Curso de C++ #62 - Map - Smart Pointer / Ponteiro Inteligente - C++11 - (C++ Moderno)
Summary
TLDRIn this tutorial, the instructor, Professor Bruno, introduces the concept of smart pointers in C++ and their key benefits over regular pointers. He explains how smart pointers automatically manage memory, preventing memory leaks and ensuring objects are properly destroyed, especially when exceptions occur. The video demonstrates the use of unique pointers (unique_ptr) and shared pointers (shared_ptr), highlighting their differences and usage scenarios. Additionally, the instructor walks through practical code examples, showing how to implement these smart pointers and avoid manual memory management errors. This tutorial provides a clear, practical guide for leveraging smart pointers in C++.
Takeaways
- 😀 Smart pointers in C++ automatically manage memory allocation and deallocation.
- 😀 Using smart pointers avoids manual memory management, reducing the risk of memory leaks.
- 😀 `unique_ptr` ensures that an object has only one owner, automatically releasing memory when it goes out of scope.
- 😀 `shared_ptr` allows multiple pointers to share ownership of an object and automatically deletes the object when all pointers are out of scope.
- 😀 One key advantage of smart pointers is exception safety, ensuring proper cleanup even in the event of exceptions.
- 😀 The traditional approach with regular pointers requires explicit memory management using `delete` or `free`.
- 😀 Smart pointers eliminate the need to manually call `delete`, simplifying code and improving reliability.
- 😀 `unique_ptr` cannot be shared, while `shared_ptr` can be used by multiple owners, allowing more flexible memory sharing.
- 😀 Using `unique_ptr` prevents multiple references to the same object, which can avoid bugs and unintended behaviors.
- 😀 Smart pointers simplify the creation of objects, with `std::make_unique` and `std::make_shared` providing easy-to-use constructors for pointer allocation.
- 😀 The lesson highlights how using smart pointers over regular pointers can lead to cleaner, safer, and more maintainable code.
Q & A
What is the main advantage of using smart pointers in C++ over regular pointers?
-The main advantage of using smart pointers in C++ is that they automatically manage the memory associated with objects. They ensure that memory is freed when no longer needed, preventing memory leaks and dangling pointers, which can occur with regular pointers.
How do smart pointers handle memory management in the case of exceptions?
-Smart pointers automatically manage memory and ensure that objects are properly destroyed, even in the event of an exception. Unlike regular pointers, which may leave memory allocated when an exception occurs, smart pointers guarantee that the memory is freed, preventing memory leaks.
What is the difference between a `std::unique_ptr` and a `std::shared_ptr`?
-A `std::unique_ptr` ensures exclusive ownership of an object, meaning only one pointer can point to the object at any given time. On the other hand, a `std::shared_ptr` allows multiple pointers to share ownership of the same object. The object is deleted only when the last `shared_ptr` is destroyed.
Why does the `std::unique_ptr` not allow multiple pointers to share ownership of the same object?
-The `std::unique_ptr` is designed for exclusive ownership, ensuring that there is only one pointer managing the object's memory. This prevents issues like double-deletion and ensures a clear ownership structure. If you try to assign a `unique_ptr` to another one, the program will raise an error.
What happens when you try to assign one `std::unique_ptr` to another?
-When you try to assign one `std::unique_ptr` to another, it will result in a compilation error. This is because `std::unique_ptr` enforces exclusive ownership, meaning you cannot have two pointers owning the same object simultaneously.
What does the `std::make_unique` function do in C++?
-The `std::make_unique` function is a safer and more efficient way to create a `std::unique_ptr`. It allocates memory for the object and initializes it, ensuring proper memory management. It also avoids potential issues with manual memory allocation using `new`.
Can `std::shared_ptr` be used with raw pointers, and if so, how does it affect memory management?
-Yes, `std::shared_ptr` can be used with raw pointers by using it to manage the lifetime of dynamically allocated objects. The shared pointer will take ownership and automatically delete the object when it is no longer needed, avoiding manual memory management and the risk of memory leaks.
How does the use of `std::shared_ptr` help avoid memory leaks?
-`std::shared_ptr` helps avoid memory leaks by automatically freeing the memory of the object it manages when the last `shared_ptr` pointing to that object goes out of scope. It uses reference counting to track how many shared pointers are pointing to an object, ensuring memory is cleaned up when no longer needed.
