#11 Standard integers (stdint.h) and mixing integer types
Summary
TLDRIn this Embedded Systems Programming lesson, Miro Samek discusses fixed-width integer types, endianness, and type mixing in C programming, focusing on their importance in embedded systems. The video explains how the C language standard defines built-in types with varying sizes across different platforms, and how the C99 standard introduced standardized fixed-width integer types via the 'stdint.h' header. The lesson covers potential issues such as type promotion and signed-unsigned mixing, offering real-world examples and solutions for writing portable code across processors like ARM and MSP430. It concludes with insights on the complexities of type conversions in C and how to avoid common pitfalls in embedded programming.
Takeaways
- 😀 The C language does not specify the size of built-in integer types, which can vary depending on the target processor.
- 😀 In embedded systems, it's essential to know the exact size, signedness, and dynamic range of variables for consistent performance across different processors.
- 😀 The 'stdint.h' header file in the C99 standard provides standardized fixed-width integer types, making code more portable across different compilers and processors.
- 😀 The 'sizeof()' operator in C allows developers to check the size of variables and data types in bytes, ensuring the expected size of variables.
- 😀 ARM Cortex-M processors use little-endian configuration by default, which affects how multi-byte variables are stored in memory.
- 😀 When mixing data types in expressions, C automatically promotes smaller-size integers to the built-in 'int' or 'unsigned int' type before performing calculations.
- 😀 Implicit type conversions in C can lead to unexpected behavior, such as overflow or loss of precision, especially when working on machines with different word sizes.
- 😀 To avoid unexpected results, it's important to explicitly cast operands to ensure that calculations are performed at the desired precision (e.g., casting to uint32_t).
- 😀 Mixing signed and unsigned integers in expressions can lead to problems, as C promotes both operands to unsigned integers, which may cause incorrect results if not handled properly.
- 😀 Comparisons between signed and unsigned integers can be problematic in C, leading to logical errors, such as always evaluating a comparison between a signed negative value and an unsigned integer as false.
- 😀 In binary operations, promoting small integers to 'int' can result in unintended behavior. It's crucial to use explicit type casting to avoid issues with small integers being promoted incorrectly.
Q & A
Why does the C standard not prescribe the size of built-in integer types like 'int' and 'unsigned'?
-The C standard allows flexibility to compiler vendors by not prescribing the size of built-in integer types. This flexibility ensures that compilers can optimize for different hardware architectures, which might vary in their integer width depending on the target machine (e.g., 16-bit, 32-bit, or 64-bit).
What is the advantage of using fixed-width integer types from 'stdint.h' in embedded programming?
-The advantage of using fixed-width integer types from 'stdint.h' is that it ensures a consistent and predictable size for integers across different platforms. This is crucial in embedded programming where exact sizes, signedness, and dynamic range are essential for system reliability and portability.
How does the 'sizeof()' operator help in understanding the size of integer types in C?
-'sizeof()' is a C operator that returns the size, in bytes, of a variable or a data type. By using this operator, developers can confirm that their fixed-width integers have the expected size (e.g., 1 byte for 'uint8_t', 2 bytes for 'uint16_t', etc.), which is critical for ensuring memory efficiency and correctness in embedded systems.
What is the significance of the compiler possibly changing the order of variable definitions in memory?
-The compiler may rearrange the order of variable definitions in memory to optimize for alignment or other factors. This means that developers should never assume the order in which variables are defined will be preserved in memory, as it could lead to unexpected behavior or bugs in embedded systems.
What does little-endian mean, and how does it relate to ARM processors?
-Little-endian means that the least significant byte of a multi-byte data type is stored at the lowest memory address. ARM processors, by default, are little-endian, meaning that when storing multi-byte integers in memory, the least significant byte is placed first, followed by the more significant bytes in increasing memory addresses.
Why is it important to avoid mixing signed and unsigned integers in expressions?
-Mixing signed and unsigned integers can lead to unexpected results due to implicit type promotion. The C standard promotes smaller integer types to 'unsigned int' in expressions, which may cause incorrect results when negative values are involved. To avoid this, it is better to explicitly cast variables to a common type to ensure the correct behavior of the operation.
What problem can occur when an expression is evaluated on a 16-bit machine like the MSP430, compared to a 32-bit machine like ARM?
