for loop
Summary
TLDRIn this educational video, the focus is on the utility of 'for' loops in Python programming. The tutorial begins with an example of a turtle named Alex drawing a square, highlighting repetitive code that can be optimized using loops. The script explains the structure and syntax of 'for' loops, emphasizing the loop variable and sequence. It illustrates the loop's flow of control with a diagram and demonstrates how loops can automate repetitive tasks, such as drawing a square with varying line colors. The video also introduces the 'range' function as a time-saving shortcut for creating sequences, promoting efficient coding practices.
Takeaways
- ๐ข The script discusses using a 'for' loop to automate repetitive tasks, exemplified by making a turtle named Alex draw a square.
- ๐ The 'for' loop is introduced as a way to replace duplicated code, making the code cleaner and more efficient.
- ๐ The format of a 'for' loop is explained, including the use of a loop variable and a sequence, followed by a colon and an indented block of statements.
- ๐ The flow of control within a loop is visualized through a diagram, showing how the loop variable is assigned the next item in the sequence and how the loop body is executed.
- ๐จ A practical example is given where the loop variable is used to change the color of the lines drawn by the turtle, demonstrating dynamic use of the loop variable.
- ๐ข The 'range' function is introduced as a shortcut for creating a sequence of numbers, which is particularly useful for loops that need to iterate a large number of times.
- ๐ก The concept of 'intelligently lazy' programming is highlighted, emphasizing the importance of finding efficient ways to solve problems, which loops exemplify.
- ๐ The script uses the turtle graphics library to provide a visual and interactive way to understand loops, making the concept more accessible.
- ๐ The loop variable's role in referencing different values in the sequence during each iteration of the loop is explained, showing how it changes state.
- ๐ A creative use of loops is demonstrated by drawing a square with each side in a different color, showcasing the versatility of loops.
Q & A
What is the purpose of using a loop in programming?
-The purpose of using a loop in programming is to repeat a pattern of code multiple times without having to manually write the same code repeatedly, which enhances efficiency and reduces redundancy.
What is the general format of a for loop in Python?
-The general format of a for loop in Python is 'for loop_variable in sequence:' followed by the indented block of statements that will be executed for each item in the sequence.
What does the colon character in a for loop indicate?
-The colon character in a for loop indicates that whatever comes next should be indented, marking the beginning of the loop's body where the statements to be executed are placed.
How does the flow of control work within a for loop?
-The flow of control within a for loop starts by checking if all items in the sequence have been iterated over. If not, it assigns the next item to the loop variable, executes the loop body, and repeats. If all items have been used, it skips the assignment and execution, continuing with the code after the loop.
Why is the loop variable not used in the example of drawing a square?
-The loop variable is not used in the example of drawing a square because the same actions (moving forward and turning left) are performed for each iteration, and there is no need to reference the loop variable for these actions.
What is the significance of the sequence 0, 1, 2, 3 in the for loop example?
-The sequence 0, 1, 2, 3 signifies the number of times the loop will execute, which in this case is four times, corresponding to the four sides of a square.
How can the loop variable be used to change the color of the lines drawn by the turtle?
-The loop variable can be used to change the color of the lines drawn by the turtle by setting the color to the value of the loop variable, which cycles through a sequence of color names, thus changing the line color with each iteration.
What is the advantage of using the 'range' function in a for loop?
-The advantage of using the 'range' function in a for loop is that it simplifies the process of iterating over a sequence of numbers, eliminating the need to manually type out each number, which is particularly useful for large sequences.
Why do computer scientists often start counting with 0?
-Computer scientists often start counting with 0 because it aligns with the way programming languages index arrays and other data structures, where the first element is typically at index 0.
What does the term 'intelligently lazy' imply in the context of programming?
-The term 'intelligently lazy' implies finding efficient ways to perform tasks, such as using loops to automate repetitive code, thereby saving time and effort without unnecessary manual work.
