Closures | JavaScript 🔥 | Lecture 128
Summary
TLDRThis video script delves into the intricate world of closures in JavaScript, a concept often deemed challenging yet deeply powerful. The instructor methodically demystifies closures, guiding learners through their inner workings using relatable analogies and practical examples. By linking closures to familiar notions like execution contexts, scope chains, and variable environments, the video unveils the seamless interplay that allows functions to retain access to variables from their birthplaces, even after those environments have ceased to exist. With its comprehensive explanations, code walkthroughs, and insightful definitions, this script illuminates a pivotal JavaScript feature, empowering developers to harness its capabilities confidently.
Takeaways
- 🔑 Closures are an automatic mechanism in JavaScript where a function remembers the variables from the environment in which it was created, even after that environment is gone.
- 🧩 Closures allow functions to access and manipulate variables from their parent's scope, even after the parent function has finished executing.
- 🕰️ Closures preserve the scope chain and variable environment of a function, even when the execution context in which the function was created is no longer on the call stack.
- 🎁 A closure is like a function carrying a 'backpack' containing all the variables that existed at the function's birthplace, which it can access anytime.
- 🌍 Closures ensure that a function never loses its connection to the variables from its 'hometown' (parent scope), even after its parent scope is gone.
- 🔎 Closures are not something we create manually; JavaScript handles them automatically when functions are created within other functions.
- 🚫 We cannot directly access or manipulate the closed-over variables in a closure, but we can observe the closure's effects.
- 🧠 Understanding closures requires a solid grasp of execution contexts, the call stack, and the scope chain.
- 🔗 Closures bring together concepts like execution contexts, call stacks, and scope chains in a beautiful and powerful way.
- 🚀 Mastering closures is crucial for becoming a confident and proficient JavaScript programmer.
Q & A
What is a closure in JavaScript?
-A closure in JavaScript is a feature where an inner function has access to the variables of its outer (enclosing) function's scope, even after the outer function has executed and its execution context is no longer on the call stack.
How are closures created in JavaScript?
-Closures are created automatically in certain situations when an inner function is defined within an outer function. This happens without the need for explicit actions by developers, like manually creating closures.
Why is the concept of closures considered difficult to understand by many developers?
-Many developers find closures difficult to understand because they involve several JavaScript concepts like execution context, call stack, and scope chain, working together in a complex way.
What is the 'secure booking' function's role in demonstrating closures?
-The 'secure booking' function demonstrates closures by encapsulating a 'passengerCount' variable and returning an inner function that modifies this variable, showcasing how the inner function retains access to the outer function's variables even after the outer function has executed.
Can closures be manually created like objects or arrays?
-No, closures are not created manually like objects or arrays. They occur automatically in certain situations when functions are created and executed.
How does the 'Booker' function illustrate the concept of closures?
-The 'Booker' function, returned from 'secure booking', illustrates closures by accessing and modifying the 'passengerCount' variable of its parent function, demonstrating how inner functions retain access to the variables of their outer functions.
Why can the 'Booker' function still access the 'passengerCount' variable after the 'secure booking' function has finished executing?
-The 'Booker' function can still access the 'passengerCount' variable due to closures, which allow functions to remember and access the variables from their birthplace's scope, even after that scope's execution context is gone.
What is the significance of the variable environment in closures?
-The variable environment in closures is significant because it contains all the local variables of a function's execution context. It is attached to the inner function, allowing it to access and manipulate these variables even after the outer function's execution context has been popped off the call stack.
How does the concept of closures alter the traditional understanding of the scope chain?
-Closures extend the traditional understanding of the scope chain by preserving the scope chain through the closure, even after a function's execution context has been destroyed, allowing inner functions to access variables from their enclosing functions' scopes.
How can developers observe closures in action within their code?
-Developers can observe closures in action by using `console.dir` to inspect functions in the console. This method allows them to see the 'scopes' internal property, where the closure's variable environment, including accessible variables like 'passengerCount', is displayed.
