React useContext() hook introduction 🧗♂️
Summary
TLDRThe video script explains the use of React's useContext hook for sharing values across multiple component levels without prop drilling. It guides through creating a nested component structure (A to D), setting up state in Component A, and using createContext to create a 'userContext'. It then demonstrates how Component D accesses the username from Component A without passing props through intermediate components, simplifying state sharing in complex component trees.
Takeaways
- 😀 The useContext hook in React allows for sharing values between multiple components without passing props down through every level.
- 📁 The script demonstrates building four components (A, B, C, and D), each nested inside the other.
- 🖱 Component A manages the state using useState, storing a 'user' value and setter, which will be shared across components.
- 💡 Prop drilling involves passing props through several nested components, but this method becomes tedious in deeply nested structures.
- 🛠 The useContext hook helps to avoid prop drilling by enabling direct access to values from any nested component.
- 🌍 The provider component (Component A) wraps child components and provides access to the shared value using the context API.
- 🧩 Consumers, such as Component D, can access the shared value by using the useContext hook, connecting them to the nearest provider.
- 📜 The script outlines three steps for setting up context: importing createContext, creating context, and wrapping child components within the provider.
- 📝 The context consumer can access the shared values by importing both useContext and the context created in Component A.
- 🔁 The useContext hook allows components to access shared data directly, making the flow of data simpler and eliminating the need for passing props down.
Q & A
What is the main purpose of the `useContext` hook in React?
-The `useContext` hook in React allows you to share values between multiple levels of components without passing props through each level, making it easier to manage state across deeply nested components.
What problem does the `useContext` hook solve?
-The `useContext` hook solves the problem of prop drilling, where props have to be passed down through many levels of nested components. By using `useContext`, you can provide data directly to any component in the tree without the need for intermediate components to pass props.
How do you set up a context provider in React?
-To set up a context provider, first import `createContext` from React, then create a context using `const MyContext = createContext()`. In the component where you want to provide the context, wrap the children components with `MyContext.Provider`, and set its value to the data you want to share.
What are the steps to use `useContext` in a consumer component?
-To use `useContext` in a consumer component, follow these steps: 1) Import the `useContext` hook and the context you want to use. 2) Inside the component, call `const value = useContext(MyContext)` where `MyContext` is the context you created. This gives you access to the shared data.
Why is prop drilling considered tedious in React?
-Prop drilling is considered tedious because it requires passing props through multiple layers of components, even when only the deeply nested components need the data. This can make the code harder to maintain and debug, especially as the component tree grows.
How do you create and export a context in React?
-To create and export a context in React, you first import `createContext` from React, then define a context with `const MyContext = createContext()`. Finally, export the context with `export const MyContext` so it can be used in other components.
How does a component become a consumer of a context in React?
-A component becomes a consumer of a context in React by using the `useContext` hook. You import both the `useContext` hook and the context you want to consume, then call `useContext` within the component to access the context’s value.
What does the context provider's `value` prop do?
-The `value` prop of the context provider defines the data that will be shared with the components consuming the context. Any component that uses the `useContext` hook with this context will have access to the `value` provided.
In the example, how is the username passed from `Component A` to `Component D` without props?
-The username is passed from `Component A` to `Component D` without props by wrapping `Component B` inside `Component A` with the `UserContext.Provider` and setting the `value` to the username. `Component D` then uses the `useContext` hook to directly access the username from the `UserContext`.
How can you access the context value in multiple components without repeating code?
-You can access the context value in multiple components without repeating code by using the `useContext` hook in each component that needs the value. Since the context value is provided at a higher level, any component that consumes the context can directly access the value.
