Understanding and Using Function Pointers in C
Summary
TLDRThis video script delves into the concept of function pointers in C programming, which can be daunting due to C's complex syntax. It explains the 'right-left rule' for declaring function pointers, emphasizing the placement of the identifier in the middle. The script illustrates how function pointers allow for passing functions as arguments, enabling dynamic function calls at runtime. It also recommends using typedefs to simplify complex declarations, making the code more readable and maintainable. The tutorial aims to demystify function pointers and enhance programming flexibility.
Takeaways
- 📚 A function pointer in C is a variable that stores the address of a function, allowing for dynamic function calls at runtime.
- 😵 The syntax for function pointers can be confusing due to the placement of the identifier in the middle, which deviates from the standard type-name pattern.
- 👉 The 'right-left rule' is introduced to help understand the declaration of function pointers: start with the identifier, then follow the type information to the right and left.
- 🔍 When declaring a function pointer, the type information is nested, with the function's return type and parameter list specified before the identifier.
- 🎯 Function pointers enable passing functions as arguments to other functions, providing flexibility in how functions are called within a program.
- 🔄 Using function pointers allows for reusing code with different operations, such as adding, subtracting, or multiplying integers, by passing different function pointers.
- 🛠️ The video recommends using typedefs to create more readable and manageable declarations for function pointers, improving code clarity.
- 👨🏫 For students and developers, understanding function pointers is crucial for advanced C programming, especially when dealing with dynamic function calls.
- 💡 The video serves as an educational resource, aiming to demystify function pointers and encourage their use in creating flexible and efficient C programs.
- ✌️ The presenter concludes by encouraging viewers to enjoy the process of learning and using function pointers, indicating the session's end.
Q & A
What is a function pointer in C?
-A function pointer in C is a variable that stores the address of a function, allowing you to call that function later on. It's a way to reference a function as if it were a variable.
Why can function pointers be confusing for students learning C?
-Function pointers can be confusing because C's syntax places the identifier in the middle of the declaration, which is different from the usual left-to-right type and name ordering seen in variable declarations.
What is the 'right-left rule' mentioned in the script for reading function pointer declarations?
-The 'right-left rule' is a method for reading function pointer declarations by first identifying the identifier, then looking to the right to determine it's a pointer, and then looking to the left to understand the function's parameters and return type.
How does the 'right-left rule' help in understanding complex function pointer declarations?
-The 'right-left rule' simplifies the process of reading complex function pointer declarations by breaking it down into manageable parts, starting with the identifier, then the pointer, and finally the function's signature.
What is the purpose of passing function pointers as arguments to other functions?
-Passing function pointers as arguments allows for dynamic function calls within other functions. This means the specific function to be called can be determined at runtime, not just at compile time, adding flexibility.
Can you provide an example of how function pointers can be used to perform different operations on integers?
-Function pointers can be used to define a function that performs an operation on two integers, where the specific operation (like addition, subtraction, or multiplication) is determined by the function pointer passed to it.
What is the benefit of using function pointers in a program?
-Using function pointers allows for more dynamic and flexible code, as it enables functions to be called based on runtime decisions rather than being hard-coded, thus promoting code reuse and modularity.
Why are typedefs recommended when dealing with function pointers?
-Typedefs are recommended for function pointers because they make the declarations more readable by putting the type information on the left and the identifier on the right, which is more consistent with typical C syntax and easier to understand.
How do typedefs simplify the process of declaring function pointers?
-Typedefs simplify declaring function pointers by creating a new type that represents the function pointer, which can then be used in a more straightforward manner, making the code cleaner and easier to read.
What is the main takeaway from the script regarding the use of function pointers in C?
-The main takeaway is that while function pointers can be initially confusing due to C's syntax, understanding the 'right-left rule' and using typedefs can make them a powerful tool for creating flexible and dynamic code.