Outlines
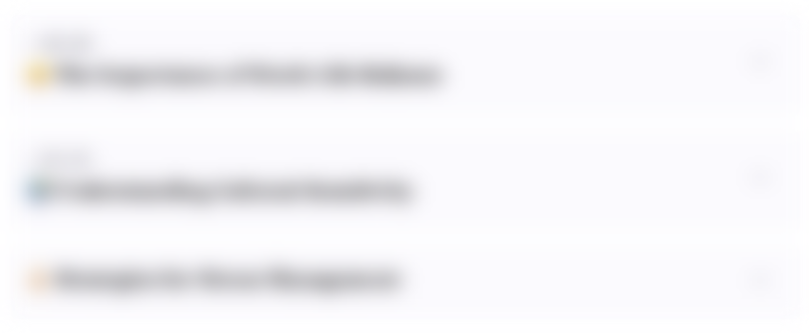
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
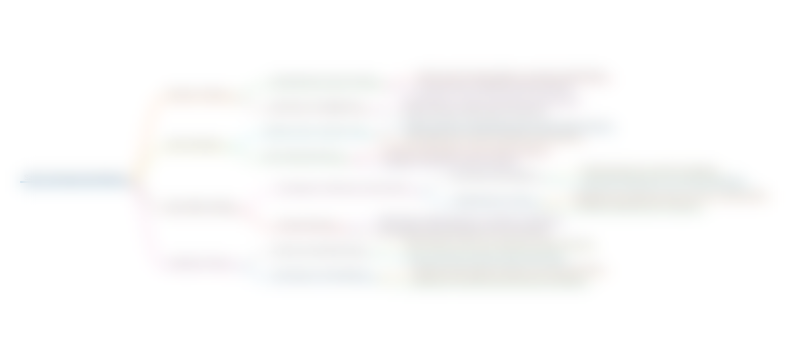
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
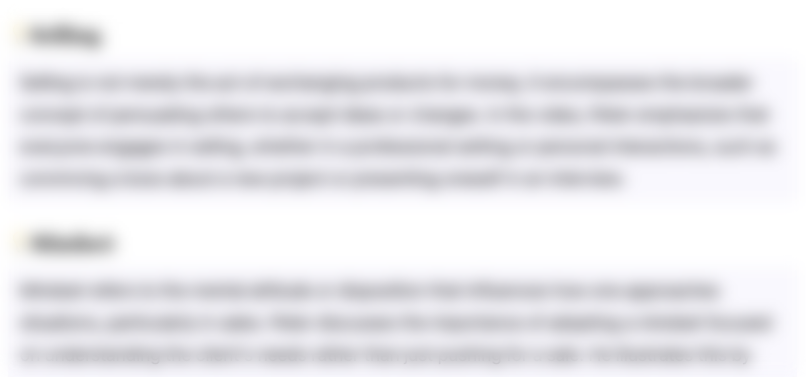
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
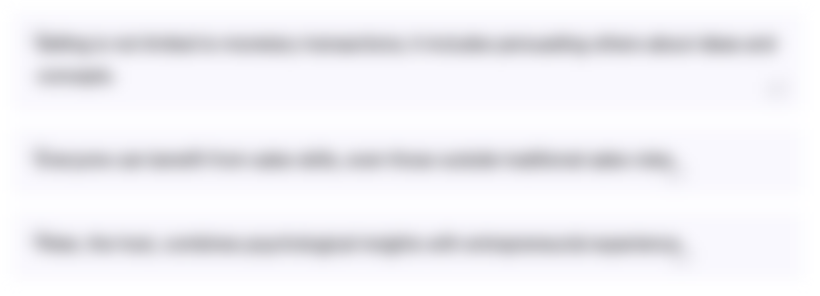
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
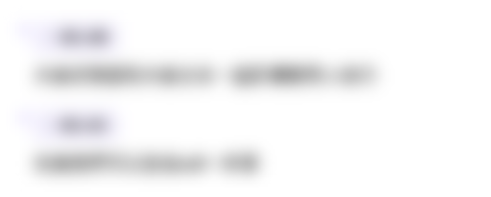
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
