How to use escaping closures in Swift | Continued Learning #20
Summary
TLDRIn this informative video by Swiffle Thinking, Nick dives into the concept of closures in Swift, with a focus on escaping closures. He explains the difference between synchronous and asynchronous code, demonstrating how regular functions work with immediate returns versus the delayed execution of asynchronous tasks. Nick illustrates the use of escaping closures to handle data retrieval from a database or the internet, showcasing how to implement them in Swift UI with practical examples. The video is designed to clarify a potentially confusing topic, making it accessible for developers new to asynchronous programming.
Takeaways
- ๐ The video is an educational resource on Swift and Swift UI, focusing on closures and specifically escaping closures.
- ๐ Nick, the presenter, explains the transition from synchronous to asynchronous code, which is necessary for tasks like downloading data from the internet.
- ๐๏ธ Synchronous code executes line by line immediately, whereas asynchronous code involves waiting for operations like data retrieval to complete.
- ๐ Escaping closures are introduced as a solution to handle asynchronous code, allowing a function to be called after an operation completes.
- ๐ ๏ธ The script demonstrates building up to escaping closures by first creating a simple ViewModel and View in a Swift UI project.
- ๐ A ViewModel with an observable object pattern is used to bind data to the UI, starting with a simple 'text' variable.
- ๐ The presenter illustrates the concept of closures by adding a 'get data' function that simulates downloading data asynchronously.
- ๐ An example of a non-escaping closure is shown, which leads to the introduction of the '@escaping' keyword to handle the delay in asynchronous operations.
- ๐ The use of 'self.' is explained to maintain a strong reference to the class within the closure to prevent deinitialization before the closure executes.
- ๐ The video also covers using 'weak self?' to allow the class to be deinitialized if not needed, addressing potential memory management issues.
- โจ The presenter concludes by showing how to improve code readability with struct models and type aliases for completion handlers in closures.
Q & A
What is the main topic of the video?
-The main topic of the video is about closures in Swift, with a focus on escaping closures, which are used in asynchronous code.
What is the difference between synchronous and asynchronous code as explained in the video?
-Synchronous code runs from top to bottom immediately and then executes, allowing functions to return immediately. Asynchronous code, on the other hand, involves operations that do not complete immediately, such as downloading data from the internet, and requires special handling with escaping closures.
What is an escaping closure in Swift?
-An escaping closure in Swift is a closure that is called after the function in which it is defined returns. It allows the function to handle asynchronous code by executing the closure at a later time, such as after data has been fetched from a database or the internet.
Why are escaping closures necessary when downloading data from the internet?
-Escaping closures are necessary because the data does not return immediately. They enable the function to perform actions after the data has been received, even if that happens after the function has returned.
What is the purpose of the 'completion handler' in the context of the video?
-The completion handler is a parameter of a function that is a closure itself. It is called when the asynchronous task, such as data downloading, is completed, allowing the code to execute subsequent actions with the returned data.
How does the video demonstrate the transition from synchronous to asynchronous code?
-The video demonstrates this by initially showing a simple synchronous function that returns a string immediately. It then modifies the function to simulate an asynchronous task with a delay, which requires the use of an escaping closure to handle the delayed return of data.
What is the issue with using regular returns in asynchronous functions?
-Regular returns cannot be used in asynchronous functions because they expect to return immediately, which is not possible when the operation involves a delay or is waiting for external data.
Why is 'self' used with a dot in front of a property when using an escaping closure?
-Using 'self.' before a property within an escaping closure ensures that the instance of the class remains alive until the closure is executed, as it creates a strong reference to the class instance.
What alternative to using 'self.' is suggested in the video to avoid potential issues with keeping the class instance alive?
-The video suggests using 'weak self' to make the reference to the class instance optional and allow the class to be de-initialized if necessary, thus avoiding potential memory management issues.
How does the video simplify the code when using escaping closures?
-The video simplifies the code by introducing a 'download result' struct to encapsulate the data being returned, and by using a type alias called 'download completion' to represent the completion handler, making the code cleaner and more readable.