Why is it important to use smart pointers instead of raw pointers in modern C++ development?
-Using smart pointers in modern C++ is crucial for ensuring safe and efficient memory management. Smart pointers handle memory allocation and deallocation automatically, preventing common errors such as memory leaks, dangling pointers, and double deletions, which can be challenging to manage with raw pointers.
What happens when you attempt to assign a `std::unique_ptr` to a `std::shared_ptr`?
-You cannot directly assign a `std::unique_ptr` to a `std::shared_ptr`, as they represent different types of ownership models. While `std::unique_ptr` implies exclusive ownership, `std::shared_ptr` allows shared ownership. The two types are not compatible in terms of ownership, and such an assignment will result in a compilation error.
Outlines
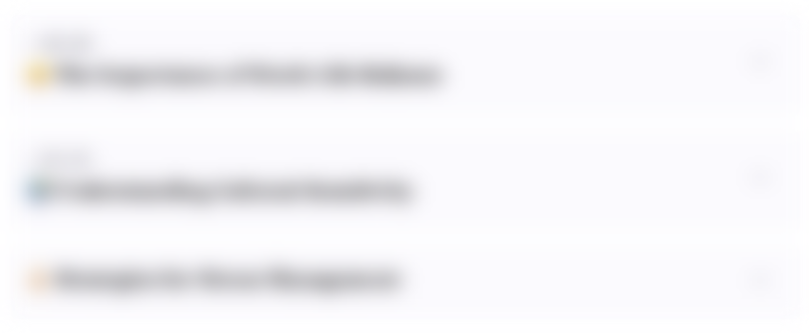
