If Else Conditional Statements in Python | Python Tutorial - Day #14
Summary
TLDRThis video provides an in-depth tutorial on Python’s conditional statements, including `if/else`, `elif`, and nested `if/else` structures. The instructor explains how to make decisions in code based on user input, demonstrating through examples such as age checks for driving eligibility and budget comparisons for purchasing apples. The video emphasizes the importance of conditional operators, indentation, and the flow of control in `if/elif/else` structures. It also covers how to handle multiple conditions using `elif` and the concept of nesting `if/else` statements. The tutorial encourages viewers to practice and experiment with code to master decision-making in Python.
Takeaways
- 😀 Decision-making in daily life is similar to using conditional statements in programming (If/Else).
- 😀 The `if/else` statement in Python allows code to be executed based on conditions, with different outcomes for 'True' or 'False'.
- 😀 Conditional operators like `>`, `<`, `==`, and `!=` are used to evaluate expressions in Python, returning a Boolean result.
- 😀 Indentation is crucial in Python as it determines the block structure for `if`, `elif`, and `else` statements, unlike languages that use curly braces.
- 😀 The `elif` statement in Python allows checking multiple conditions in a sequence, offering more flexibility than simple `if/else` statements.
- 😀 In `if/else` statements, the first condition that evaluates to `True` will execute, and the remaining conditions are ignored.
- 😀 You can nest `if/else` statements inside each other, enabling complex conditional logic within a program.
- 😀 To handle more than two conditions, `elif` helps by providing additional conditions between `if` and `else`.
- 😀 The importance of testing your code is emphasized, as practicing repeatedly helps solidify programming concepts, especially conditional statements.
- 😀 It's critical to avoid naming Python scripts after Python keywords (like `if` or `else`) to prevent conflicts or errors in code execution.
Q & A
What is the purpose of using `if/else` statements in Python?
-The `if/else` statement in Python is used for decision making. It allows the program to execute different blocks of code based on whether a specified condition is true or false.
What does the `elif` statement do in Python?
-`elif` (else if) allows you to check multiple conditions. If the condition in the `if` statement is not true, the program moves to check the condition in the `elif` block. If none of the conditions match, the `else` block is executed.
Why is `Indentation` important in Python?
-Indentation in Python is used to define code blocks. Unlike languages like C or Java that use curly braces `{}`, Python uses indentation to determine what code belongs to a particular `if`, `else`, or loop block. Improper indentation will result in an error.
What are the common conditional operators in Python?
-The common conditional operators in Python are: `>`, `<`, `>=`, `<=`, `==` (equal to), and `!=` (not equal to). These operators help to compare values and determine the flow of control in an `if/else` statement.
How does the `input()` function work in Python?
-The `input()` function in Python reads input from the user as a string. To convert this input into an integer or another type, you can use type casting functions such as `int()` for integers.
What happens when an `if` condition evaluates to `True`?
-When an `if` condition evaluates to `True`, the block of code inside the `if` statement is executed. If the condition evaluates to `False`, the program moves to the `else` block, if it exists.
What is a `nested if/else` statement?
-A `nested if/else` statement refers to placing one `if` or `else` statement inside another. This allows for more complex decision-making by evaluating multiple conditions at different levels.
What is the difference between `if/else` and `if/elif/else`?
-The `if/else` statement is used when you have two options to choose from. The `if/elif/else` chain is used when you need to check for multiple conditions. `elif` allows you to add more conditions between the `if` and `else` blocks.
How does Python handle comparison for equality and inequality?
-In Python, `==` is used to check if two values are equal, while `!=` is used to check if two values are not equal. These are fundamental comparison operators used in conditional statements.
Can we use logical operators like `and` and `or` in `if/else` statements?
-Yes, logical operators like `and` and `or` can be used in `if/else` statements to combine multiple conditions. For example, `if age > 18 and budget > 100:` will only execute the block if both conditions are true.
