#8 Functions in C and the call stack
Summary
TLDRIn this lesson on Embedded Systems Programming, Miro Samek introduces C functions and the stack, emphasizing their importance in understanding low-level behaviors like function calls, interrupts, and RTOS. The lesson explains how functions work, starting with a simple delay function, and explores concepts such as function prototypes, the stack pointer (SP), and how arguments are passed to functions. By the end, learners understand how the stack manages local variables and return addresses, while gaining insight into how functions can be reused and optimized in embedded systems programming.
Takeaways
- 😀 Functions in C are reusable blocks of code that can be called from different parts of the program.
- 😀 The C stack is a key component for understanding function calls, interrupts, context switches, and RTOS.
- 😀 The DRY (Don't Repeat Yourself) principle encourages using functions to avoid code repetition.
- 😀 A function in C is defined by its name, arguments, and return type, collectively known as its signature.
- 😀 Calling a function in C involves transferring control to the function, executing it, and then returning to the next instruction.
- 😀 Function prototypes, which contain the function's signature, must be defined before the function itself in C programming.
- 😀 The 'Link Register' (LR) is used to store the return address during function calls, enabling the program to return to the correct location.
- 😀 The stack pointer (SP) in ARM Cortex-M processors is responsible for managing the call stack, which grows and shrinks as functions are called and return.
- 😀 When a function is called, the stack is used to store local variables and return addresses. This is essential for function execution and returning to the correct point in the code.
- 😀 Function arguments in C allow the customization of function behavior, and the compiler ensures that the correct number and type of arguments are provided for each call.
Q & A
What is the main focus of this lesson on embedded systems programming?
-The main focus of this lesson is to explain how the C Stack works to enable function calls, including calling functions that call other functions, and understanding their low-level behavior, which is crucial for functions, interrupts, context switches, and RTOS.
What is the DRY principle in programming and how does it relate to this lesson?
-The DRY principle stands for 'Do Not Repeat Yourself.' It emphasizes reducing repetition in code. In this lesson, the principle is applied by replacing repetitive delay loops with a reusable function, which can be called multiple times instead of writing the same code over and over.
What are the three main elements that make up the signature of a C function?
-The three main elements of a C function's signature are the return type, the function name, and the list of arguments.
What is a function prototype in C, and why is it important?
-A function prototype is a declaration of a function, which includes the signature (return type, name, and arguments) followed by a semicolon. It is important because it informs the compiler of the function’s existence and helps it check for errors before the function is defined.
What happens when the function call is made in ARM Cortex-M processors?
-When a function is called in ARM Cortex-M processors, a branch instruction (BL) is executed. This instruction changes the program counter to the function's address and saves the return address in the Link Register (LR), allowing the processor to return to the correct place after the function completes.
What is the role of the Stack Pointer (SP) in function calls?
-The Stack Pointer (SP) is a register that points to the top of the stack, which is a section of RAM. In function calls, the SP is used to allocate space for local variables, and when a function call is made, the stack grows or shrinks accordingly.
How does the ARM Cortex-M processor handle function return using the BX instruction?
-The BX instruction is used to return from a function by setting the Program Counter (PC) to the address stored in the Link Register (LR). It also checks the least-significant bit of LR to determine whether to use the THUMB instruction set, though on Cortex-M, it always uses the THUMB2 instruction set due to hardware limitations.
What is the importance of the stack in handling function calls in C?
-The stack plays two key roles in function calls: it holds local variables of the called functions and stores return addresses. The stack allows functions to execute without overwriting important data and ensures that control returns to the correct place after a function finishes.
Why is the 'require prototypes' option recommended when working with functions?
-The 'require prototypes' option is recommended because it enforces stricter checks by ensuring that the function prototypes match the actual function calls. This prevents errors such as missing or mismatched arguments, making the code more robust.
How does the delay function use arguments to control its behavior?
-The delay function takes an argument 'iter', which controls the number of iterations for the delay loop. By passing different values for 'iter' when calling the function, the delay duration can be adjusted dynamically, allowing for more flexible timing control.
