Python Bangla Tutorials 11 : Formatted String | Type function
Summary
TLDRThis programming tutorial covers essential Python concepts, focusing on string formatting, variable types, functions, and loops. It explains how to work with different data types, including integers, floats, and booleans, while demonstrating various methods to format and manipulate strings. Through examples and practical exercises, users learn how to define variables, apply functions, and implement loops to process and display results effectively. The tutorial emphasizes the importance of understanding these fundamental concepts to build a solid foundation for coding in Python.
Takeaways
- 😀 The script discusses the use of the 'type' function in Python for identifying variable types.
- 😀 Variables in Python can be of different data types, such as integers, floats, and strings.
- 😀 The example program demonstrates type conversion, showing how an integer can be converted into a float or string.
- 😀 String formatting is highlighted as a key concept, with mention of how it can be finicky and cause errors in code.
- 😀 An example program is shared, where two numbers are added and the result is printed using string formatting.
- 😀 It is suggested that Python can automatically determine the data type of a variable without needing explicit conversion in some cases.
- 😀 The script covers boolean types in Python, showing how they can represent 'True' or 'False' values.
- 😀 The importance of loops in programming is mentioned, with the example of adding two numbers using a loop.
- 😀 The use of functions in Python is introduced, explaining how to create and call them for different operations.
- 😀 The script concludes with tips on handling input/output in Python, especially when using formatting to display results cleanly.
Q & A
What are the common data types in Python?
-The common data types in Python include integers (int), floats (float), strings (str), and booleans (bool).
How do you declare a variable in Python?
-In Python, variables are declared by simply assigning a value to a name. For example: `number = 10` for an integer or `message = 'Hello'` for a string.
What is a function in Python and how do you define one?
-A function in Python is a block of reusable code that performs a specific task. You define a function using the `def` keyword followed by the function name and parameters. Example: `def add_numbers(a, b): return a + b`.
What is string formatting and why is it important in Python?
-String formatting allows you to create dynamic strings by embedding variables inside strings. It is important for presenting data clearly and making outputs user-friendly. F-strings (e.g., `f'Hello {name}'`) are a common method in Python.
Can you explain the difference between integers and floats in Python?
-Integers are whole numbers without decimals, such as 10 or 100, while floats are numbers that contain decimal points, such as 3.14 or 20.5.
How can you format a string in Python to include variables?
-You can format a string in Python using f-strings. For example: `name = 'Alice'; age = 30; print(f'Hello, my name is {name} and I am {age} years old.')`.
What is the purpose of a function that accepts parameters in Python?
-The purpose of a function that accepts parameters is to allow it to perform operations on different inputs, making the function reusable with different values. For example, `add_numbers(a, b)` can add any two numbers provided as arguments.
How do you return a value from a function in Python?
-To return a value from a function in Python, you use the `return` keyword. For example, in a function like `def add_numbers(a, b): return a + b`, it returns the sum of `a` and `b`.
What does the following code do: `print(f'The sum of {a} and {b} is {result}')`?
-This code prints a formatted string that includes the values of `a`, `b`, and `result`. It dynamically inserts the values of the variables into the string and outputs something like: `The sum of 10 and 20 is 30`.
What is the significance of using `def` in Python?
-The `def` keyword in Python is used to define a function. It signals the start of a function definition and is followed by the function name and parameters.
