Scope Levels | Godot GDScript Tutorial | Ep 14
Summary
TLDRThis episode of the GD Script Fundamental tutorial series delves into the concept of scopes in programming. Scopes are regions where named bindings, associating names with entities like variables, are valid. The tutorial explains four key scopes: global, class, function, and code block scopes, each with varying levels of accessibility. The presenter illustrates the scope hierarchy and how variables can propagate down but not up through these levels. Practical examples and code snippets clarify how variable accessibility works within each scope, emphasizing the intentional design to limit access for better program organization and functionality.
Takeaways
- π A scope is a region in a computer program where a name binding is valid, and it associates a name with an entity like a variable.
- π Scopes can range from small loops to an entire application, with varying levels of scope that are important for a programmer to understand.
- π Four fundamental levels of scopes are global, class, function, and code block scopes, each with specific accessibility rules.
- π Global scope variables, values, or functions are accessible throughout the entire program, such as the 'Node' class in GDScript.
- π Class scope variables, values, or functions are accessible only within the file they are declared, like the '.gd' file in GDScript.
- π Function scope variables are only visible within the function they are declared in, including parameters and local variable declarations.
- π Code block scope variables are only visible within the code blocks like if statements, while loops, and other control structures.
- β¬οΈ Scopes have a hierarchy, with global at the top and code block at the bottom, allowing variables to propagate down but not up the hierarchy.
- π« Variables declared in lower scopes are not accessible in higher scopes, enforcing encapsulation and preventing unintended access.
- π Understanding scope hierarchy helps in determining the accessibility of variables, functions, and classes within different parts of a program.
- π‘ Declaring variables higher in the scope hierarchy makes them more accessible, while those declared lower are intentionally less accessible to limit their use to specific contexts.
- π οΈ Practical examples in the script illustrate how to work with different scopes and the errors that occur when trying to access variables outside their declared scope.
Q & A
What is a scope in the context of programming?
-A scope is a region of a computer program where a named binding is valid. It refers to the visibility and accessibility of variables, functions, and classes within different parts of the code.
What are the four levels of scopes mentioned in the script?
-The four levels of scopes mentioned are global scope, class scope, function scope, and code block scope.
Can you explain the global scope and provide an example?
-The global scope refers to variables, values, or functions that can be used anywhere in the entire program. An example given in the script is the 'Node' class, which is globally accessible.
What is the class scope and how does it differ from the global scope?
-The class scope consists of variables, values, or functions that can only be accessed from within the file, such as a .GD file in GDScript. It differs from the global scope in that it is limited to the file's context, not the entire program.
What is a function scope and how does it relate to variable visibility?
-A function scope refers to variables, values, or parameters that are only visible inside the function where they are declared. This means they cannot be accessed outside of the function.
Can you describe the code block scope and its significance?
-The code block scope pertains to variables that are only visible inside code blocks, such as those declared in if statements, while loops, match statements, and for loops. It signifies the limited visibility of these variables to the specific block they are declared in.
How does the scope hierarchy work in programming?
-Scope hierarchy in programming defines the order and accessibility of different scopes. At the top is the global scope, followed by file module, class, function, and code block scopes at the bottom. Variables can propagate down the hierarchy but not up.
Why is it important to understand scope hierarchy when programming?
-Understanding scope hierarchy is crucial because it dictates where and how variables, functions, and classes can be accessed within a program. This helps in avoiding naming conflicts and ensures proper data encapsulation and security.
What happens if you declare a variable in a scope that is lower in the hierarchy, like a code block?
-Declaring a variable in a lower scope, such as a code block, means that this variable is not accessible to scopes above it. It is confined to the specific block it is declared in and cannot propagate up the scope hierarchy.
Can you provide an example of how a class member variable is used in GDScript?
-In the script, a class member variable is declared within a class. This variable can be accessed within the class's functions and even within loops, demonstrating its accessibility based on the class scope.
What error might occur if you try to access a function-scoped variable outside of its function in GDScript?
-Attempting to access a function-scoped variable outside of its function will result in an error. The GDScript compiler will throw an 'unknown variable name' error because the function scope does not have access to variables declared in scopes above it.
