For Loops | Godot GDScript Tutorial | Ep 08
Summary
TLDRThis episode of the G.D. script tutorial series introduces the for loop in Godot, an iterator-based control flow statement used for repeated code execution. It explains how to iterate through numbers, arrays, and dictionaries, with the range determining the iteration count. The script provides a basic for loop structure and flowchart, along with coding examples demonstrating how to print values from an integer range, an array, and a dictionary's keys and values. The tutorial simplifies understanding of for loops in Godot, making it accessible for both beginners and experienced developers.
Takeaways
- 🔁 A for loop is a control flow statement used for repeated code execution in programming.
- 🔢 Godot utilizes iterator-based for loops, which allow enumeration of various sets of items, including numbers, arrays, and dictionaries.
- 📝 The basic structure of a for loop in Godot includes the 'for' keyword, a placeholder variable, the 'in' keyword, and a range that dictates the iteration count.
- 🕒 When iterating with numbers, the loop variable starts at zero and increments until it reaches or exceeds the specified range.
- 📊 In the case of arrays, the loop variable represents each element in the array, running the block statement for each item.
- 🔑 When using dictionaries, the loop variable takes the form of keys from the dictionary.
- 🔄 The for loop flowchart illustrates the process of entering the loop, executing the block statement, updating the counter, and checking the loop condition until it becomes false.
- 📝 The script provides coding examples demonstrating how to use for loops with integers, arrays, and dictionaries in Godot.
- 🖨️ For integers, the script example prints numbers from 0 through 9 using a for loop.
- 🗃️ The array example iterates through elements, printing each one, starting from index position zero.
- 🔑 The dictionary example shows how to print keys and, optionally, the values associated with those keys using the standard dictionary brackets.
Q & A
What is a for loop in programming?
-A for loop is a control flow statement that allows code to be executed repeatedly. It is used to iterate through a range, such as numbers, arrays, or even dictionaries.
How does Godot's for loop differ from traditional for loops?
-Godot uses iterator-based for loops, which means they are designed to enumerate sets of items and can work with various data types, not just numbers.
What is the purpose of the 'in' keyword in a for loop?
-The 'in' keyword is used to specify the range or collection that the for loop will iterate over, such as an array or dictionary.
What is the role of the loop variable in a for loop?
-The loop variable holds the current element during the iteration. In the case of an array, it stores the current item, and in a dictionary, it stores the current key.
How does the range in a for loop determine the number of iterations?
-The range in a for loop dictates the number of times the code block inside the loop will execute. It can be an integer, an array, or a dictionary, and the loop runs until the end of the range is reached.
What happens when a for loop iterates over an array?
-When iterating over an array, the loop variable is assigned the value of each item in the array in sequence, and the code block is executed for each item.
Can a for loop iterate over a dictionary in Godot?
-Yes, a for loop can iterate over a dictionary. In this case, the loop variable will be assigned the keys of the dictionary.
How does the flowchart of a basic for loop work in Godot?
-The flowchart starts with entering the for loop, checking the condition (not reached the end of the range), executing the code block, updating the counter, and then rechecking the condition. This continues until the condition is false.
What is the starting value of the loop variable when iterating over a number range in Godot?
-When iterating over a number range, the loop variable starts at zero and increments until it is greater than or equal to the specified range number.
How can you access the value associated with a key in a dictionary during a for loop iteration?
-To access the value associated with a key in a dictionary during a for loop iteration, you use the standard dictionary brackets with the key to retrieve the corresponding value.