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
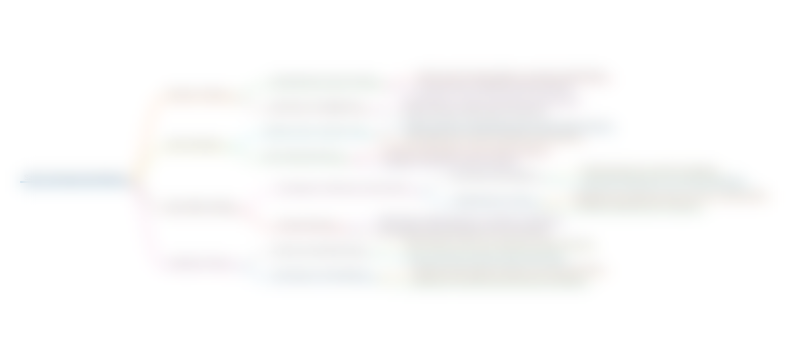
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
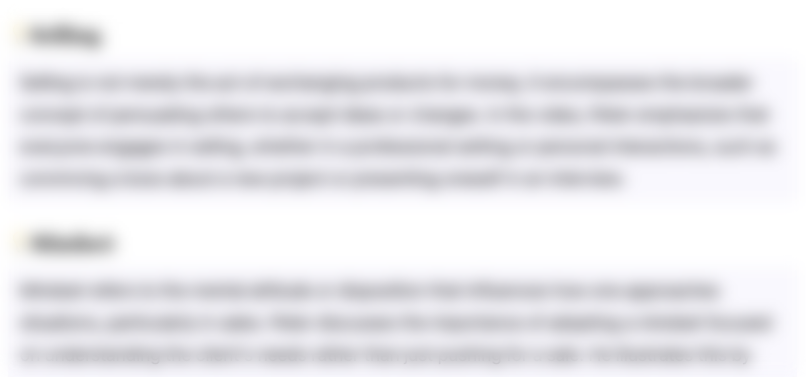
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
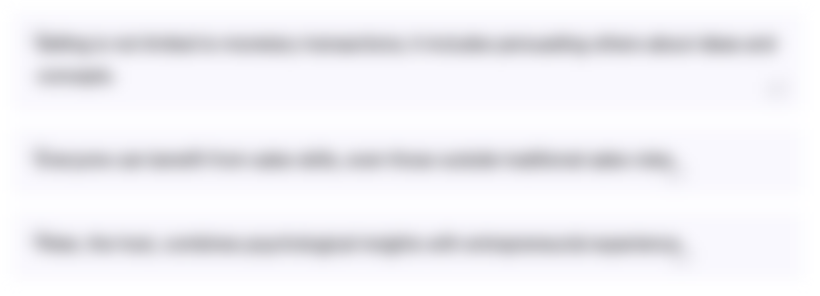
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
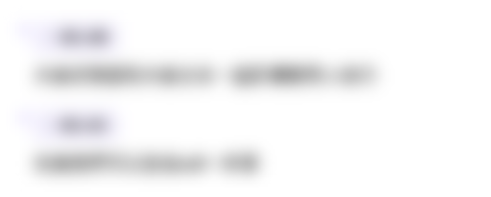
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
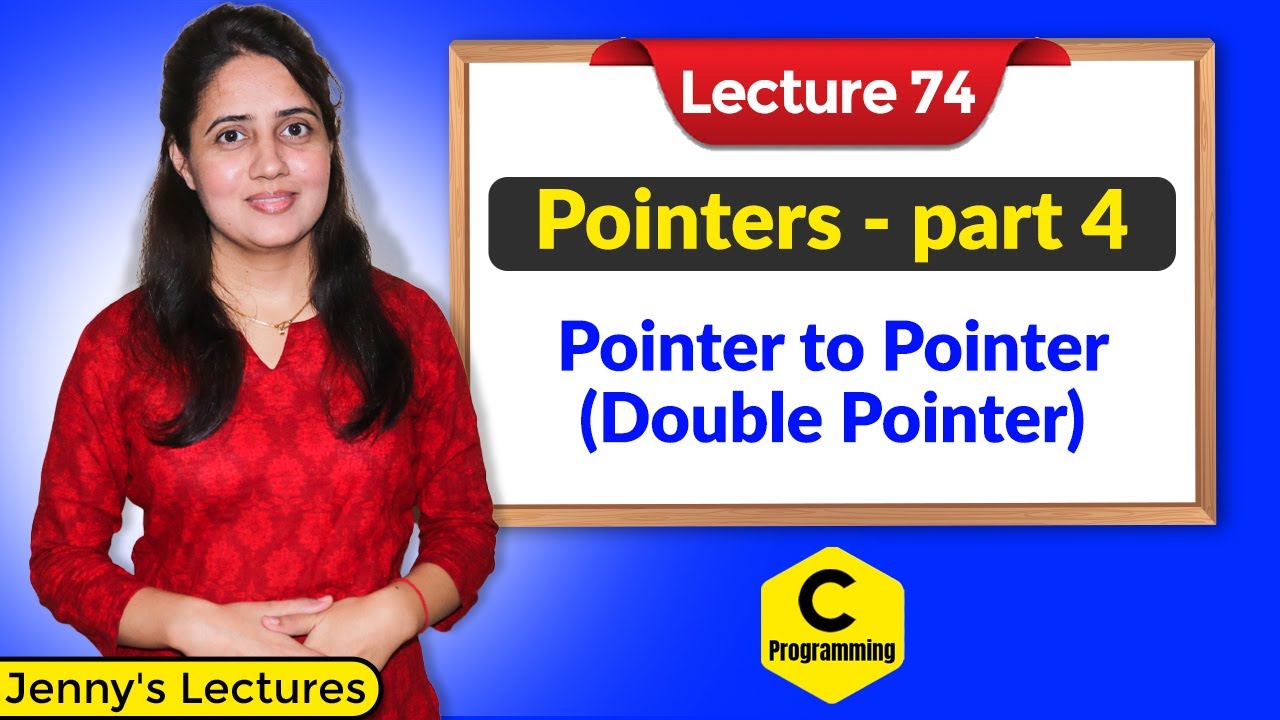
