If Else Statements in Python | Python for Beginners
Summary
TLDRThis video explains how to use the if-elif-else statement in Python. The instructor demonstrates how to create conditional statements that check whether certain conditions are true or false, using comparison and logical operators. The video walks through writing code for various conditions, including nested if statements and one-line if-else expressions. It also highlights how the Python interpreter evaluates these conditions step-by-step. The guide aims to help viewers understand the structure and flexibility of Python's conditional statements, making it ideal for those learning programming basics.
Takeaways
- 😀 The video covers the if-else statement in Python programming.
- 🤓 The if-else statement evaluates conditions and executes a body of code if true or false.
- 📊 The 'elif' statement checks a secondary condition if the initial 'if' condition is false.
- 🔁 You can have multiple 'elif' statements, but only one 'if' and one 'else' statement in a block.
- 💻 An example was shown where 'if 25 is greater than 10' evaluates as true and prints 'it worked'.
- 🔎 If a condition in 'if' is false, the code moves to the 'else' block for execution.
- 📝 You can chain multiple conditions with 'elif', as demonstrated in the 25, 30, 40, and 50 comparisons.
- ⚙️ Logical operators like 'and' or 'or' can be used inside the condition statements for more complex logic.
- 🚀 Python also allows writing if-else statements in a single line for concise conditions.
- 🧩 Nested if statements are possible, where an if statement is placed within another, allowing more intricate decision-making.
Q & A
What is the basic structure of an if-else statement in Python?
-An if-else statement starts with an 'if' condition. If the condition is true, a block of code is executed. If it's false, the 'else' block of code runs.
