Assertion reason based questions in Java | ICSE
Summary
TLDRThis educational video introduces assertion-reason based questions, a new type highlighted in the ICSC 2024 paper. It covers topics like Java's method overloading, object creation from classes, array indexing, multi-level inheritance, do-while loops, constructor inheritance, increment/decrement operators, variable mutability, and access specifiers for local variables. Each concept is paired with an assertion and a reason, followed by a multiple-choice question to test understanding. The video aims to clarify these computer science concepts and enhance students' problem-solving skills.
Takeaways
- 🔑 Java supports method overloading, allowing multiple methods with the same name but different parameters.
- 🏭 A class in Java is referred to as an object factory because it is the blueprint from which objects are instantiated.
- 📊 The index of the last element in an array is one less than the total number of elements, not equal to it, as arrays are zero-indexed.
- 🔄 In multi-level inheritance, a derived class can act as a base class for another class, demonstrating the concept of polymorphism.
- 🔁 The do-while loop always executes at least once because its condition is checked after the loop body is executed, making it an exit-controlled loop.
- 🚫 Constructors in Java cannot be inherited because they are not members of a class; they are used to initialize objects.
- ➕ Increment and decrement operators are unary, not binary, as they operate on a single operand to modify its value.
- 🔒 The value of a variable can be fixed by declaring it as 'final', which means the variable becomes immutable after initialization.
- 🔍 Pure methods, also known as accessors, are methods that do not modify the state of an object and are typically used to retrieve values.
- 📍 Local variables do not require an access specifier because they are declared within methods, constructors, or blocks and their scope is limited to the block they are defined in.
Q & A
What is the significance of method overloading in Java as mentioned in the script?
-Method overloading in Java allows the creation of multiple methods with the same name but different parameters. This is possible due to Java's implementation of polymorphism, which enables the same method name to be used for different purposes.
How is a class referred to as an object factory, and why is this term used?
-A class is referred to as an object factory because it serves as a blueprint for creating objects. The term is used because objects are instantiated or 'manufactured' from classes, which contain the common attributes and behaviors that the objects will inherit.
What is the relationship between the index of the last element of an array and the number of elements in the array?
-The index of the last element of an array is one less than the number of elements in the array. This is because array indexing in Java begins at 0, so the last element's index is the array's length minus one.
Can a derived class act as a base class for another class, and how does this relate to multi-level inheritance?
-Yes, a derived class can act as a base class for another class. This is a fundamental concept in multi-level inheritance, where a class that is derived from another can itself be the ancestor for further derived classes, creating a hierarchy of classes.
Does the do-while loop always execute at least once, and why?
-Yes, the do-while loop always executes at least once because it is an exit-controlled loop. The conditional expression is evaluated after the execution of the loop body, ensuring that the loop runs at least once before the condition is checked.
Can constructors be inherited in Java, and what does this imply about their nature within a class?
-Constructors cannot be inherited in Java. This implies that constructors are not considered members of a class in the same way variables and methods are. They are specific to the class in which they are defined and are not part of the class's inheritance hierarchy.
What are the characteristics of increment and decrement operators, and why are they considered unary operators?
-Increment and decrement operators are unary operators because they require only one operand to function. They are used to modify the value of a variable by incrementing or decrementing it by one.
Can the value of a variable be fixed in Java, and if so, how?
-Yes, the value of a variable can be fixed in Java by using the 'final' keyword. When a variable is declared as 'final', its value cannot be changed once it has been assigned.
What is a pure method, and how does it relate to the state of an object?
-A pure method, also known as an accessor, is a method that does not change the state of an object. It is a type of method that only retrieves or returns information without modifying the object's state or data.
Why can't local variables use access specifiers, and where are they typically declared?
-Local variables cannot use access specifiers because they are declared within methods, constructors, or blocks, and their scope is limited to that block. Access specifiers are not applicable to local variables as their visibility and lifetime are confined to the block in which they are declared.
