Numpy - Part 01
Summary
TLDRThis lecture introduces the NumPy library in Python, emphasizing its role in data manipulation and linear algebra. It covers creating and reshaping NumPy arrays, accessing elements through slicing, and iterating through arrays. The lecture highlights the efficiency of NumPy arrays compared to lists for mathematical operations, making it an essential tool for data science.
Takeaways
- 😀 Numpy is a fundamental library in Python for data manipulation, especially useful for working with arrays and matrices.
- 🔢 Numpy arrays are n-dimensional, meaning they can have any number of dimensions, and they must contain items of the same type.
- 📈 The `reshape` function in numpy allows for the reshaping of arrays into different dimensions, which is not possible with standard Python lists.
- 🚀 Numpy arrays can be created using a variety of methods, including `np.arange`, `np.tile`, `np.zeros`, and `np.ones`.
- 💾 Numpy arrays are indexed starting at zero, following the Python convention, and support slicing and advanced indexing.
- 🔄 The slicing in numpy arrays is more powerful than lists, allowing for steps in slicing and the selection of entire rows or columns.
- 🔀 Iterating through numpy arrays can be done with loops, and `enumerate` can be used to get both the index and value during iteration.
- 🔁 The `np.arange` function in numpy is similar to the `range` function in Python, but returns a numpy array instead of a range object.
- 📚 Numpy arrays are more efficient for mathematical operations than lists, which is why they are widely used in scientific computing.
- 📝 The script emphasizes the importance of hands-on practice with numpy to fully understand its capabilities and advantages over native Python lists.
Q & A
What is NumPy?
-NumPy is a library in Python used for scientific computing. It allows for the creation and manipulation of arrays and matrices, which are essential for various mathematical and scientific operations.
Why is NumPy preferred over lists for mathematical operations?
-NumPy is preferred because it is designed for numerical operations and supports vectorization, which makes it faster and more efficient than lists for mathematical computations.
What is the significance of NumPy arrays being n-dimensional?
-NumPy arrays can be n-dimensional, meaning they can represent data in multiple dimensions (like vectors, matrices, or higher-dimensional arrays), which is useful for complex mathematical and scientific computations.
How do you import NumPy in Python?
-You can import NumPy in Python using the 'import' statement, often with a nickname 'np' for convenience, like so: 'import numpy as np'.
What is the difference between a list and a NumPy array?
-A NumPy array is a collection of elements of the same type, unlike a Python list which can contain elements of different types. NumPy arrays are also more memory efficient and support a large number of mathematical operations.
How can you create a NumPy array?
-You can create a NumPy array using the 'np.array()' function, providing a list or any iterable as an argument. Other functions like 'np.arange()', 'np.zeros()', and 'np.ones()' can also be used to create arrays with specific patterns.
What is the purpose of the 'reshape' method in NumPy?
-The 'reshape' method in NumPy is used to change the shape of an array without altering its data. This is useful for organizing data into a desired format for specific operations.
How does slicing work with NumPy arrays?
-Slicing in NumPy arrays works similarly to lists but with additional capabilities. You can use slicing to access rows, columns, or specific elements using indices. This includes using steps and negative indices to reverse through the array.
Can you iterate through a NumPy array?
-Yes, you can iterate through a NumPy array using loops. You can loop through each element, or use 'enumerate' to get both the index and the element during iteration.
What are some common operations you can perform with NumPy arrays?
-Common operations include mathematical computations like addition, subtraction, multiplication, and division, as well as more complex operations like matrix multiplication, statistical analysis, and random number generation.