Outlines
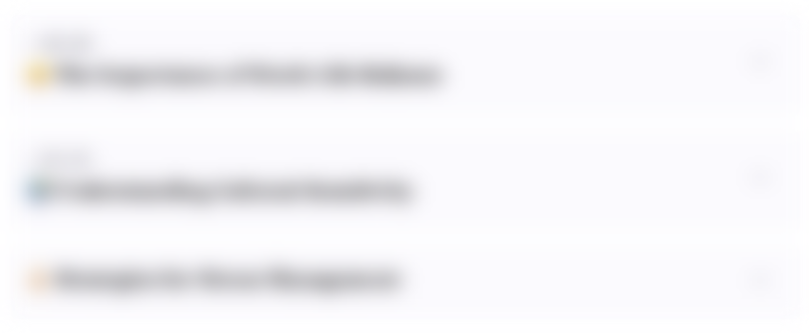
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
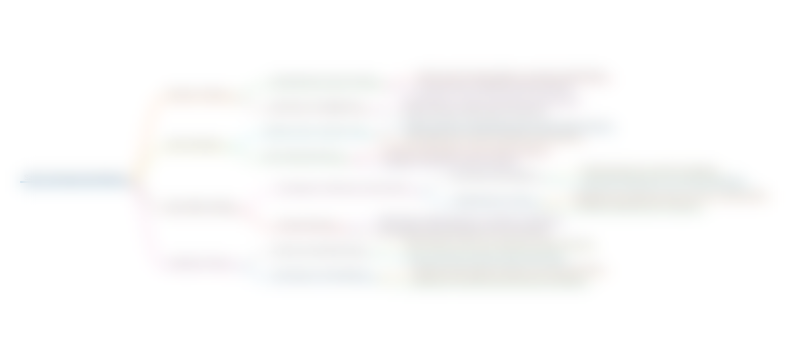
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
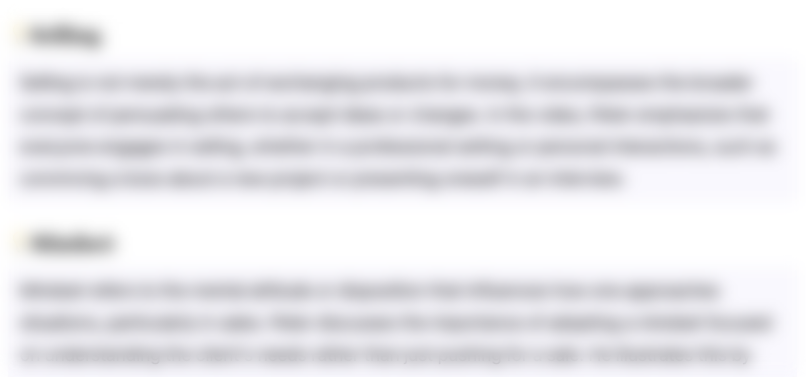
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
