The Weather App in Jetpack Compose using Kotlin | Part 1: Prerequisites | Android Knowledge
Summary
TLDRIn this tutorial series, the creator guides viewers through building a weather app using Jetpack Compose. They start by setting up prerequisites like adding dependencies for Retrofit and MVVM, defining colors, and obtaining an API key from OpenWeatherMap. The UI is kept simple, focusing on logic over design. The app will fetch and display weather data for a user-specified city, including temperature, humidity, and a description, using the OpenWeatherMap API. The series is divided into three parts: prerequisites, Retrofit setup, and UI design with Jetpack Compose.
Takeaways
- 🌐 The video is about creating a weather app using Jetpack Compose.
- 📱 The previous series focused on creating a contacts app with Room Database and MVVM.
- 📈 The new series will teach about Retrofit and API integration.
- 🎨 The UI is kept minimal, emphasizing logic over design.
- 🌡 The demo shows a weather app displaying city name, temperature, humidity, and description.
- 🔍 The app retrieves data from the OpenWeatherMap API.
- 🛠️ The tutorial is divided into three parts: prerequisites, Retrofit setup, and UI design with Jetpack Compose.
- 🧩 The first video covers the prerequisites for the project.
- 🎨 The second video will focus on setting up Retrofit.
- 📱 The third video will cover designing the UI using Jetpack Compose.
- 🔑 An API key from OpenWeatherMap is required for accessing the weather data.
Q & A
What is the main focus of the new series on the channel?
-The main focus of the new series is to create a weather app using Jetpack Compose.
What was the app created in the previous series?
-In the previous series, a contacts app was created where they learned about Room Database and MVVM.
What new concepts will be covered in the weather app series?
-The series will cover Retrofit and API usage within the context of Jetpack Compose.
What does the UI of the weather app look like?
-The UI is minimal, focusing on displaying the city name, temperature, humidity, and a description in cards.
How many attributes does the weather app display for each city?
