More Closure Examples | JavaScript ๐ฅ | Lecture 129
Summary
TLDRThis video tutorial explores the concept of closures in JavaScript, offering two illustrative examples to help viewers recognize closures in their own code. The first example demonstrates how a closure enables a function to remember and access variables from its lexical scope, even after that scope has exited. The second example uses a timer to show that closures allow callback functions to access variables from the enclosing function's scope, even after it has executed. These examples clarify that closures form without needing to return a function from another function, showcasing their ability to maintain access to the birthplace variables, thus deepening the understanding of closures and their practical applications in coding.
Takeaways
- ๐ฅ Closures do not require returning a function from another function; they can occur in various situations.
- ๐ The example with variables 'f' and 'g' demonstrates that a closure can capture and remember the environment in which a function was created, allowing access to local variables even after their parent function has executed.
- ๐ Reassigning a function to a variable (like 'f' in the examples) shows how closures can update to encapsulate new environments, demonstrating the dynamic nature of closures.
- ๐ค Closures ensure that functions retain access to the variables of their originating scope, effectively 'remembering' the values at the time they were defined.
- ๐ก The analogy of a variable being inside the 'backpack' of a function helps illustrate how closures carry their scope with them.
- ๐ Modifying the 'f' variable with different functions (as shown with functions 'g' and 'h') highlights how closures adapt to the current scope, changing the variables they enclose.
- ๐ The timer example with 'board passengers' function illustrates closures in asynchronous operations, where functions can access variables from their creation scope even if the parent function has completed.
- ๐ป Closures have precedence over the scope chain, meaning a function will use variables from its closure before considering the global scope.
- ๐ Identifying closures in your code is crucial for understanding behavior, especially in complex scenarios involving callbacks, timers, and asynchronous execution.
- ๐ง The script underscores the importance of closures in JavaScript, showing their versatility and critical role in function scope and execution context management.
Q & A
What is a closure in JavaScript?
-A closure in JavaScript is a feature where an inner function has access to the variables of its outer enclosing function, even after the outer function has executed.
Can closures be created without returning a function from another function?
-Yes, closures can be created without returning a function from another function, as demonstrated in the examples where functions manipulate external variables.
How was the closure demonstrated in the first example with the variable 'f'?
-In the first example, a closure was demonstrated by defining a function 'g' that assigns a new function to the variable 'f', which accesses and modifies an external variable 'a'. Calling 'f' later shows it retains access to 'a', demonstrating closure.
What happens to the closure when the variable 'f' is reassigned to a new function in the examples?
-When 'f' is reassigned to a new function, the closure changes to enclose the variables of the new assignment context, demonstrating that a closure always maintains a connection to the variables present at its 'birthplace'.
How does the second example involving a timer demonstrate a closure?
-The second example demonstrates a closure with a timer by showing that a callback function used in 'setTimeout' retains access to the variables of its enclosing function ('board passengers'), even after the enclosing function has executed.
Why does the callback function in the timer example still have access to 'n' and 'perGroup' variables?
-The callback function retains access to 'n' and 'perGroup' due to closure, which allows it to 'remember' and access variables from its enclosing function's scope, even after the function has executed.
What proves that closures have priority over the scope chain?
-The fact that the callback function in the timer example uses the 'perGroup' variable from its enclosing function's scope, instead of a global 'perGroup' variable, proves that closures have priority over the scope chain.
How is a closure's ability to 'remember' variables from its birthplace significant in JavaScript?
-A closure's ability to 'remember' variables from its birthplace is significant because it ensures that a function does not lose connection to its defining environment's variables, allowing for powerful programming patterns like encapsulation and factory functions.
What is the impact of reassigning a function to the variable 'f' on its closure?
-Reassigning a function to the variable 'f' updates its closure to enclose variables from the new assignment's context, illustrating how closures adapt to reflect the current scope of a function.
How does the concept of closures enhance the identification of them in code?
-Understanding closures helps in identifying them in code by recognizing patterns where functions access and manipulate variables from an outer scope, even after the outer function has executed, enabling more effective debugging and code optimization.
