Swift Closures: @escaping Explained
Summary
TLDRThis video delves into Swift's escaping and non-escaping closures, focusing on their practical differences and usage scenarios. Sponsored by DevMountain, a boot camp for coding and design, the presenter uses a network call example to illustrate the necessity of an escaping closure when the function's execution extends beyond its scope, such as waiting for a network response. The video also touches on the importance of avoiding retain cycles when using escaping closures, providing a clear explanation of when and why to use each type of closure in Swift.
Takeaways
- π The video discusses the concept of escaping and non-escaping closures in Swift and their practical applications.
- π« The video is sponsored by DevMountain, a coding and design boot camp offering various programs including iOS development.
- π The presenter is a boot camp graduate and has been an iOS developer for five years, providing credibility to the discussion.
- π DevMountain offers career services and financing options, and they are interested in feedback from the presenter's viewers.
- π The 'escaping' keyword in Swift is used in the context of closures that need to outlive the function they are passed to, such as in network calls.
- π The example given is a network call to a GitHub API to fetch a list of followers, which is a common scenario for using escaping closures.
- ποΈ Non-escaping closures are executed immediately within the scope of the function they are defined in, without needing to 'escape'.
- β±οΈ Escaping closures are used when a task, like a network call, takes time to complete and the closure needs to wait for the result.
- π The presenter mentions automatic reference counting in Swift, which is crucial for understanding how escaping closures work in memory.
- π The video touches on the importance of avoiding retain cycles when using escaping closures, as they maintain a reference to the function's context.
- π¨βπ« The presenter offers to create courses and invites viewers to check out their site for more educational content.
Q & A
What is the main topic of the video?
-The main topic of the video is about explaining the concept of escaping and non-escaping closures in Swift.
What does the term 'escaping' refer to in the context of closures in Swift?
-In the context of closures in Swift, 'escaping' refers to a closure that needs to outlive the function it is passed to, often because it is used asynchronously, such as in a network call.
Why is the 'escaping' keyword used in the 'get followers' function example?
-The 'escaping' keyword is used in the 'get followers' function example because the closure needs to execute after the function has completed, specifically after a network call has returned its data.
What is the difference between escaping and non-escaping closures?
-Escaping closures are those that need to outlive the function they are passed to, often due to asynchronous execution. Non-escaping closures, on the other hand, are executed immediately within the scope of the function they are passed to.
What is the common scenario presented in the video where an escaping closure is used?
-The common scenario presented in the video where an escaping closure is used is making a network call, such as fetching a list of followers from an API.
Why is it important to understand the difference between escaping and non-escaping closures?
-Understanding the difference between escaping and non-escaping closures is important to manage memory correctly and avoid retain cycles, especially when closures are used in asynchronous tasks.
What is the role of the 'self' reference in the context of escaping closures?
-In the context of escaping closures, the 'self' reference is used to prevent retain cycles and to ensure that the closure has access to the instance of the class or struct it was created in, as it may outlive the original function's execution.
What is the sponsor of the video mentioned in the script?
-The sponsor of the video is DevMountain, an in-person coding and design boot camp that offers housing at no extra cost for full-time immersive students.
What additional services does DevMountain offer besides their iOS development program?
-DevMountain offers additional services such as programs in web development, software QA, and UX design, as well as a career services team to help with job placement and financing options.
What is the significance of the 'weak self' capture list in the context of the script?
-The 'weak self' capture list is used to prevent strong reference cycles in closures that capture 'self'. It ensures that the closure does not inadvertently keep a strong reference to the instance it is part of, thus avoiding memory leaks.
How does the video help the viewer understand the practical application of escaping closures?
-The video helps the viewer understand the practical application of escaping closures by demonstrating a real-world example of making a network call to fetch data and then using the closure to update the UI once the data is received.