Outlines
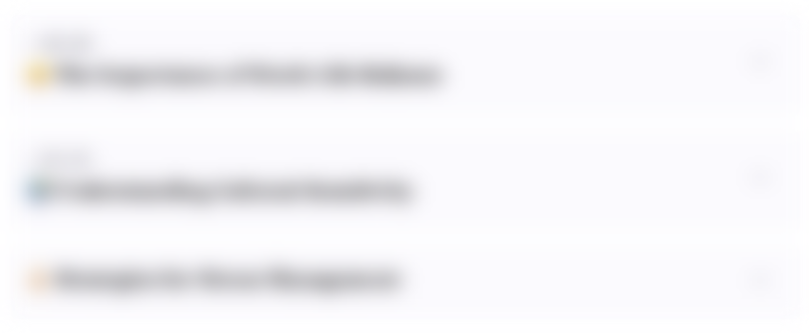
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
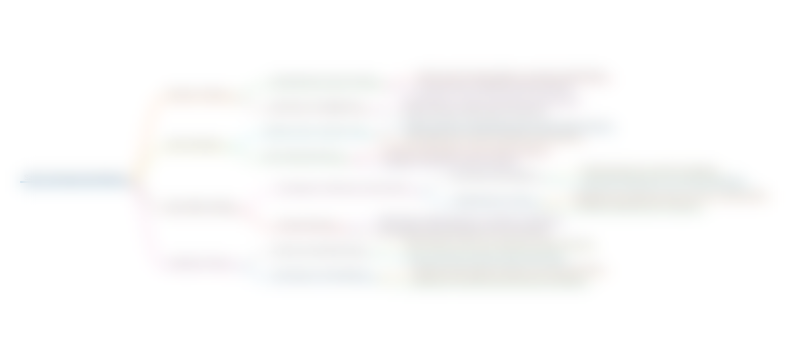
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
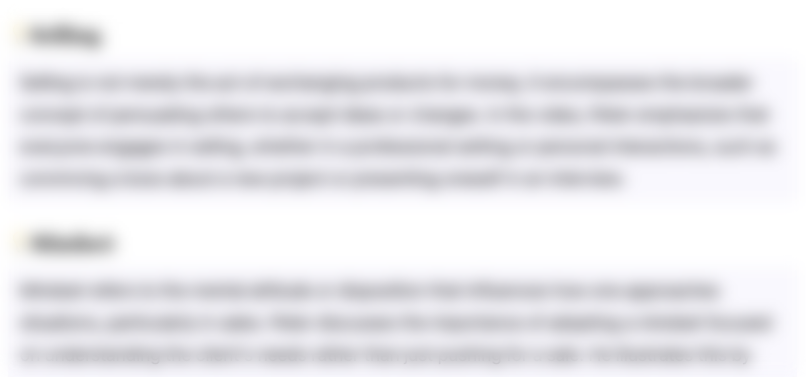
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
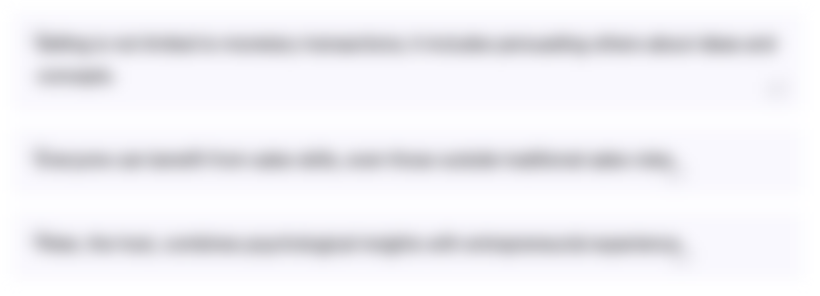
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
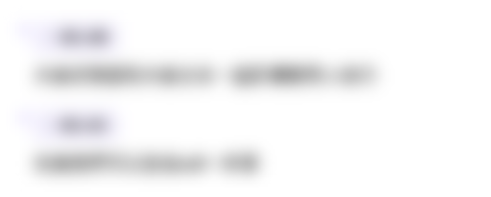
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)