01. Stopwatch/Count-Up Timer || Learn React Through Mini Projects
Summary
TLDRThis tutorial demonstrates how to build a simple React stopwatch app in under 20 minutes. It covers setting up the project, using React hooks like useState, performing calculations, adding start/stop/reset buttons, utilizing useEffect for side effects, and applying basic Tailwind CSS for styling.
Takeaways
- 🛠️ Start by setting up a React project using `create-react-app` and name it 'stopwatch'.
- ⏱ Use the `useState` hook to track the stopwatch's time and provide a method to update it.
- 📝 Destructure the `useState` return value to get the current state and the function to update it, initializing with zero.
- 🔢 Calculate the stopwatch display time by dividing the milliseconds and using the remainder operator to format minutes and seconds.
- 🔴 Add buttons for start, stop, and reset functionalities, updating the 'running' state accordingly.
- 🔁 Use the `useEffect` hook to handle side effects like starting and stopping the timer with `setInterval` and `clearInterval`.
- ✂️ Include the 'running' state in the `useEffect` dependencies to ensure the timer stops when the component unmounts.
- 🔄 Implement conditional rendering to show the stop button when the stopwatch is running and the start button when it's not.
- 🎨 Introduce basic styling with Tailwind CSS, which can be expanded as the project progresses.
- 🔗 Provide links to resources such as W3Schools for understanding `setInterval` and Tailwind CSS documentation for styling.
- 🎉 Complete a functioning stopwatch app in six steps, demonstrating practical React and CSS skills in under 20 minutes.
Q & A
What is the main purpose of the video script?
-The main purpose of the video script is to provide a step-by-step guide on how to create a simple React stopwatch application using Tailwind CSS.
What is the first step mentioned in the script for setting up a React project?
-The first step is to use 'create-react-app' to set up the project and name it 'stopwatch'.
What is a React hook and why is 'useState' considered a React hook?
-A React hook is a special function that allows you to 'hook into' React state and other features without writing a class. 'useState' is a hook that helps track state or properties between function calls.
What does the 'useState' hook return and how is it used in the script?
-The 'useState' hook returns an array with the current state value and a function to update it. In the script, it is used to initialize the 'time' state to zero.
How is time calculated in the stopwatch application?
-Time is calculated by dividing the elapsed time in milliseconds by the number of milliseconds per unit of time, using the remainder operator to get the seconds, minutes, and milliseconds.
What are the functionalities provided by the buttons in the stopwatch application?
-The buttons provide functionalities for starting, stopping, and resetting the stopwatch.
What is the 'useEffect' hook used for in the script?
-The 'useEffect' hook is used to perform side effects in React components, such as fetching data, manually changing the DOM, or setting up timers. In the script, it is used to start and clear the interval for the stopwatch.
Why is 'running' used as a dependency in the 'useEffect' hook?
-Using 'running' as a dependency ensures that the effect (starting or clearing the interval) only runs when the 'running' state changes, preventing unnecessary re-renders.
What is conditional rendering and how is it used in the script?
-Conditional rendering is a technique in React for rendering different components or elements based on certain conditions. In the script, it is used to show the stop button when the timer is running and the start button when it is not.
How is Tailwind CSS introduced in the script?
-Tailwind CSS is introduced as a basic styling tool for the stopwatch application, with a mention of the documentation for further customization.
What is the final outcome of following the script?
-The final outcome is a functioning stopwatch application created in React with basic styling using Tailwind CSS, completed in six steps and under 20 minutes.