Outlines
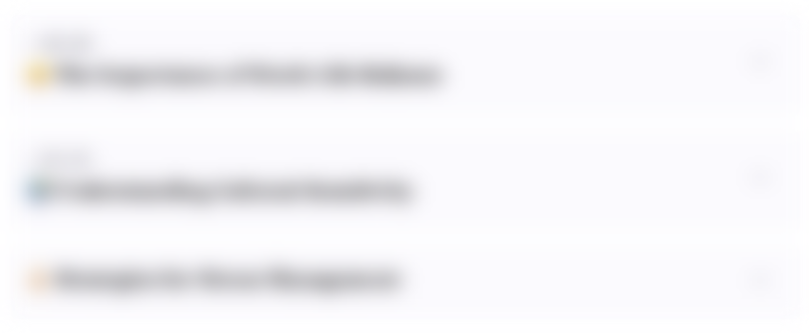
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
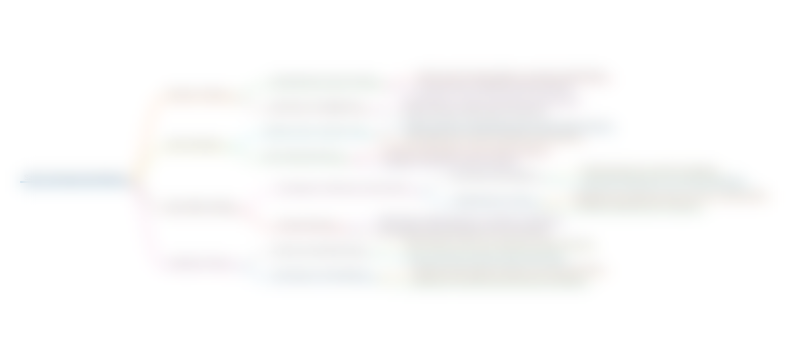
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
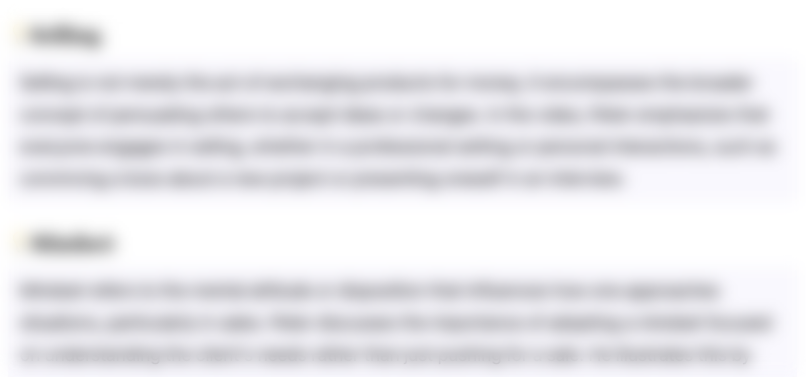