Outlines
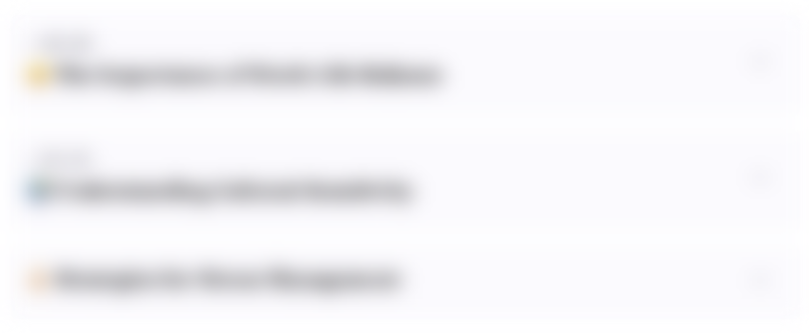
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
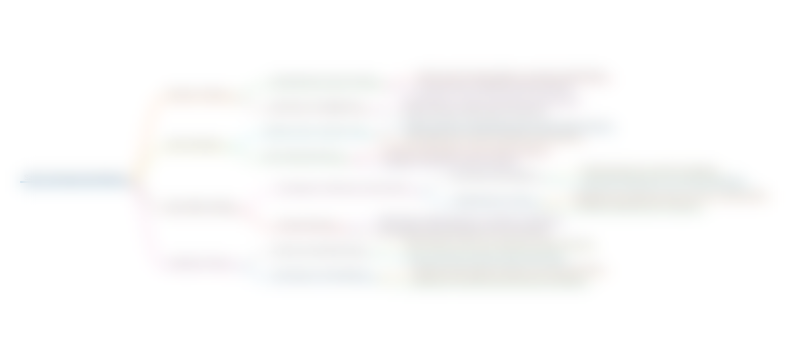
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
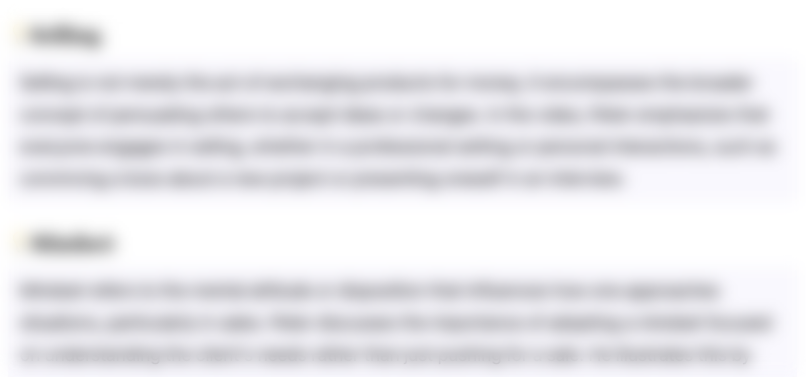
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
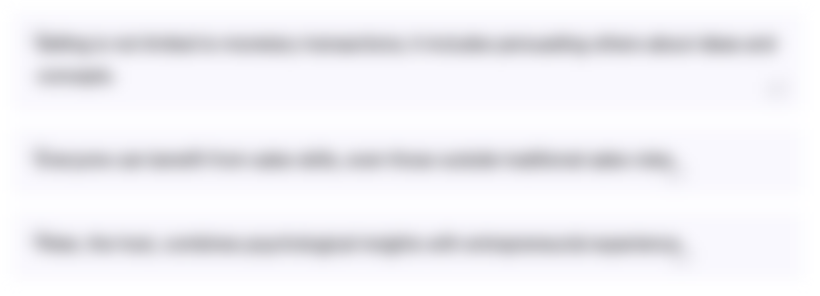
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
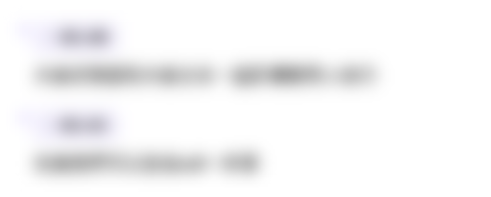
