Numpy Operations - Data Analysis with Python Course
Summary
TLDRThis script delves into the concept of vectorized operations and broadcasting in NumPy, emphasizing their efficiency and importance in array manipulation. It explains how operations between arrays and scalars are executed at an array level, with each element being processed simultaneously, resulting in a new array. The script also touches on the immutability of NumPy arrays and the use of list comprehensions as a comparison to vectorized operations, highlighting the speed and optimization of NumPy's approach.
Takeaways
- 📚 Broadcasting is a fundamental concept in NumPy that allows for efficient array operations.
- 🔢 Vectorized operations in NumPy are performed between arrays and arrays, or arrays and scalars, and are highly optimized for speed.
- 💡 NumPy's operations are immutable by default; performing an operation on an array returns a new array rather than modifying the original.
- 🔄 The concept of vectorization involves applying an operation to each element of an array, which is done internally through broadcasting.
- 📈 When performing operations between arrays, they must be aligned and have the same shape for broadcasting to work correctly.
- 🛠️ NumPy provides an interface for in-place modifications using operations like '+=', '-=', '*=', etc., which can alter arrays directly.
- 📝 The result of vectorized operations is a new array, emphasizing the importance of understanding NumPy's immutable nature.
- 🔑 Understanding broadcasting is crucial for leveraging NumPy's full potential, especially when dealing with large datasets.
- 📚 The script compares vectorized operations in NumPy to list comprehensions in pure Python, highlighting the performance benefits of NumPy.
- 🔍 The importance of vectorized operations is underscored by their frequent use in advanced NumPy functionalities and applications.
- 📘 The script encourages revisiting exercises to solidify understanding of vectorized operations and broadcasting, indicating their significance in NumPy.
Q & A
What is the main topic discussed in the script?
-The main topic discussed in the script is vectorized operations and broadcasting in NumPy, which are fundamental concepts related to Boolean arrays and their importance in efficient array computations.
Why are vectorized operations important in NumPy?
-Vectorized operations are important in NumPy because they allow for fast and efficient array computations, as they are optimized for performance and can be applied to both arrays and scalars.
What is the difference between vectorized operations and list comprehensions in Python?
-While both vectorized operations in NumPy and list comprehensions in Python allow for element-wise operations, the key difference is that NumPy's vectorized operations are highly optimized and much faster, making them suitable for large-scale numerical computations.
How does broadcasting work in NumPy?
-Broadcasting in NumPy allows for arithmetic operations between arrays of different shapes. It works by replicating the elements of the smaller array to match the shape of the larger array, enabling element-wise operations without the need for explicit loops.
What is the result of adding a scalar to an entire NumPy array?
-The result of adding a scalar to an entire NumPy array is a new array where each element of the original array has the scalar value added to it, without modifying the original array due to NumPy's immutable nature.
Why is it said that NumPy is an immutable library?
-NumPy is considered immutable because performing an operation on an array does not modify the original array. Instead, it returns a new array with the result of the operation.
What are the conditions required for broadcasting to work between two arrays?
-For broadcasting to work between two arrays, they must be aligned in shape, meaning they can either be the same shape or one of them can be a scalar, or one dimension can differ where the array has a size of one.
Can you provide an example of a vectorized operation in NumPy?
-An example of a vectorized operation in NumPy is adding a scalar to an array, such as 'a + 10', where 'a' is an array and '10' is the scalar. This operation is applied to each element of the array, resulting in a new array.
What is the significance of the term 'immutable' in the context of NumPy arrays?
-The term 'immutable' in the context of NumPy arrays signifies that the arrays cannot be altered after they are created. Any operation performed on an array results in the creation of a new array, leaving the original array unchanged.
How can one override the immutable behavior of NumPy arrays?
-The immutable behavior of NumPy arrays can be overridden by using in-place operators such as '+=', '-=', '*=', etc., which modify the array directly instead of creating a new one.
What is the relationship between vectorized operations and memory usage in NumPy?
-Vectorized operations in NumPy are designed to be memory efficient. They perform operations in a way that is aligned with the memory layout of arrays, which minimizes the need for temporary storage and copying of data, thus optimizing memory usage.