-On a 16-bit machine like MSP430, smaller integers are promoted to 16-bit 'int' types, leading to overflow in certain computations, as seen when adding 40,000 and 30,000. In contrast, a 32-bit machine like ARM promotes integers to 32-bit 'int', allowing the computation to work as expected without overflow. This discrepancy can lead to unexpected results on different platforms.
How can the issue of overflow on a 16-bit machine be solved when performing arithmetic operations?
-To solve the overflow issue on a 16-bit machine, you can enforce promotion to a 32-bit precision by explicitly casting one or both operands to a 32-bit type (e.g., 'uint32_t'). This ensures that the computation is performed at the larger precision and avoids overflow.
What happens when a signed and unsigned integer are mixed in an arithmetic operation?
-When a signed and unsigned integer are mixed, both operands are promoted to 'unsigned int', and the result is also treated as 'unsigned int'. If the result is assigned to a signed variable, it may lead to unexpected behavior, especially if the unsigned result exceeds the signed range.
Why does the compiler give a warning for a comparison between signed and unsigned integers in C?
-The compiler issues a warning for comparisons between signed and unsigned integers because, due to type promotion rules, the signed integer is converted to an unsigned type. This can result in incorrect comparisons, as negative values in signed integers are treated as large positive numbers when promoted to unsigned types.
Outlines
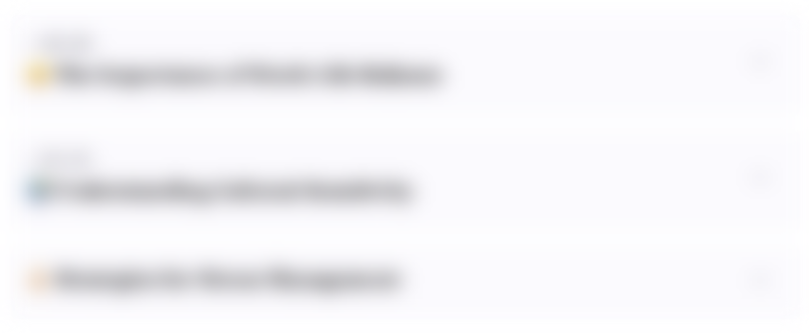