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
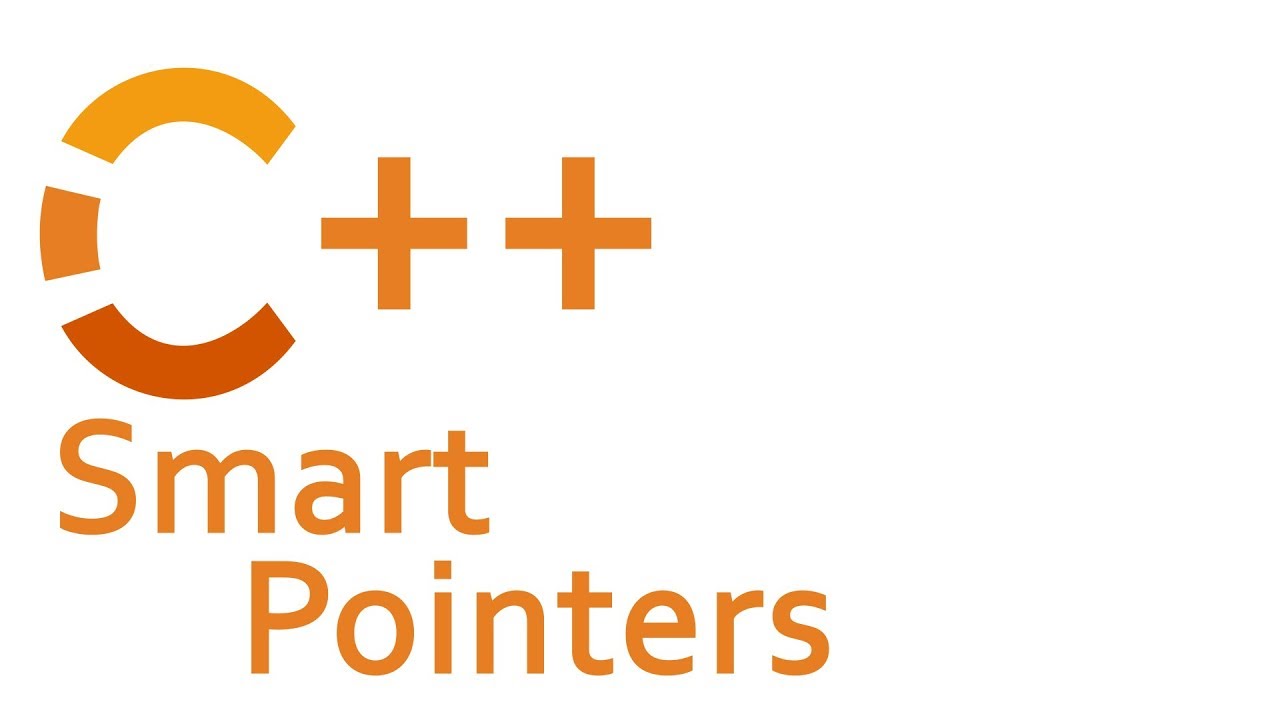
SMART POINTERS in C++ (std::unique_ptr, std::shared_ptr, std::weak_ptr)
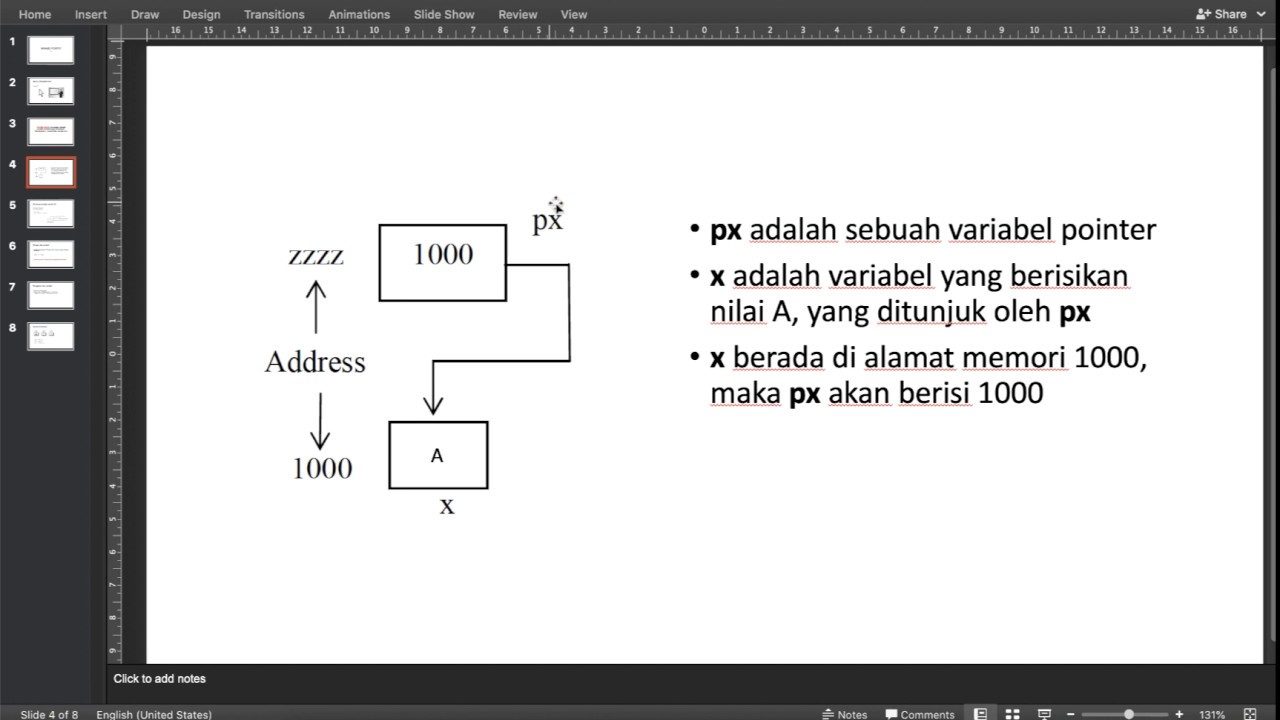
Variabel Pointer

C_99 Returning Pointer from Function in C | C Language Tutorials
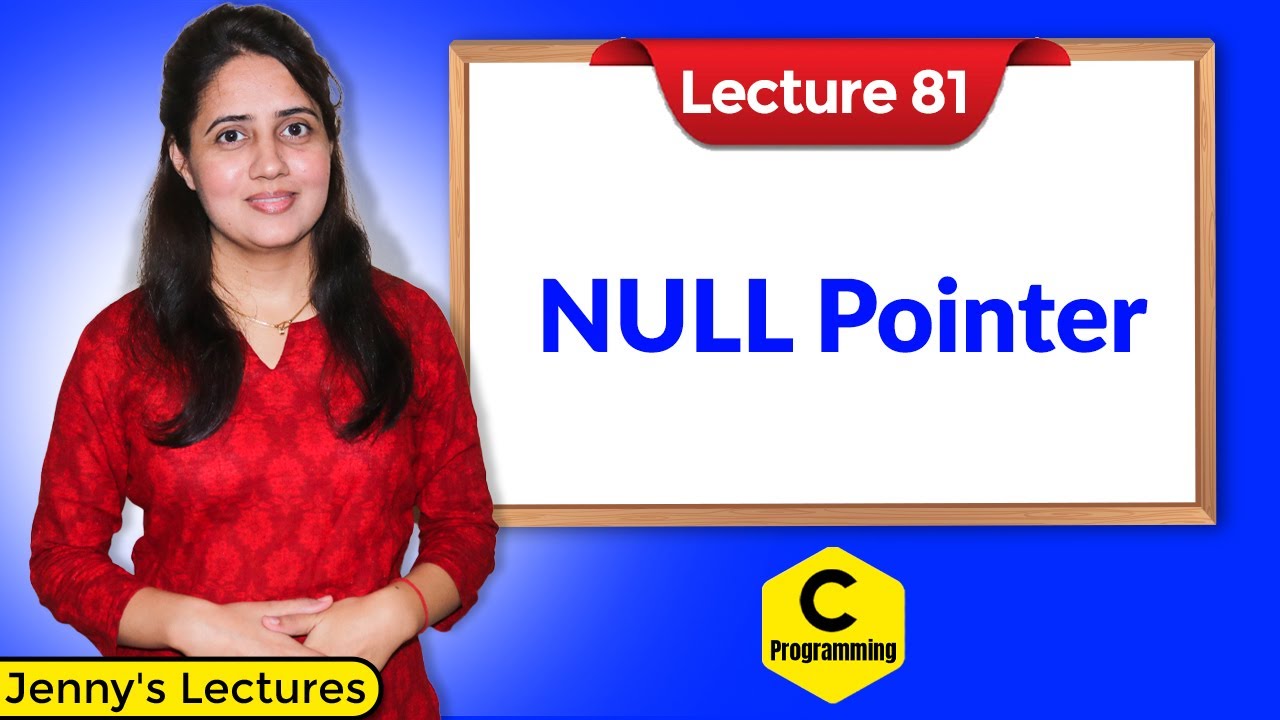
C_81 Null Pointer in C | C Programming Tutorials

DAY 30 | COMPUTER SCIENCE | II PUC | POINTERS | L1
5.0 / 5 (0 votes)