Outlines
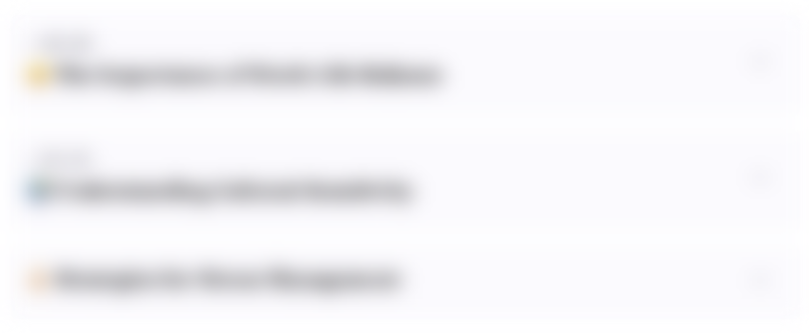
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
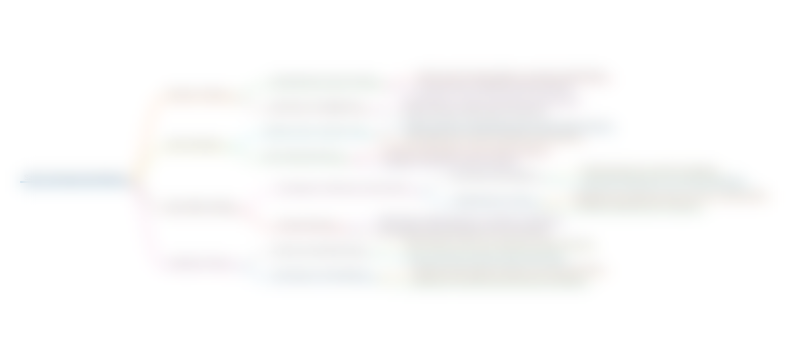
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