Outlines
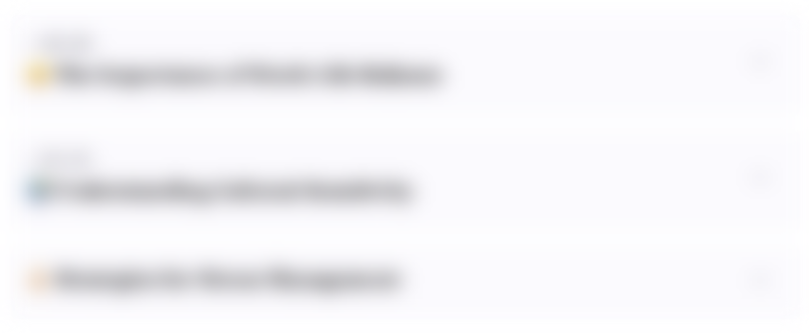
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
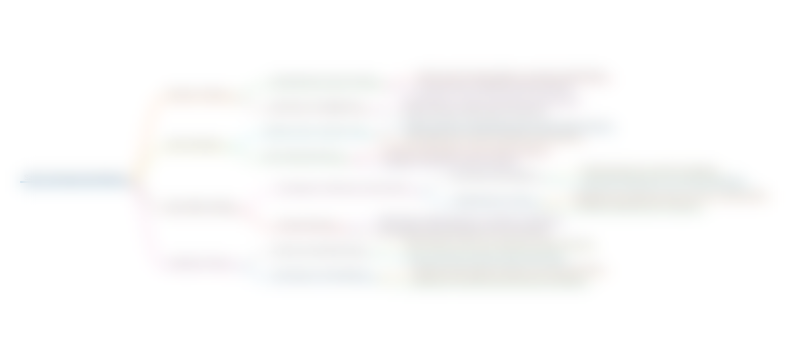
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
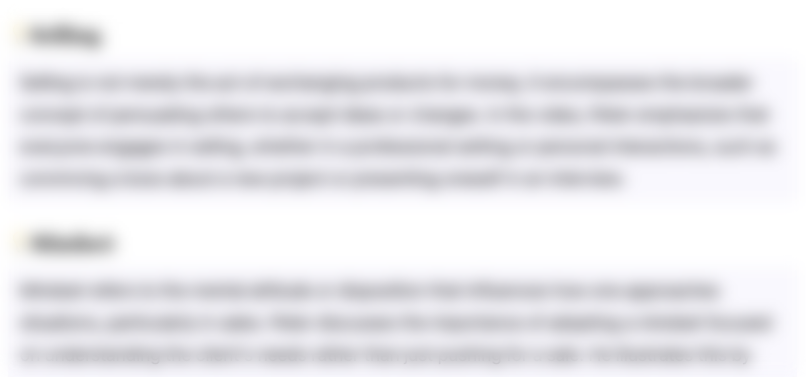
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
