Control flow dalam pemrograman Python
Summary
TLDRIn this Python programming tutorial, the instructor introduces control flow concepts, including conditional statements (if, elif, else) and looping mechanisms (for, while). The video covers how to use these tools to make decisions and control the execution sequence of a program. Nested conditionals and the break and continue commands are explained in the context of loops. The instructor emphasizes practical examples and provides a clear breakdown of how to implement these concepts in code. The video concludes with an invitation to continue learning about algorithms and applying sequential logic in programming.
Takeaways
- 😀 Control flow in programming refers to the order in which instructions are executed based on conditions or loops.
- 😀 Conditional statements in Python include 'if', 'elif', and 'else' for decision-making based on specified conditions.
- 😀 An 'if' statement runs a block of code only if a specified condition is true.
- 😀 'Elif' (else if) allows for additional checks when the 'if' condition is not satisfied.
- 😀 'Else' handles cases where none of the preceding conditions are met, acting as a fallback.
- 😀 Nested conditions allow combining multiple conditional statements within one another to create more complex logic.
- 😀 Loops in Python, such as 'for' and 'while', allow for repeating a block of code multiple times.
- 😀 A 'for' loop is used to iterate over sequences like lists or ranges, executing a block of code for each item.
- 😀 A 'while' loop repeats code as long as a condition remains true, useful for indefinite iterations.
- 😀 The 'break' statement immediately exits a loop when a certain condition is met, stopping further iterations.
- 😀 The 'continue' statement skips the current iteration of the loop and proceeds to the next iteration, useful for controlling flow within a loop.
Q & A
What is control flow in programming?
-Control flow in programming refers to the sequence in which individual statements or instructions are executed or evaluated. It determines the order in which code is executed based on conditions and loops.
What is the purpose of a conditional statement in Python?
-A conditional statement in Python, such as `if`, `elif`, or `else`, is used to execute certain blocks of code based on whether specific conditions are met. It allows the program to make decisions and alter its behavior accordingly.
How does an 'if' statement work in Python?
-An 'if' statement in Python evaluates a condition. If the condition is true, the associated block of code is executed. If the condition is false, the program skips the block of code.
What is the difference between 'elif' and 'else' in Python?
-'elif' is used to check additional conditions after an 'if' statement, while 'else' executes a block of code when none of the preceding conditions ('if' or 'elif') are met.
Can you give an example of how 'elif' is used in Python?
-Certainly! An example of 'elif' would be: ```python if temperature > 30: print('Hot') elif temperature > 20: print('Warm') else: print('Cold') ``` This checks multiple conditions sequentially.
What is a nested condition, and how does it work?
-A nested condition involves placing one `if` statement inside another. This allows for more complex decision-making processes, as multiple conditions can be checked within the same program structure.
What is the role of a 'for' loop in Python?
-A 'for' loop in Python is used to iterate over a sequence (such as a list or range) and execute a block of code for each item in the sequence.
How does the 'break' statement work in a loop?
-The 'break' statement in a loop is used to exit the loop prematurely. When the condition for 'break' is met, the loop stops executing and control is transferred to the next statement after the loop.
What is the difference between 'continue' and 'break' in Python loops?
-'continue' skips the current iteration of the loop and moves to the next iteration, whereas 'break' completely exits the loop when the specified condition is met.
What is a 'while' loop, and how is it different from a 'for' loop?
-A 'while' loop in Python continues to execute as long as a specified condition remains `True`. Unlike a 'for' loop, which iterates over a sequence, a 'while' loop is typically used when the number of iterations is not known in advance and depends on the condition.
Outlines
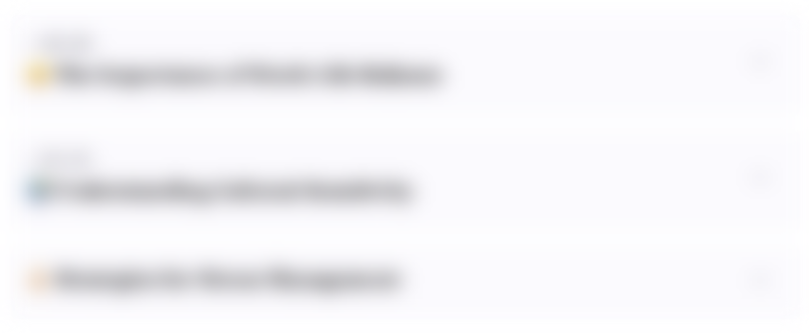
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
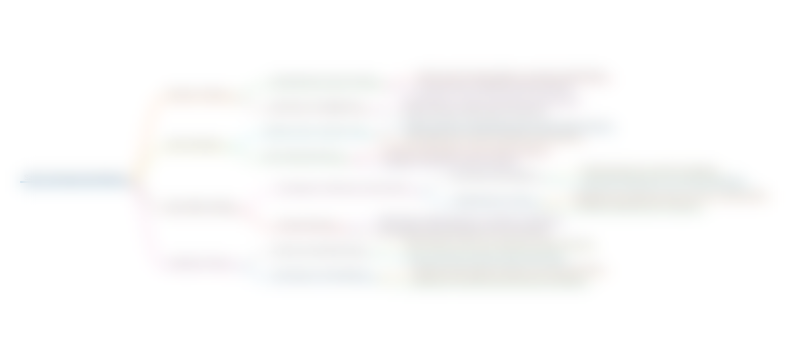
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
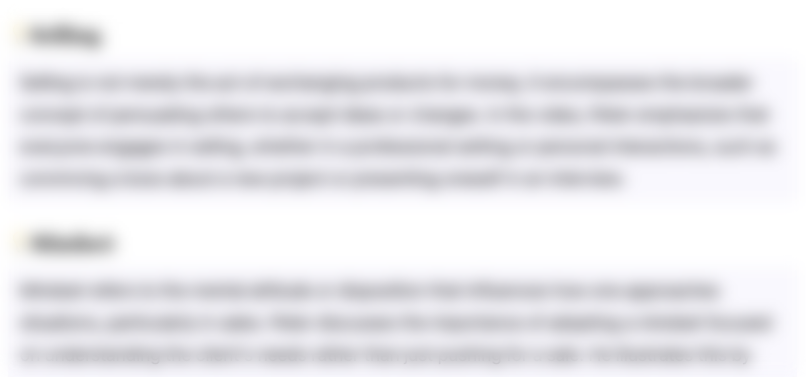
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
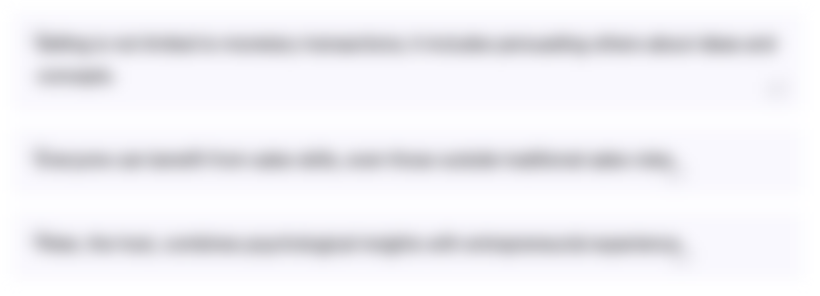
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
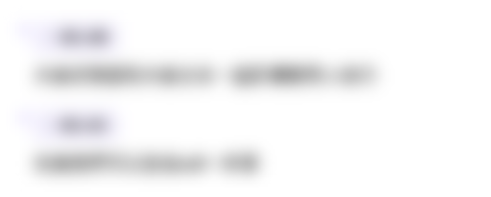
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
5.0 / 5 (0 votes)