Outlines
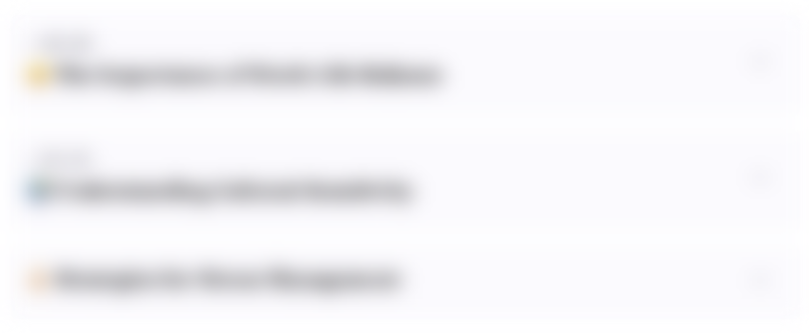
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
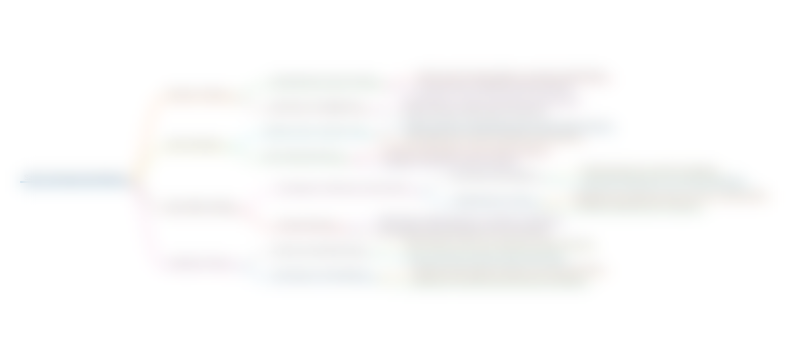
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
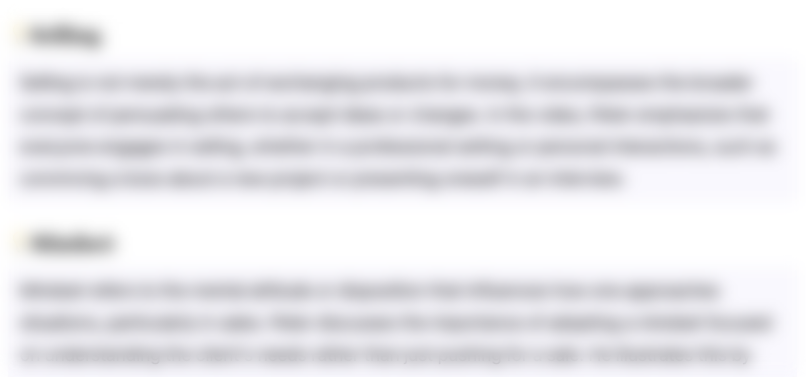
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
