Arrays | Godot GDScript Tutorial | Ep 10
Summary
TLDRThis episode of the GD Script Fundamental Tutorial series delves into the concept of arrays in Godot programming. Arrays are introduced as sequential collections of items stored in contiguous memory locations, capable of holding different data types. The tutorial explains array indexing, including normal and inverse positions, and demonstrates how to declare, manipulate, and access array elements in GDScript. It covers sub-arrays, array methods like push, pop, clear, and duplicate, and the importance of choosing between deep and shallow duplication. The episode concludes with practical examples and encourages viewers to download a GitHub project for hands-on experience, aiming to solidify their understanding of array manipulation in Godot.
Takeaways
- 📚 Arrays in GDScript are collections of items stored in contiguous memory locations.
- 🔢 Arrays can contain elements of different data types, including other arrays and dictionaries.
- 👤 The position of an item in an array is called an index, starting at 0 for the first element.
- 🔄 You can use both positive and negative indices to access elements in an array, with negative indices accessing elements from the end.
- 📝 To declare an array in GDScript, use square brackets with values separated by commas.
- 🔑 Retrieving a specific element from an array requires using the variable name followed by square brackets with the index.
- 💡 GDScript supports sub-arrays, which are arrays within arrays, and can be multi-dimensional.
- 🔄 The push method adds elements to the end of an array, while the pop method removes elements from the beginning or end.
- 🗑️ Clearing an array can be done by reassigning an empty array, using the resize method, or calling the clear method.
- 🔍 The duplicate method creates a copy of an array, with options for deep or shallow duplication.
- 📏 The size method returns the length of an array, which is the last index position plus one.
Q & A
What is an array in the context of programming?
-An array is a collection of items stored at contiguous memory locations, allowing for the storage of multiple items together in a sequential manner.
Can arrays in Godot contain elements of different data types?
-Yes, in Godot, arrays can contain elements of different data types, including numbers, strings, and even other arrays and dictionaries.
How is the position of an element in an array referred to?
-The position of an element in an array is referred to as its index position, which starts at 0 for the first element.
What is an inverse index position and how is it used?
-An inverse index position is a way to access elements from the end of an array using negative numbers, with -1 referring to the last element and -2 referring to the second-to-last element.
How do you declare an array in Godot's GDScript?
-In GDScript, you declare an array by using square brackets. An empty array is declared with '[]', and an array with predetermined values is declared by listing the values separated by commas within the brackets.
How can you retrieve a specific element from an array?
-To retrieve a specific element, you type out the variable name followed by square brackets containing the index position of the desired element.
What are sub-arrays and how are they created?
-Sub-arrays are arrays within arrays, also known as multi-dimensional arrays. They are created by using square brackets followed by another pair of square brackets.
How do the push and pop methods work in GDScript arrays?
-The push method adds an element to the beginning or end of an array, while the pop method removes and returns an element from the beginning or end of an array.
What is the difference between a deep copy and a shallow copy when duplicating arrays in GDScript?
-A deep copy duplicates all nested arrays and dictionaries, creating independent copies that do not affect the original. A shallow copy, however, keeps references to the original nested arrays and dictionaries, meaning changes to these nested structures in the copy will also impact the original.
How can you get the length of an array in GDScript?
-To get the length of an array, you call the 'size' method on the array, which returns an integer representing the number of elements in the array.
What are the different ways to clear an array in GDScript?
-There are three ways to clear an array in GDScript: 1) Assigning an empty array to the variable, 2) Using the 'resize' method with a length of 0, and 3) Calling the 'clear' method on the array.