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
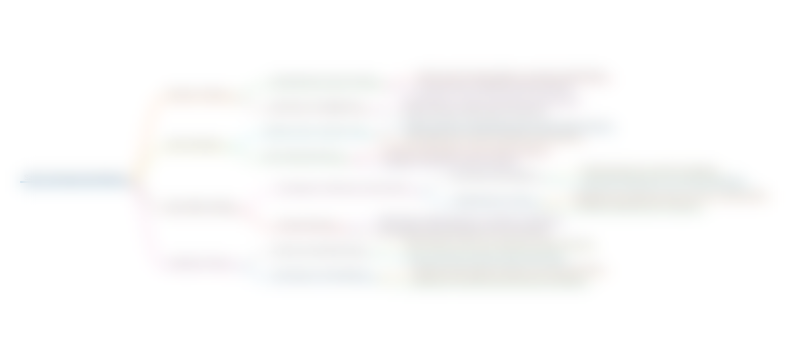
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
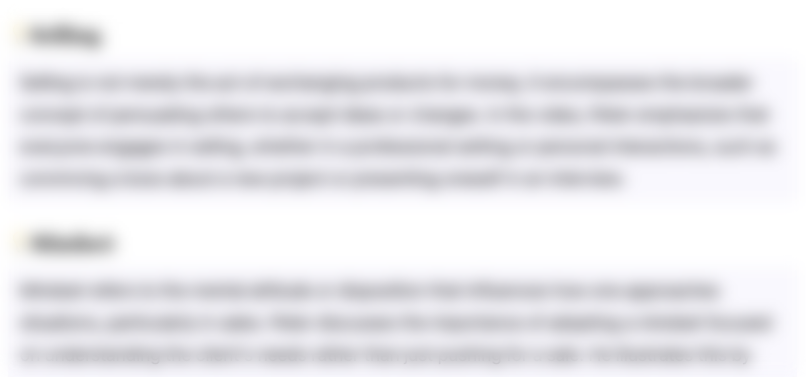
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
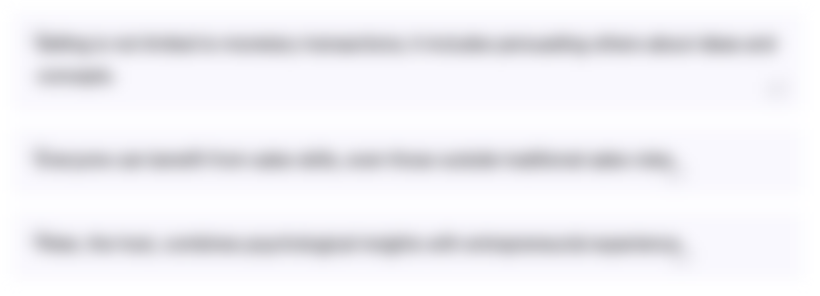
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
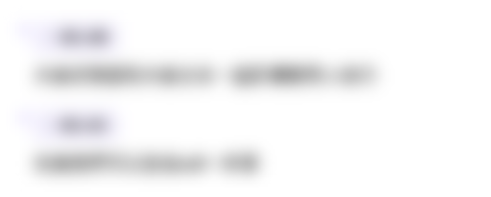
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
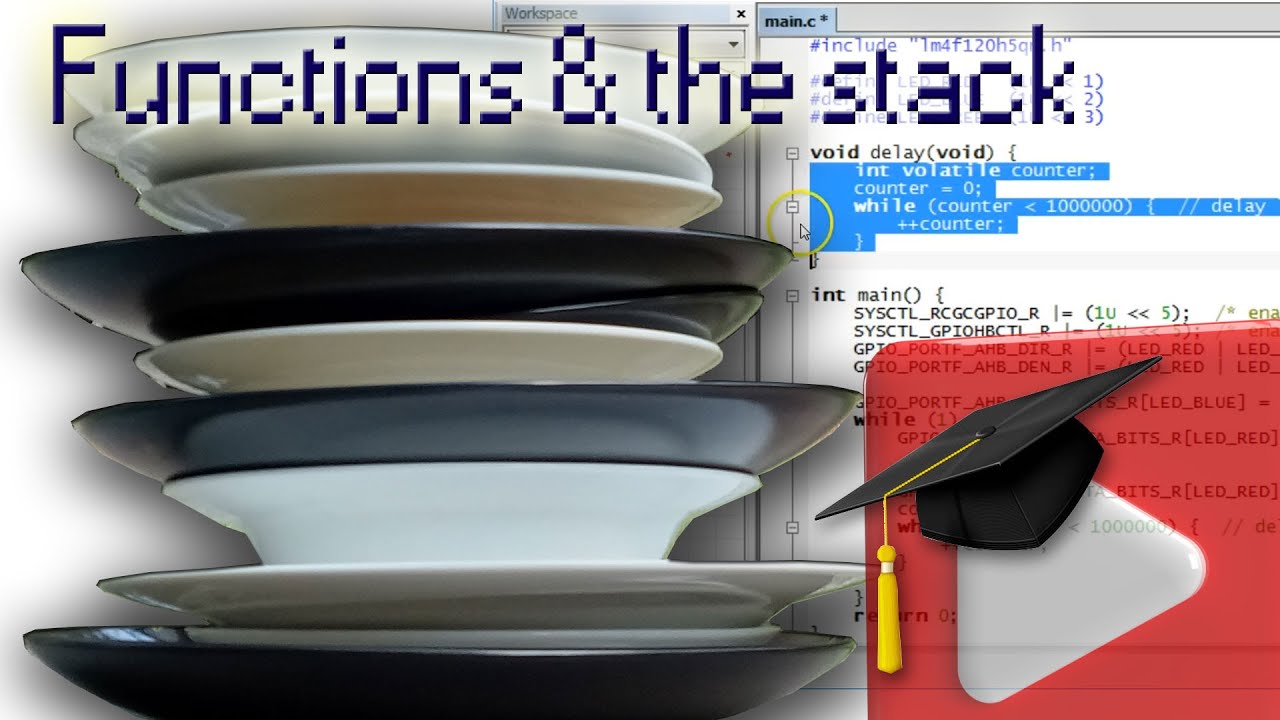
#8 Functions in C and the call stack
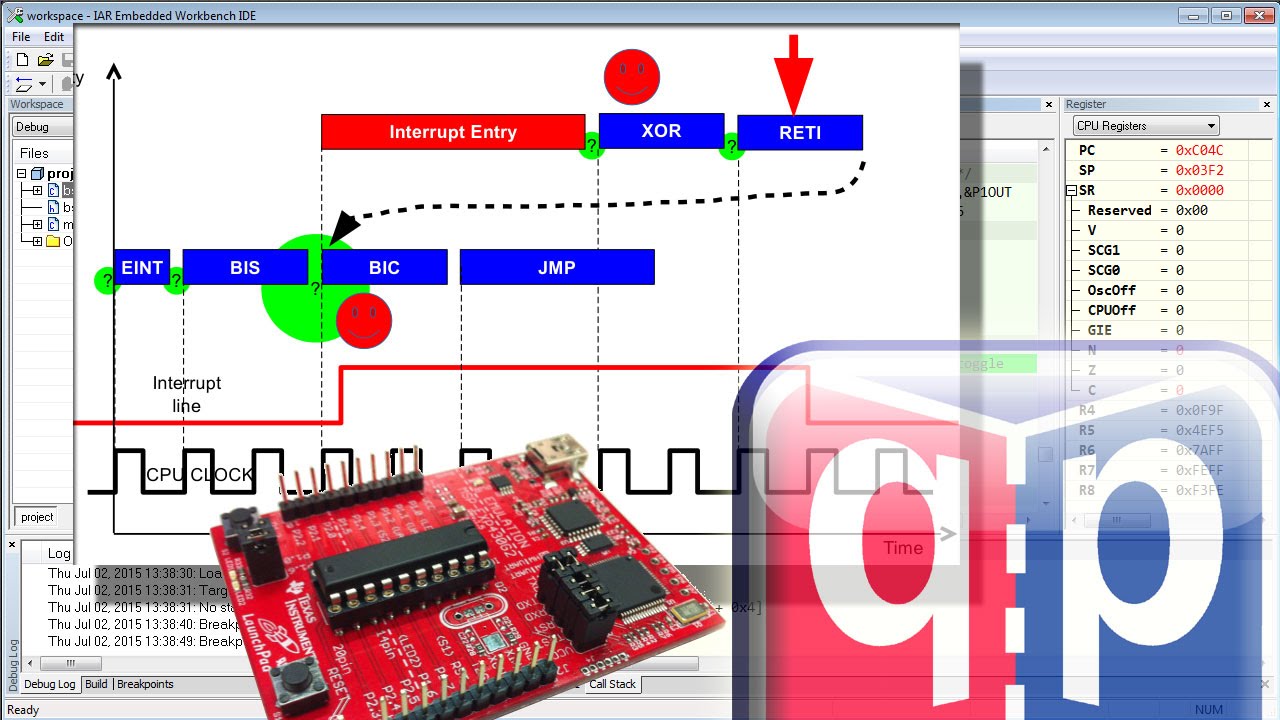
#17 interrupts Part-2: How most CPUs (e.g. MSP430) handle interrupts?

#2 How to change the flow of control through your code
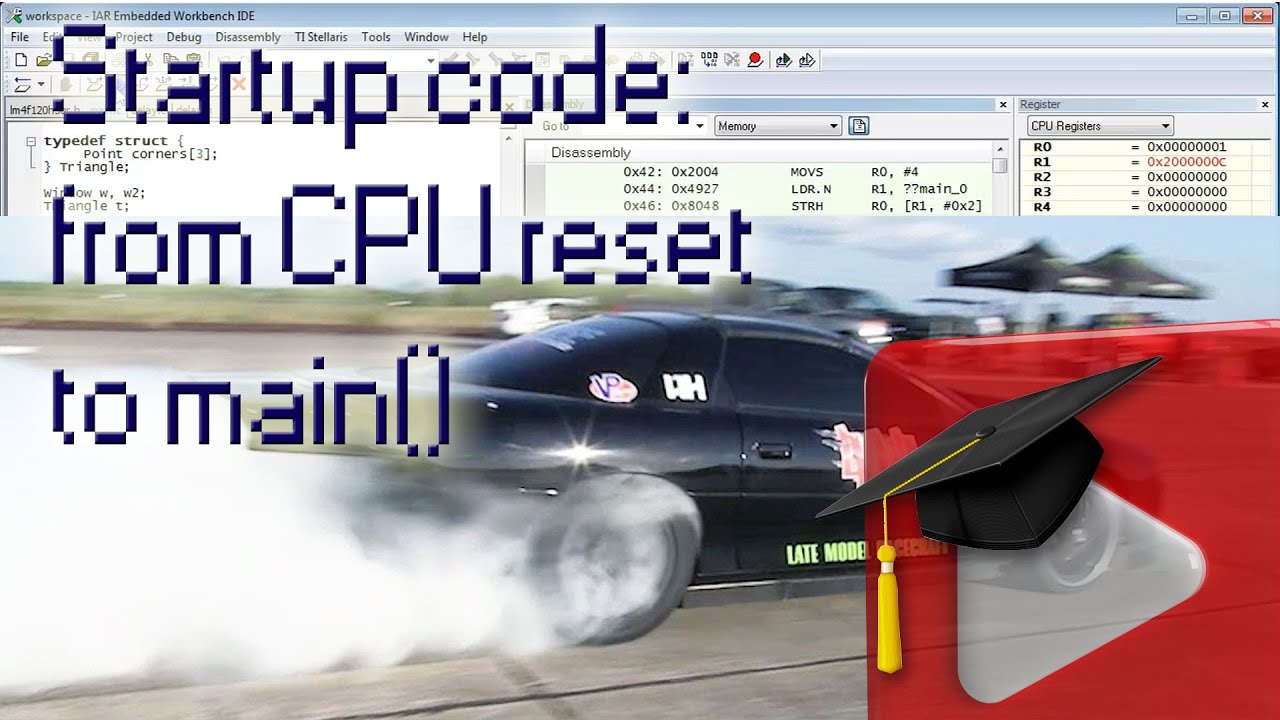
#13 Startup Code Part-1: What is startup code and how the CPU gets from reset to main?