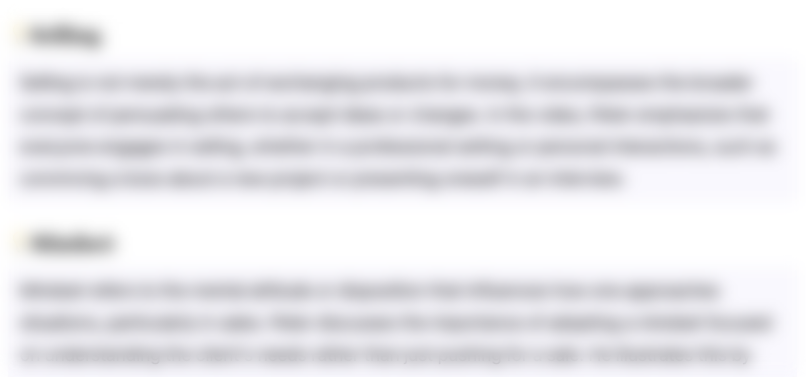
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
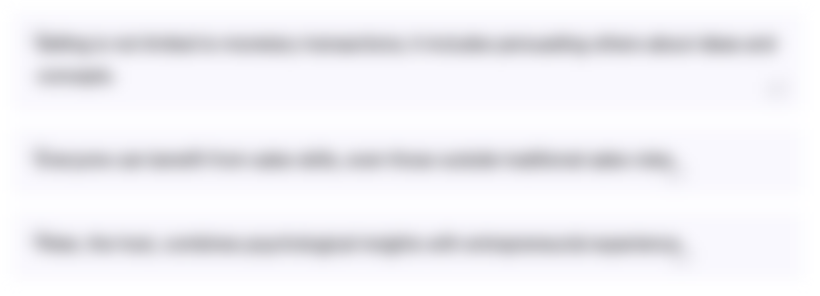
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
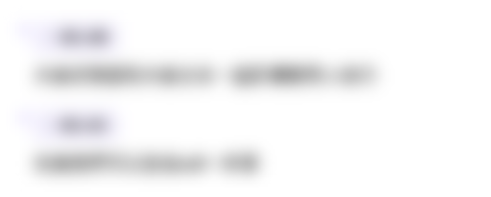
