How to use weak self in Swift | Continued Learning #18
Summary
TLDRIn this educational video, Nick from Swiftly Thinking dives into the concept of 'weak self' in Swift programming, a crucial topic often overlooked in beginner courses. He explains the importance of understanding Automatic Reference Counting (ARC) for efficient app development. Through a practical example, Nick illustrates how to avoid creating strong references that can lead to memory issues, especially during asynchronous tasks like internet data downloads. He emphasizes the use of 'weak self' to allow classes to de-initialize properly, ensuring app efficiency and performance.
Takeaways
- ๐ The video is an educational resource on 'Swiftly Thinking', focusing on Swift and SwiftUI development.
- ๐ The main topic of the video is 'weak self', explaining its importance in Swift development, especially with asynchronous tasks.
- ๐ค The concept of 'weak self' is often overlooked in beginner courses and can lead to memory inefficiencies if not properly understood.
- ๐ 'Automatic Reference Counting (ARC)' is fundamental to understanding 'weak self', as it manages the lifetime of objects in Swift.
- ๐ Keeping the reference count low improves app efficiency and performance by allowing de-initialization of objects not in use.
- ๐ 'Weak self' is used to prevent strong reference cycles, which can occur when asynchronous tasks are performed and the object should not stay alive.
- ๐ The presenter, Nick, emphasizes the importance of 'weak self' for efficient app development and to avoid memory leaks.
- ๐ ๏ธ The video provides a practical example of creating a navigation view with two screens to demonstrate the use of 'weak self'.
- ๐ It illustrates the use of 'weak self' in the context of a ViewModel to handle data asynchronously without retaining a strong reference to the view.
- โฑ๏ธ A simulated long task is used to show how 'weak self' allows a view to de-initialize even when an asynchronous task is pending.
- ๐ The video concludes with the advice to use 'weak self' in asynchronous operations to maintain app efficiency and prevent slowdowns.
Q & A
What is the main topic of the video?
-The main topic of the video is explaining the concept of 'weak self' in the context of Swift programming, particularly when dealing with asynchronous tasks and automatic reference counting (ARC).
Why is understanding ARC important for app development?
-Understanding ARC is important for app development because it helps manage memory efficiently, making the app run faster and more smoothly by keeping the reference count of objects as low as possible.
What is a strong reference in the context of ARC?
-A strong reference in ARC is a regular reference to an object that keeps the object alive in memory. It prevents the object from being de-initialized until the reference is removed or the object is no longer needed.
What is the purpose of using 'weak self' in Swift?
-The purpose of using 'weak self' in Swift is to create a weak reference to the current class or object, allowing it to be de-initialized when it's no longer in use, which helps prevent memory leaks and improve app efficiency.
Why is 'weak self' particularly important when downloading data from the internet?
-'Weak self' is particularly important when downloading data from the internet because it ensures that the memory associated with the current screen or view can be freed up if the user navigates away while the download is in progress.
What does the term 'de-initialize' mean in the context of this video?
-In the context of this video, 'de-initialize' refers to the process where an object or screen is removed from memory when it's no longer needed or when the user navigates away from it.
How does the video demonstrate the use of 'weak self'?
-The video demonstrates the use of 'weak self' by creating a simple app with two screens and a view model, then simulating a long-running task to show how 'weak self' allows the app to de-initialize objects that are no longer in use.
What is the potential issue with using 'self.' instead of 'weak self' in asynchronous tasks?
-The potential issue with using 'self.' instead of 'weak self' in asynchronous tasks is that it creates a strong reference to the current class or object, preventing it from being de-initialized until the task is completed, which can lead to increased memory usage and decreased app performance.
How does the video simulate a long-running task?
-The video simulates a long-running task by using a delay within a DispatchQueue.main.async block, setting it to 500 seconds, to mimic the time it might take to download data from the internet.
What is the significance of the 'count' variable in the app's user interface?
-The 'count' variable in the app's user interface is used to track the number of view models that have been initialized. It helps visualize the impact of using 'weak self' versus 'self.' on the number of objects kept in memory.