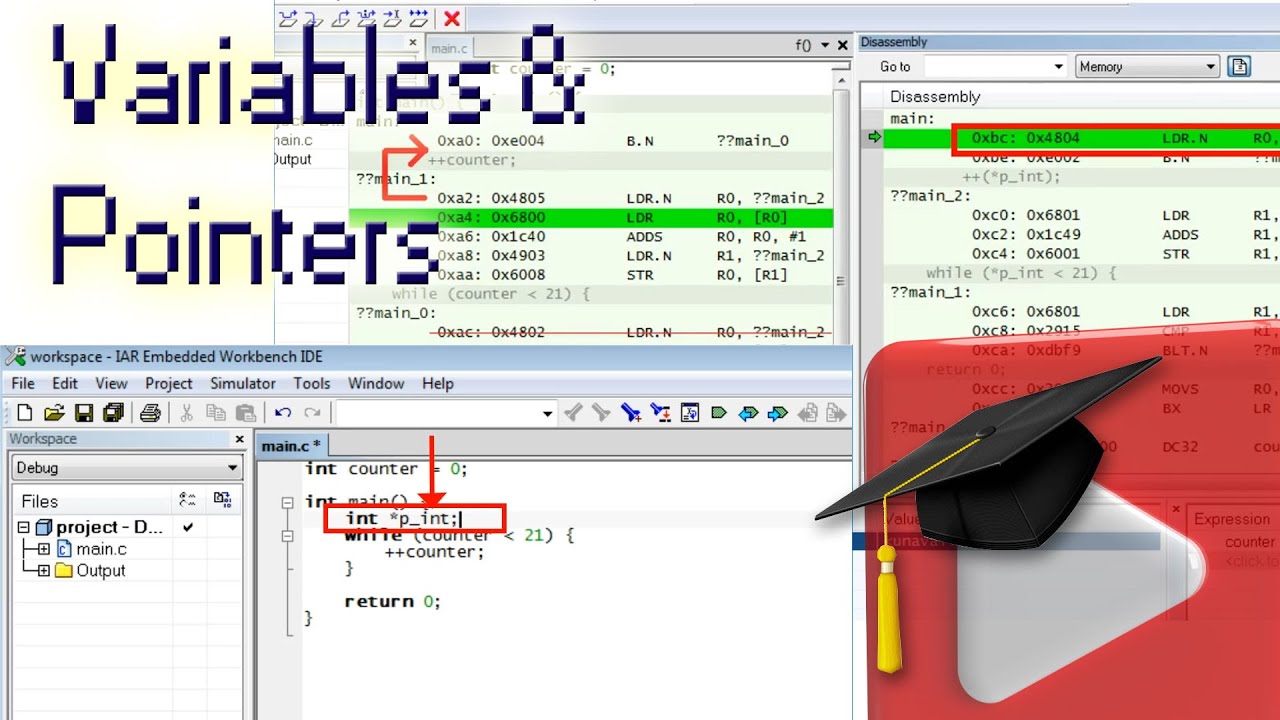
#3 Variables and Pointers

#5 Preprocessor and the "volatile" keyword in C
5.0 / 5 (0 votes)