Outlines
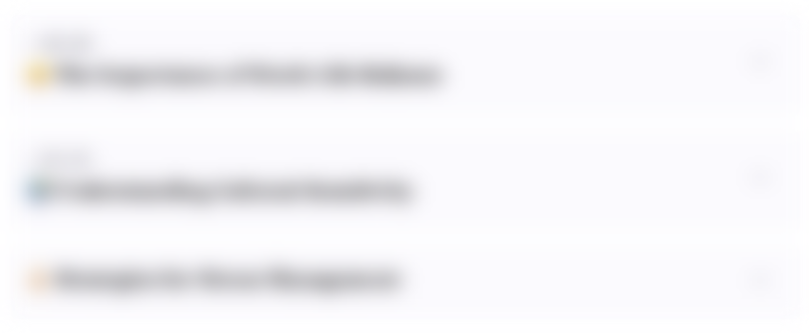
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
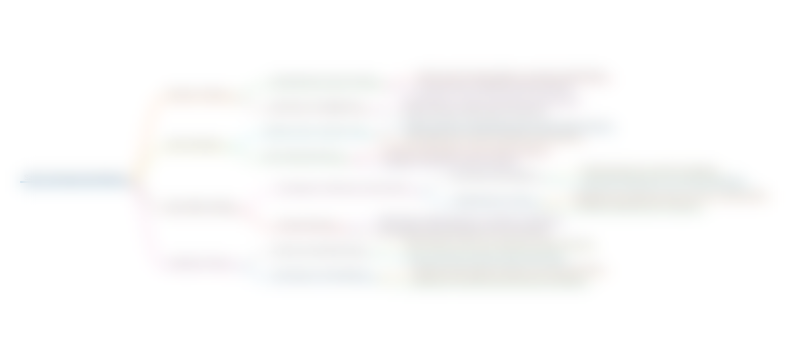
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
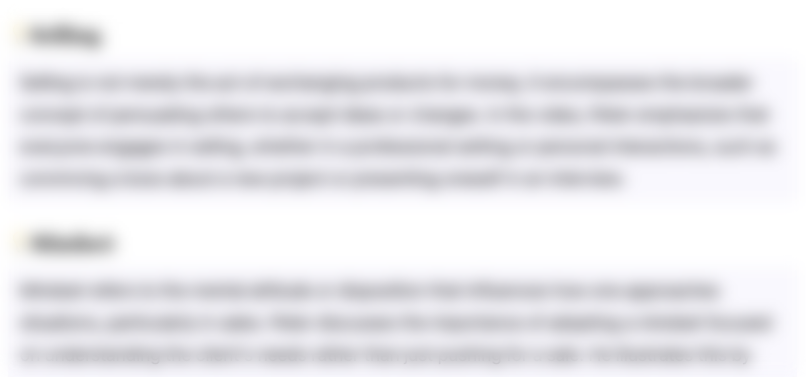