Outlines
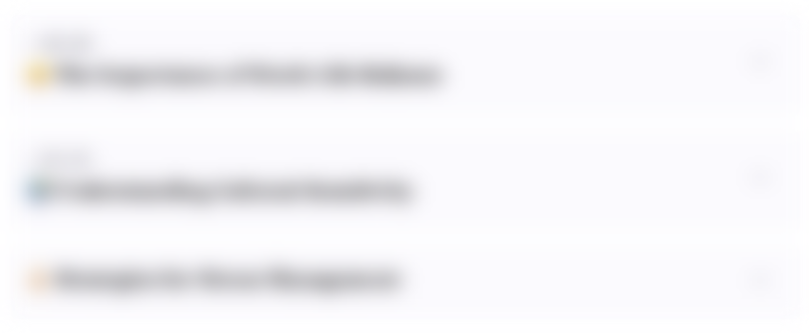
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
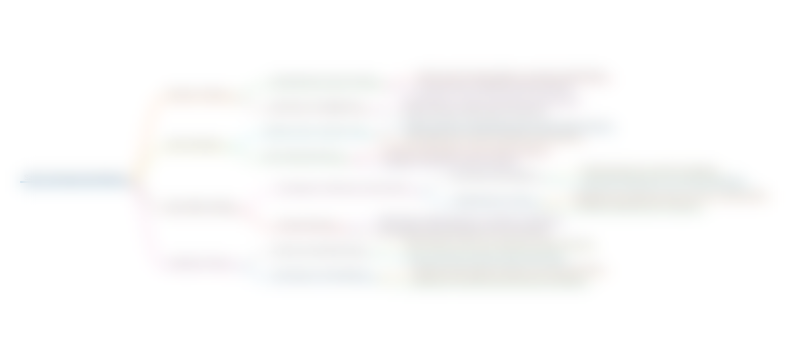
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
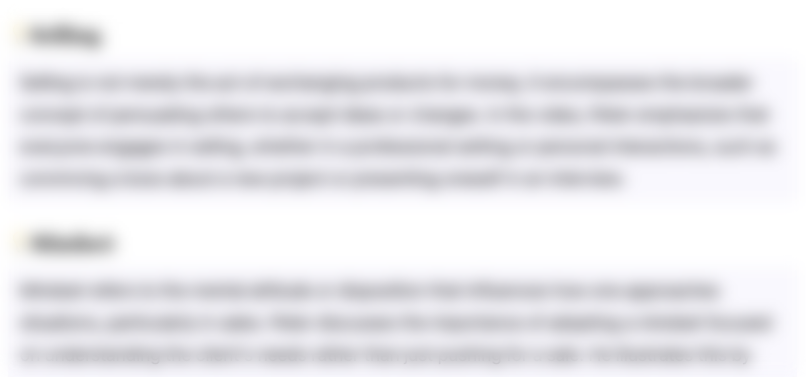
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
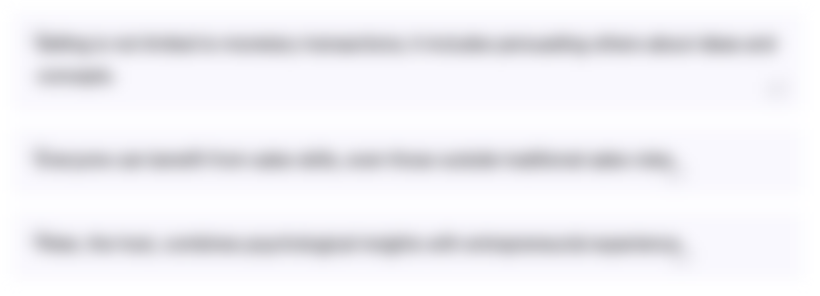
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
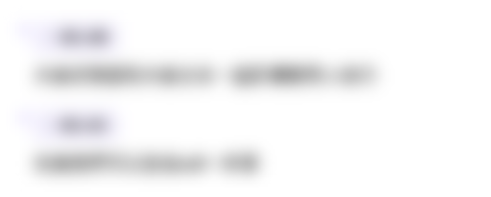
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

