Go (Golang) Tutorial #9 - Using Functions
Summary
TLDRThis script introduces the concept of functions in Go, a popular programming language. It explains the main function and how to create custom functions, including those with parameters and return values. The video demonstrates how to reuse code effectively by defining and calling functions like 'greeting' and 'bye', as well as more complex examples involving slices and functions as arguments. The script also covers formatting output for better readability, showcasing the versatility and utility of functions in Go for various programming tasks.
Takeaways
- π Functions in Go are a way to organize and reuse code, improving efficiency and maintainability.
- π The main function in Go executes automatically when the program runs, serving as the entry point for the application.
- π Functions can be defined outside of the main function, allowing them to be used in the main function and potentially in other files.
- π― Functions can take parameters, which are specified in the function declaration, ensuring type safety and functionality.
- π Functions can perform actions such as printing to the console, as demonstrated with the greeting and bye functions.
- π Functions can be called multiple times with different arguments, showcasing the reusability of functions in Go.
- π Functions can accept multiple arguments, enabling complex operations with various inputs.
- π Functions can also take other functions as arguments, allowing for dynamic and flexible behavior.
- π The concept of 'range' and slices is used to iterate over collections, which can be utilized inside functions.
- π Returning values from functions is a key feature, allowing the results of computations to be used elsewhere in the code.
- π Functions can return values of any type, and the return type must be specified in the function signature.
- π¨ Formatting output, such as limiting decimal places, can be achieved using formatted strings (e.g., printf).
Q & A
What is the main purpose of functions in Go programming language?
-The main purpose of functions in Go is to organize and reuse code effectively. Functions allow developers to create reusable blocks of code that can be called upon as needed, promoting modularity and code maintainability.
How does the main function in Go behave?
-The main function in Go is the entry point of the program. It is the first function that gets executed when the program runs, and it does not require an explicit call to start. It is marked with the 'func' keyword followed by 'main' and a parameter list that includes 'int' and 'string'.
How can you define a function in Go outside of the main function?
-You can define a function outside of the main function by using the 'func' keyword, followed by the function name, parameter list (if any), and a block of code enclosed in curly braces. The function can then be called from within the main function or other parts of the code.
What is the purpose of parameters in a function?
-Parameters in a function serve as inputs that allow the function to accept data from the caller. They enable the function to be flexible and dynamic, as it can operate on different data each time it is called, enhancing its reusability.
How do you pass a variable to a function in Go?
-In Go, you pass a variable to a function by specifying the variable's name within the argument list when calling the function. The variable's value is then passed to the function, allowing it to be used within the function's body.
What is the significance of the 'fmt.Println' and 'printf' in the context of the script?
-'fmt.Println' and 'printf' are standard Go packages used for printing output to the console. 'fmt.Println' prints the given arguments and a newline character, while 'printf' allows for formatted string output, which can include variables as part of the output using the '%v' verb.
How can you reuse code with functions in Go?
-You can reuse code in Go by defining functions that perform specific tasks and then calling these functions whenever those tasks need to be executed. This practice reduces redundancy and makes the code more efficient and easier to maintain.
What is a slice in Go and how is it used in the context of the script?
-A slice in Go is a dynamic array that holds a reference to an array and provides a way to access elements of the array. In the script, a slice of strings is used as an argument in a function to demonstrate how multiple elements can be iterated over and processed by a function.
How do you pass a function as an argument to another function in Go?
-In Go, you can pass a function as an argument to another function by specifying the type 'func' in the parameter list of the receiving function. The function being passed must match the expected signature (i.e., the number and types of parameters) of the function argument in the receiving function.
What is the purpose of the 'range' keyword in a for loop when iterating over a slice?
-The 'range' keyword in a for loop is used to iterate over the elements of a slice without needing the index. It automatically assigns the current element to a variable (denoted by the underscore '_' if the index is not needed) and loops through the entire slice.
How do you return a value from a function in Go?
-In Go, you return a value from a function using the 'return' keyword followed by the value you wish to return. The function's signature must specify the return type, and the type of the value being returned must match the declared return type.
How can you format the output of a float in Go?
-In Go, you can format the output of a float using the 'fmt.Printf' function with a formatted string that includes the '%f' verb followed by a precision specifier (e.g., '%.2f' for two decimal places). This allows you to control the appearance of the floating-point number when it is printed to the console.