Outlines
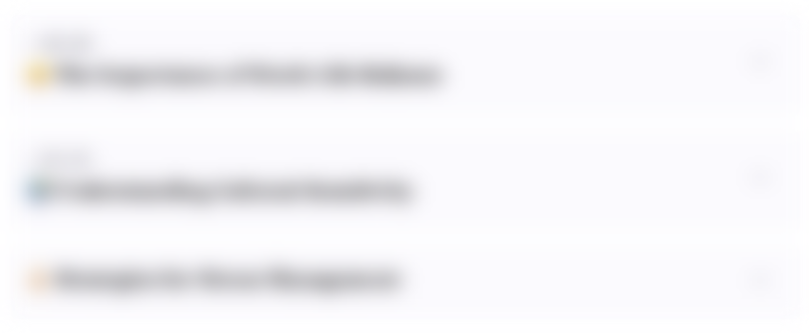
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
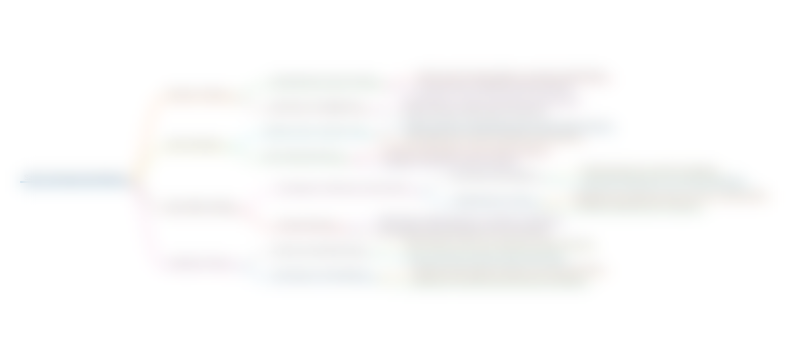
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
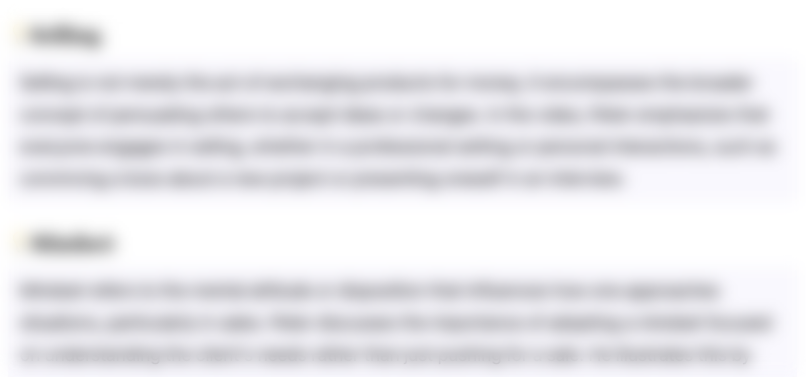
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
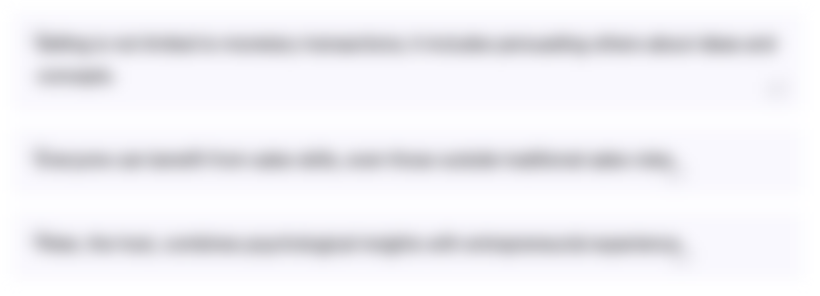
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
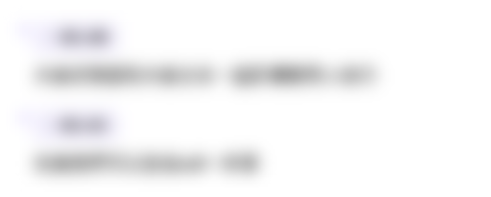
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)