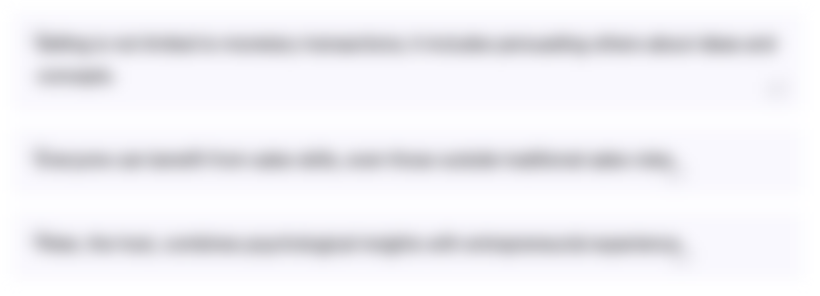
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
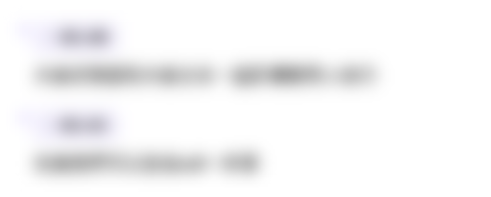
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
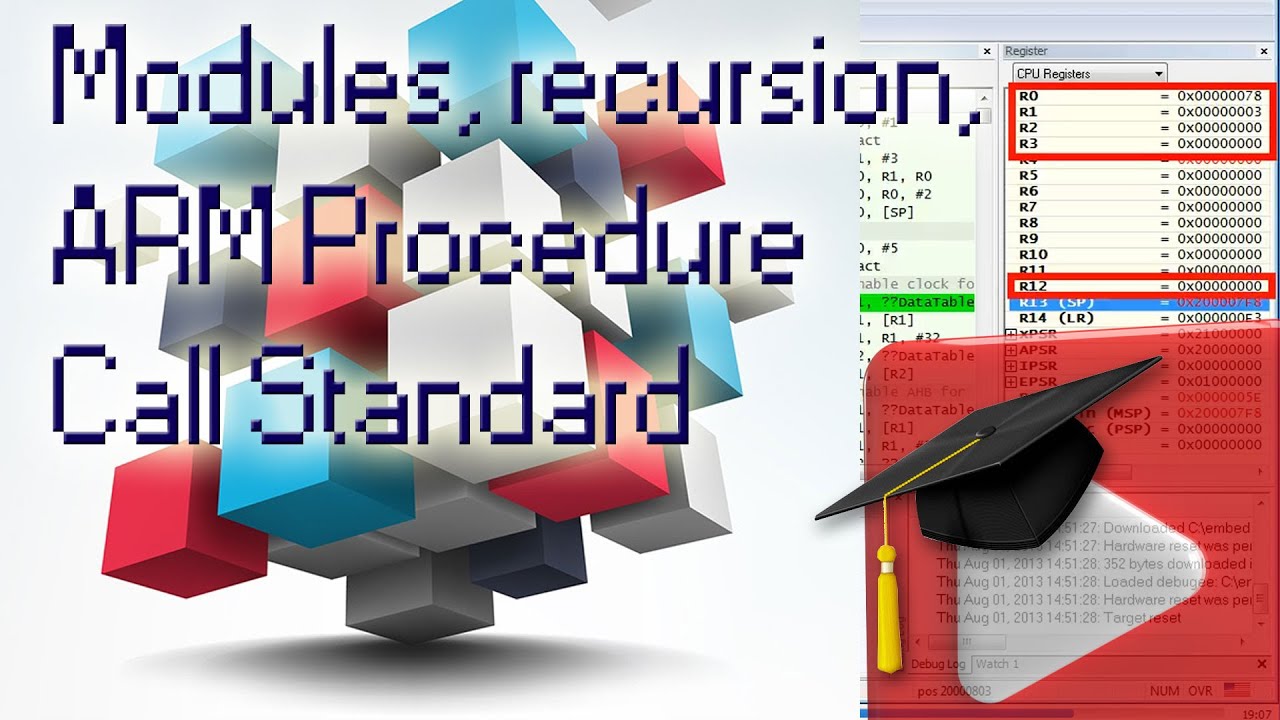