Outlines
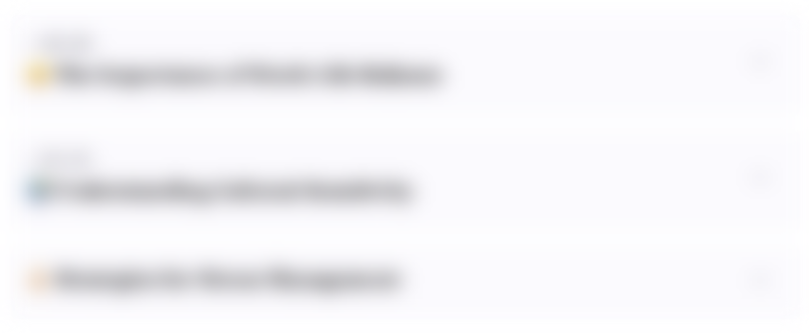
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
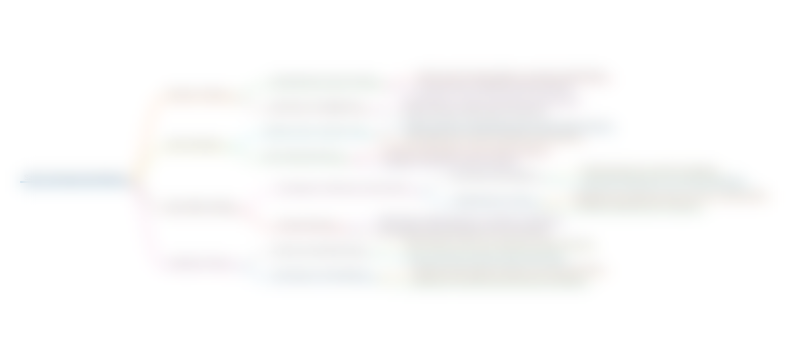
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
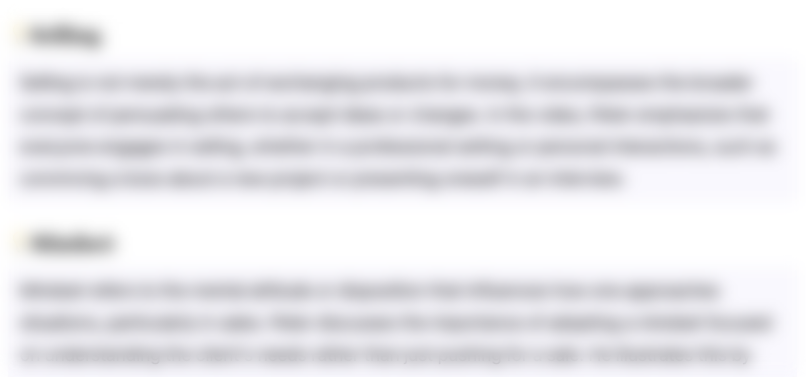
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
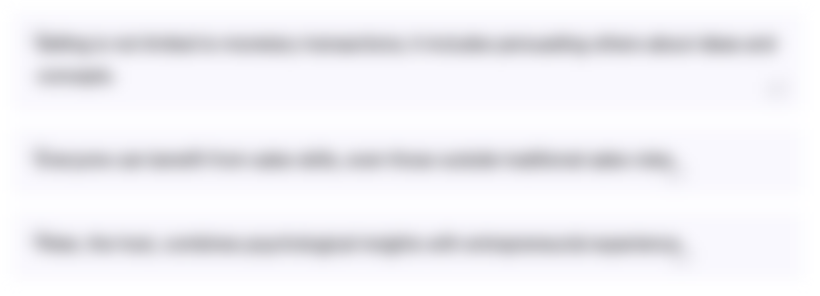