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
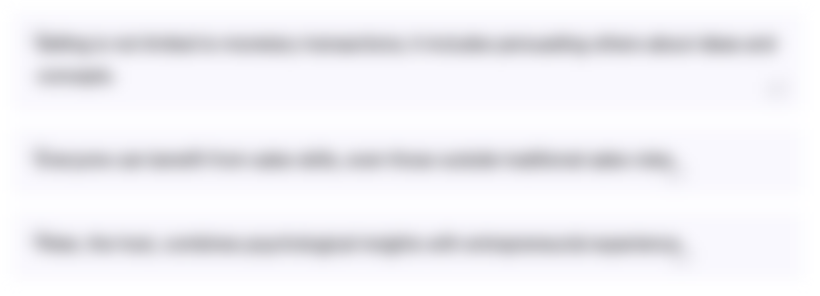
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
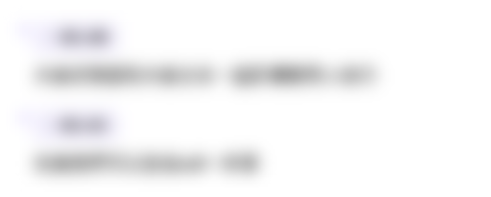
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
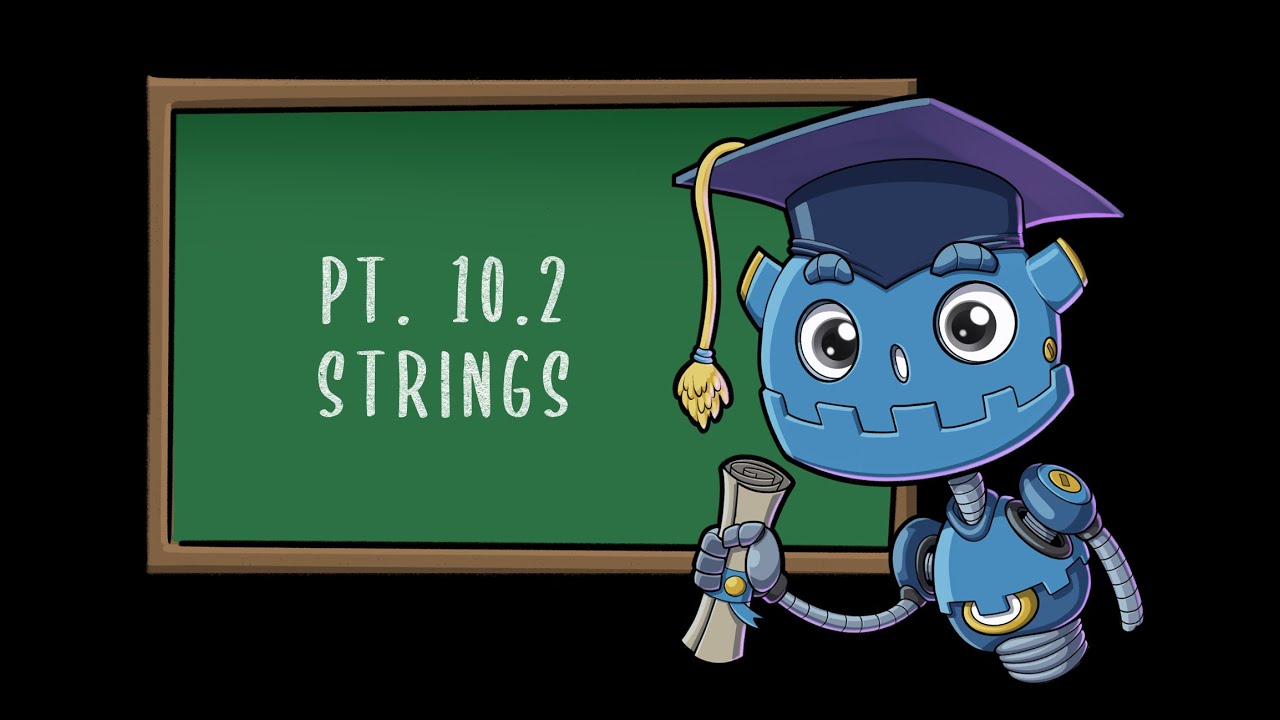
Strings (Basics of Memory) | Godot GDScript Tutorial | Ep 10.2
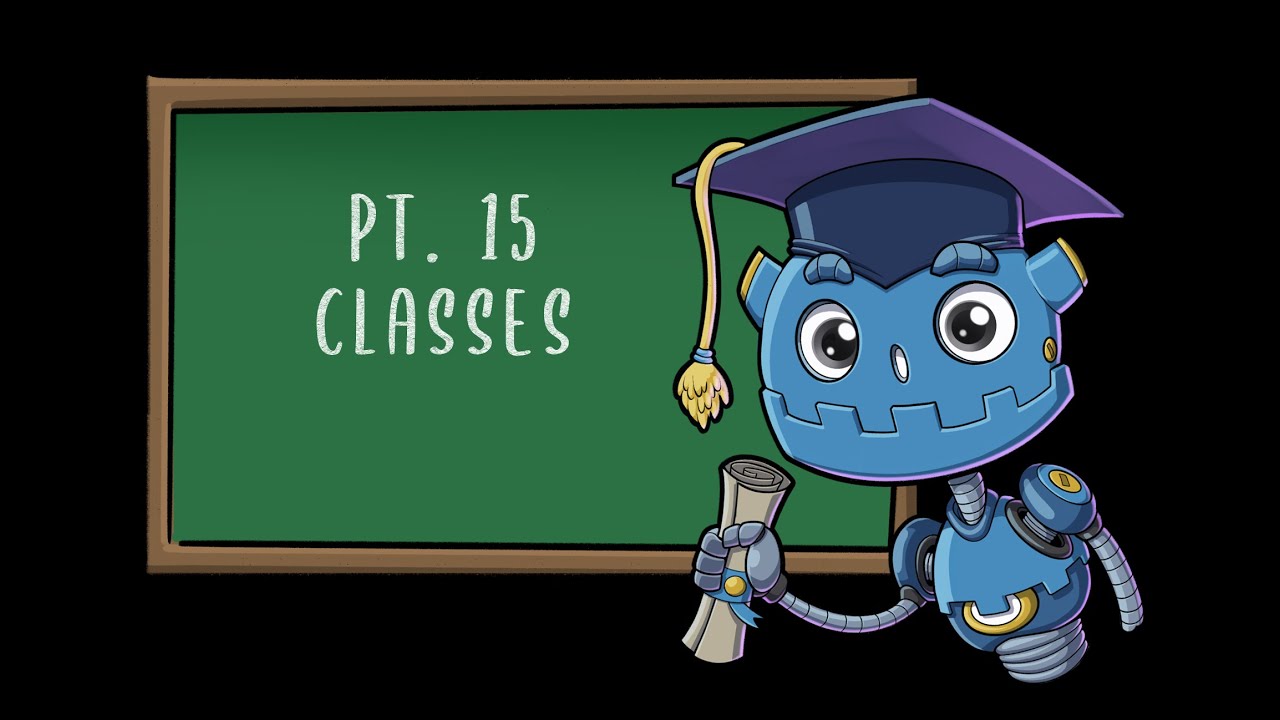
Classes | Godot GDScript Tutorial | Ep 15

Scope Levels | Godot GDScript Tutorial | Ep 14