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
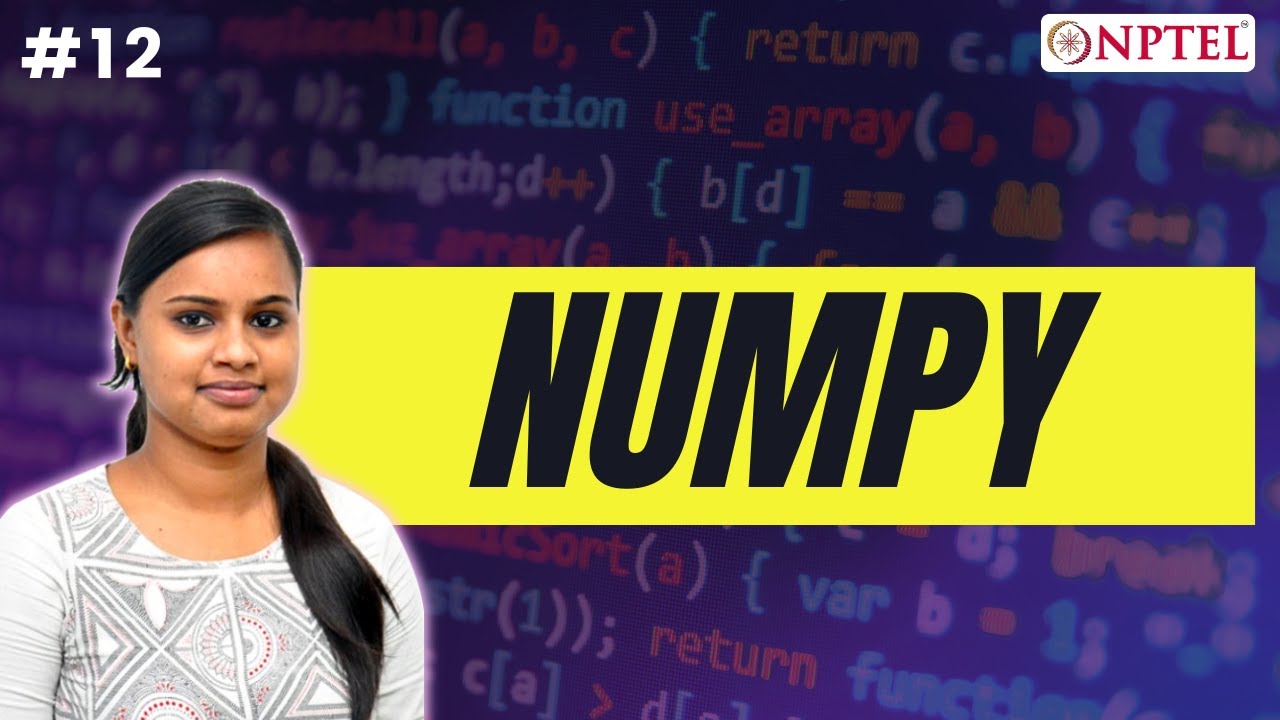
Numpy
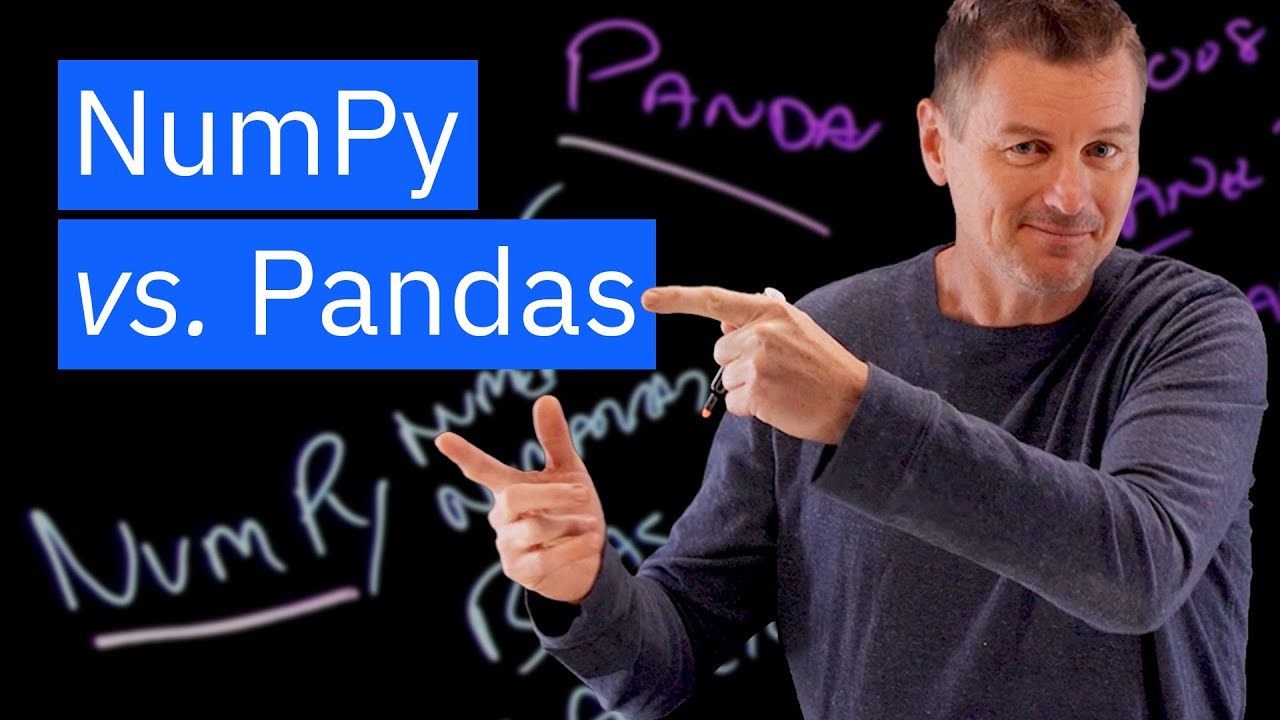
NumPy vs Pandas
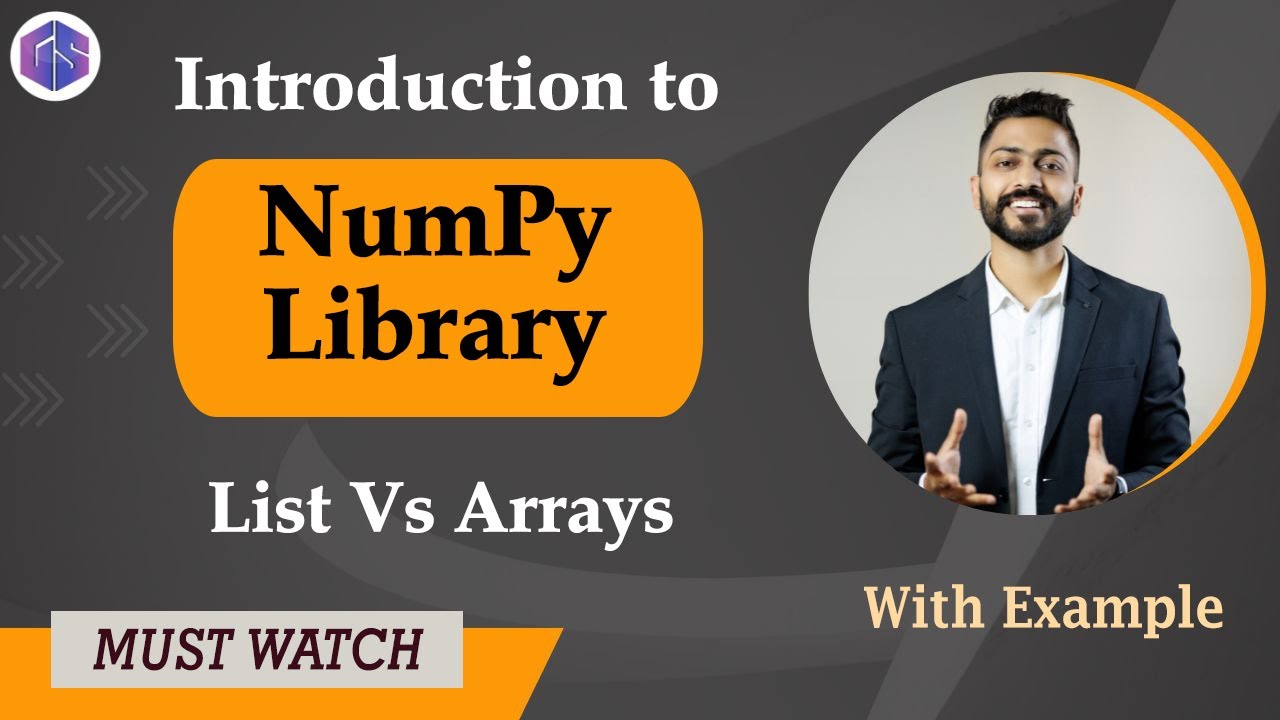
