) followed by a value. For example, 'if (nilai >= 90)' tests if the value of 'nilai' is greater than or equal to 90.
What happens if the first condition in an 'else if' statement is not met?
-If the first condition is not met, the program will move on to check the next condition in the 'else if' chain, or if no conditions are met, the 'else' block will be executed.
Can 'else if' statements be used to check multiple ranges of values?
-Yes, 'else if' statements are ideal for checking multiple ranges of values, as each 'else if' block can handle a different condition, like checking if 'nilai' is greater than or equal to 90, 80, or 70.
What happens when the value of 'nilai' is 80 in the script?
-If the value of 'nilai' is 80, the 'else if' block checking if 'nilai >= 80' will be true, and the output will be 'nilai B' printed in the browser.
What is the purpose of the 'else' block in this script?
-The 'else' block handles cases where none of the 'if' or 'else if' conditions are met. In this case, if the value of 'nilai' is lower than 70, the 'else' block will print 'Belajar lagi' (study again).
What will happen if the value of 'nilai' is 90 or higher?
-If the value of 'nilai' is 90 or higher, the first condition ('nilai >= 90') will be true, and the output will be 'nilai A'.
How does the script handle a situation where the value of 'nilai' is less than 70?
-If the value of 'nilai' is less than 70, none of the 'if' or 'else if' conditions are satisfied, so the 'else' block is executed, printing 'Belajar lagi'.
What does the 'document.write()' function do in this script?
-The 'document.write()' function writes text directly to the web page. In this script, it is used to display messages like 'nilai A', 'nilai B', and 'Belajar lagi' based on the value of 'nilai'.
Why does the browser not display anything when 'nilai' is 80 in the initial test?