Outlines
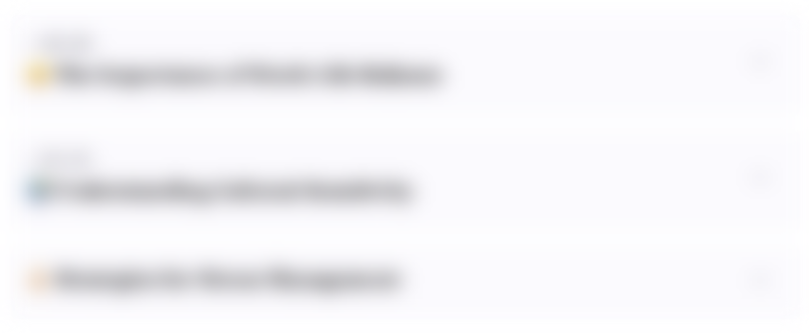
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
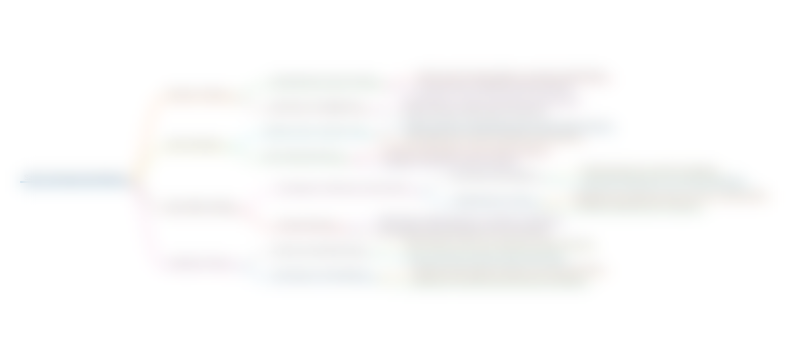
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
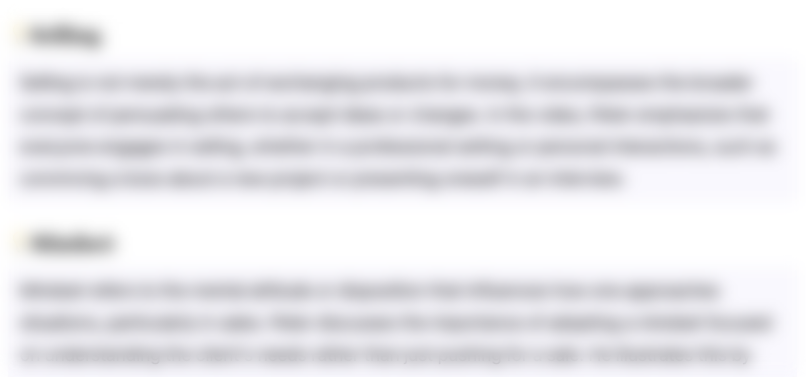
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
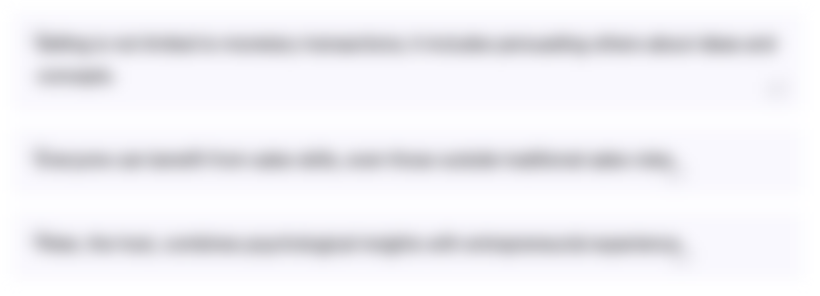