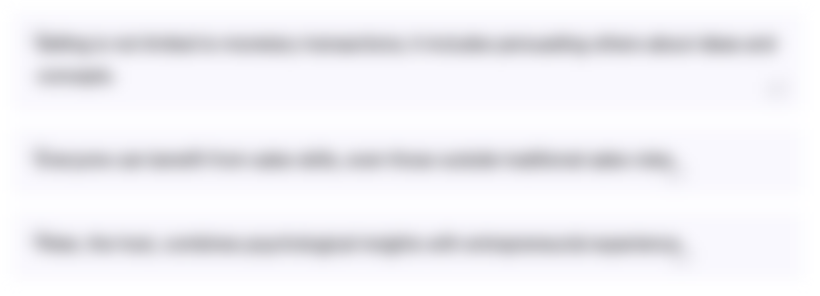
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
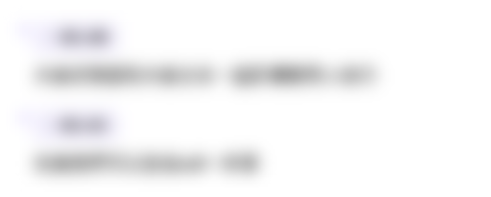
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
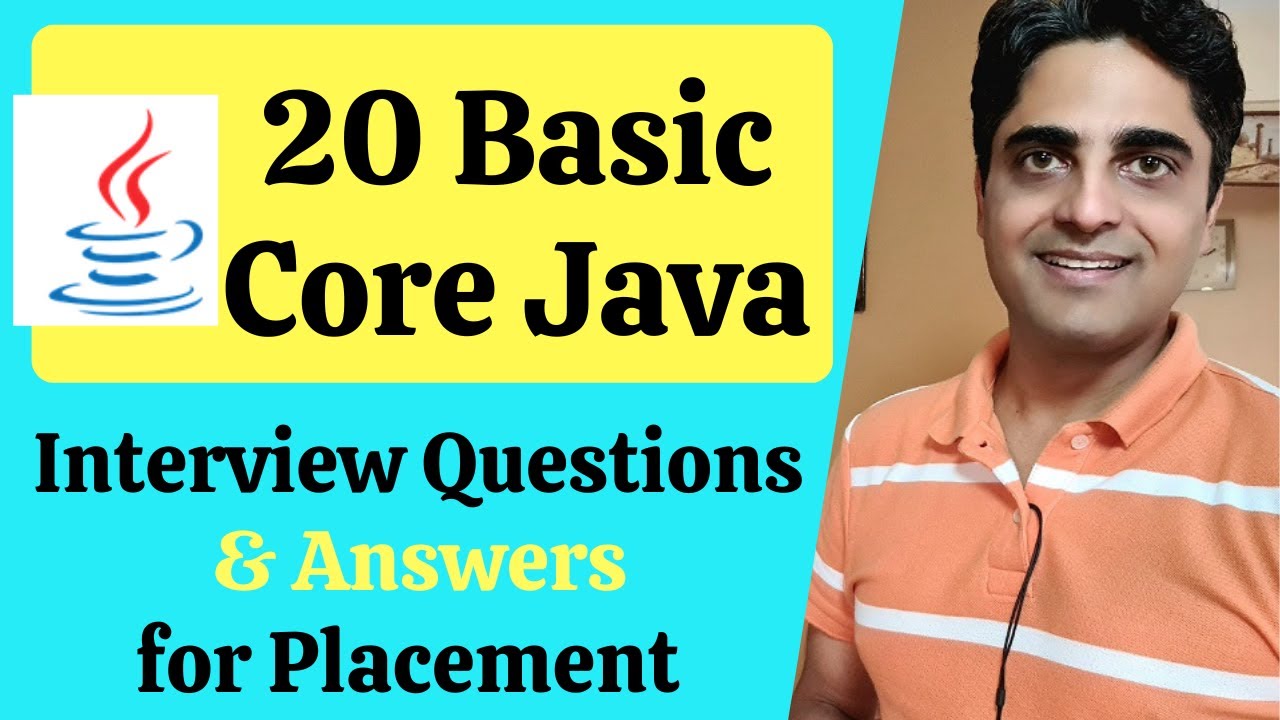