#9 Modules, Recursion, ARM Application Procedure Call Standard (AAPCS)
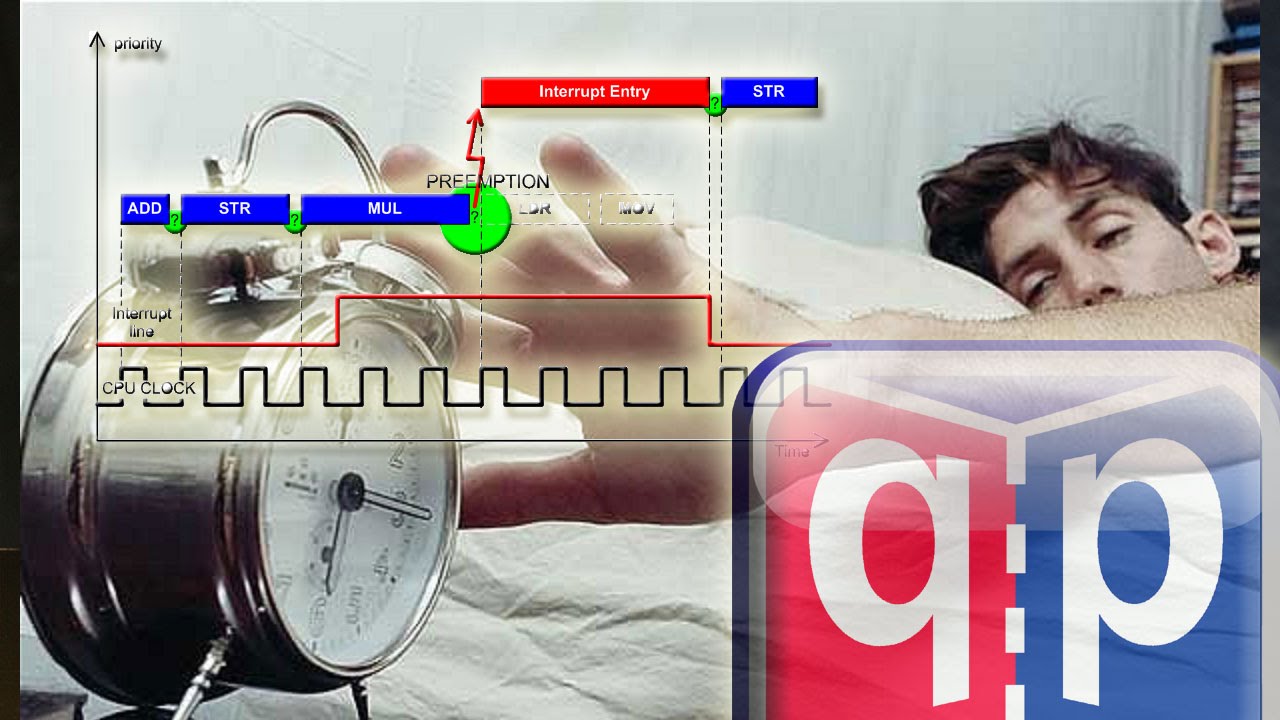
#16 Interrupts Part-1: What are interrupts, and how they work
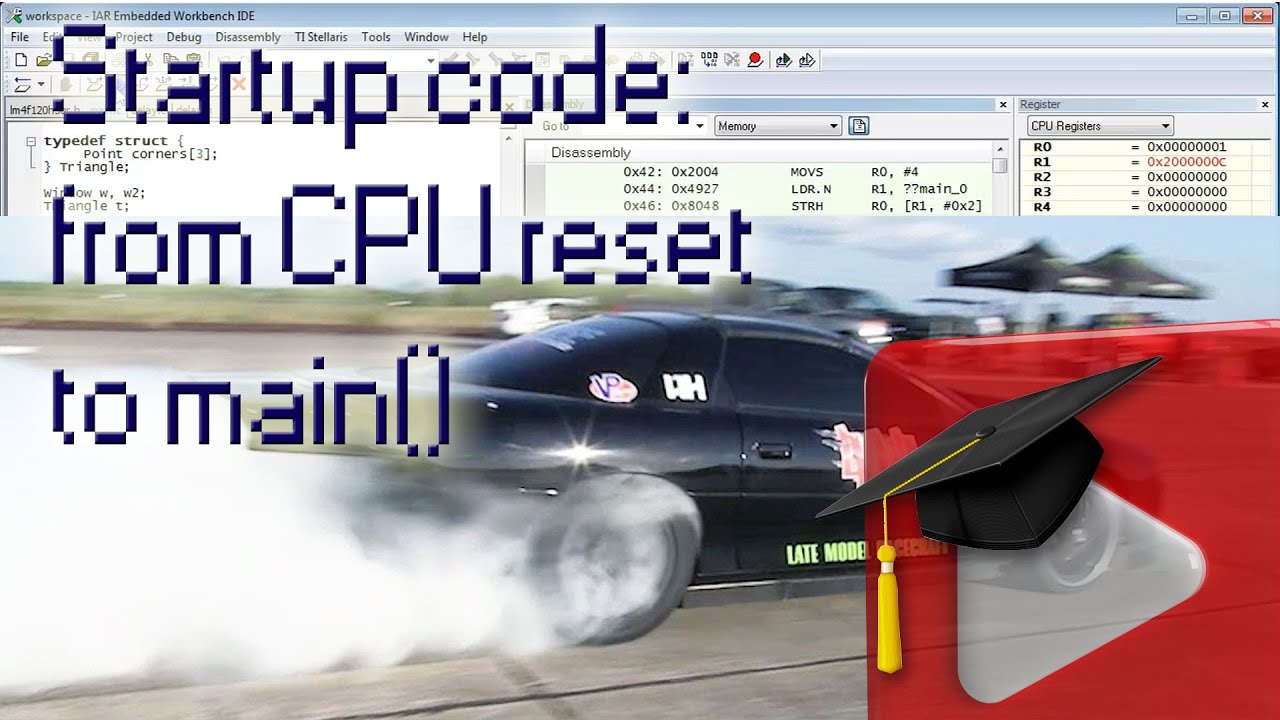
#13 Startup Code Part-1: What is startup code and how the CPU gets from reset to main?