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
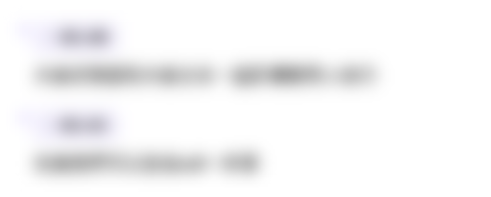
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
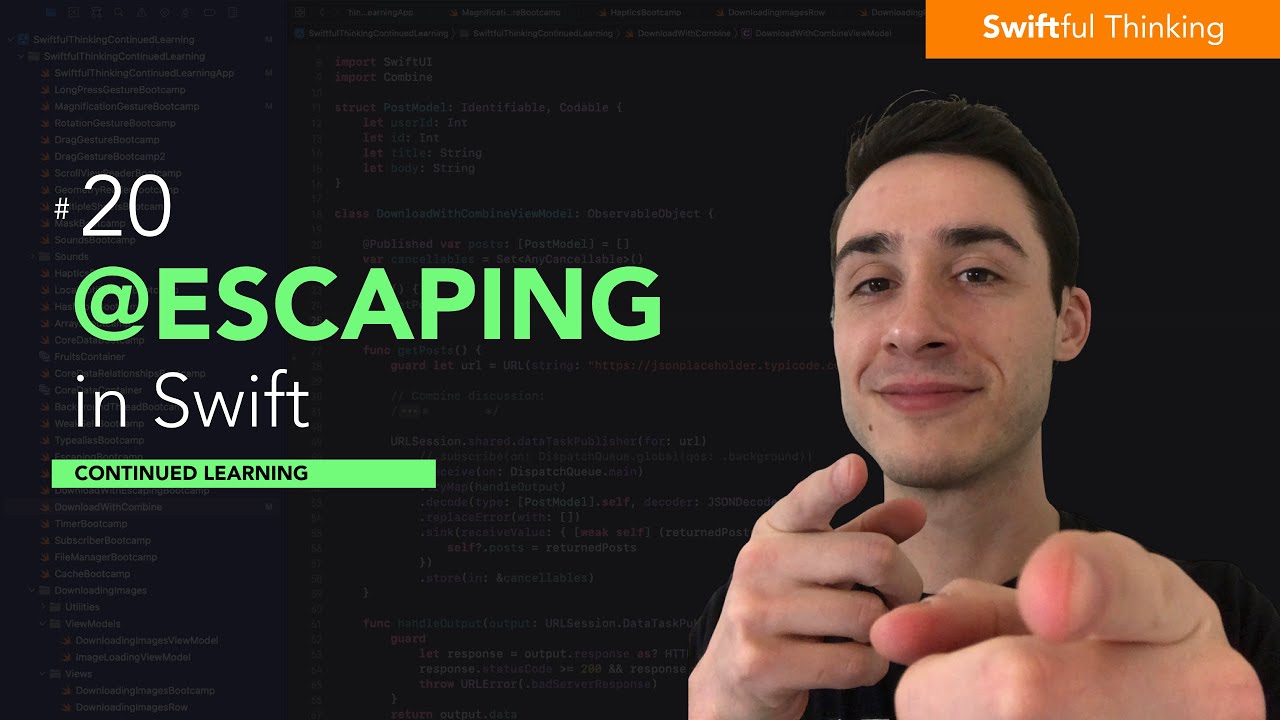
How to use escaping closures in Swift | Continued Learning #20

Aula: Imunologia - Sistema Complemento | Imunologia #8