-The weather app displays four attributes: city name, temperature, humidity, and a description.
Which API will be used to retrieve weather data?
-The Open Weather Map API will be used to retrieve weather data for the app.
What are the steps to get started with the weather app project?
-The steps include opening Android Studio, creating a new project, naming it 'The Weather App', and setting up the prerequisites.
What are the prerequisites for the weather app project?
-The prerequisites include adding Retrofit and MVVM dependencies, defining colors, adding a background image, requesting internet permissions in the Android manifest, and obtaining an API key from Open Weather Map.
Why is an API key necessary for the weather app?
-An API key is necessary for exclusive access to the Open Weather Map API to retrieve current weather data.
How long does it take for the API key to activate after registration?
-The API key might take up to an hour to activate after registration.
What is the structure of the video series for creating the weather app?
-The video series is divided into three parts: the first covers prerequisites, the second covers Retrofit, and the third covers designing the UI in Jetpack Compose.
Outlines
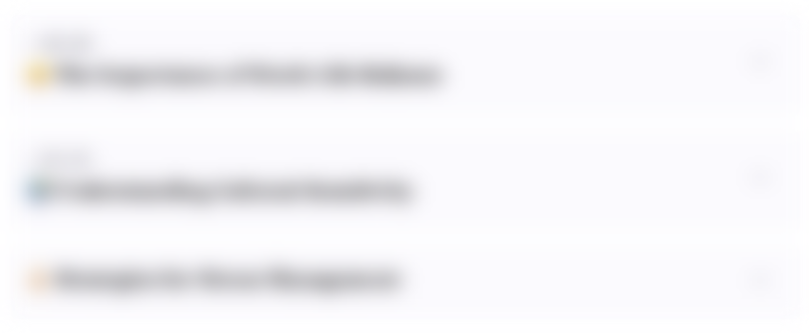
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
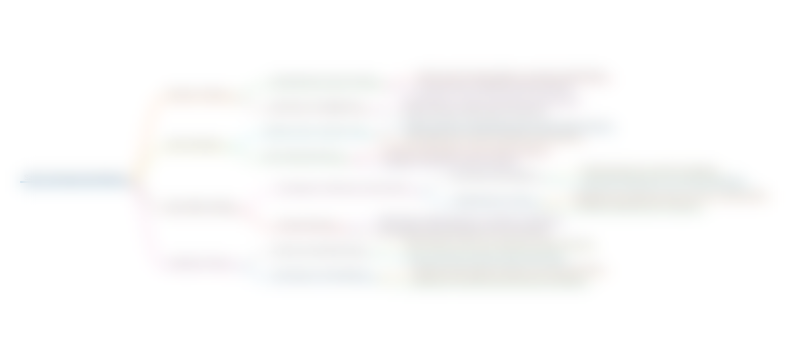
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
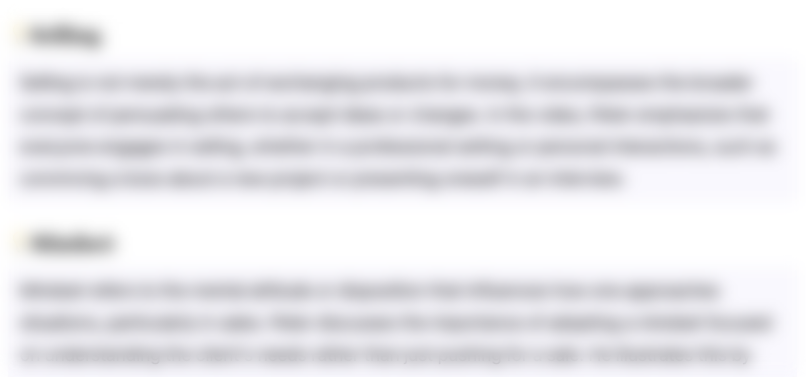
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
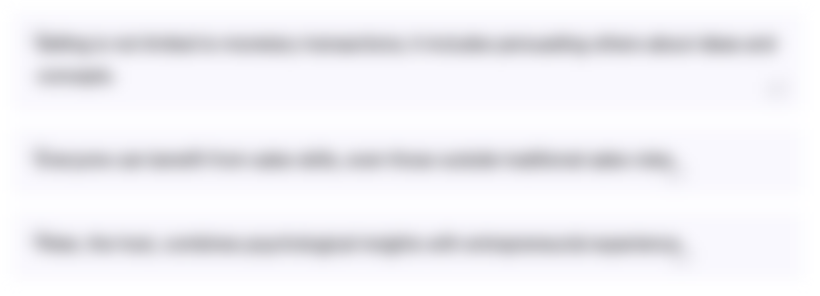
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
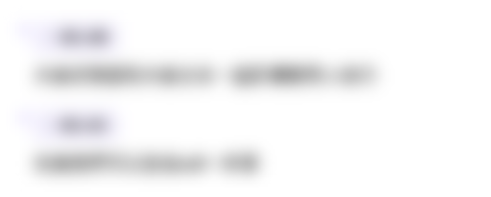
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Intuitive: Thinking in Compose - MAD Skills

How to Make a Simple and Very Easy Tic Tac Toe Game in Mit App Inventor
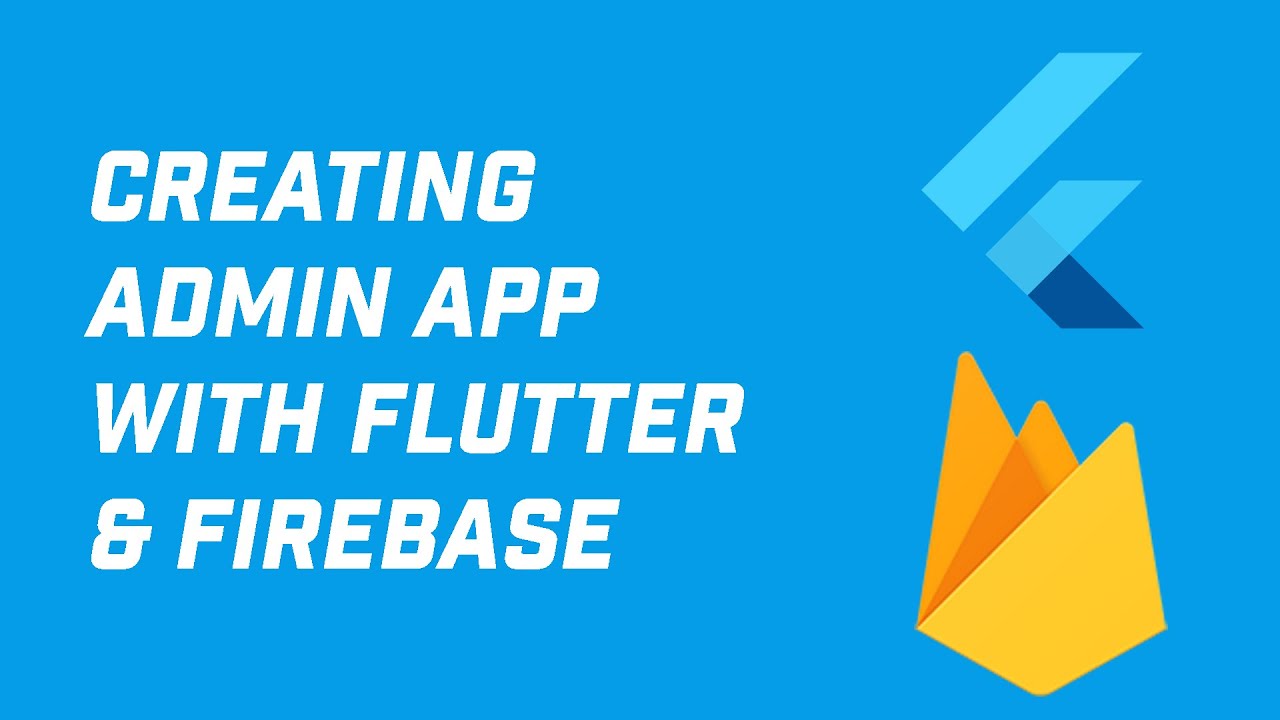
Creating Admin App in Flutter with Firebase Auth and Firestore (Your App Idea 1)

Build a modern android ecommerce app from scratch | Kotlin, Firebase, MVVM
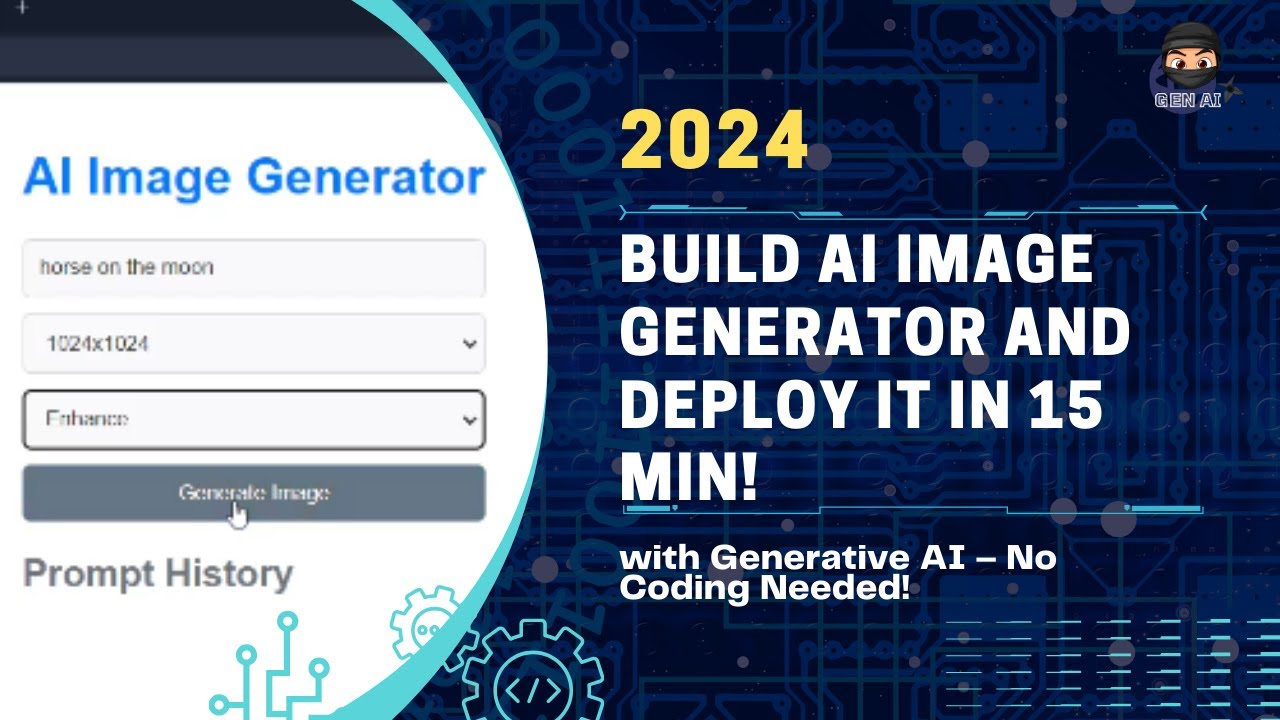
How to Build a No-Code Text-to-Image Mobile/Web App Using Replit Code Agent
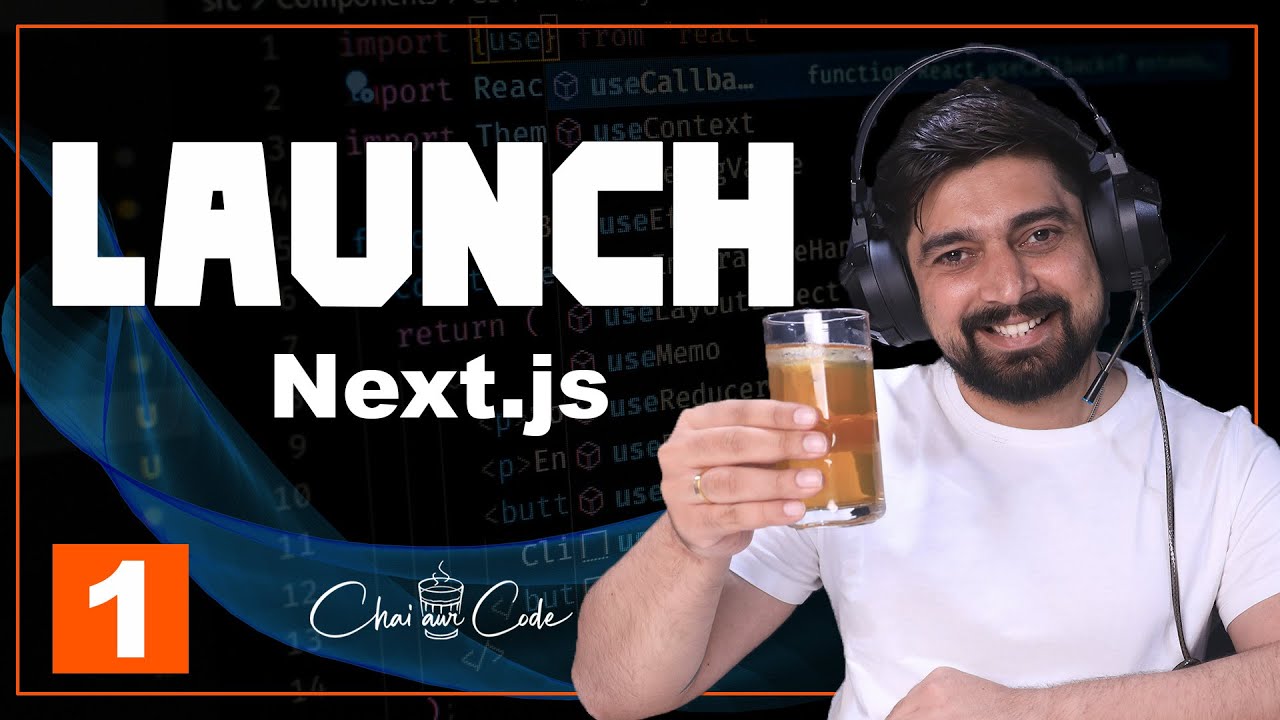
Complete full stack freelance ready course
5.0 / 5 (0 votes)