-When 'nilai' is 80, the first condition ('nilai >= 90') is false, so the program moves to the next 'else if' block. However, if 'nilai' is not within any valid condition, nothing is displayed until it matches a condition like 'nilai >= 80' for 'nilai B'.
Outlines
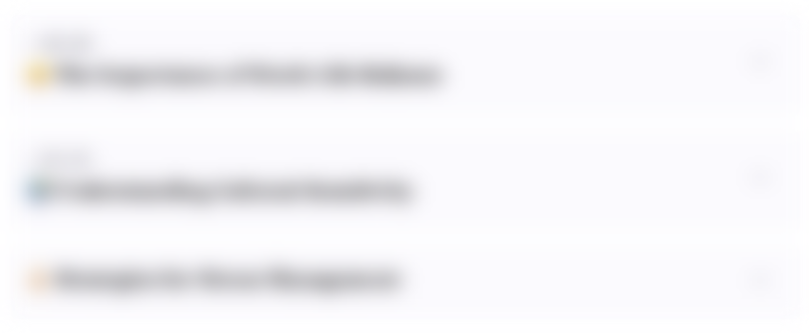
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
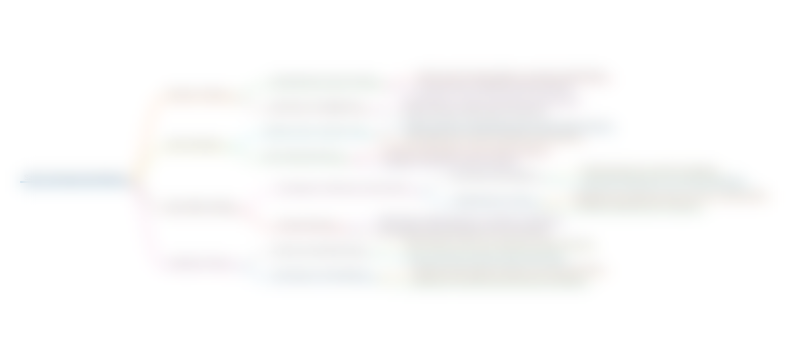
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
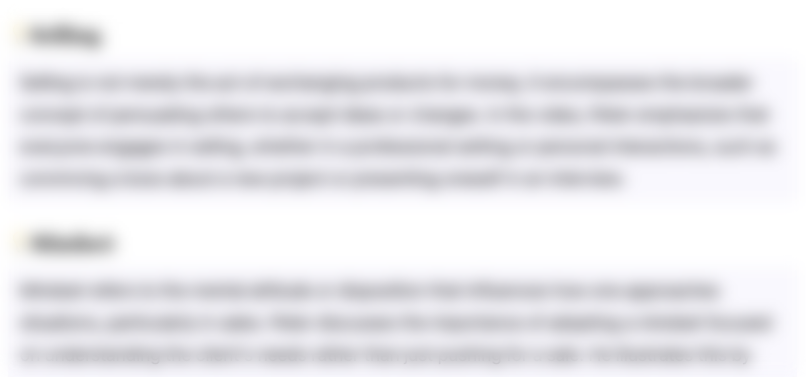
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
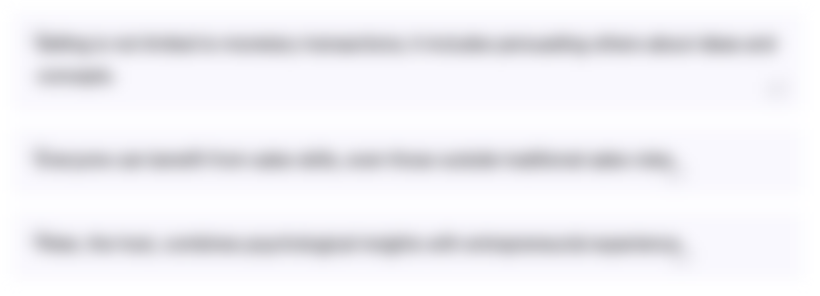
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