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
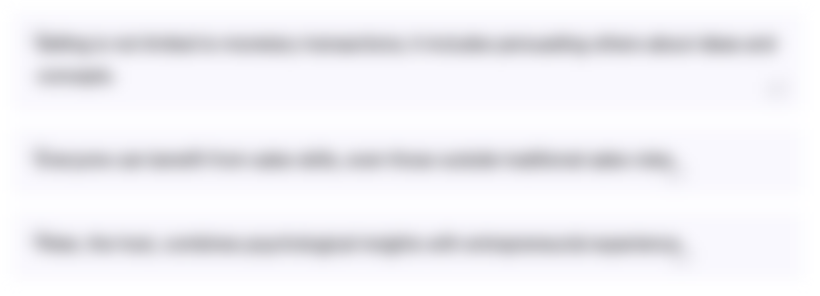
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
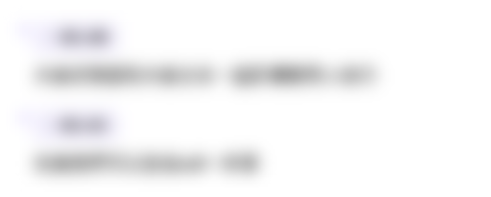
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
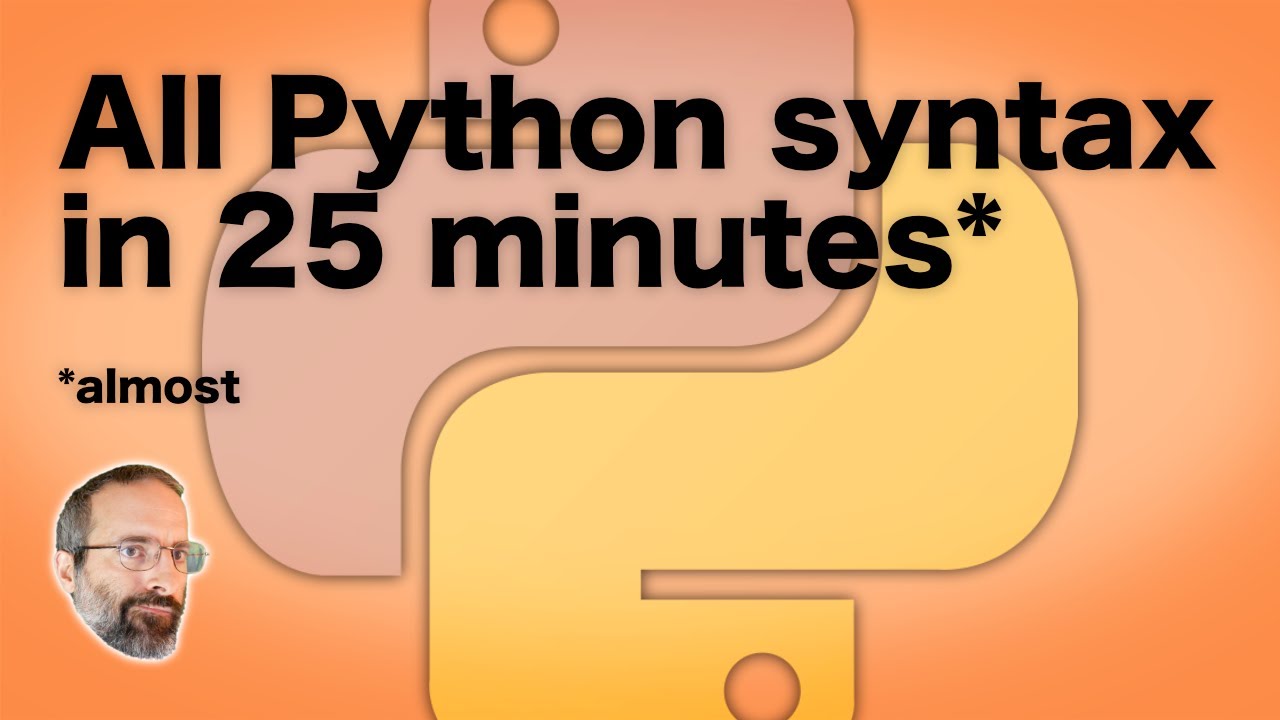