Hidrolisis Garam (1) | Sifat Garam | Reaksi Hidrolisis

9th Science | Measurements of matter | Chapter 4 | Lecture 2 | maharashtra board |
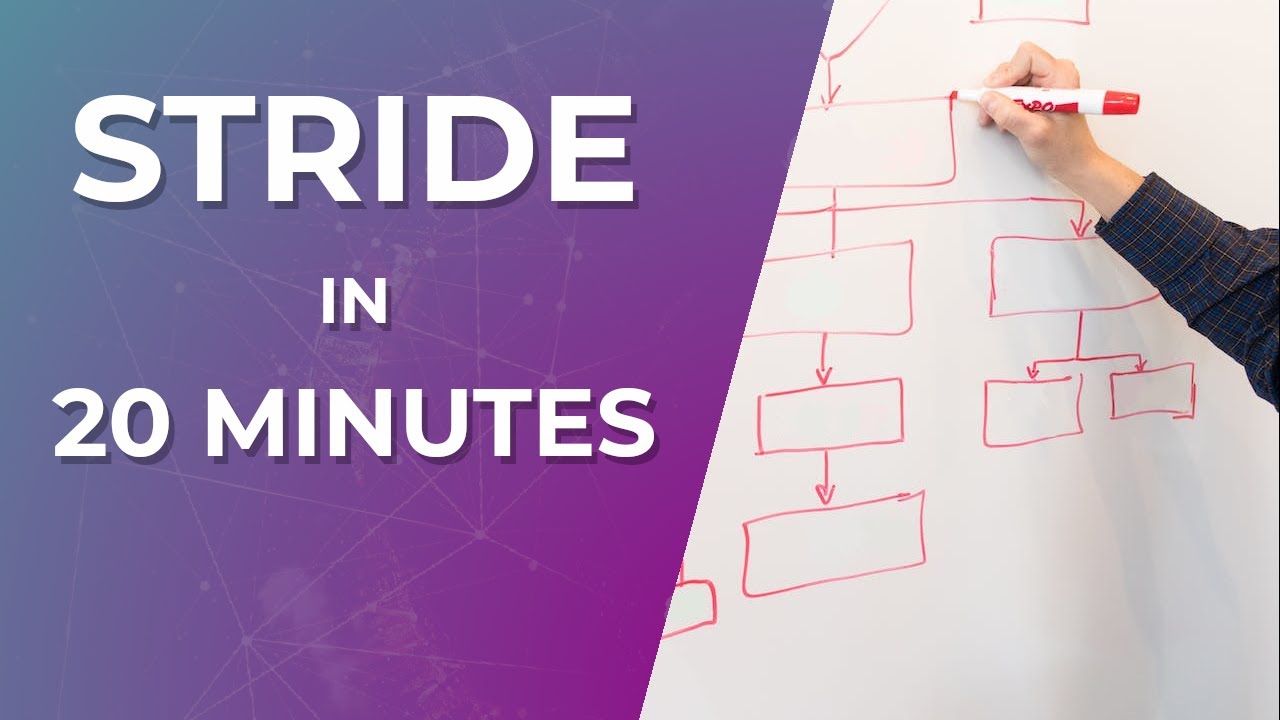
STRIDE Threat Modeling for Beginners - In 20 Minutes
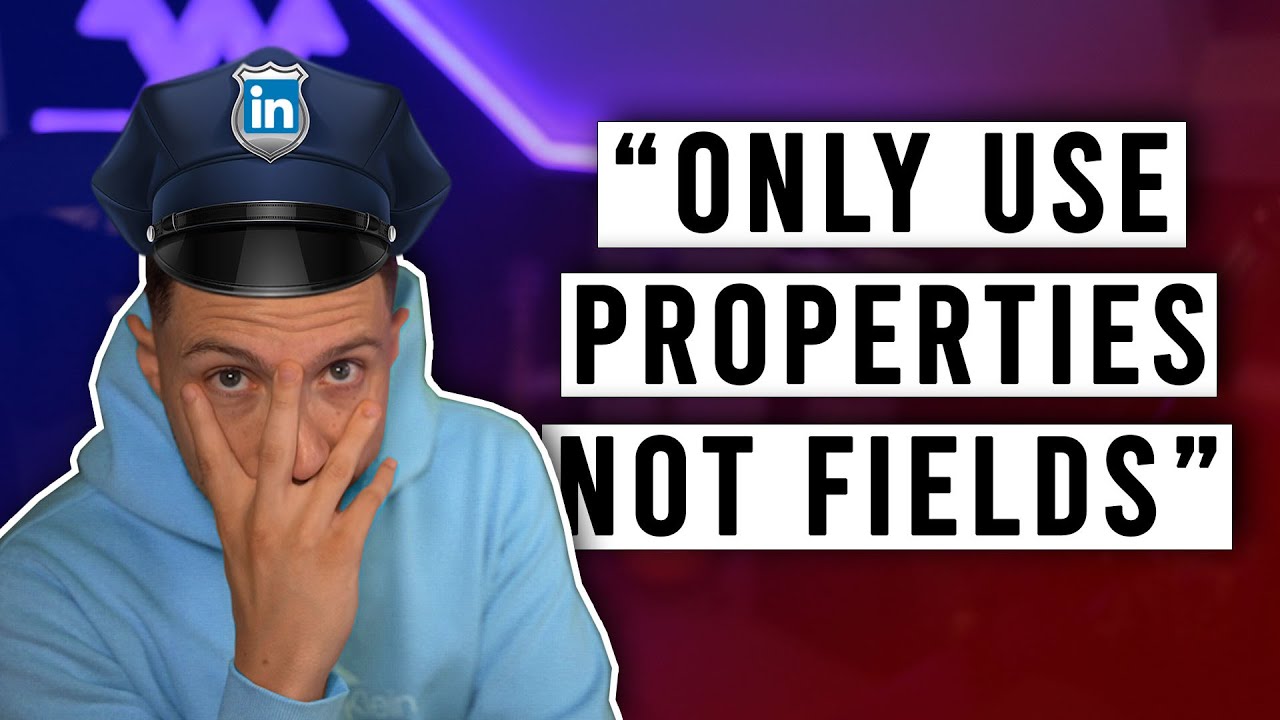
"Don't Use Fields in C#! Use Properties Instead" | Code Cop #003
5.0 / 5 (0 votes)