Lec-31: Introduction to NumPy Library in Python 🐍 List vs Arrays in Python 🐍 with examples
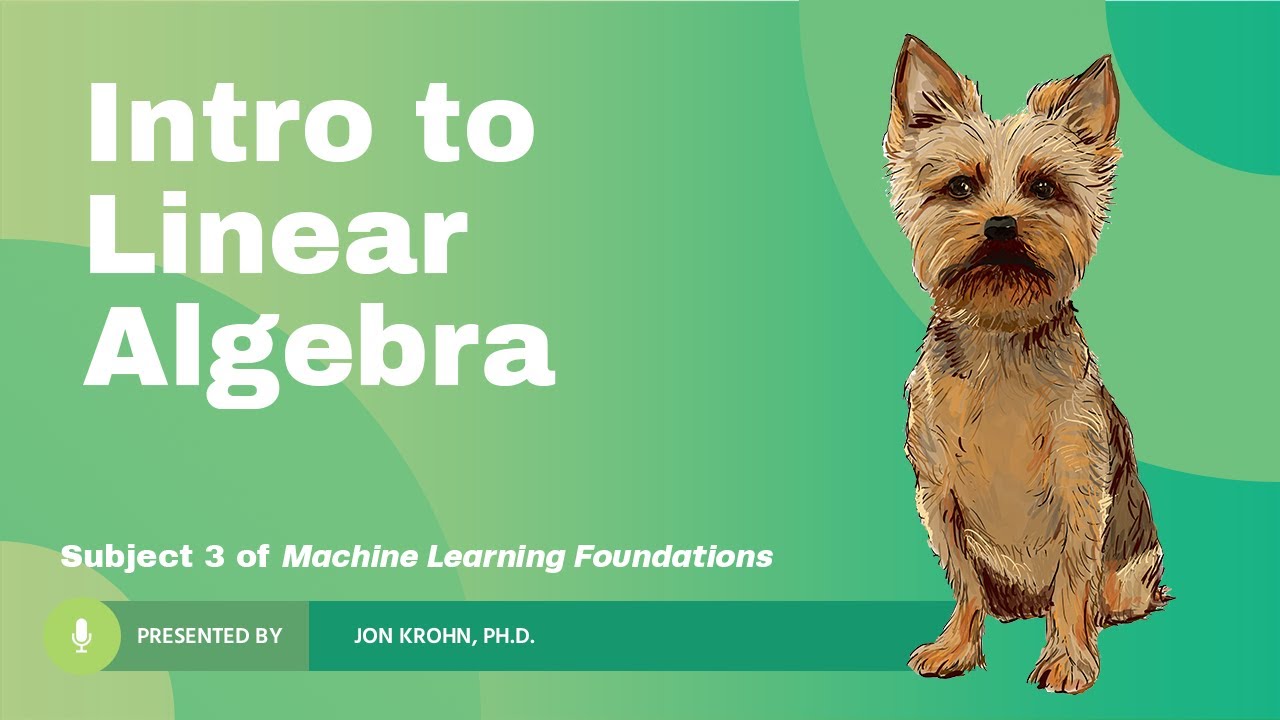
What Linear Algebra Is — Topic 1 of Machine Learning Foundations
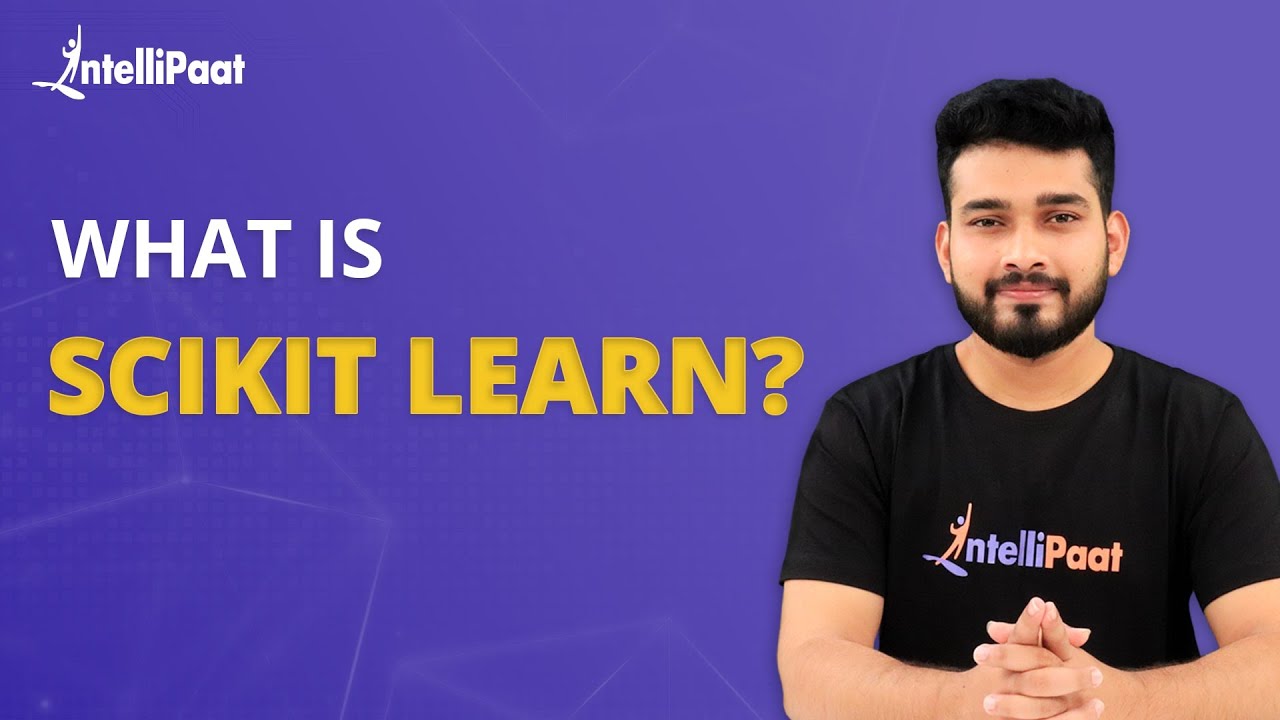