All Python Syntax in 25 Minutes – Tutorial

Informatika Analisis Data Pengenalan Bahasa Phyton Pada Google Collab Perintah print dan array
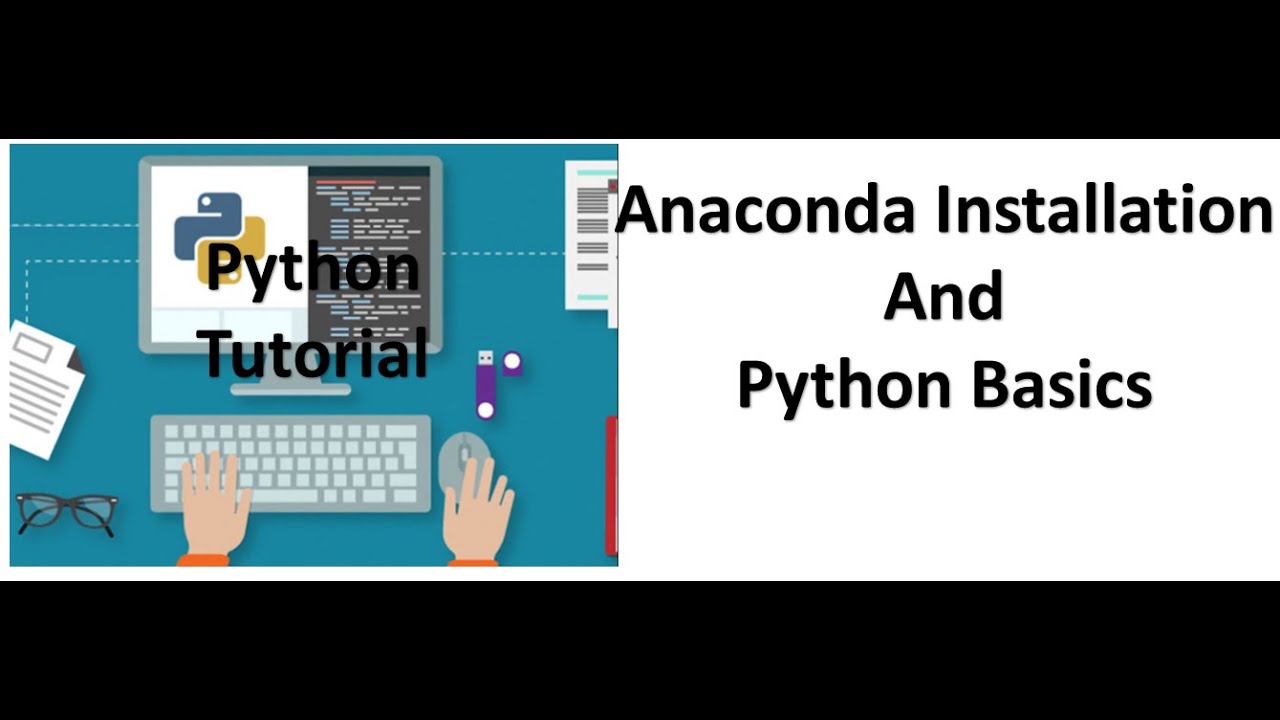
Tutorial 1- Anaconda Installation and Python Basics
