Python Tutorial for Beginners 8: Functions
Summary
TLDRThis educational video script teaches the basics of functions in Python. It explains how functions are defined with the 'def' keyword, can have parameters, and may return values. The script covers the benefits of reusing code with functions, the importance of keeping code DRY (Don't Repeat Yourself), and the concept of function arguments and default values. It also touches on unpacking arguments using * and **, and ends with an example tying together the concepts using Python's standard library.
Takeaways
- 📚 Functions in programming are blocks of code designed to perform a specific task.
- 🔑 The 'def' keyword in Python is used to define a function, which groups together instructions.
- 👤 Functions can have parameters, which are inputs that you can pass to the function when calling it.
- 🔍 The 'pass' keyword can be used as a placeholder within a function when you don't want it to perform any action yet.
- 💡 To execute a function in Python, you must include parentheses after the function name.
- 🔄 Functions help to avoid code repetition by allowing you to reuse the same block of code multiple times.
- 🔀 The concept of 'DRY' (Don't Repeat Yourself) is emphasized by using functions to keep code concise.
- 🔔 Functions can return values, which means they can output data after performing an operation.
- 🔀 Functions can be used to process data and then return the processed data to the caller.
- 📝 Docstrings are used to document what a function does and are placed right after the function definition.
Q & A
What is the purpose of using the 'def' keyword in Python?
-The 'def' keyword in Python is used to define a function, which is a block of organized, reusable code that is used to perform a single, related action.
How do you create a function in Python without any parameters?
-You create a function without any parameters by using the 'def' keyword followed by the function name and empty parentheses: `def functionName():`.
What does the 'pass' keyword do in a Python function?
-The 'pass' keyword in a Python function is a null operation that does nothing. It is used when a statement is required syntactically but no action is needed.
How do you execute a function in Python?
-You execute a function in Python by calling its name followed by parentheses: `functionName()`.
What is the benefit of using functions to reuse code?
-Using functions to reuse code allows you to make changes in one place instead of multiple places throughout your program, reducing the chance of errors and making your code more maintainable.
What does the acronym DRY stand for in programming?
-DRY stands for 'Don't Repeat Yourself', a principle in software development aimed at reducing repetition of software patterns.
How can you make a function return a value in Python?
-You can make a function return a value in Python by using the 'return' keyword followed by the value you want to return: `return someValue`.
What is the significance of the 'None' value when a function does not return anything?
-If a Python function does not explicitly return a value, it defaults to returning 'None', which is a built-in constant that represents the absence of a value or a null value.
How do you pass arguments to a function in Python?
-You pass arguments to a function in Python by specifying them within parentheses after the function name when calling the function: `functionName(arg1, arg2, ...)`.
What is the difference between positional and keyword arguments in Python functions?
-Positional arguments are passed to a function in the order they are defined, without needing to specify the parameter name. Keyword arguments are passed by naming the parameter followed by the value.
How can you accept a variable number of arguments in a Python function?
-You can accept a variable number of positional arguments using *args and a variable number of keyword arguments using **kwargs in the function definition.
What is the purpose of using * and ** in function calls in Python?
-In function calls, * is used to unpack iterables into positional arguments, and ** is used to unpack dictionaries into keyword arguments.
Outlines
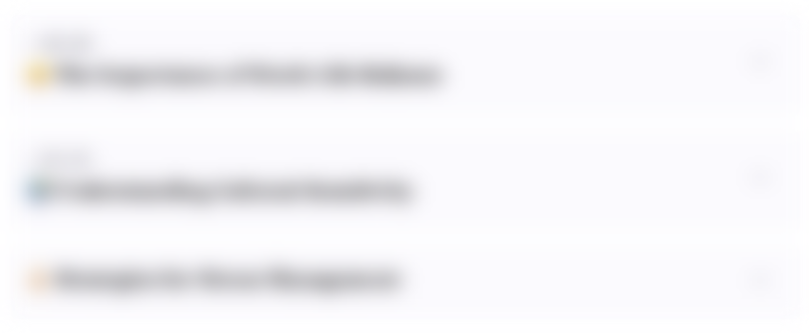
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
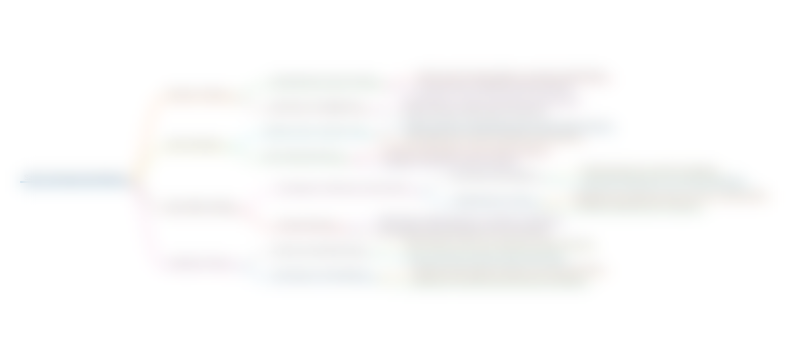
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
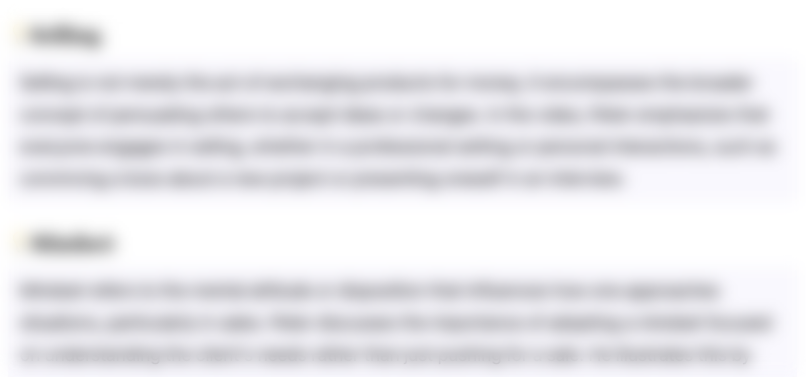
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
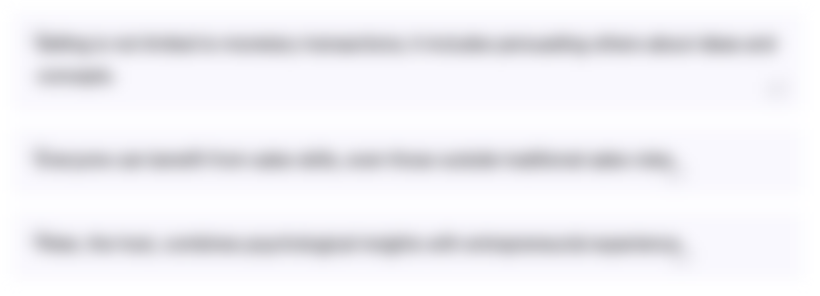
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
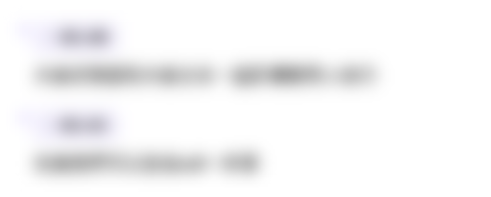
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)