Variabel Pointer
Summary
TLDRIn this video, viewers are introduced to the concept of pointers in C programming. The tutorial explains how pointers are variables that store memory addresses of other variables, rather than values. It covers how to declare, assign, and use pointers to manipulate data, including dereferencing to access or modify values. The video also explores advanced topics like pointers to pointers, providing practical examples to demonstrate how pointers are utilized in real programming scenarios. This guide is perfect for anyone seeking to understand memory management and improve their skills in C programming.
Takeaways
- π Pointer variables store the memory address of other variables, not their actual values.
- π A pointer is declared using an asterisk (*) symbol, indicating it stores the address of another variable.
- π The & operator is used to get the address of a variable and assign it to a pointer.
- π Pointers enable indirect access to variables by using the dereference operator (*).
- π The value of a variable can be modified indirectly using pointers.
- π A pointer's data type must match the type of the variable it points to.
- π When using a pointer to modify a variable's value, the dereference operator (*) is required.
- π Pointers can point to other pointers, creating a chain of indirect memory access (pointer to pointer).
- π A pointer must be properly initialized with a valid memory address before accessing or modifying values.
- π The distinction between pointer and non-pointer variables is crucial for understanding memory handling in C programming.
- π Understanding pointers is essential for dynamic memory management and efficient programming in languages like C.
Q & A
What is a pointer variable in programming?
-A pointer variable is a variable that stores the memory address of another variable, rather than the value itself. It 'points' to the location in memory where the data is stored.
How do pointer variables differ from regular variables?
-Regular variables store actual values like integers, characters, or booleans, while pointer variables store memory addresses of other variables. Essentially, pointer variables point to the location where the data is held, rather than the data itself.
What is the syntax for declaring a pointer variable in C?
-In C, a pointer variable is declared by placing an asterisk (*) before its name, followed by the variable's type. For example, 'int *ptr;' declares a pointer to an integer.
What does the asterisk (*) signify in pointer variable declarations?
-The asterisk (*) in a pointer declaration indicates that the variable is a pointer. It tells the compiler that the variable will store the memory address of another variable, not the variable's value.
How do you assign a memory address to a pointer variable?
-To assign a memory address to a pointer variable, use the address-of operator (&) in front of a variable. For example, 'ptr = &x;' assigns the address of variable 'x' to the pointer 'ptr'.
What is the role of the ampersand (&) operator in pointer assignment?
-The ampersand (&) operator is used to obtain the memory address of a variable. It is essential for assigning the address of a variable to a pointer, as in 'ptr = &x;', where 'ptr' will store the address of 'x'.
How can you access the value stored at the memory address pointed to by a pointer?
-To access the value stored at the memory address pointed to by a pointer, use the dereference operator (*). For example, 'int value = *ptr;' will store the value at the memory address 'ptr' points to into 'value'.
What does dereferencing a pointer mean?
-Dereferencing a pointer means accessing the value stored at the memory address the pointer is pointing to. This is done using the asterisk (*) operator, such as '*ptr'.
Can pointer variables be used to modify the value of other variables? How?
-Yes, pointer variables can modify the values of other variables by dereferencing them and assigning new values. For example, '*ptr = 10;' changes the value of the variable that 'ptr' points to.
What is the significance of using pointer variables in C programming?
-Pointer variables are essential in C programming for dynamic memory allocation, efficient data manipulation, and managing large data structures. They allow direct memory access and modification, which can lead to more efficient and flexible code.
Outlines
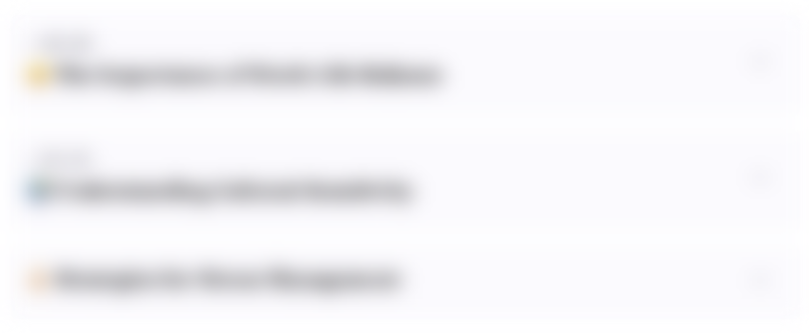
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
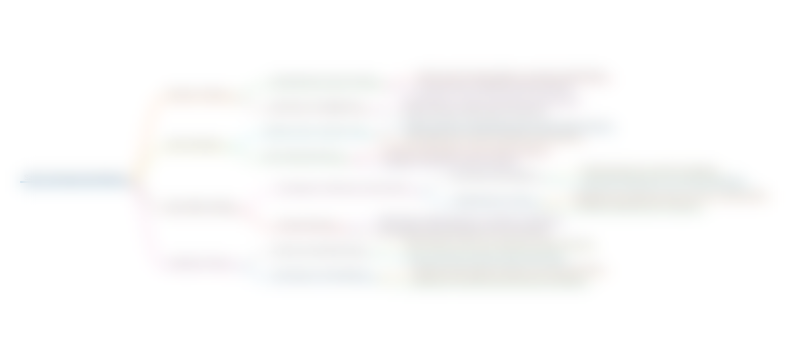
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
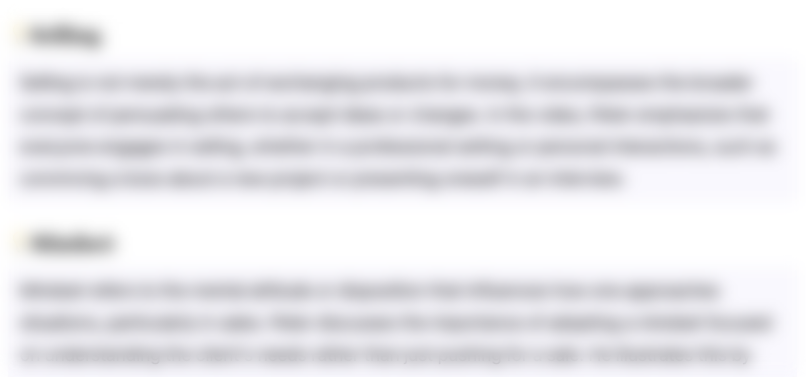
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
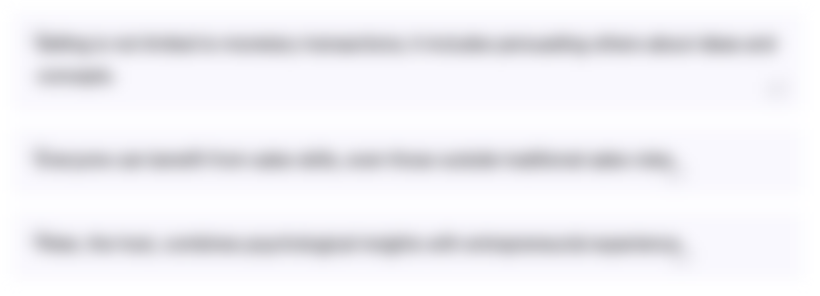
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
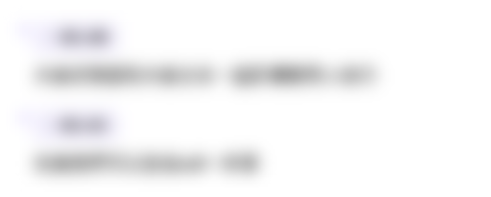
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)