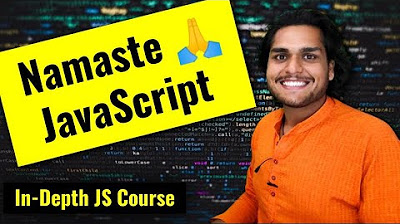
Namaste JavaScript 🙏 Course - JS Video Tutorials by Akshay Saini

Learn Closures In 7 Minutes

JavaScript Visualized - Execution Contexts

Ecosystem Ecology: Links in the Chain - Crash Course Ecology #7

Noam Chomsky - The 5 Filters of the Mass Media Machine
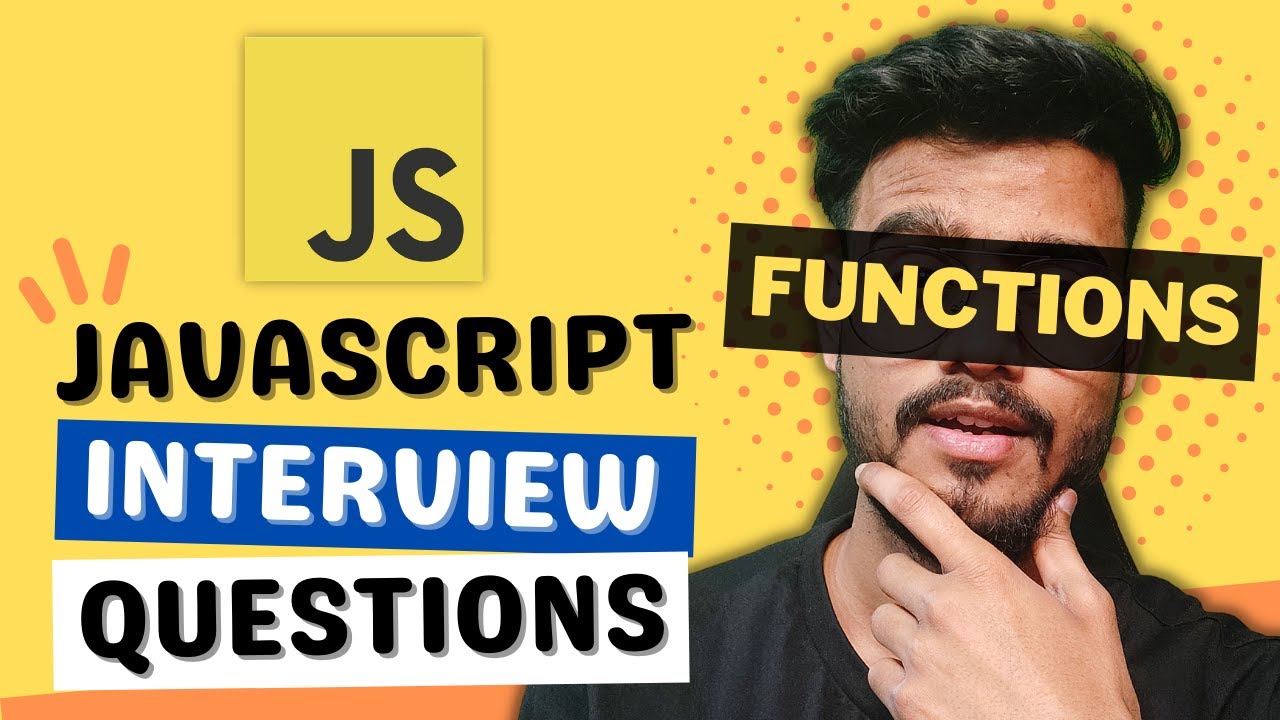
Javascript Interview Questions ( Functions ) - Hoisting, Scope, Callback, Arrow Functions etc
5.0 / 5 (0 votes)