20 Basic Core Java Interview Questions & Answers- TCS, Accenture, Cognizant, Infosys, Wipro, HCL
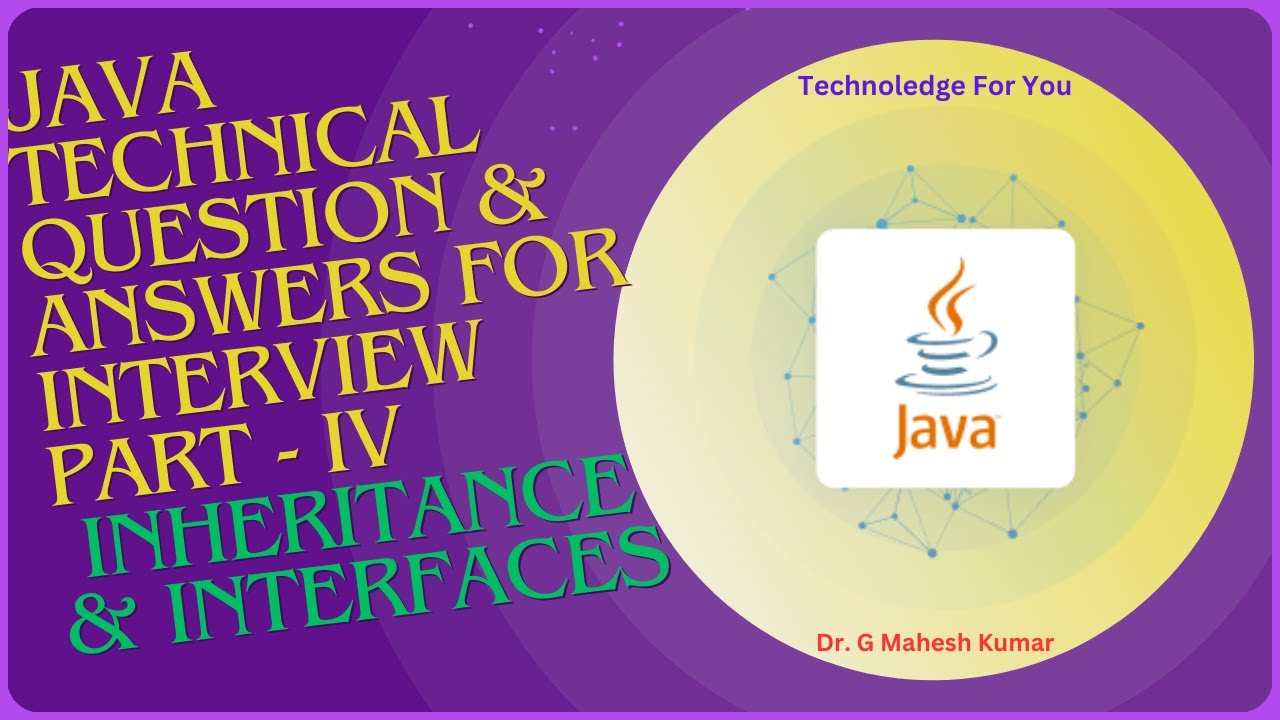
JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART IV INHERITANCE & INTERFACES
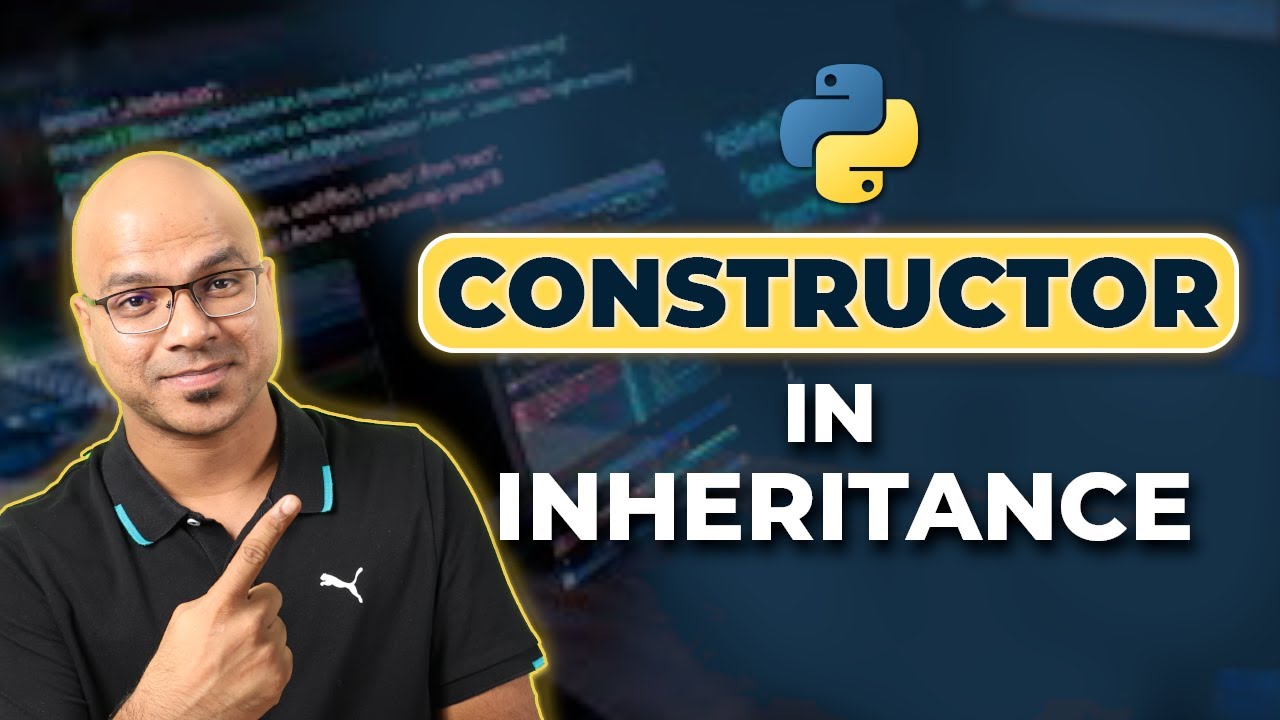