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Closures | JavaScript ๐ฅ | Lecture 128
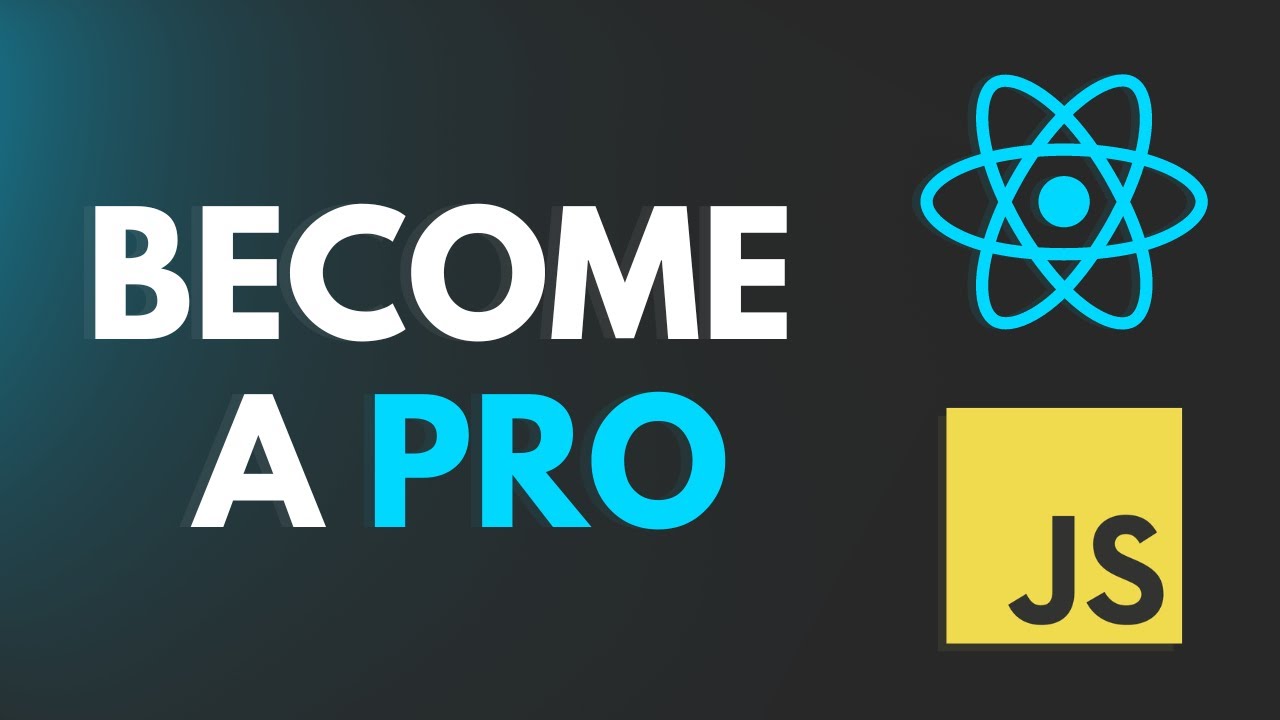
3 JavaScript Concepts that Will Make You A Better React Developer
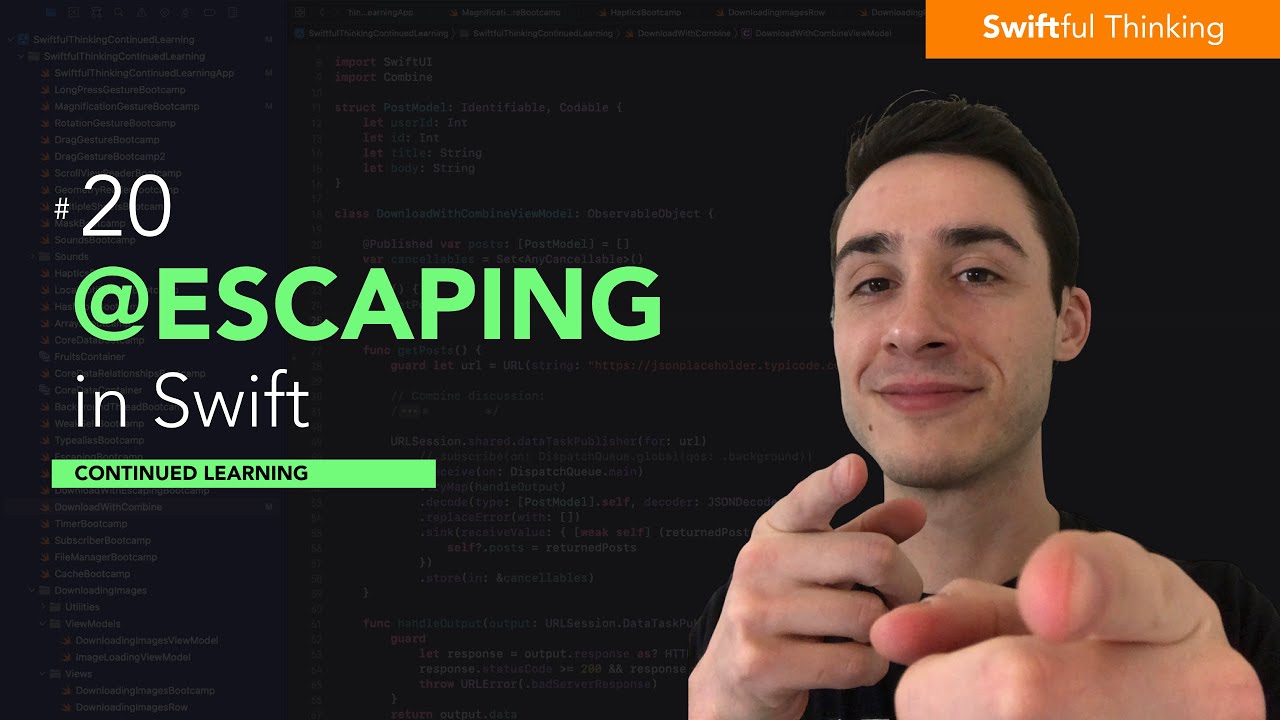
How to use escaping closures in Swift | Continued Learning #20

#8 Teori Bahasa & Otomata - ฮต-move dan ฮต-closure pada Non Deterministic Finite State Otomata

Learn Closures In 7 Minutes

Boot Camp Day 6: Break of Structure
5.0 / 5 (0 votes)