#04 Angular files and folder structure| Getting Started with Angular | A Complete Angular Course
Summary
TLDRThis lecture offers an insightful overview of Angular project structure, focusing on the files and folders generated by Angular CLI. It explains the purpose of each, including the 'node_modules' for third-party libraries, 'package.json' for project configurations and dependencies, and the crucial 'angular.json' for project-wide settings. The video also delves into the 'src' folder, highlighting its role in housing application source code and differentiating between global and component-specific CSS styles. The session aims to familiarize developers with essential Angular components, setting a foundation for deeper exploration in subsequent lectures.
Takeaways
- đ The 'node_modules' folder stores all third-party libraries that the Angular application depends on and is not deployed in production or checked into the repo.
- đ ïž Developers can reinstall dependencies by running 'npm install' after checking out the project code, which is not including the 'node_modules' folder.
- đ The 'package.json' file contains project configurations and lists of dependencies and devDependencies that the Angular project relies on.
- đ The 'package-lock.json' file ensures consistent installation of dependencies across different environments by recording their exact versions.
- đ„ The '.editorconfig' file sets coding standards for a team, helping to maintain consistency across multiple developers' contributions.
- đ« The '.gitignore' file specifies which files and folders to exclude from the git repository, such as 'node_modules' and other non-essential files.
- đ ïž The 'angular.json' file is crucial for Angular project configuration, detailing project settings, styles, scripts, and the main entry point.
- đ The 'tsconfig.json' file includes settings for the TypeScript compiler, which compiles TypeScript code into JavaScript for browser execution.
- đïž The 'src' folder is the central location for all application source code, including components, services, and modules.
- đž The 'assets' folder holds static assets like images and icons, which are publicly accessible when referenced in the application.
- đ The 'index.html' file is the main HTML rendered in the browser, with dependencies injected during the build process by Angular CLI.
Q & A
What is the purpose of the 'node_modules' folder in an Angular project?
-The 'node_modules' folder is used to store all the third-party libraries that the Angular application might depend on. These libraries are downloaded from npm and are essential for the development of the application. However, this folder is not deployed to the production server nor checked into the version control system like git.
Why is the 'node_modules' folder not included when pushing to a git repository?
-The 'node_modules' folder is not included in the git repository because it is purely for development purposes. It can be regenerated by running 'npm install' whenever needed, which makes it unnecessary to keep in version control.
How does an Angular application function without the 'node_modules' folder?
-If the 'node_modules' folder is missing, such as after checking out code from a repository, the developer can run 'npm install' to download and install all the necessary packages that the Angular project depends on, thus regenerating the 'node_modules' folder.
What role does the 'package.json' file play in an Angular project?
-The 'package.json' file is a standard configuration file for Node projects, including Angular. It contains project-related configurations, such as the project's name and version, as well as scripts for starting and building the project. Importantly, it lists the dependencies and devDependencies that the project relies on.
How does 'npm install' know which packages to install for an Angular project?
-The 'npm install' command looks inside the 'package.json' file to determine the dependencies and devDependencies that need to be installed. It then downloads and installs these packages into the 'node_modules' folder.
What is the purpose of the '.editorconfig' file in a development team environment?
-The '.editorconfig' file is used to define and maintain consistent coding styles between different editors and IDEs used by developers in a team. It helps to avoid errors and maintain a consistent code style across the project.
What does the '.gitignore' file specify?
-The '.gitignore' file specifies which files and folders should be excluded from the git repository. This is useful for not tracking files that are not needed in version control, such as the 'node_modules' folder or temporary files.
What is the significance of the 'angular.json' file in an Angular project?
-The 'angular.json' file contains all the configurations related to the Angular project, such as project name, type, styles, scripts, and the main entry point. It is the most important configuration file for an Angular application.
What is the 'package-lock.json' file and why is it important?
-The 'package-lock.json' file records the exact version of every installed dependency, including their sub-dependencies. It ensures that the same versions of dependencies are installed consistently across different environments, which is crucial for avoiding inconsistencies in development, production, and other environments.
What is the role of the 'tsconfig.json' file in an Angular project?
-The 'tsconfig.json' file contains settings for the TypeScript compiler. The compiler uses these settings to compile TypeScript code into JavaScript that browsers can understand. It's an important configuration for managing how the code is transpiled.
What is the 'src' folder in an Angular project and why is it important?
-The 'src' folder is where all the application's source code is stored. It contains components, services, modules, and other essential parts of the application. Developers spend most of their time in this folder, creating and managing the application's functionality.