What is the purpose of the 'elif' statement in Python?
-'Elif' (short for 'else if') checks another condition if the previous 'if' condition is false. You can have multiple 'elif' statements in a single if-else structure.
How many 'if' and 'else' statements can be used in a Python if-else structure?
-You can only have one 'if' statement and one 'else' statement in a structure, but you can have multiple 'elif' statements between them.
What happens when an 'if' statement's condition is true?
-If the condition is true, Python executes the block of code under the 'if' statement and skips any 'elif' or 'else' blocks.
What happens if all conditions in the if-elif structure are false?
-If all conditions in the 'if' and 'elif' statements are false, the code in the 'else' block is executed.
How does Python handle indentation in if-else statements?
-When writing an if-else statement, Python automatically indents the code inside the body of the statement after a colon (':'). Proper indentation is crucial for defining code blocks.
Can you use multiple comparison operators in an if statement?
-Yes, you can use logical operators like 'and' or 'or' to combine multiple conditions in a single if statement.
How does Python handle a one-line if-else statement?
-Python allows if-else statements to be written on one line using the syntax: 'print(output_if_true) if condition else print(output_if_false)'.
What is a nested if statement?
-A nested if statement is an if statement inside another if statement. It allows for multiple levels of condition checks, creating more complex logic.
What happens when a condition in the elif chain becomes true?
-When a condition in an 'elif' statement becomes true, its corresponding block of code is executed, and the rest of the 'elif' and 'else' statements are skipped.
Outlines
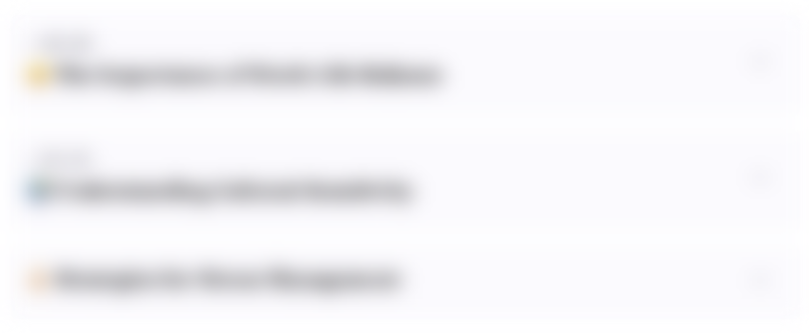
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