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
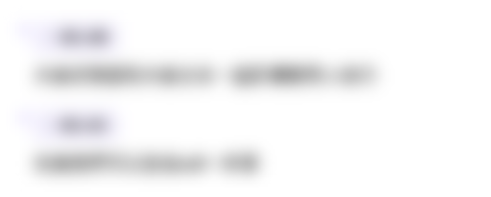
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
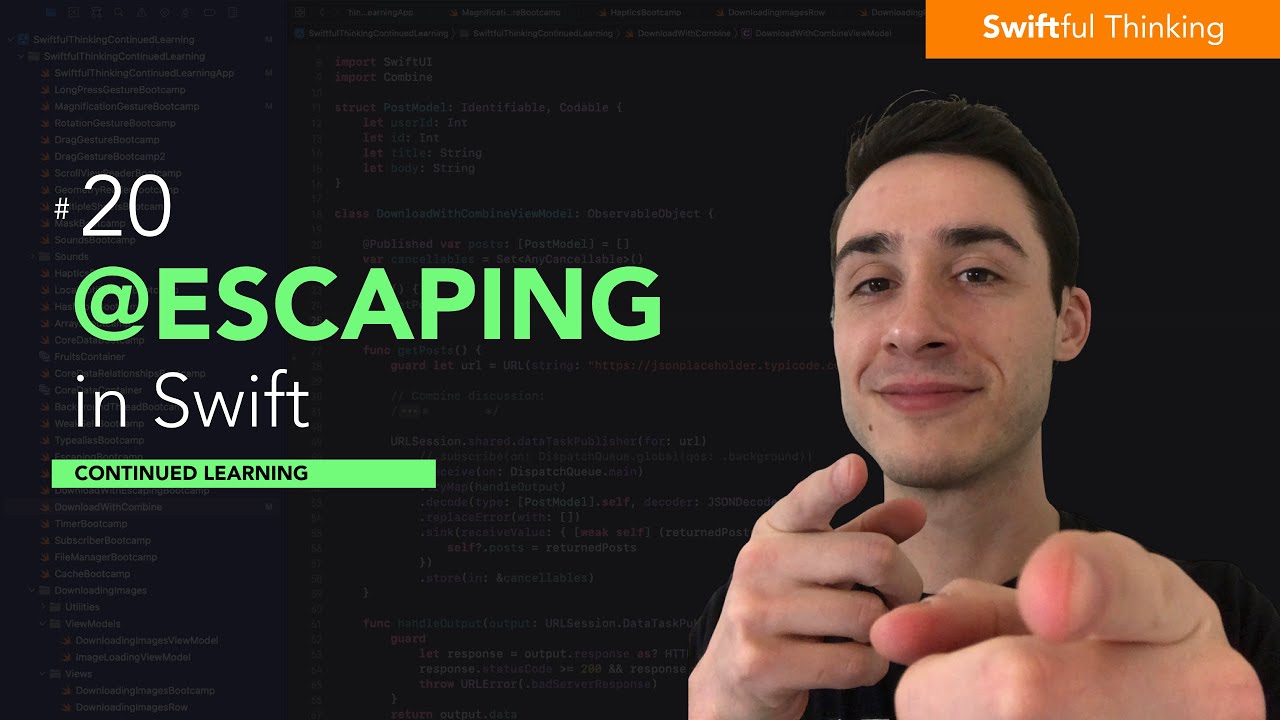
How to use escaping closures in Swift | Continued Learning #20
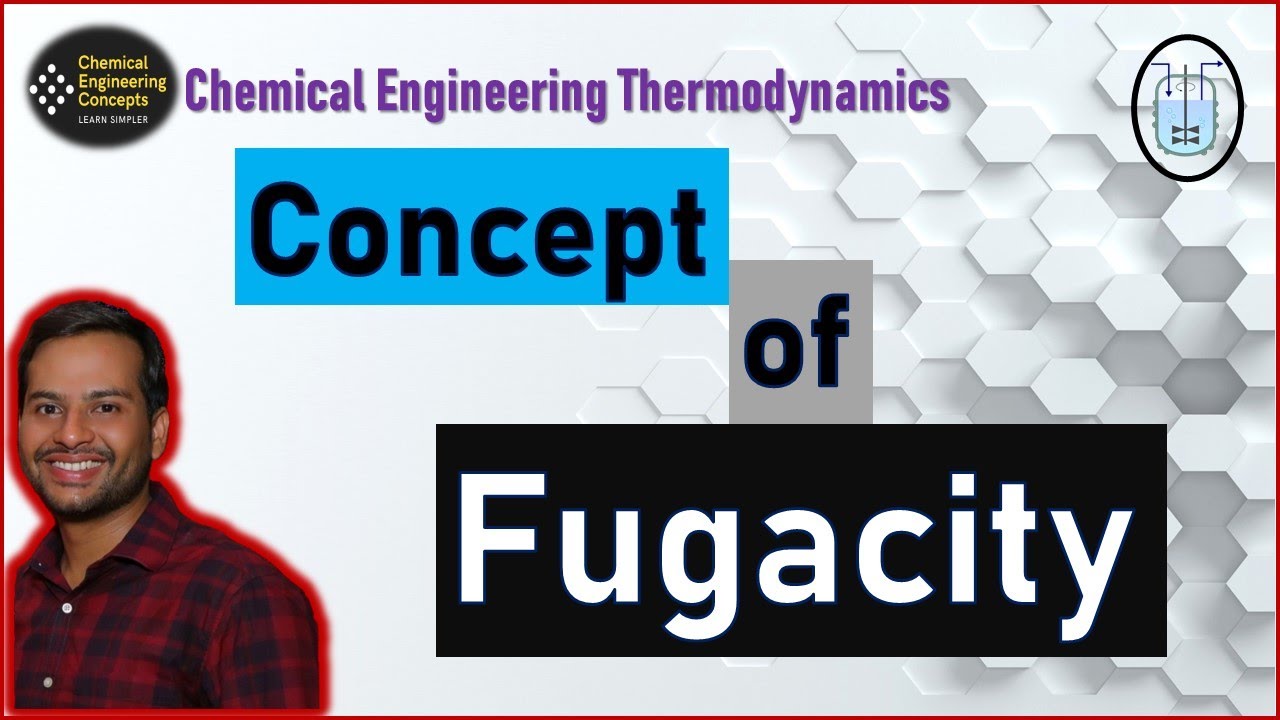