What is the purpose of the 'assets' folder in an Angular project?
-The 'assets' folder is used to store static assets such as images, icons, and text files. These assets are made public and can be accessed directly from the browser, making it the place to keep all publicly accessible resources.
Why is the 'index.html' file in the 'src' folder important for an Angular application?
-The 'index.html' file is the main HTML file that gets rendered in the browser when the Angular application runs. It is where the application is bootstrapped, and all dependencies are injected during the build process by the Angular CLI.
What is the 'main.ts' file and its significance in starting an Angular application?
-The 'main.ts' file is the starting point of an Angular application. It is where the app module is bootstrapped, and the application's execution begins. It is similar to a main method in other programming languages, marking the entry point of the application.
What is the difference between global CSS styles and component-specific CSS styles in an Angular project?
-Global CSS styles are defined in the 'style.css' file and are applied to the entire application, affecting all components and directives. In contrast, component-specific CSS styles are defined in a component's own CSS file (e.g., 'app.component.css') and only affect the HTML elements of that particular component.
Outlines
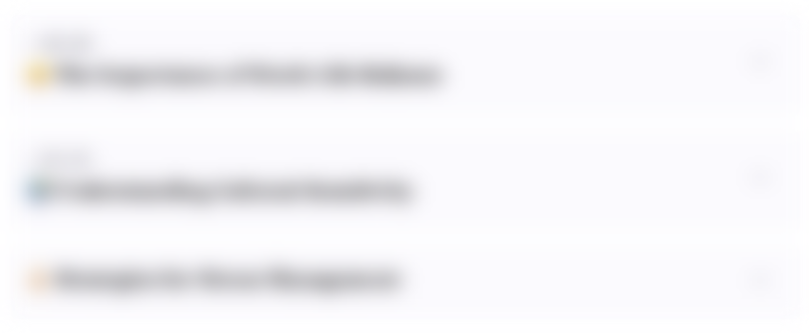
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
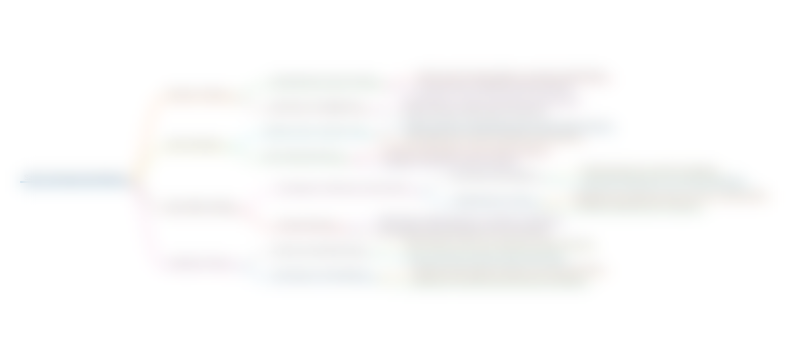
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
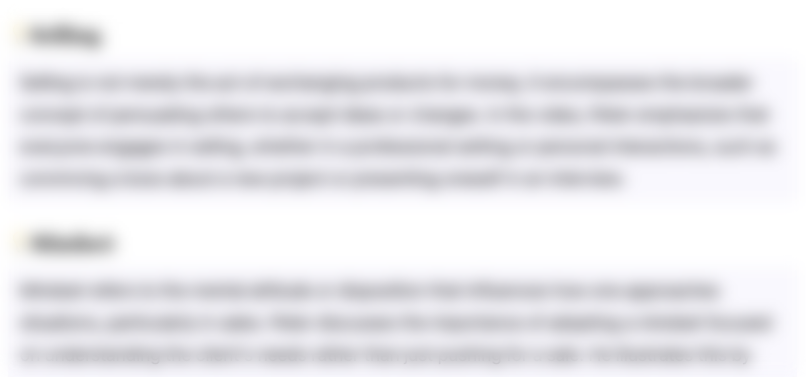
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
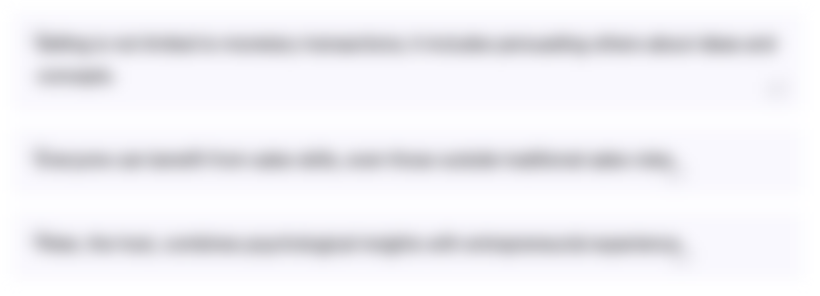
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
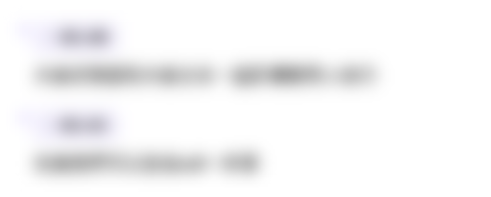
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
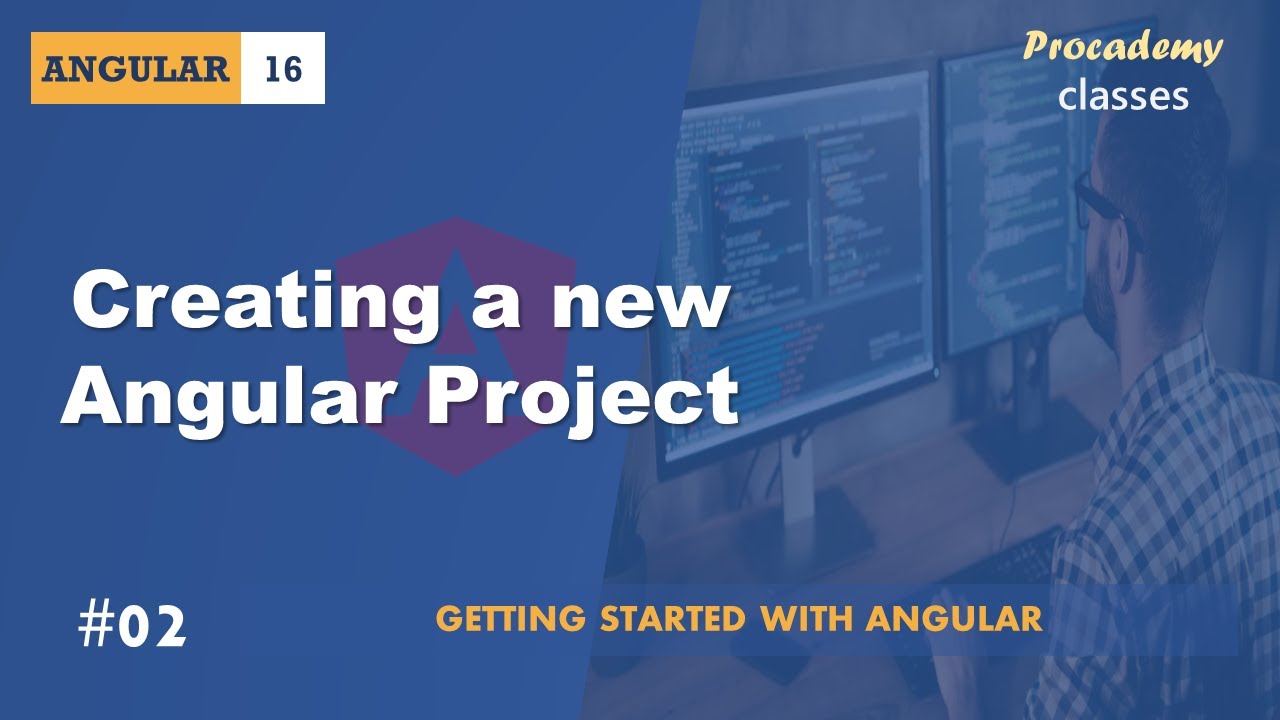
