DAY 30 | COMPUTER SCIENCE | II PUC | POINTERS | L1
Summary
TLDRThis video tutorial covers the essential concept of pointers in C programming, breaking down the declaration, initialization, and use of pointers. It explains the key operators—ampersand (&) for referencing addresses and asterisk (*) for dereferencing values. The video highlights how pointers help manage memory efficiently, interact with variables indirectly, and allow for dynamic memory allocation. Through practical examples, the tutorial demonstrates how to store variable addresses, dereference them, and access data stored at those memory locations. It aims to simplify a complex topic, offering valuable insights for budding C programmers.
Takeaways
- 😀 Pointers are variables that store the memory address of another variable, rather than directly storing data.
- 😀 The `&` (ampersand) operator is used to get the memory address of a variable.
- 😀 A pointer is declared with a data type followed by an asterisk (`*`), for example, `int *ptr`.
- 😀 The `*` (dereference) operator allows accessing the value stored at the memory address a pointer is pointing to.
- 😀 Initializing a pointer with `&variable` copies the address of the variable into the pointer.
- 😀 Using the `*` operator on a pointer gives you access to the value at the address the pointer holds.
- 😀 Pointers can be used to pass large data structures like arrays or structs to functions more efficiently, without copying the entire data.
- 😀 Pointers are critical for dynamic memory management, as they enable functions like `malloc()`, `calloc()`, and `free()` for runtime memory allocation and deallocation.
- 😀 Dereferencing a pointer with the `*` operator can help assign or access the value stored at the address, such as `*ptr = 25`.
- 😀 Pointers must be properly initialized and managed to avoid issues such as memory leaks, accessing invalid memory, or dereferencing null pointers.
Q & A
What is the main purpose of a pointer in C and C++?
-A pointer is a variable that stores the memory address of another variable. It allows direct access to memory, making programs more efficient and providing low-level memory control.
How do you declare a pointer in C or C++?
-To declare a pointer, you specify the data type of the variable it will point to, followed by an asterisk (*), and then the pointer name. For example: `int *ptr;` declares a pointer to an integer.
What is the function of the '&' (address-of) operator in the context of pointers?
-The '&' operator is used to obtain the memory address of a variable. When used with a variable, it returns the address where that variable is stored in memory, which can then be assigned to a pointer.
What does the '*' (dereference) operator do in pointer manipulation?
-The '*' operator is used to dereference a pointer. This means it accesses the value stored at the memory address the pointer is holding. It allows you to modify or read the value of the variable being pointed to.
In the example `int *ptr; ptr = #`, what is happening?
-In this example, `ptr` is a pointer variable of type `int`. The statement `ptr = #` assigns the memory address of the variable `num` to the pointer `ptr`. Now, `ptr` holds the address of `num`.
What will be the value of `c` in the code `int c = *ptr;` if `ptr` holds the address of `num`, where `num = 25`?
-The value of `c` will be 25 because the pointer `ptr` holds the address of `num`. Using the dereference operator `*ptr` accesses the value stored at that address, which is 25.
Why is it important to understand pointers when programming in C or C++?
-Understanding pointers is essential because they allow for efficient memory management, enable direct manipulation of memory, and facilitate advanced techniques such as dynamic memory allocation and interfacing with hardware.
What is the difference between the declaration of a normal variable and a pointer variable?
-A normal variable holds a value directly, whereas a pointer variable holds the memory address of another variable. For example, `int num = 10;` defines a normal variable, while `int *ptr;` defines a pointer variable that can hold an address of an integer.
What happens if you attempt to dereference a pointer that does not hold a valid address?
-Dereferencing a pointer that does not hold a valid address (e.g., a null pointer or an uninitialized pointer) results in undefined behavior, which can lead to crashes or unpredictable program behavior.
How can you safely initialize a pointer in C or C++?
-A pointer should be initialized to a valid memory address before dereferencing. It is often initialized to `nullptr` (in C++) or `NULL` (in C) to indicate that it does not point to any valid address until explicitly assigned to one.