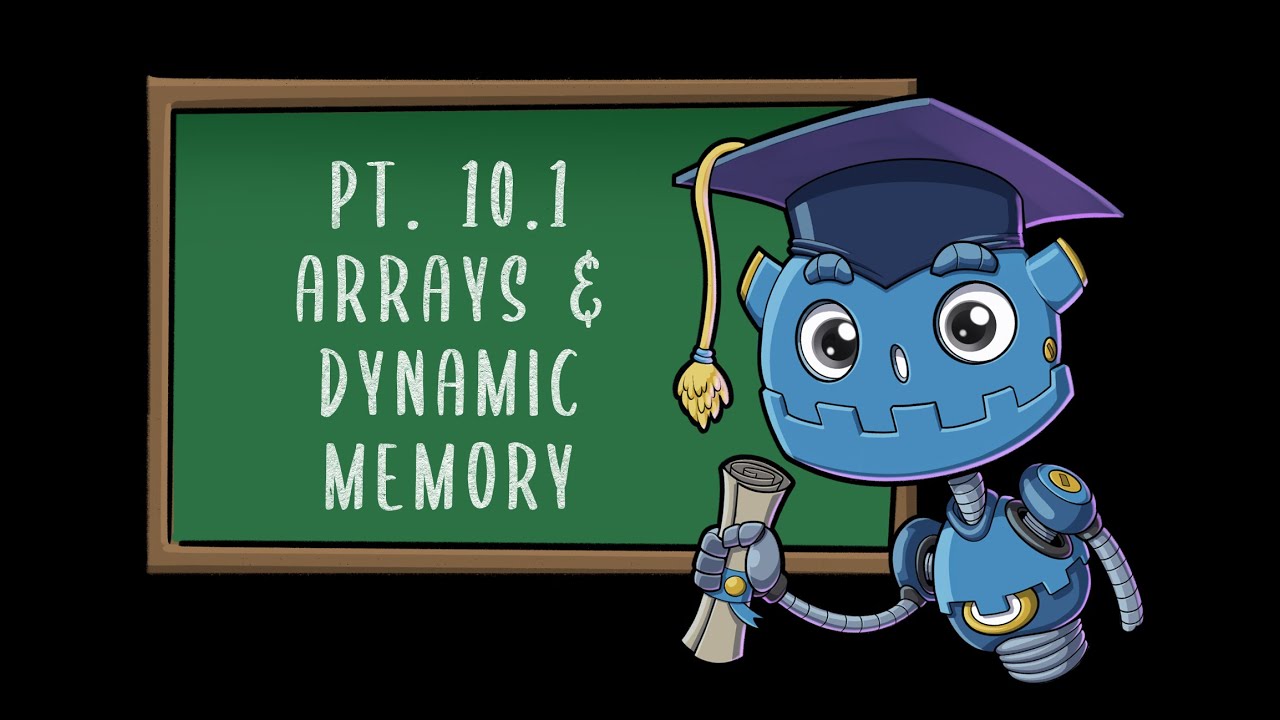
Arrays & Dynamic Memory Address | Godot GDScript Tutorial | Ep 10.1

Dictionaries | Godot GDScript Tutorial | Ep 12
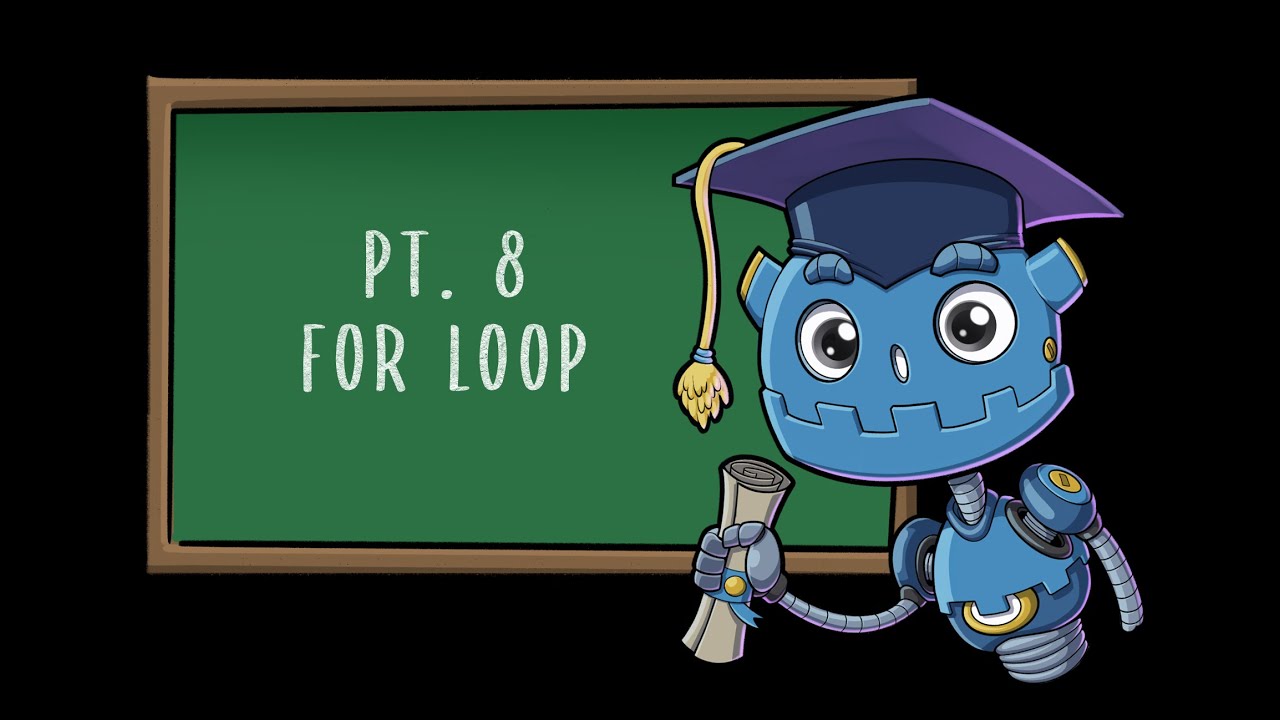
For Loops | Godot GDScript Tutorial | Ep 08
5.0 / 5 (0 votes)