#02 Creating a new Angular Project | Getting Started with Angular | A Complete Angular Course
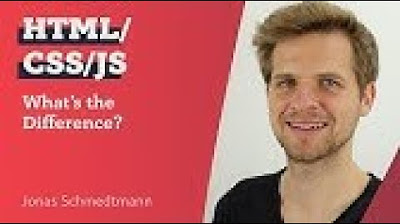
FAQs Angular Maximilian Schwarzmuller
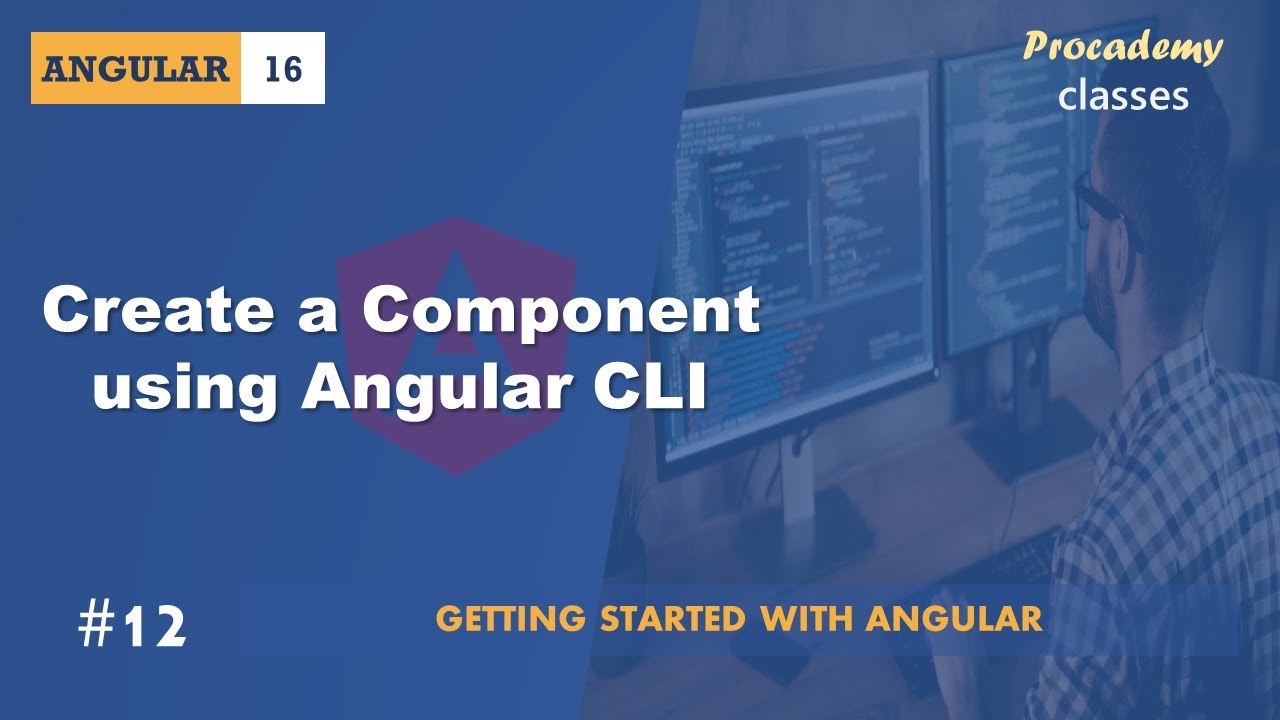
#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course
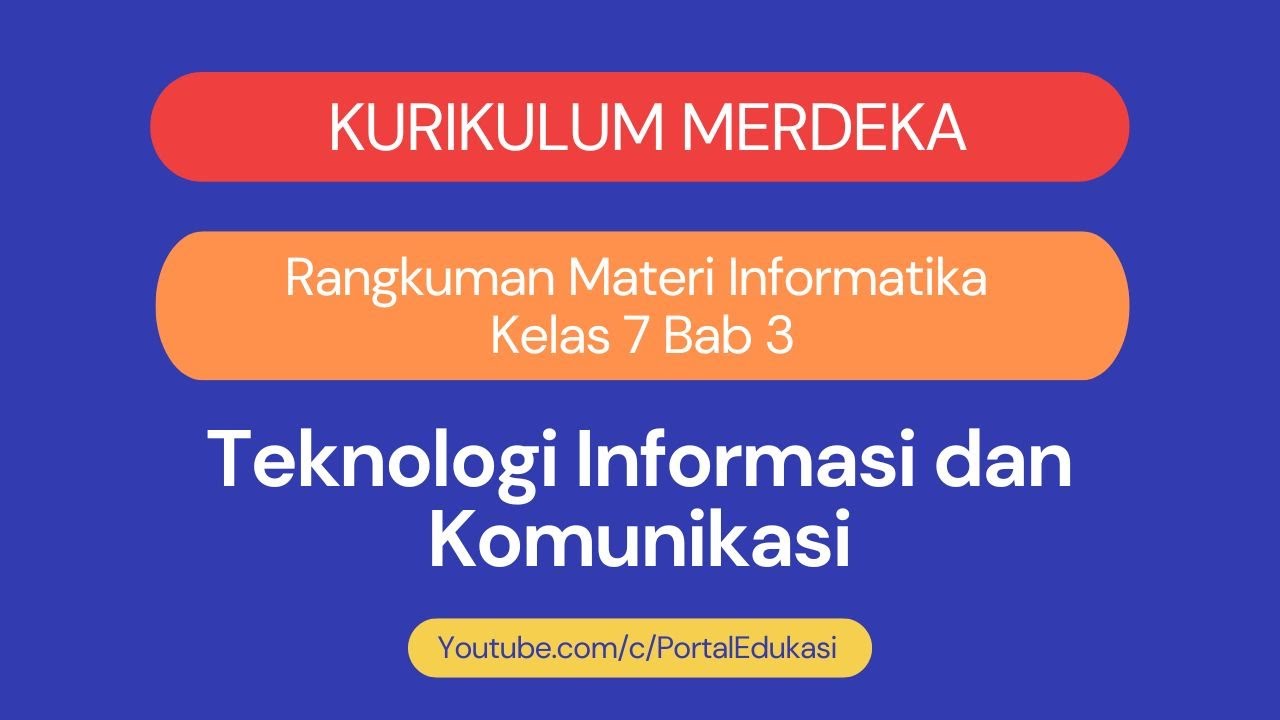
Kurikulum Merdeka Materi Informatika Kelas 7 Bab 3 Teknologi Informasi dan Komunikasi

#03 Editing the First Angular Project | Getting Started with Angular | A Complete Angular Course
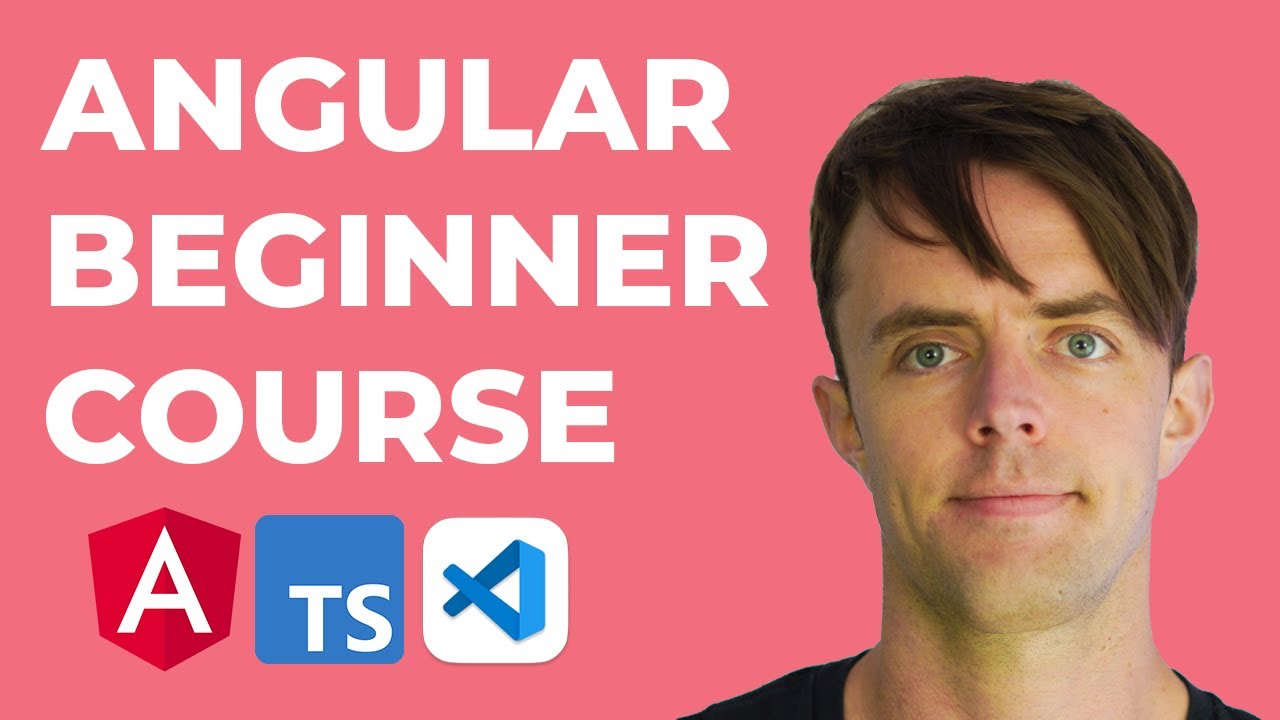
Angular Tutorial For Beginners 2022 - 1. Install & Folder Structure
5.0 / 5 (0 votes)