#56 Python Tutorial for Beginners | Constructor in Inheritance

Inheritance in Java - Java Inheritance Tutorial - Part 1

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
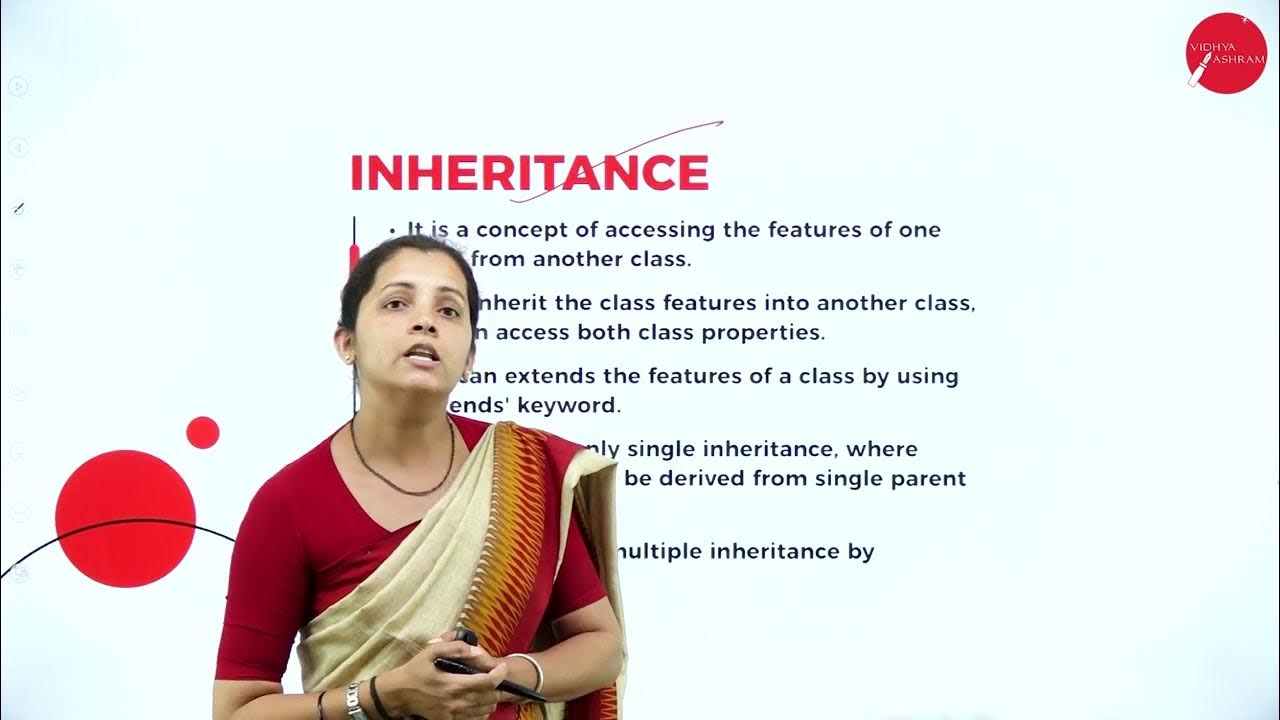
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
5.0 / 5 (0 votes)