Prop Drilling | Lecture 107 | React.JS 🔥

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series

Component Composition | Lecture 108 | React.JS 🔥
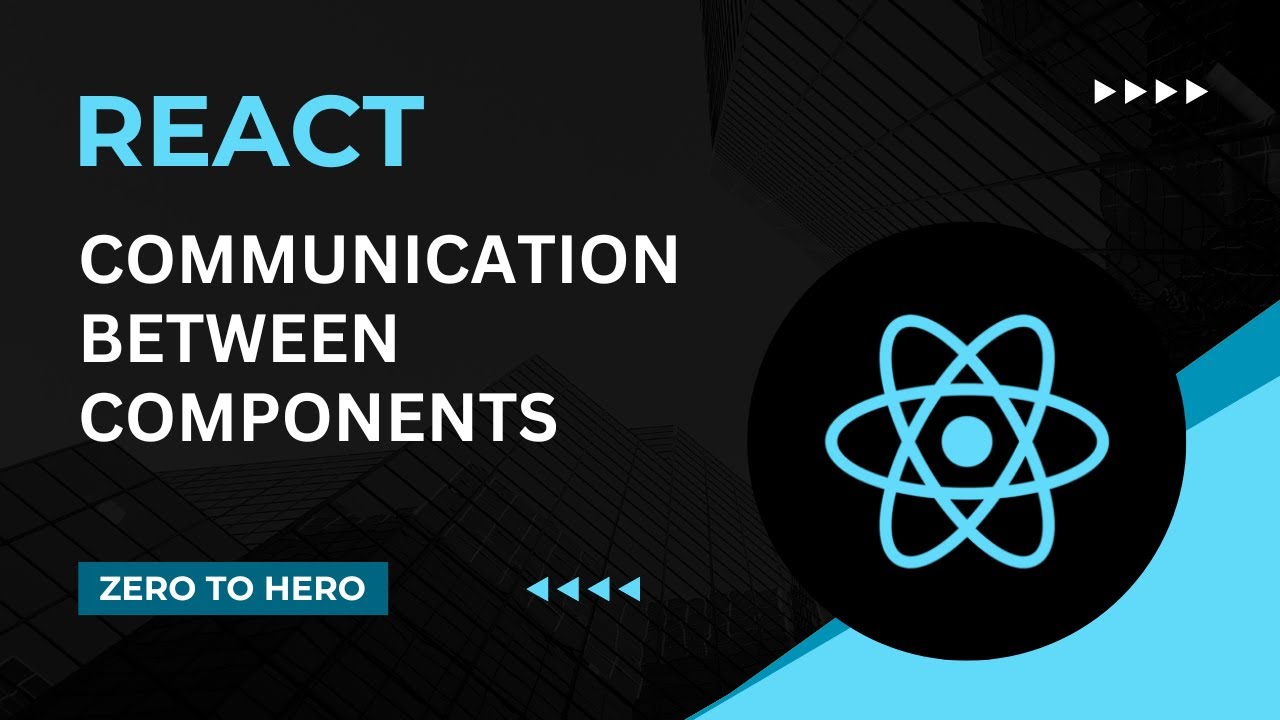
Communication between components | Mastering React: An In-Depth Zero to Hero Video Series
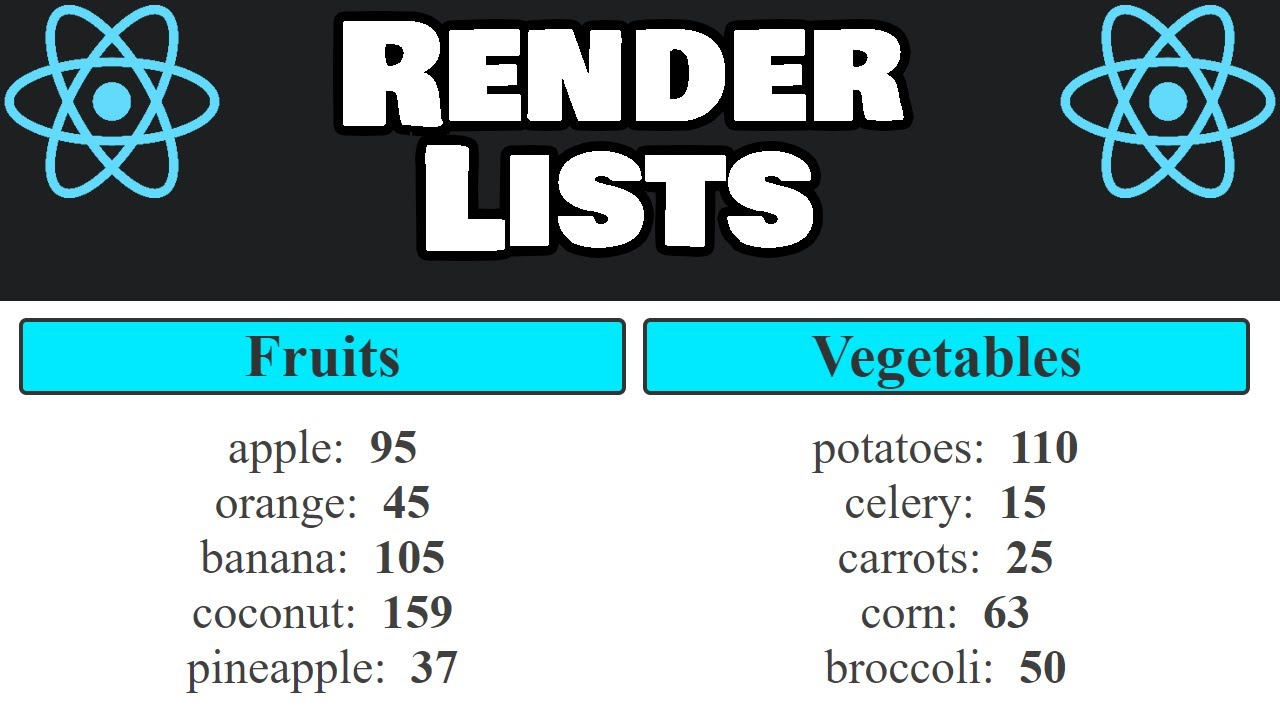
How to render LISTS in React 📃

The useEffect Dependency Array | Lecture 145 | React.JS 🔥
5.0 / 5 (0 votes)