Outlines
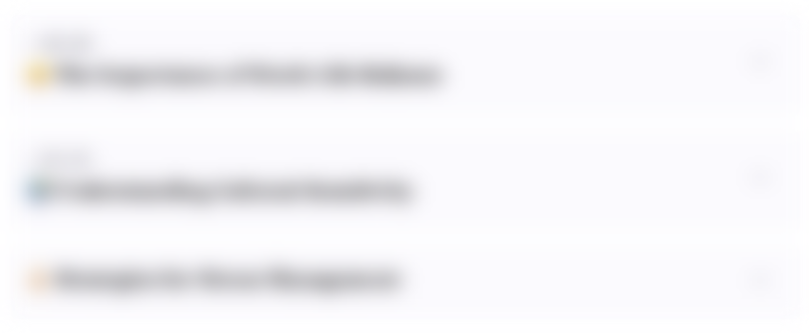
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
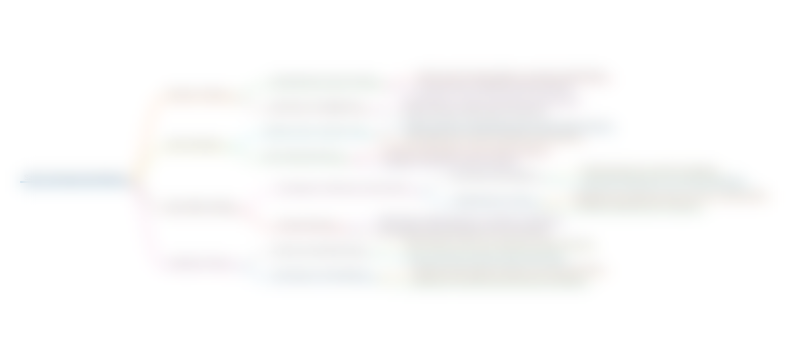
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
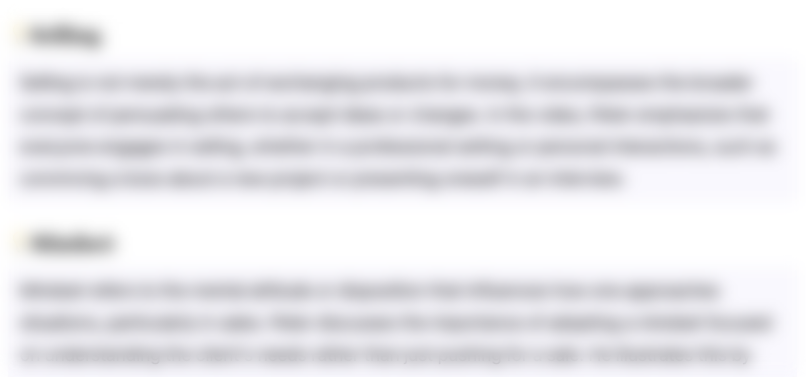