Outlines
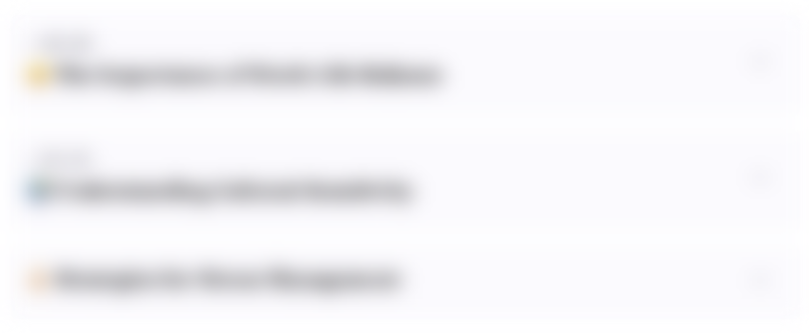
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
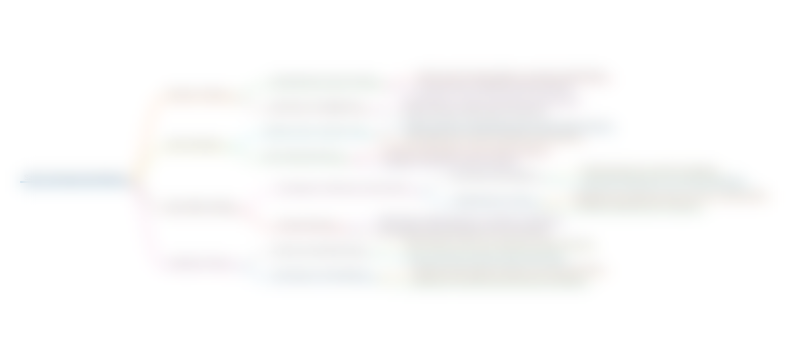
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
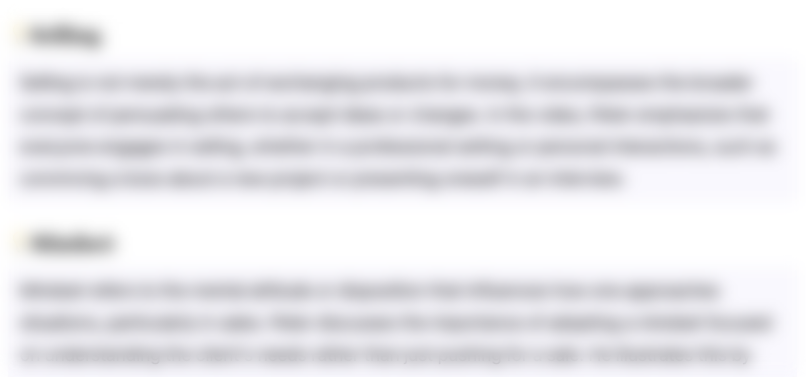
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
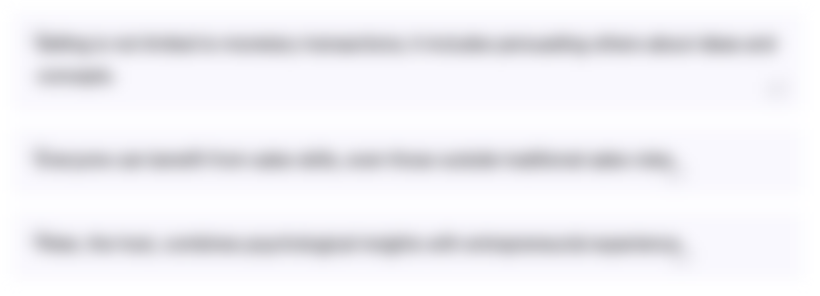
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
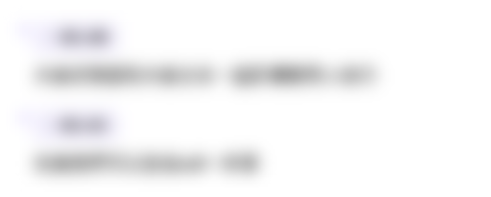
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)