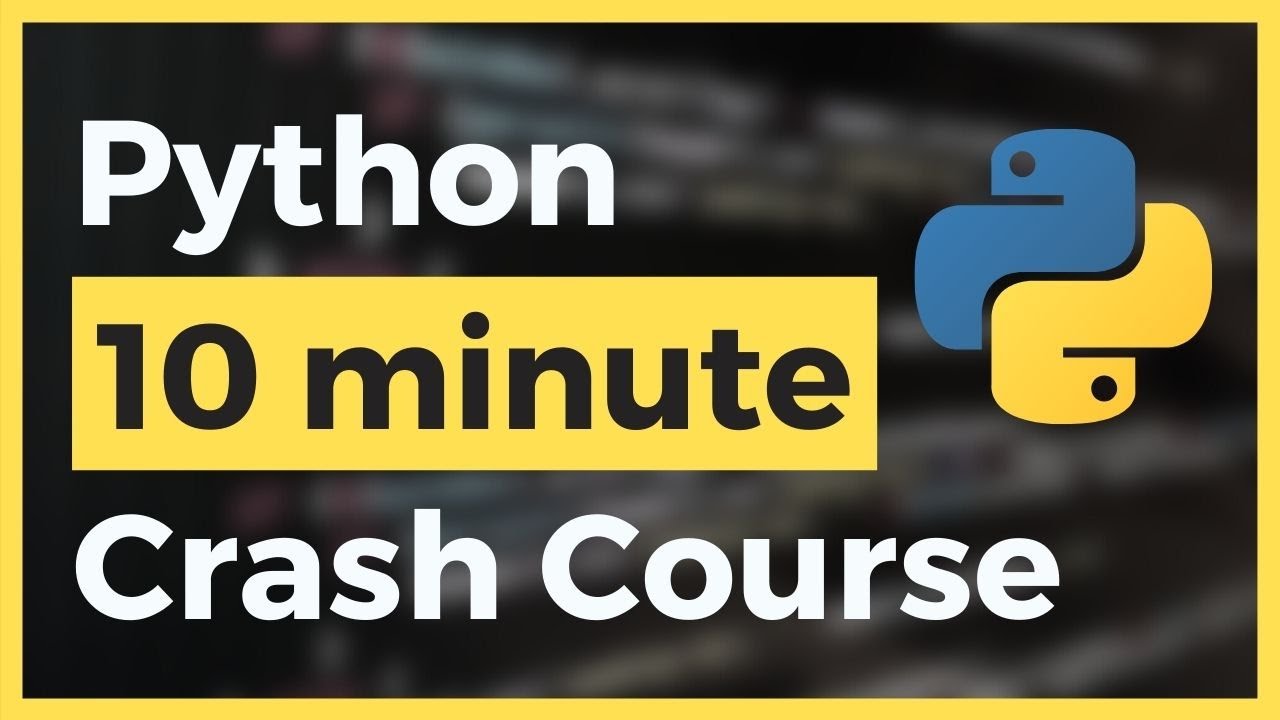
Learn Python in Less than 10 Minutes for Beginners (Fast & Easy)
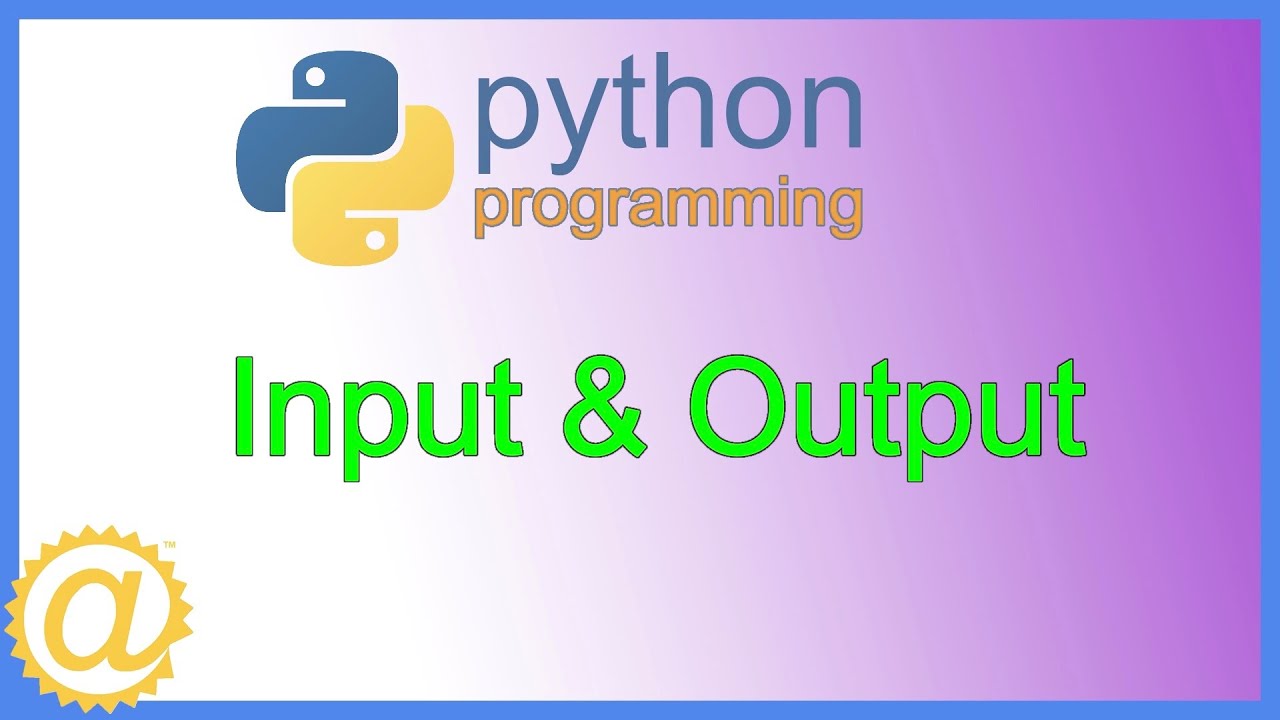
Python Programming - Basic Input and Output - print and input functions

Variables and Datatypes
5.0 / 5 (0 votes)