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
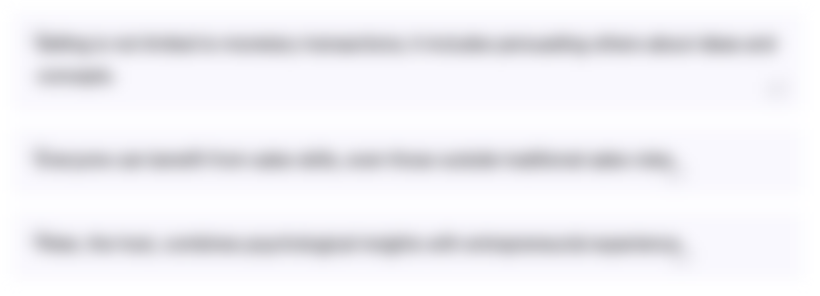
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
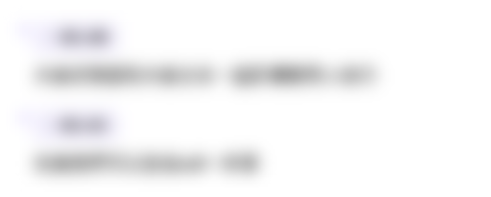
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Build an AI Voice Translator: Keep Your Voice in Any Language! (Python + Gradio Tutorial)
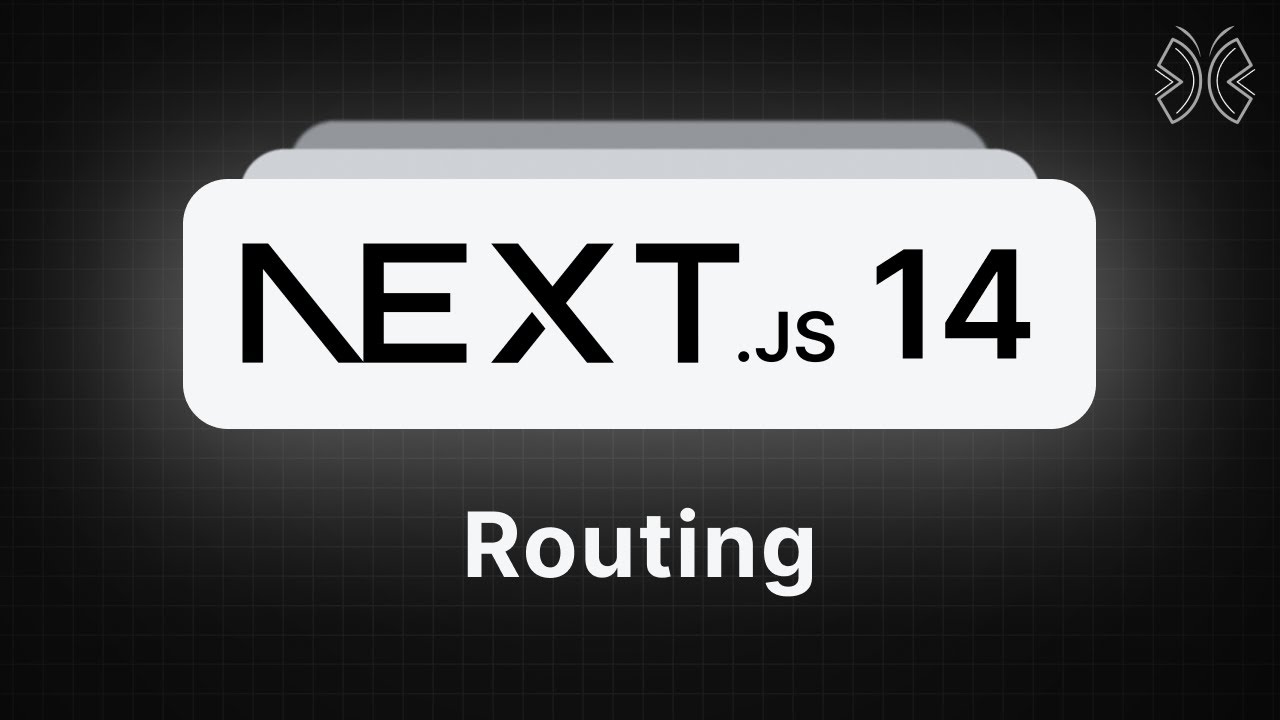
Next.js 14 Tutorial - 5 - Routing

Want to MASTER React JS? Watch This Now
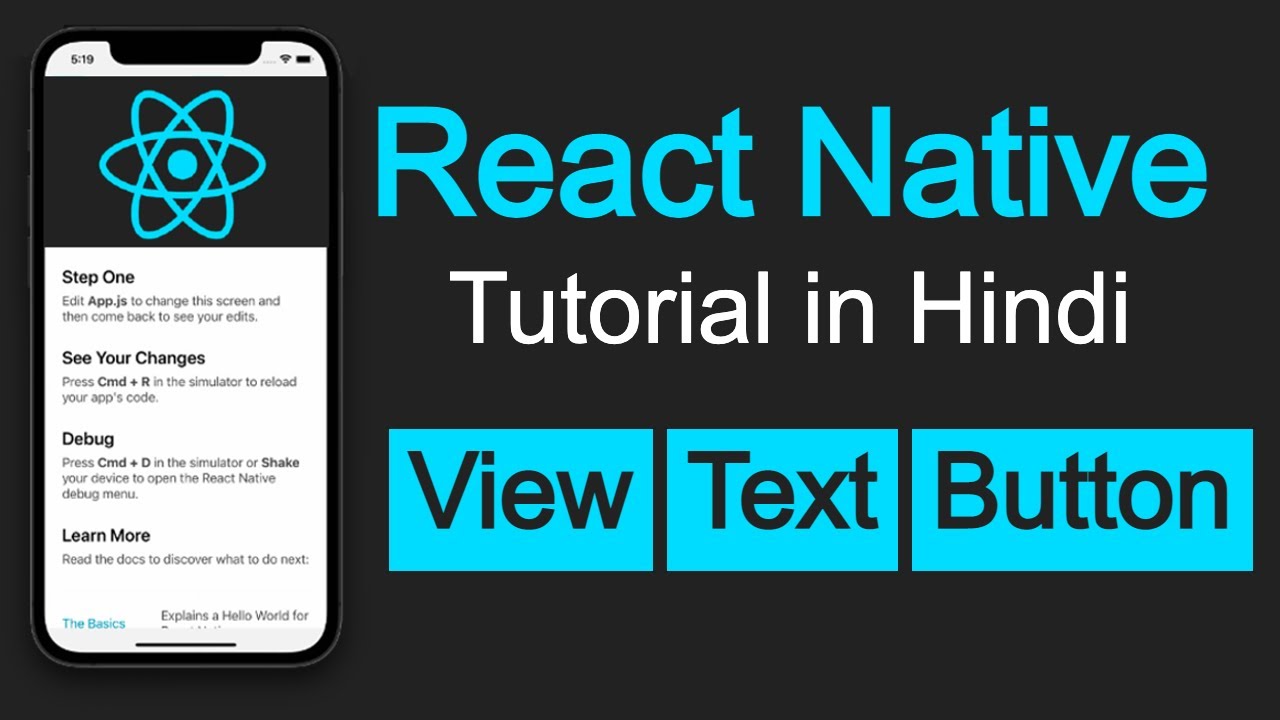
React native tutorial in Hindi #5 Basics of View, Text and Button UI