Outlines
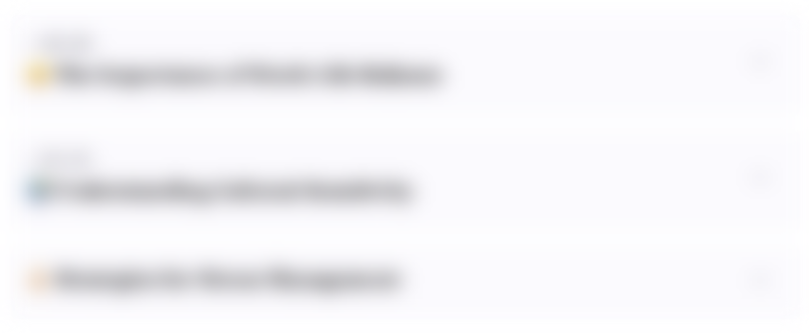
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
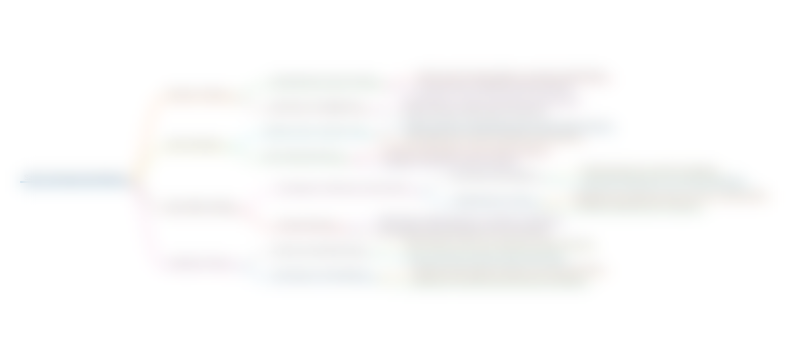
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
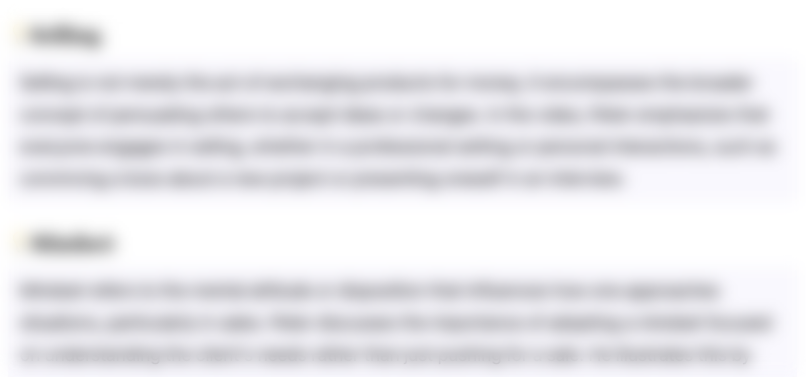
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
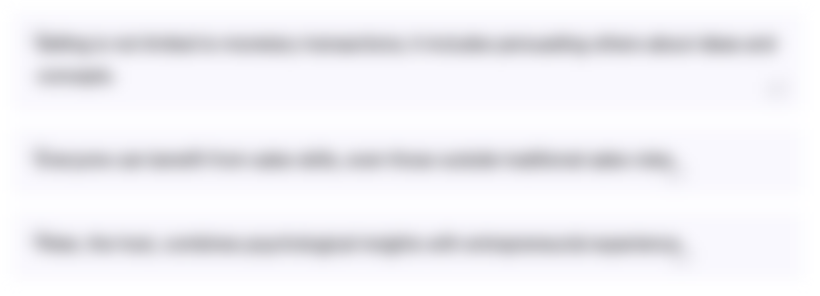
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
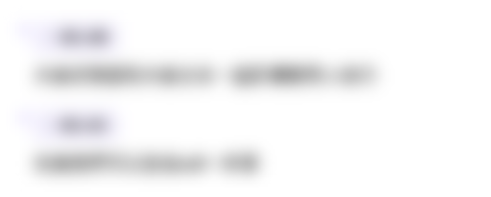