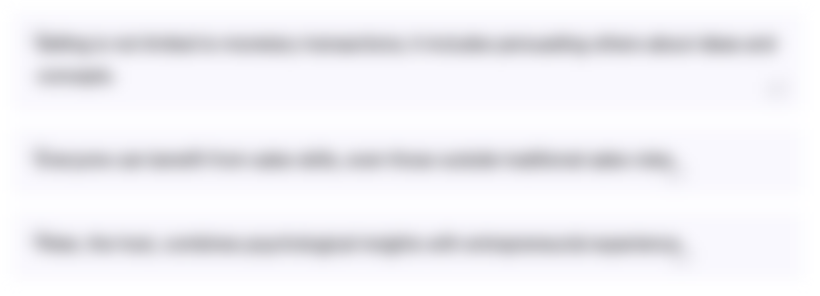
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
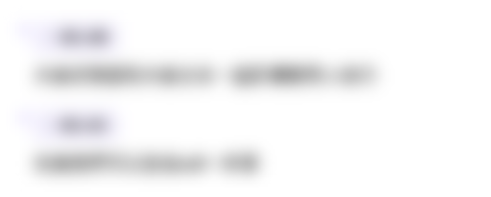
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
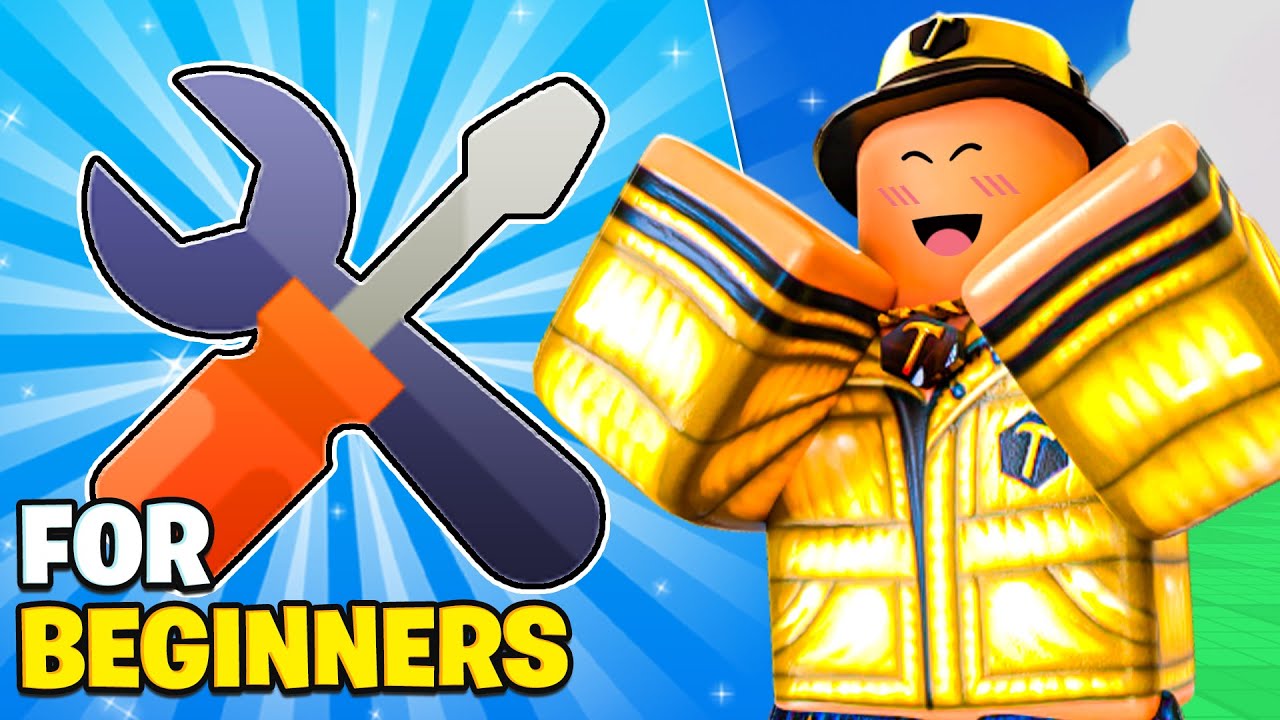
Beginner's Roblox Building Tutorial #3 - Part Tools & Groups