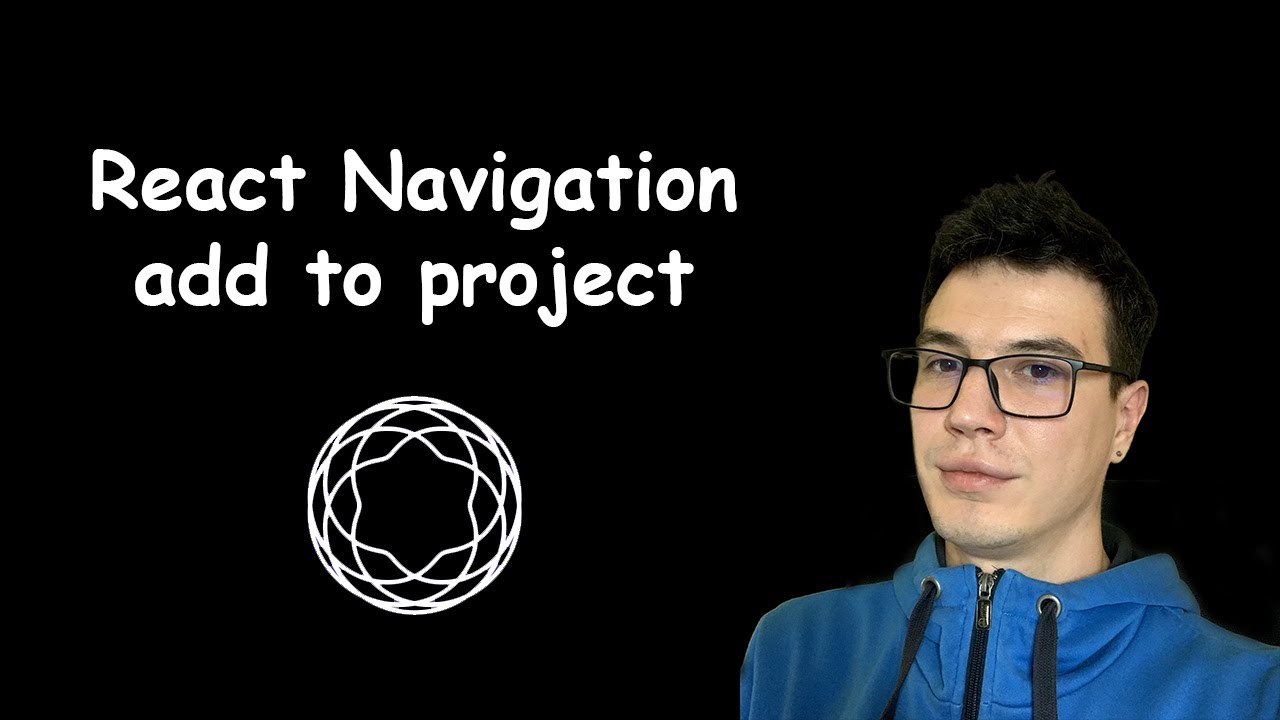
#5 - Add React Navigation | React Native open-source eCommerce App
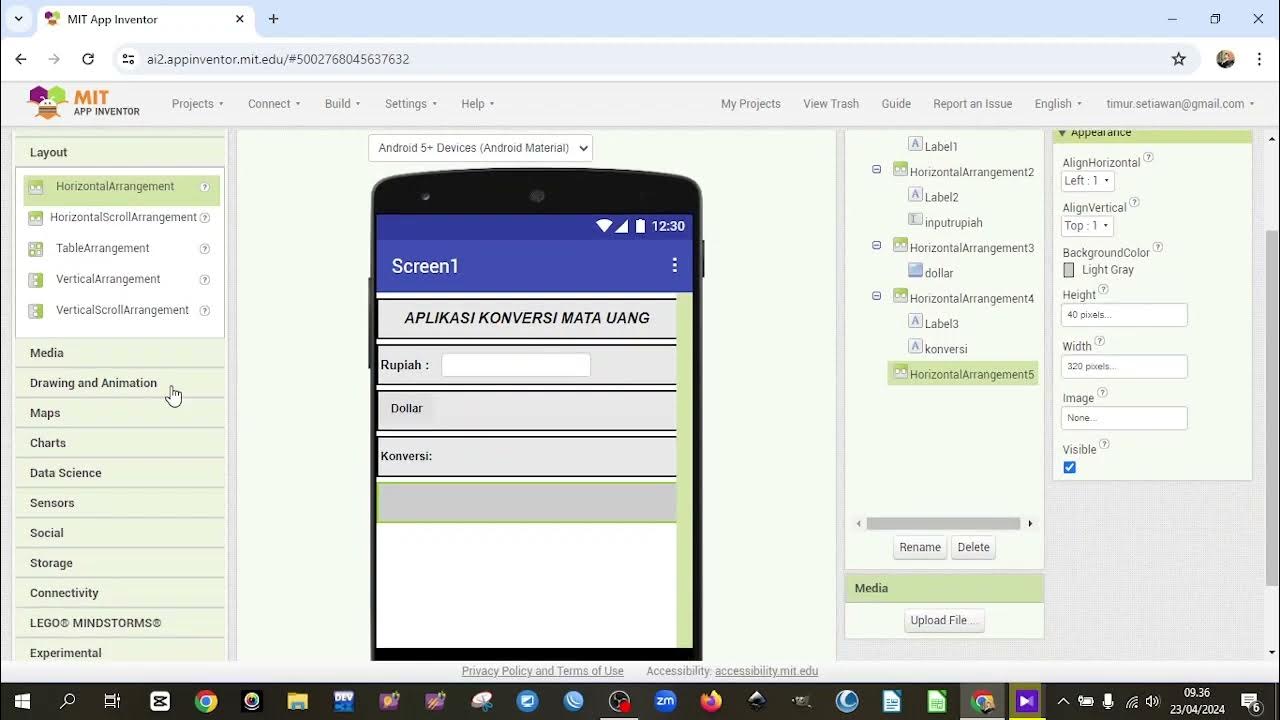
2. TUTORIAL MEMBUAT APLIKASI KONVERSI MATA UANG DENGAN MIT APP INVENTOR
5.0 / 5 (0 votes)