GCSE Python Programming 6 - For Loops
Summary
TLDRIn this episode of 'Programming with Python,' the focus is on the importance of loops for efficient coding. The tutorial explains how to use 'for' loops to automate repetitive tasks, showcasing how to print sequences without manual repetition. It covers the syntax and functionality of 'for' loops, including setting start and end values, and adjusting the step size. Practical examples are given, such as printing multiplication tables and creating a countdown timer. The video concludes with an invitation to learn about using 'for' loops with lists in the next installment.
Takeaways
- π Loops are essential in programming to avoid repetitive code and allow the computer to perform repetitive tasks efficiently.
- π’ The 'for' loop in Python is used for iteration and is structured with a counter variable and a range to define the start and end of the loop.
- π The 'range' function in Python is used to generate a sequence of numbers, which can be customized to start at a specific number and end one less than the specified number.
- π The syntax of a 'for' loop includes the keyword 'for', a counter variable, the 'in' keyword, the 'range' function, and a colon followed by indented code block.
- π The 'for' loop can be used to iterate through a sequence of numbers, printing each number one by one, as demonstrated by printing numbers from 0 to 9.
- π The 'range' function can also be used to count down, as shown by the example that counts from 100 down to 0 in steps of -1.
- π Customizing the 'for' loop allows for printing specific sequences, such as the multiplication tables, by adjusting the start, end, and step values in the 'range' function.
- π The loop counter can increment by different values, not just 1, allowing for more complex iterations like printing even numbers or multiples of a specific number.
- π― The 'for' loop can be used to create countdown timers, as demonstrated by the example that counts down from 10 to 1 for a rocket launch sequence.
- π Understanding and correctly implementing 'for' loops is crucial for writing efficient and readable code, especially when dealing with sequences and iterations.
- π The next episode will explore using 'for' loops with lists, including one-dimensional and two-dimensional arrays, expanding the capabilities of iteration in Python.
Q & A
What is the main purpose of using loops in programming?
-The main purpose of using loops in programming is to avoid writing sequences of code repeatedly by allowing the computer to perform repetitive tasks automatically.
What is the fundamental concept of iteration in programming?
-Iteration is the fundamental concept that allows repeating tasks so that the computer does the hard work for us, instead of manually writing the same code over and over.
How does the 'for' loop in Python work?
-The 'for' loop in Python works by using a counter variable that iterates over a range of values. The loop starts counting at a specified start point and stops one less than the end point, executing the indented code block for each iteration.
What is the syntax for a basic 'for' loop in Python?
-The syntax for a basic 'for' loop in Python is 'for X in range(start, end):' followed by the indented code block to be executed during each iteration.
Why is the colon (:) necessary at the end of the 'for' loop declaration?
-The colon is necessary to indicate the start of the block of code that is associated with the loop, similar to how it is used in if/elif/else blocks.
How can you specify the start and end numbers of a 'for' loop in Python?
-You can specify the start and end numbers of a 'for' loop in Python by using the 'range(start, end)' function, where 'start' is the initial number and 'end' is the number at which the loop will stop, one less than the actual number displayed.
What does the 'range' function do in a 'for' loop?
-The 'range' function generates a sequence of numbers starting from the first parameter (inclusive) and ending at the second parameter (exclusive), which is used to control the iterations of the 'for' loop.
Can you specify the step value in a 'for' loop in Python?
-Yes, you can specify the step value in a 'for' loop by providing a third parameter to the 'range' function, which determines the increment or decrement between each iteration.
How can you create a countdown timer using a 'for' loop in Python?
-You can create a countdown timer using a 'for' loop by starting at the desired count and decrementing by one in each iteration until reaching zero, with the loop printing the current count each time.
What is the significance of indentation in Python code blocks, such as loops?
-Indentation in Python is used to define the scope of code blocks, such as loops, functions, and conditionals. It is a requirement for the Python interpreter to understand which lines of code are part of the block.
How can you print a multiplication table using a 'for' loop in Python?
-You can print a multiplication table by setting the 'for' loop to iterate over the range of numbers you want to multiply, and using an expression within the loop to calculate and print the products.