Przegłosowane! Będzie 37 Stref Czystego Transportu w Polsce
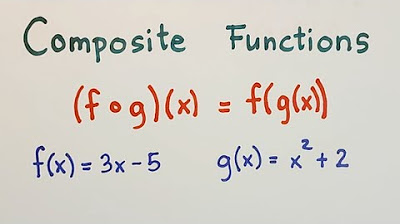
Composition of Functions - Grade 11 - General Mathematics

Grade 11 & 12 | Earth Science | Structure and Evolution of Ocean Basins | Theresa Reyes Q2 W6
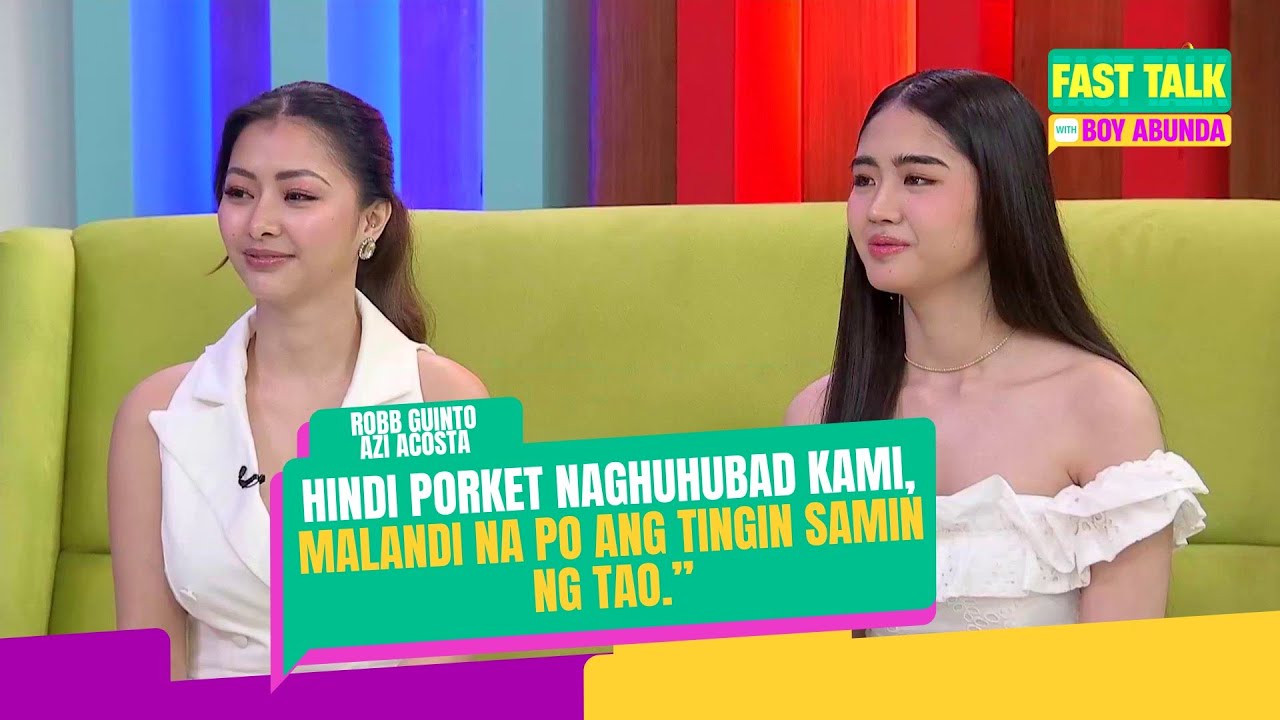