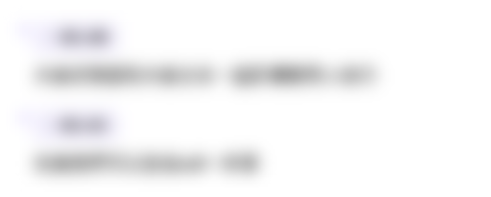
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
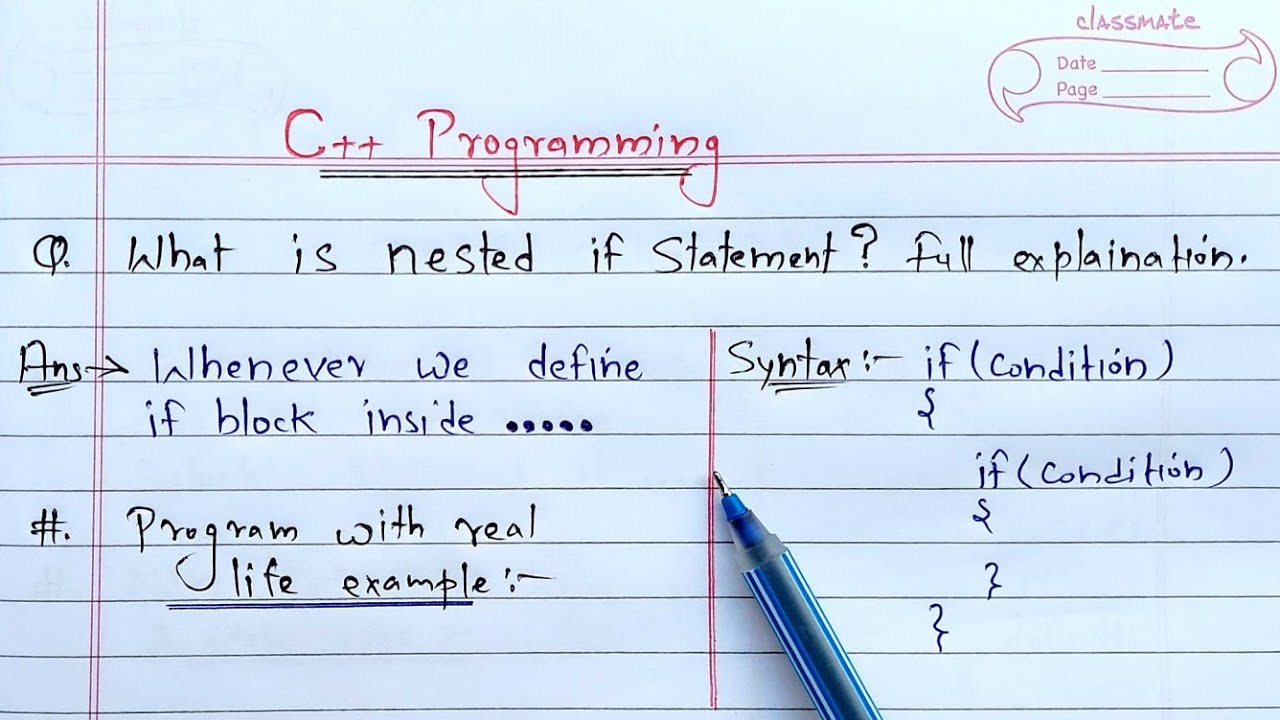
C++ Nested If Statement | Learn Coding

Learn JavaScript Programming Basics: Switch Case Logic Structure
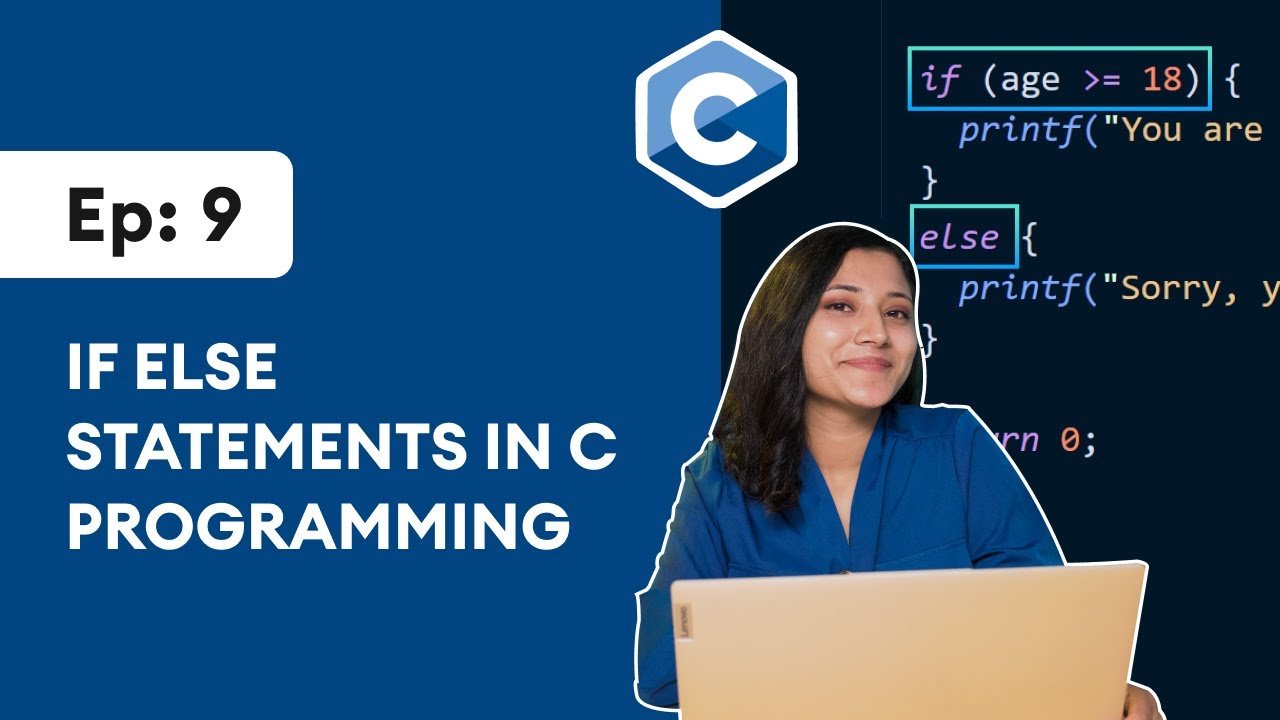
#9: If Else Statements in C | C Programming for Beginners
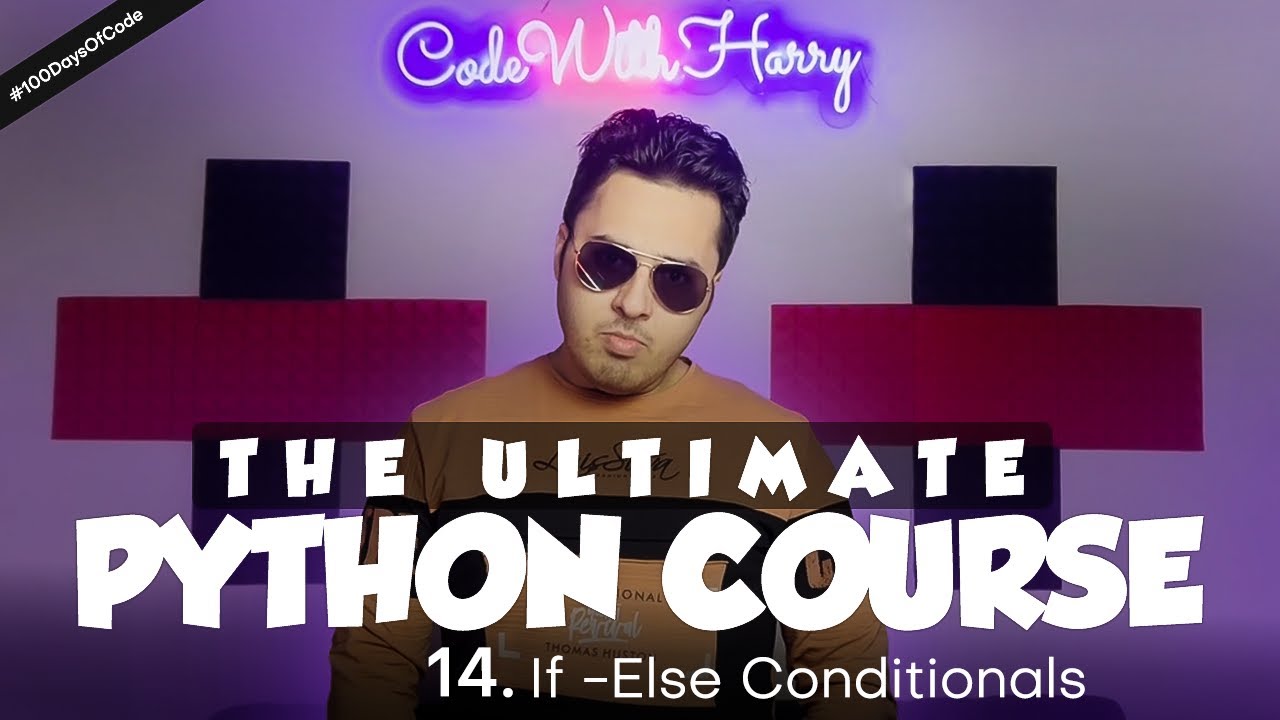
If Else Conditional Statements in Python | Python Tutorial - Day #14
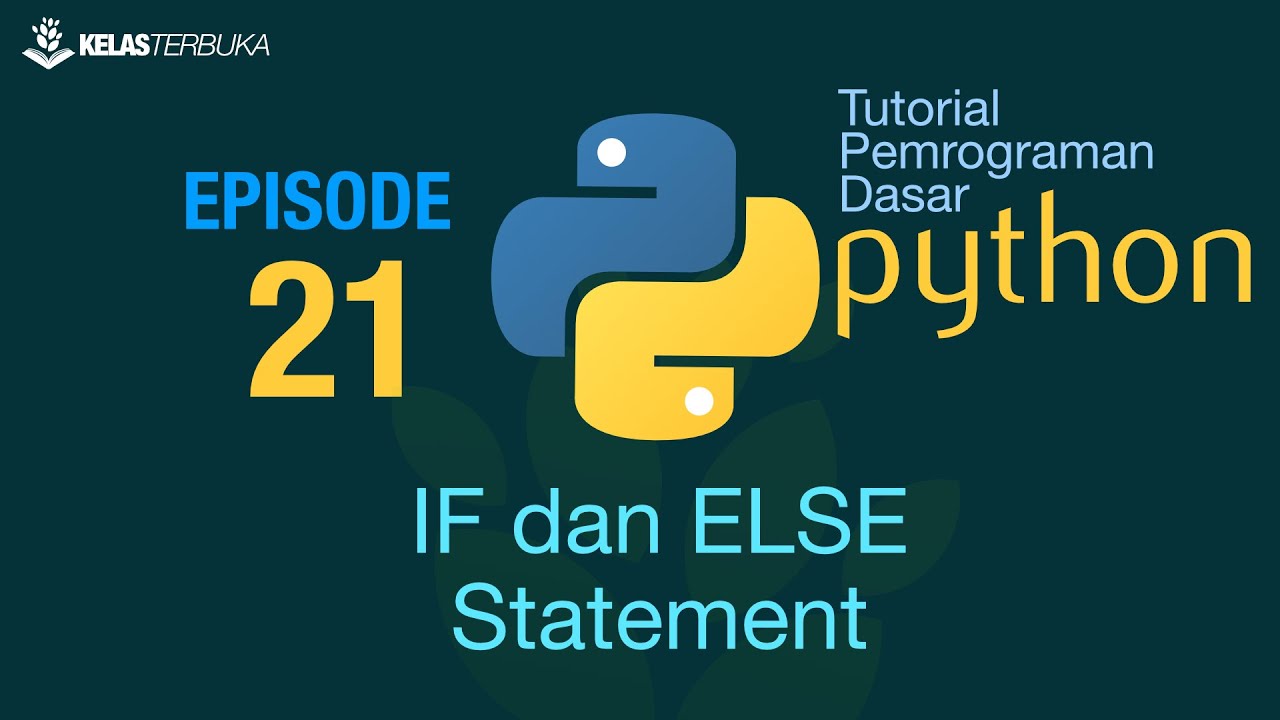
Belajar Python [Dasar] - 21 - IF dan ELSE Statement
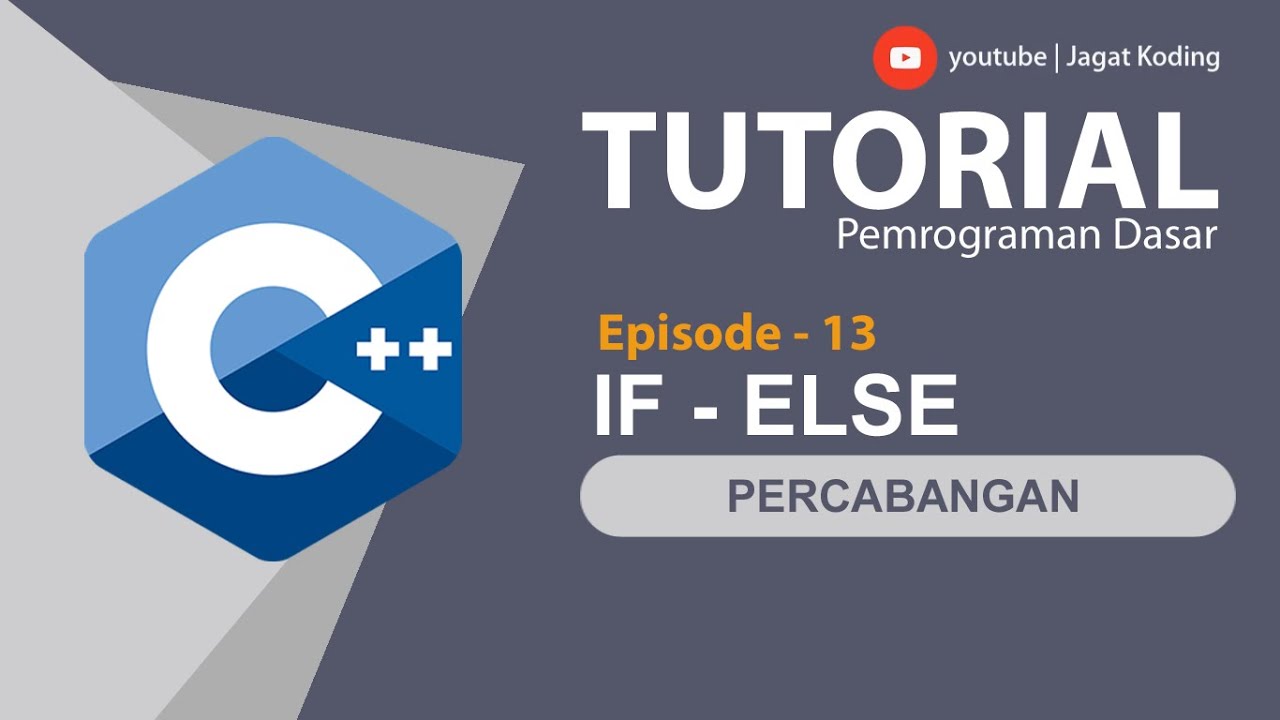
C++ 13 | If Else | Tutorial Percabangan C++
5.0 / 5 (0 votes)