Outlines
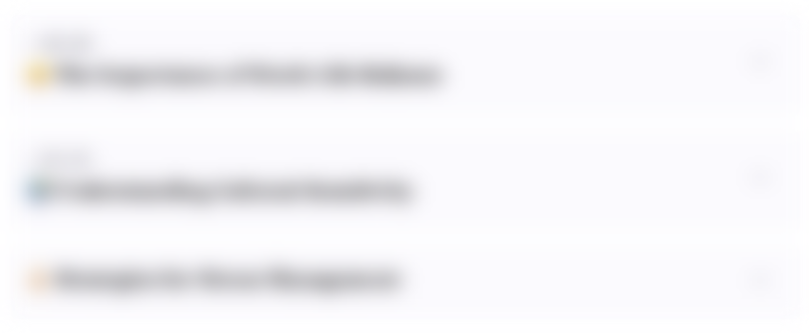
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
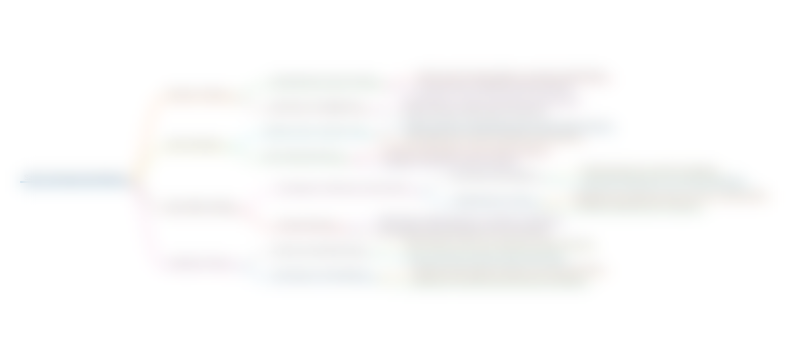
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
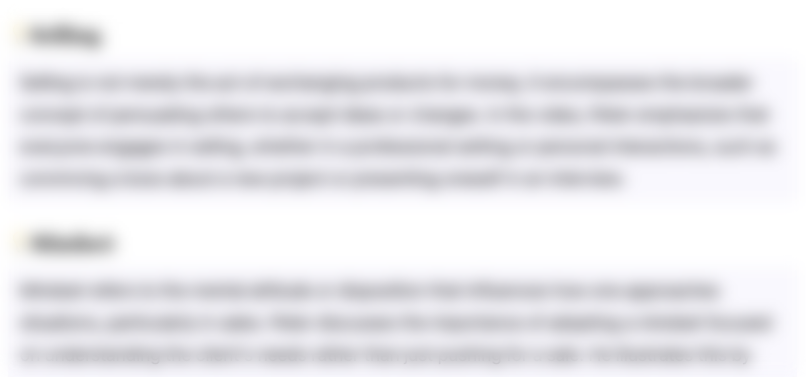
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
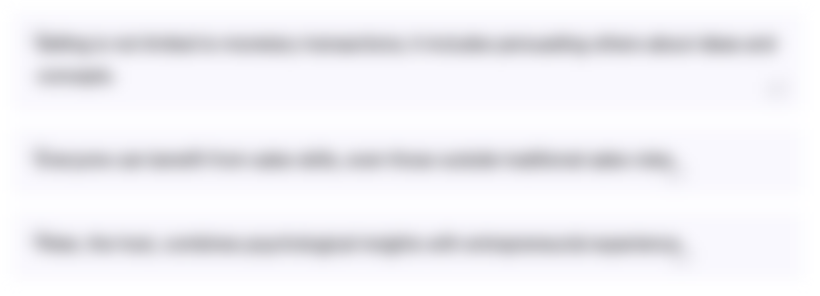
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
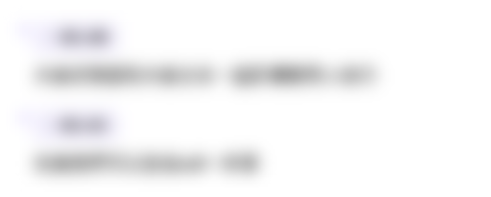
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)