Fast Talk with Boy Abunda: Vivamax stars, BINASAG ang sexy star misconceptions! (Full Episode 438)
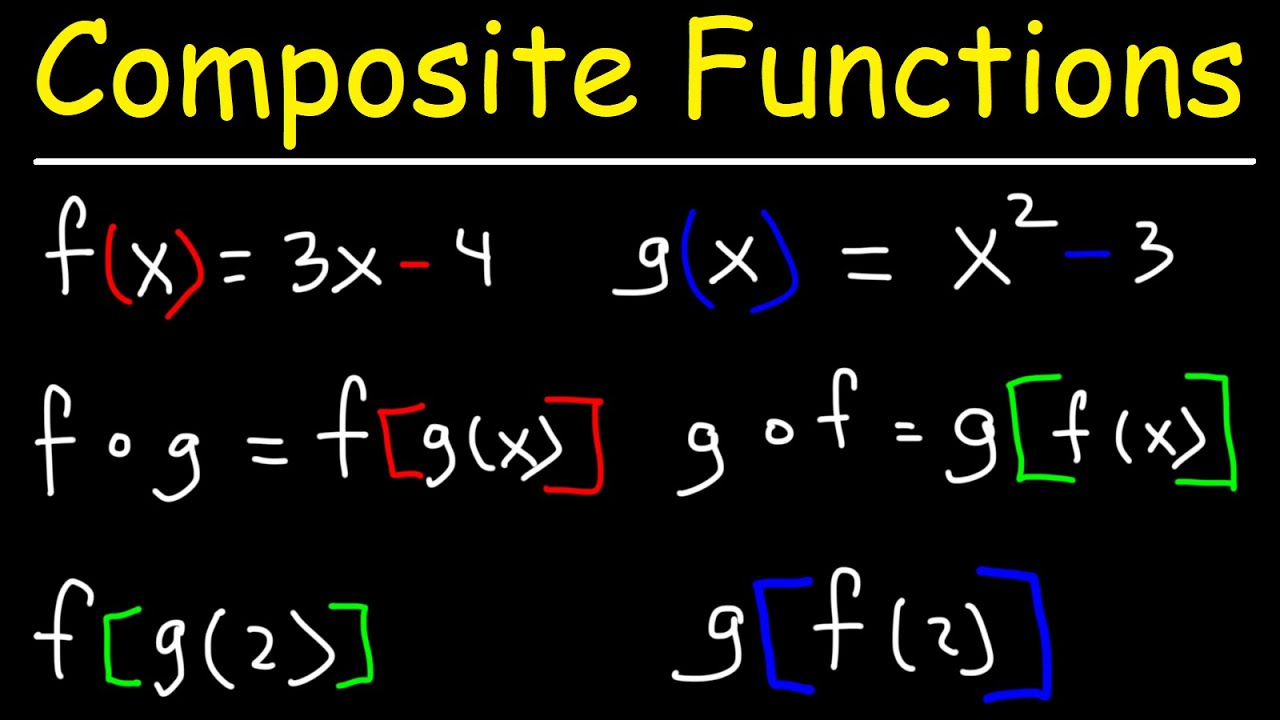
Composite Functions
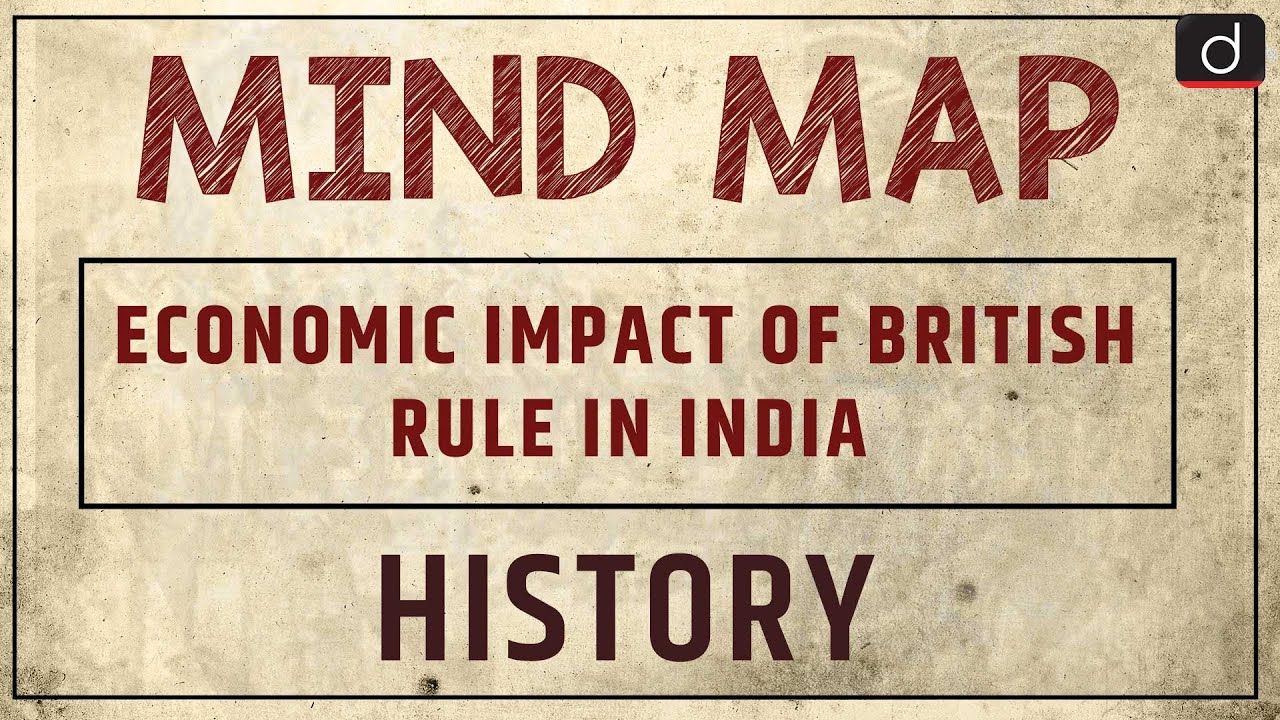
Economic Impact of British Rule in India - MIND MAP | Drishti IAS English
5.0 / 5 (0 votes)