Outlines
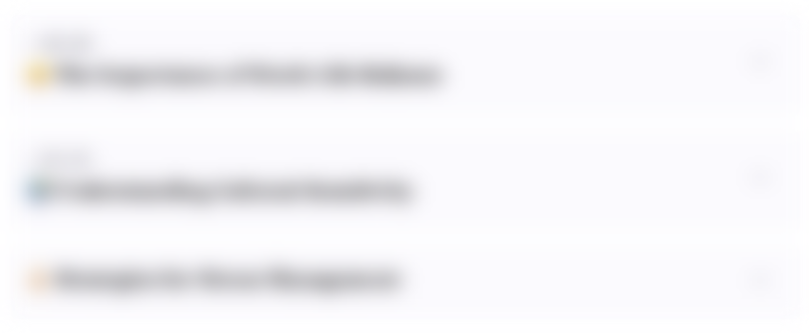
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
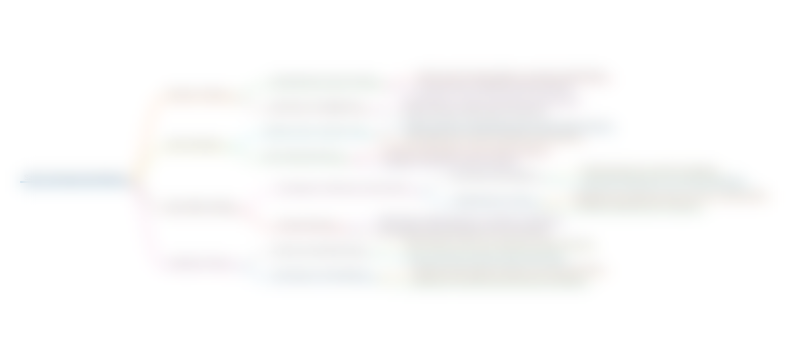
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
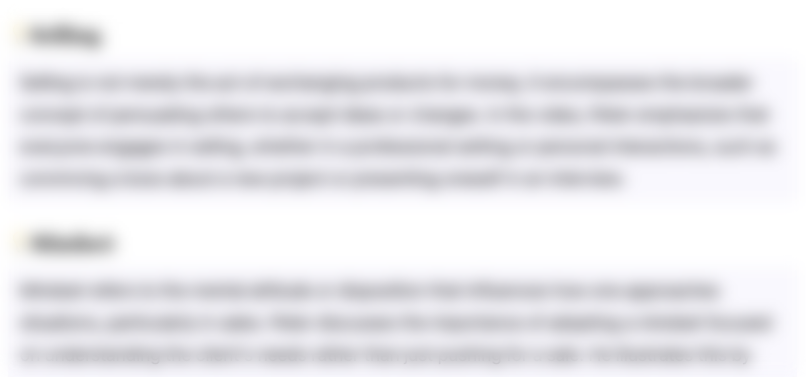
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
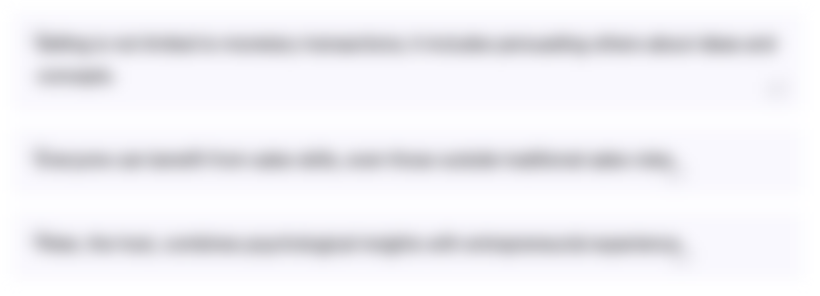
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
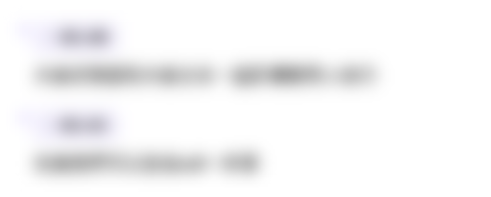
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)