Outlines
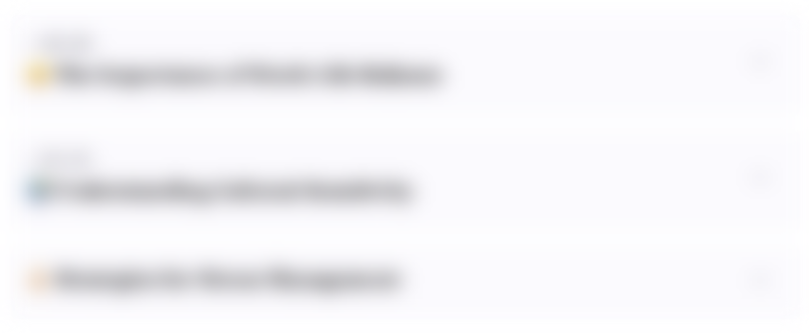
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
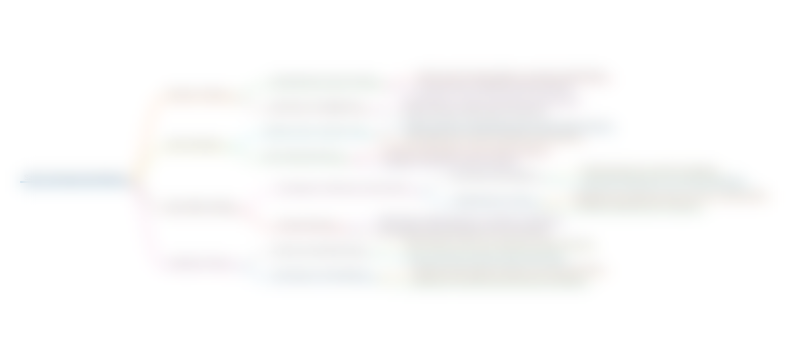
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
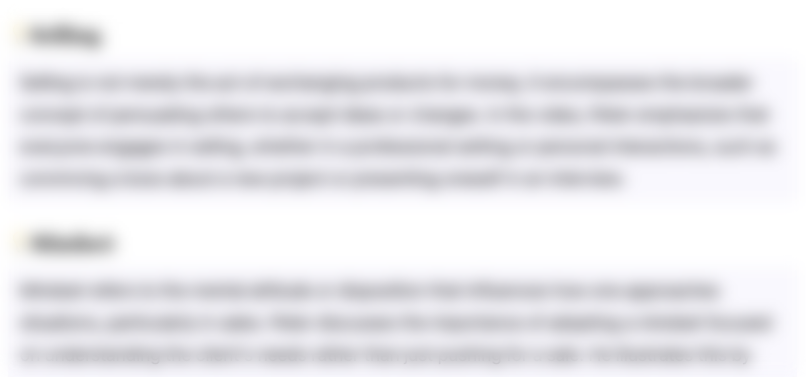
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
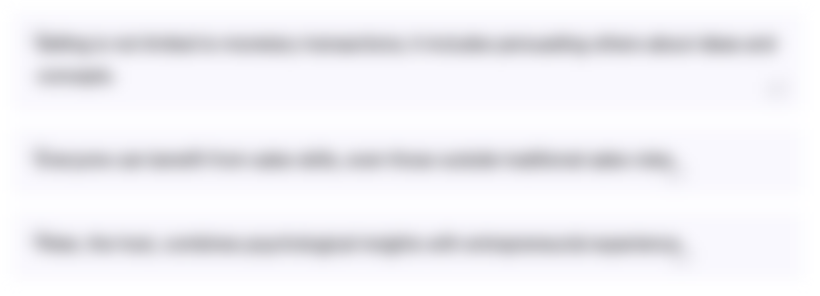
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
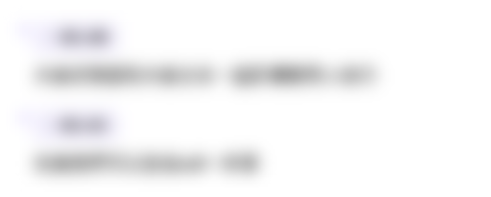
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)