Outlines
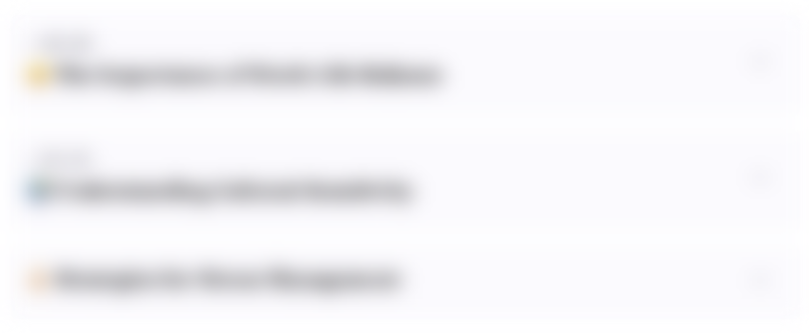
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
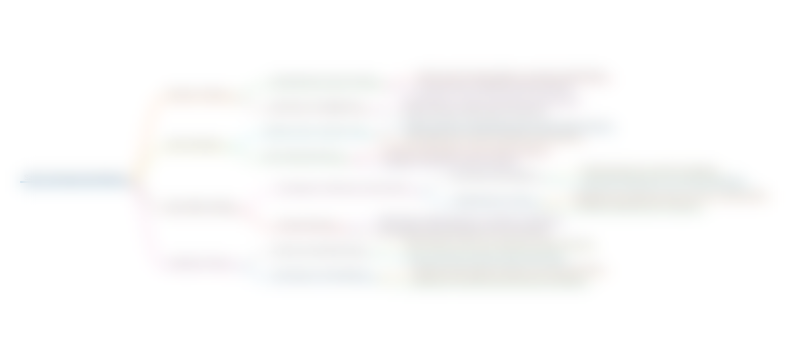
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
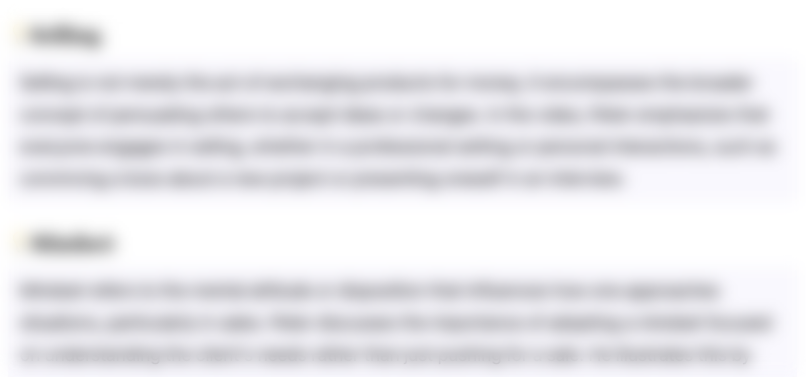
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
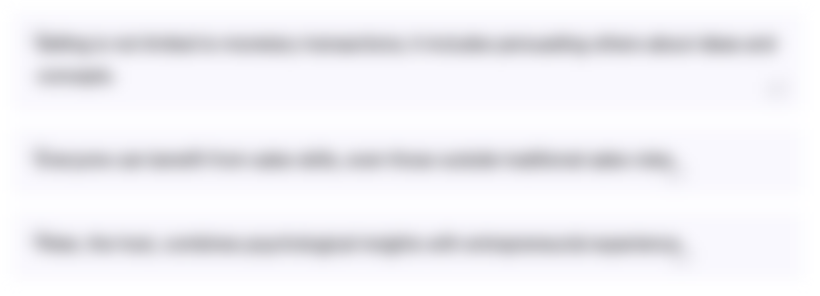
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
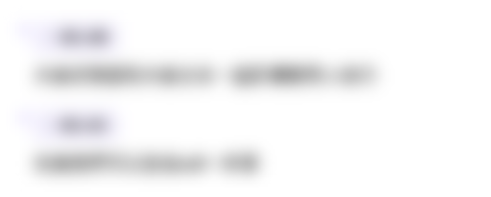
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)