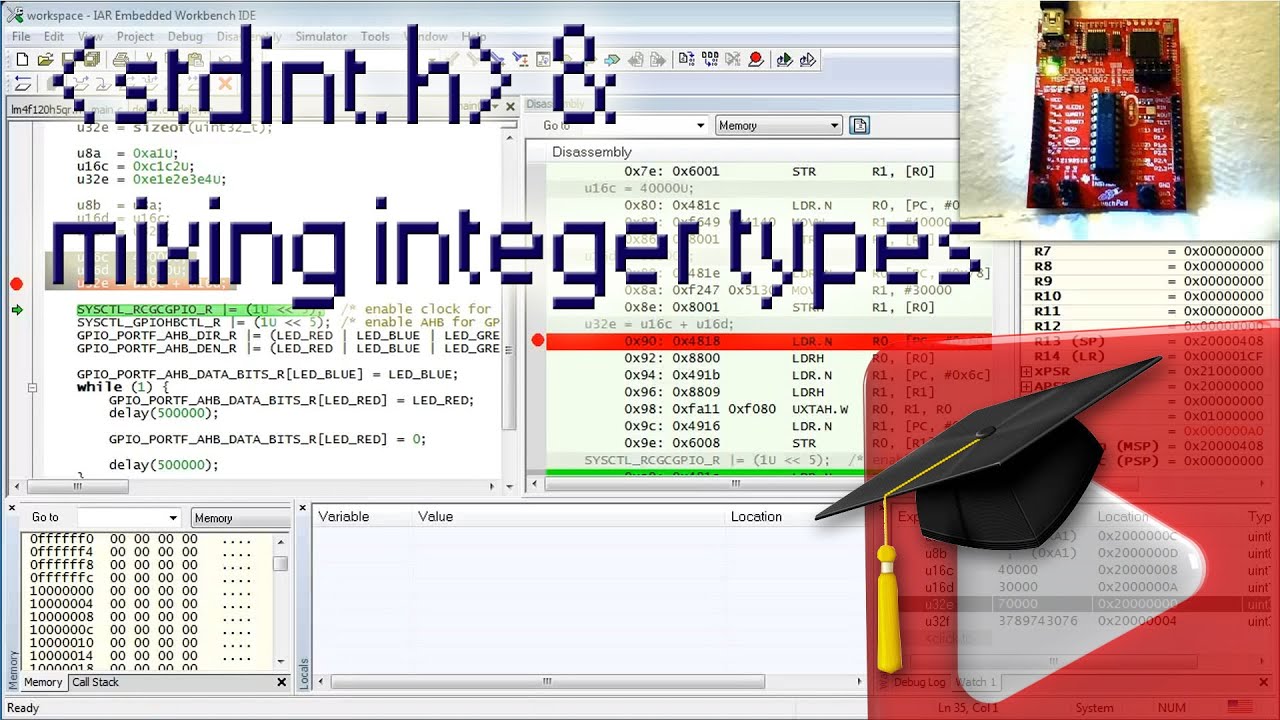
#11 Standard integers (stdint.h) and mixing integer types
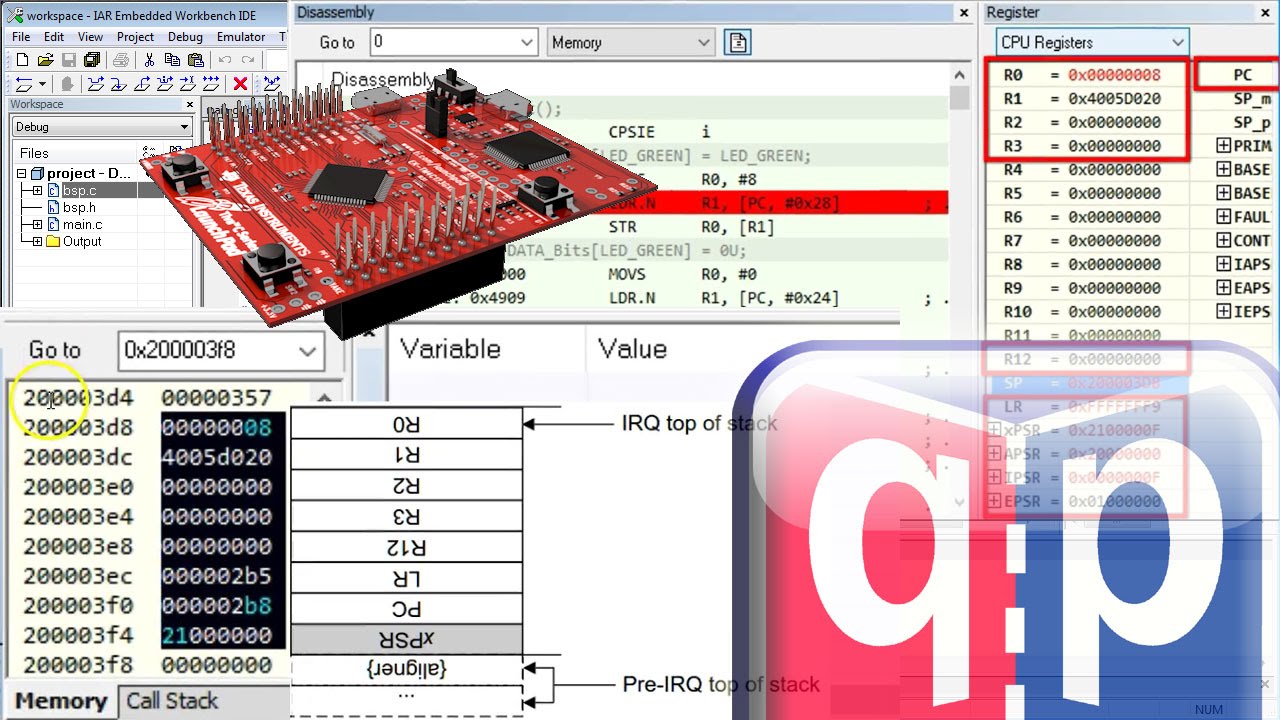