What Is Scikit-Learn | Introduction To Scikit-Learn | Machine Learning Tutorial | Intellipaat
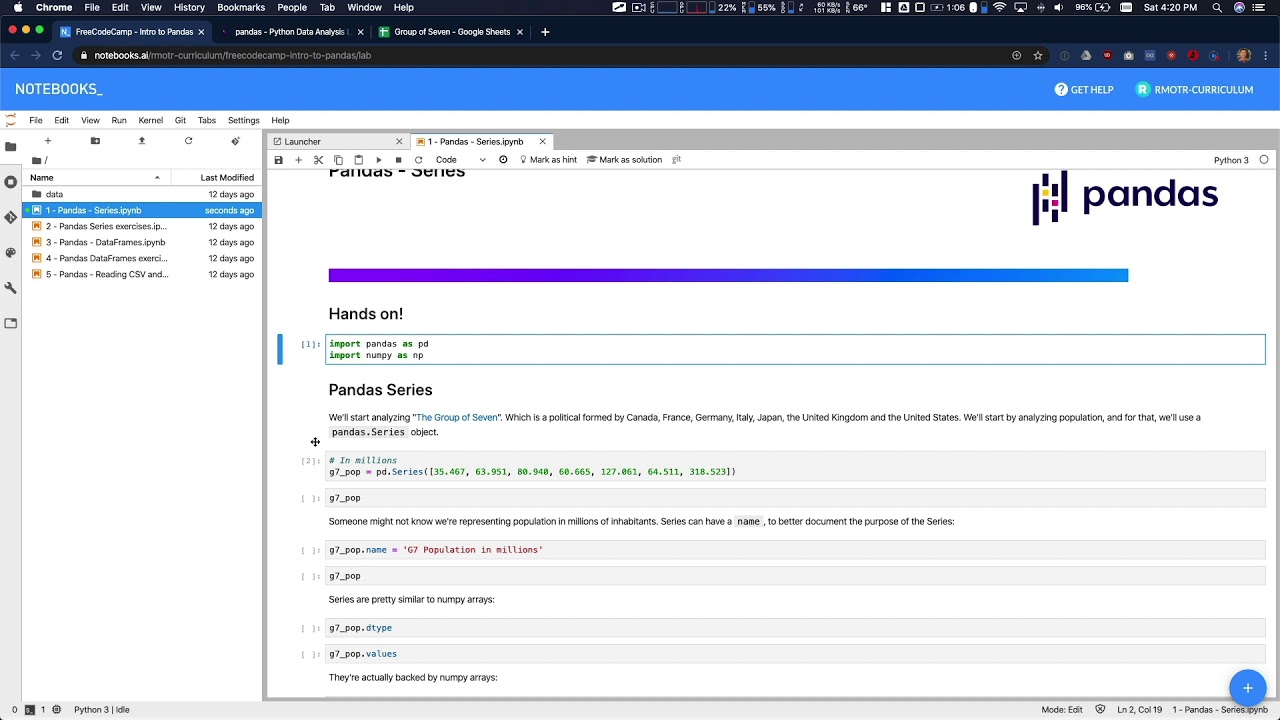
Pandas Introduction - Data Analysis with Python Course
5.0 / 5 (0 votes)