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
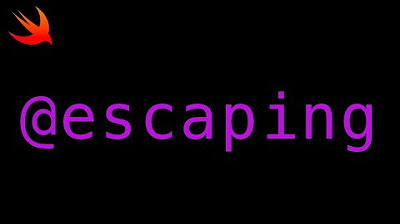
Swift Closures: @escaping Explained
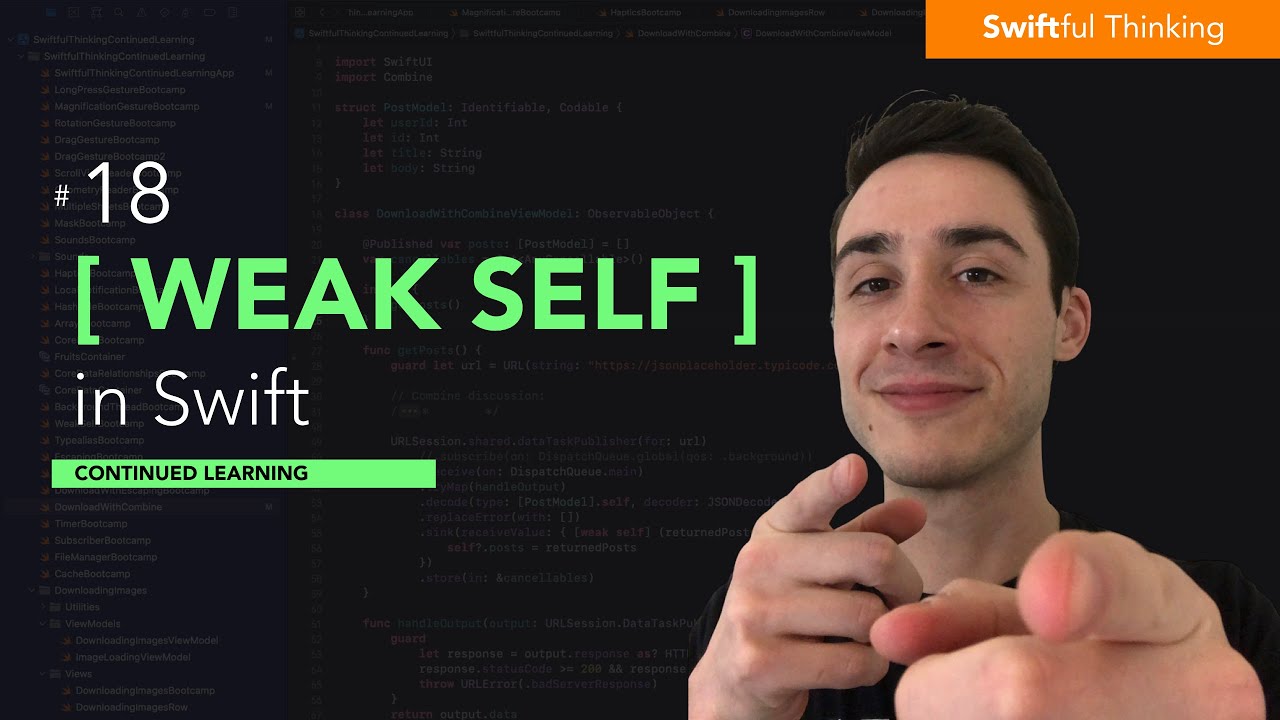
How to use weak self in Swift | Continued Learning #18

Tanda Candlestick akan berbalik arah ( Penutupan harga) - Startegi trading Support resisten

Learn Closures In 7 Minutes

Closures | JavaScript ๐ฅ | Lecture 128

More Closure Examples | JavaScript ๐ฅ | Lecture 129
5.0 / 5 (0 votes)