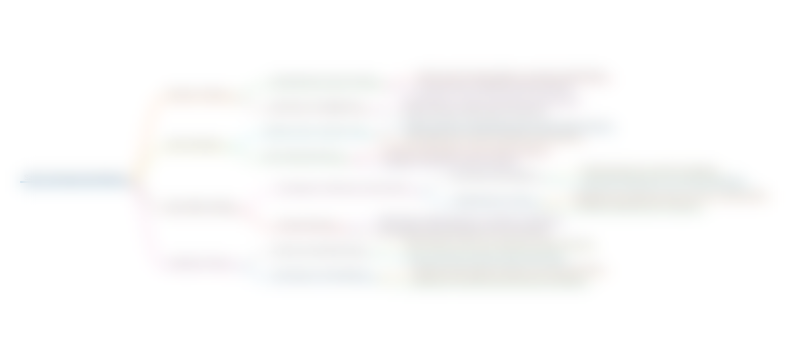
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
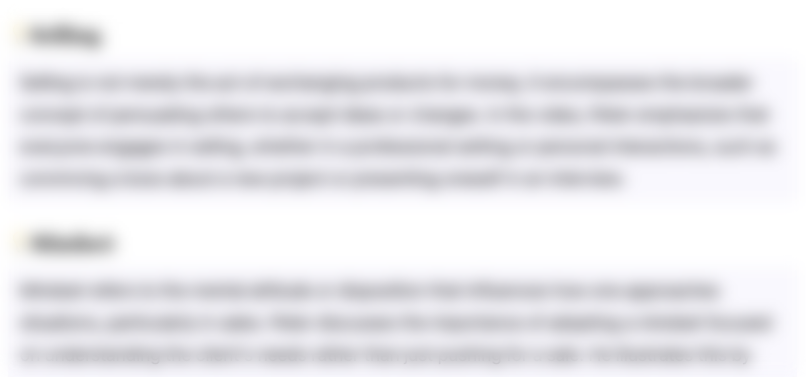
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
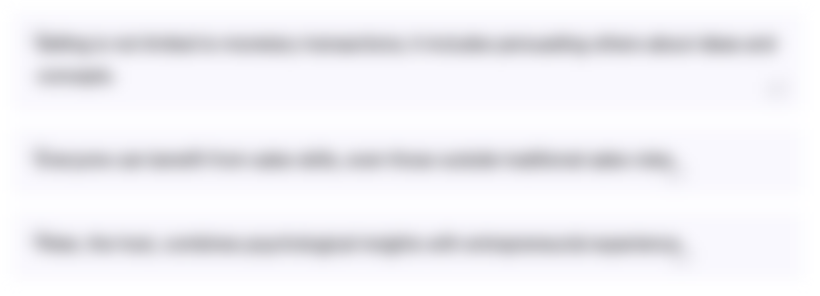
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
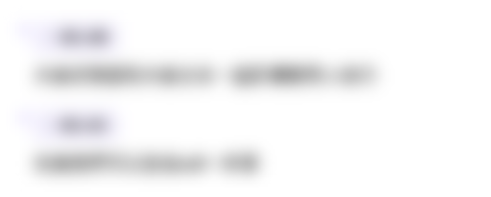
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
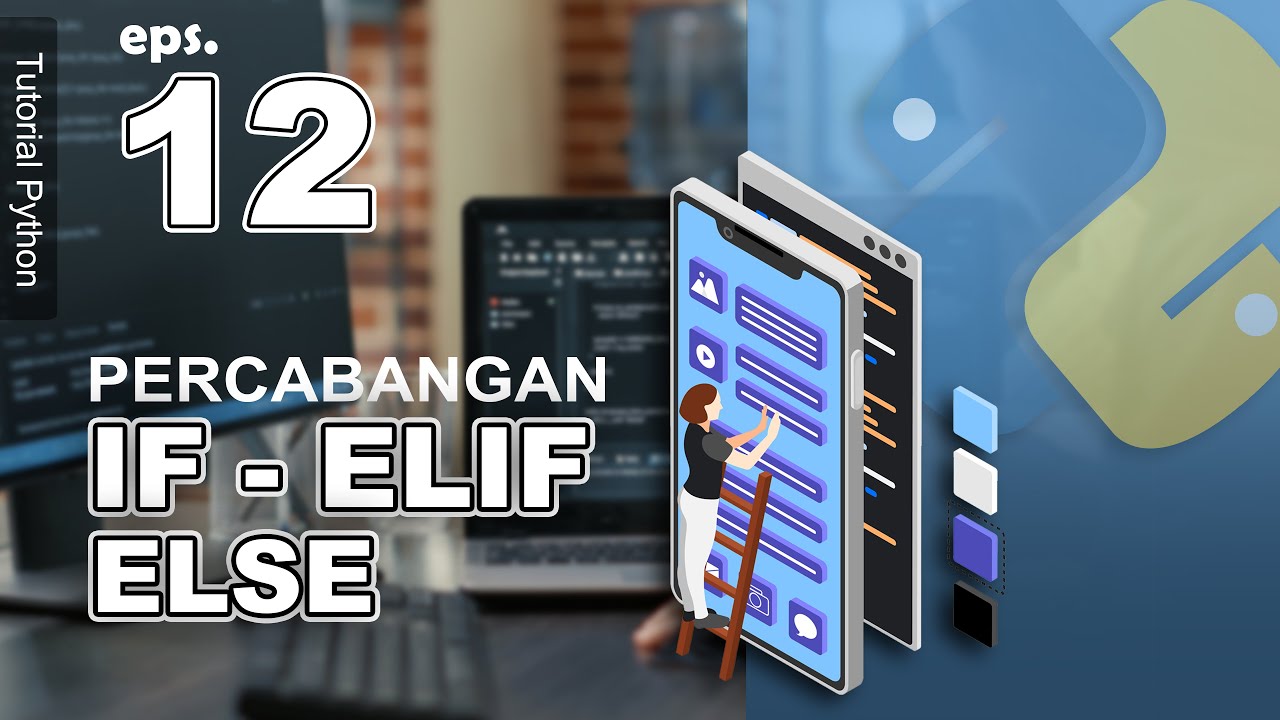
12 - IF - ELIF- ELSE (Conditional Statement) Branching - Indonesian Python Tutorial
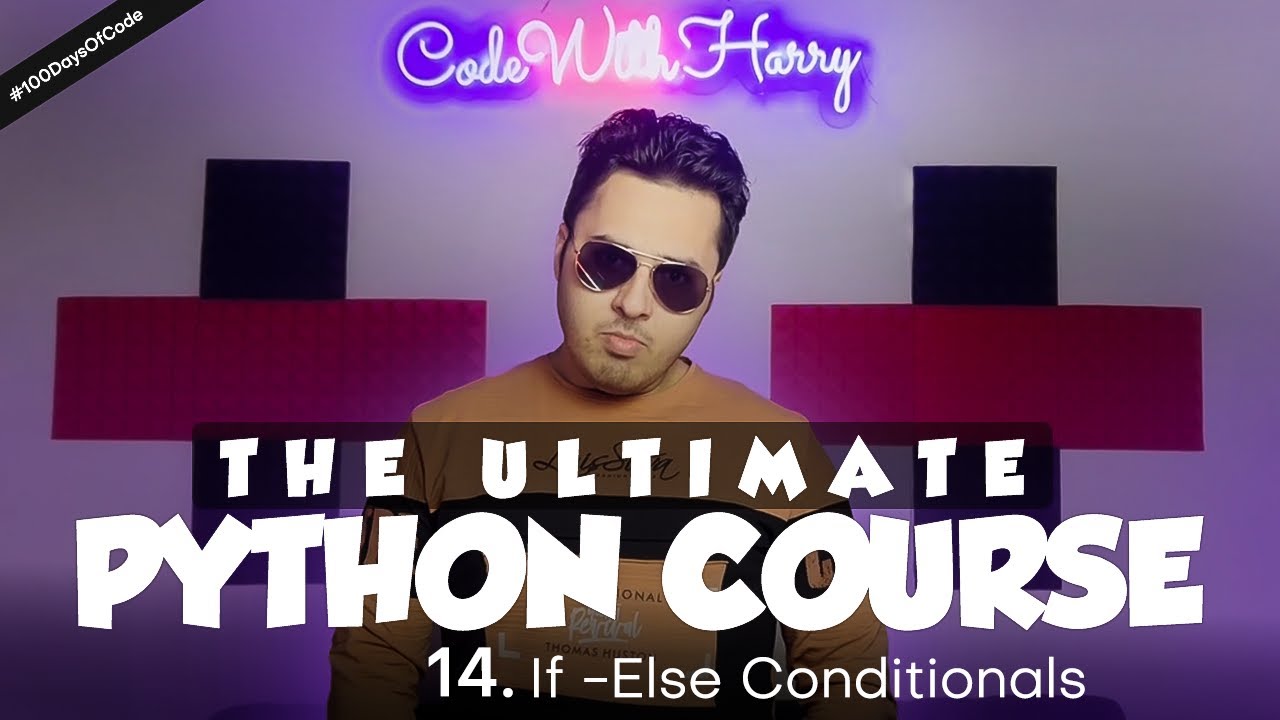
If Else Conditional Statements in Python | Python Tutorial - Day #14
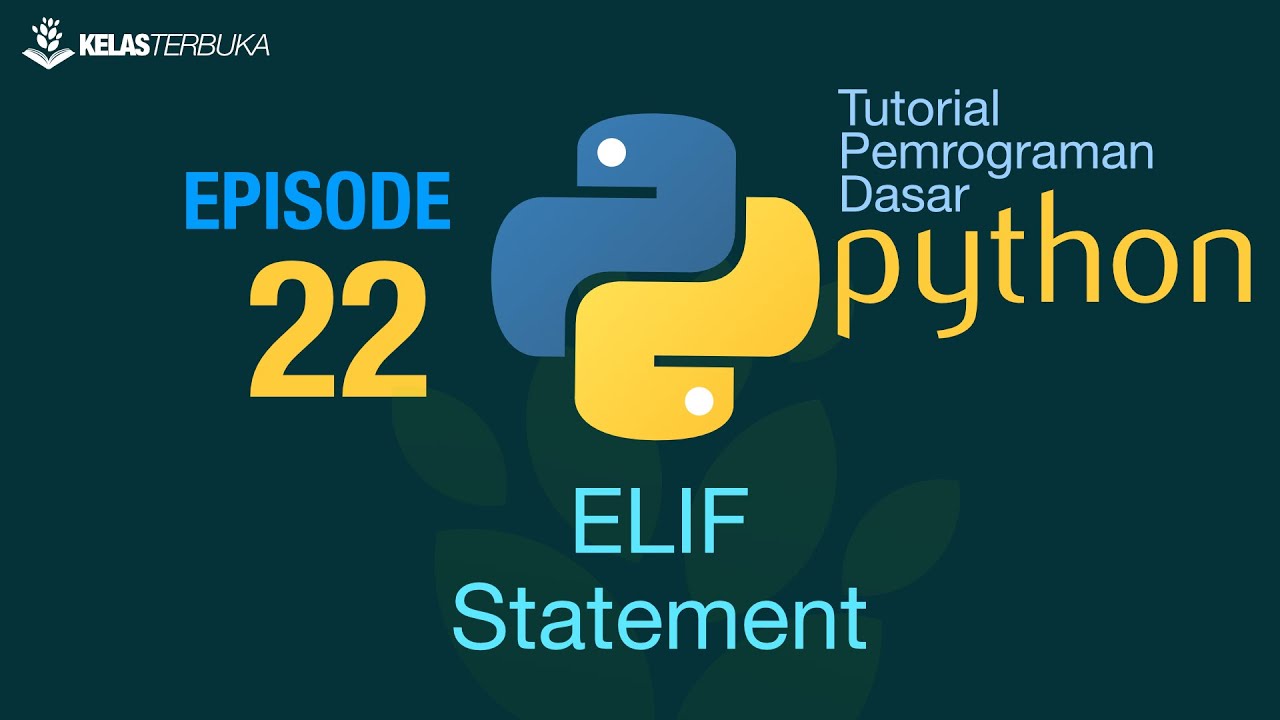
Belajar Python [Dasar] - 22 - ELIF Statement
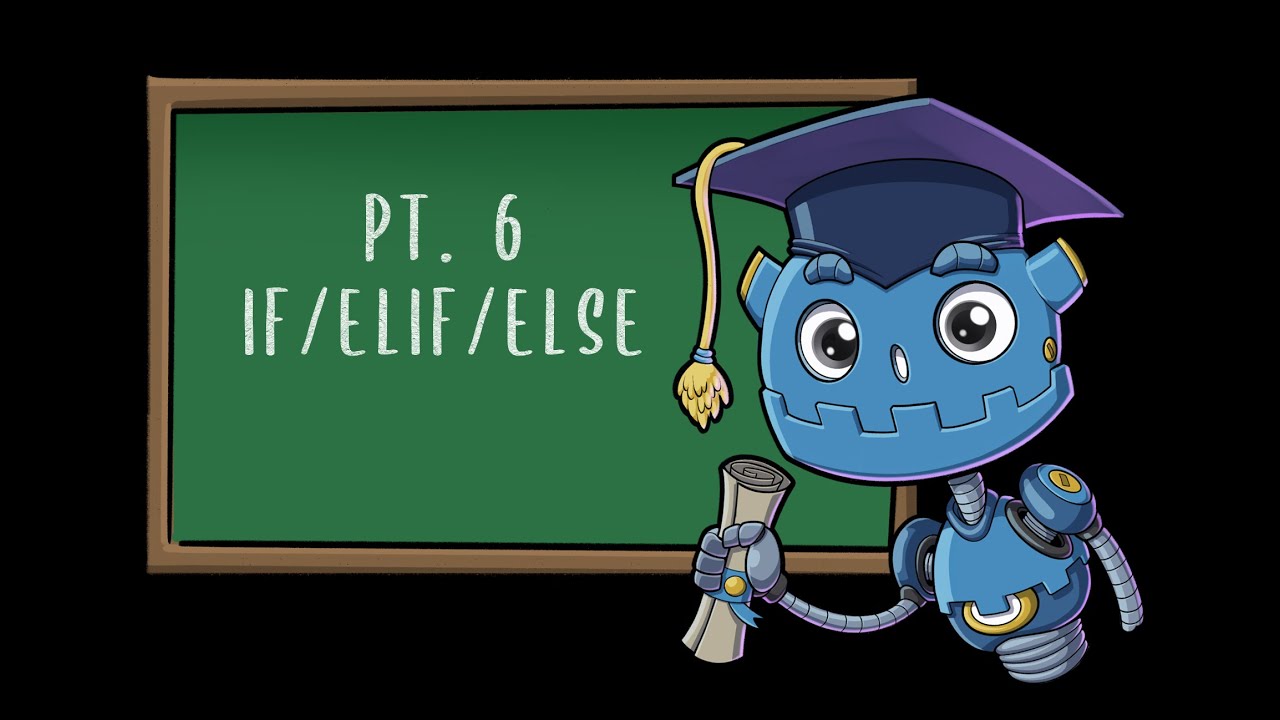
If/Elif/Else Statement Chain | Godot GDScript Tutorial | Ep 06
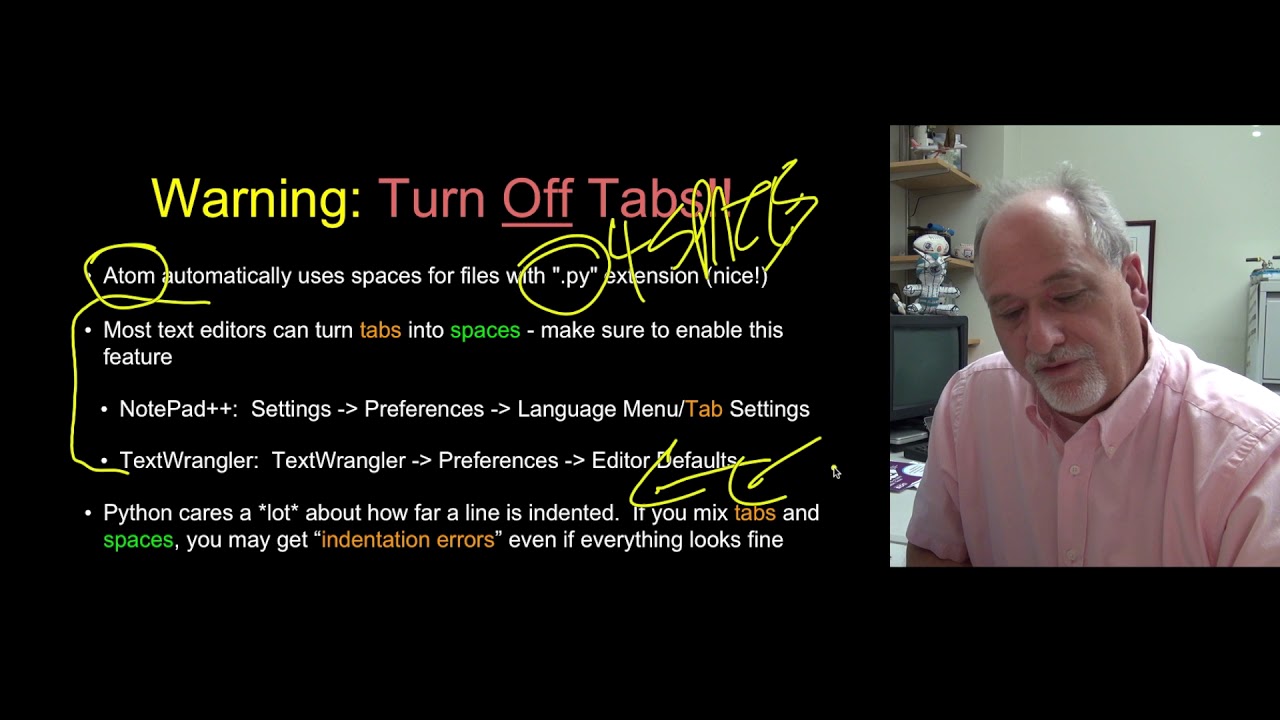
03 - Conditional A - Python for Everybody Course

CSP: Conditionals pt 2B - if/else statements
5.0 / 5 (0 votes)