#18 interrupts Part-3: How interrupts work on ARM Cortex-M?
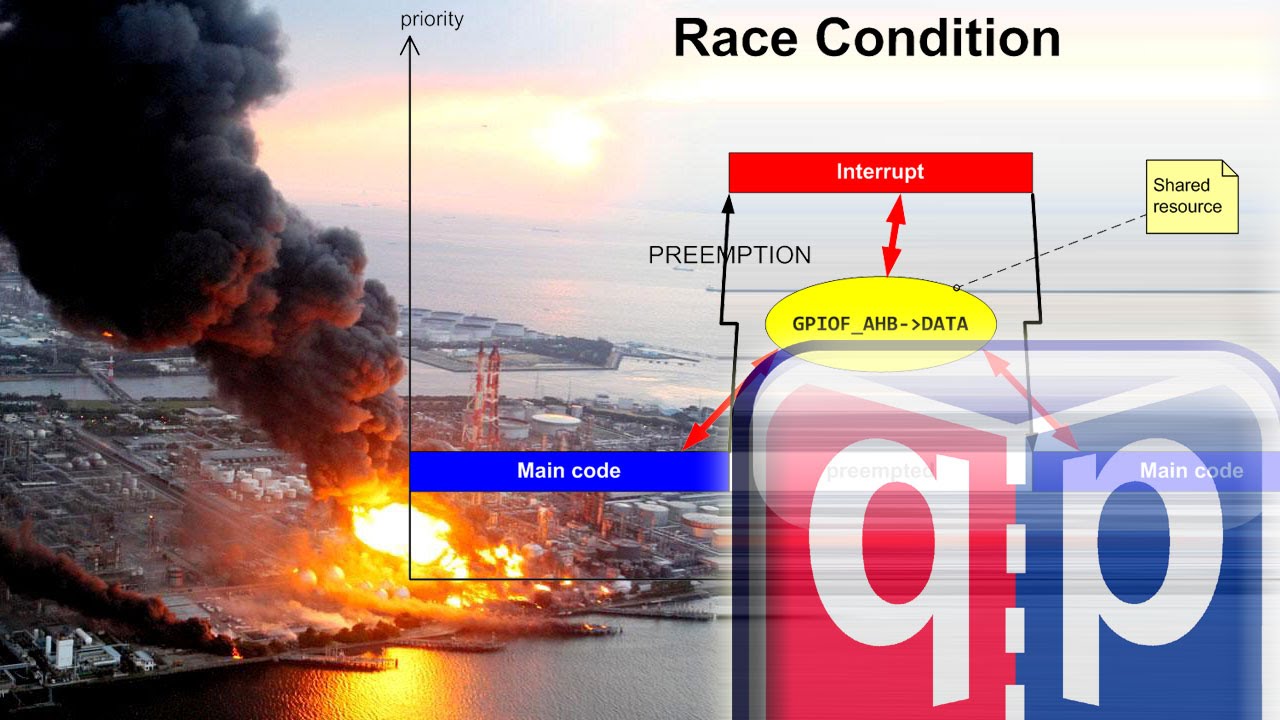
#20 Race Conditions: What are they and how to avoid them?
5.0 / 5 (0 votes)