Concept of Fugacity || Solution Thermodynamics || Chemical Engineering

Eurocentrism: a many-layered thing. | Halil Berktay | TEDxIbnHaldunUniversity

Piaget's Concrete Operational Stage

What If You Fell In A Quicksand? | How to Survive QUICKSAND | Dr Binocs Show | Peekaboo Kidz
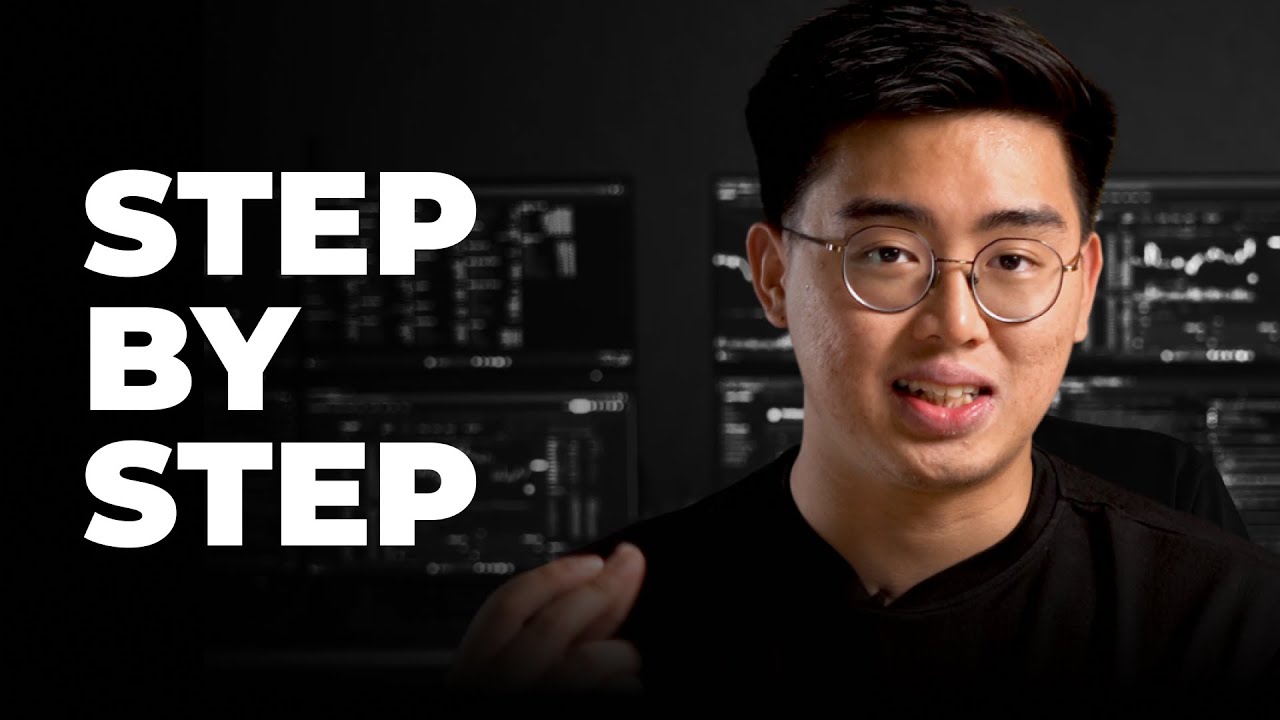
Cara Keluar Dari Kemiskinan
5.0 / 5 (0 votes)