Outlines
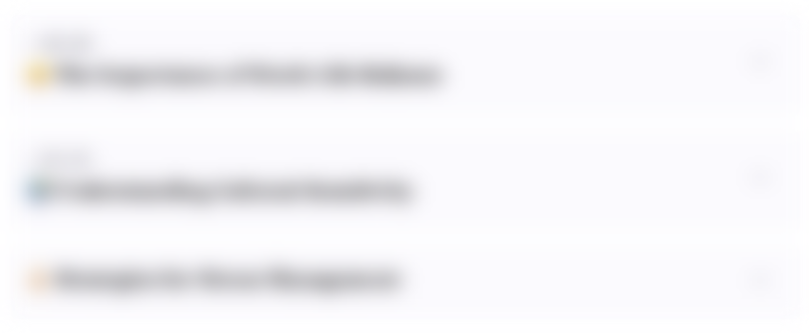
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
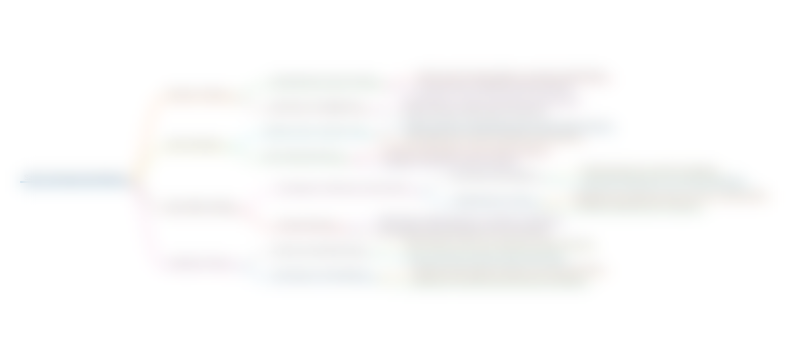
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
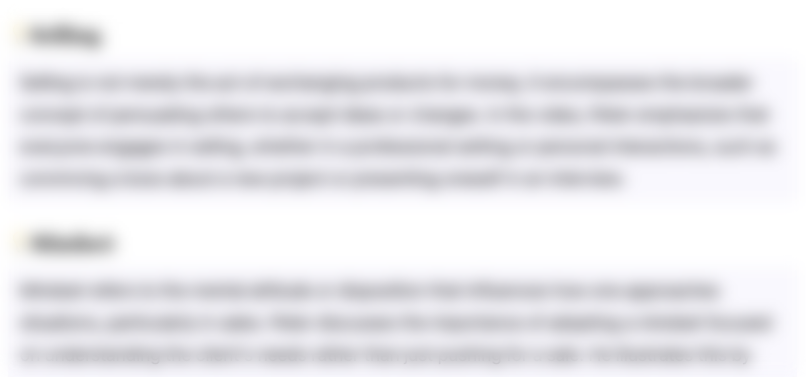
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
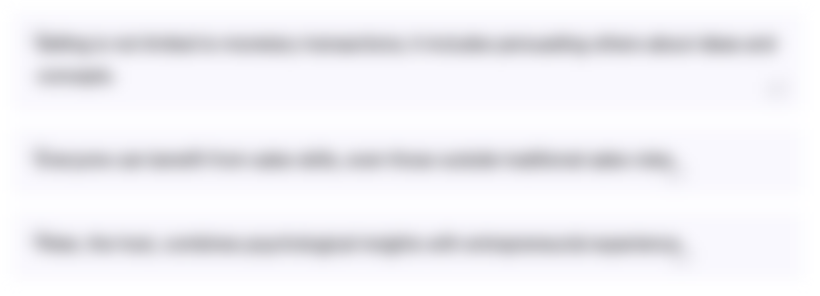
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
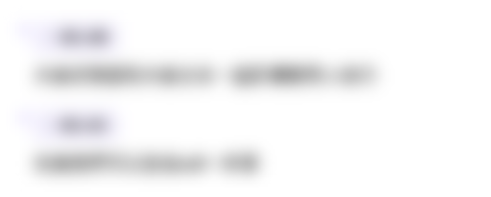
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)