The useEffect Dependency Array | Lecture 145 | React.JS 🔥
Summary
TLDRThis lecture delves into the intricacies of React's useEffect hook, explaining its dependency array and how it synchronizes effects with state and props. It highlights the importance of correctly specifying dependencies to avoid bugs and ensure that effects run only when necessary, after the component has been rendered. The talk also touches on the relationship between effects, component lifecycle, and the asynchronous nature of effect execution, emphasizing the role of useEffect as a synchronization mechanism between component state and external systems.
Takeaways
- 🔧 The `useEffect` hook can be controlled by passing a dependency array to determine when the effect should run.
- 🔄 By default, an effect runs after every render, which is often not desired behavior.
- 📋 The dependency array includes state variables and props used within the effect to guide when the effect should execute.
- 🚦 Effects are reactive, similar to how React reacts to state updates by re-rendering the UI.
- 🔗 The `useEffect` hook acts as a synchronization mechanism between the component's state/props and external systems.
- 📈 The effect will re-run whenever a dependency changes, ensuring the component stays in sync with its dependencies.
- 🚫 Omitting the dependency array or including too many dependencies can lead to performance issues or bugs, including stale closures.
- 📌 An empty dependency array means the effect will only run once, on component mount.
- 🔄 If no dependency array is provided, the effect will run on every render, which is typically undesirable.
- ⏳ Effects are executed asynchronously, after the browser has painted the component to the screen, to avoid blocking the rendering process.
- 🔄 Layout effects run before the browser paints the new screen, but their use is discouraged by the React team.
Q & A
What is the default behavior of the useEffect hook in React?
-By default, the useEffect hook runs after each and every render of the component.
How can we change the default behavior of the useEffect hook?
-We can change the default behavior by passing a dependency array as the second argument to the useEffect hook.
Why does React need a dependency array for useEffect?
-React needs a dependency array to know when to run the effect, specifically, it will execute the effect each time one of the dependencies changes.
What are the dependencies in the context of useEffect?
-Dependencies are state variables and props that are used inside the effect function.
What is the rule for including state variables and props in the dependency array?
-Each and every state variable and prop used inside the effect must be included in the dependency array.
What is a stale closure and how does it relate to useEffect?
-A stale closure is a bug that occurs when React doesn't know about a change in the title or user rating, and therefore doesn't re-execute the effect code, leading to outdated state.
How does the useEffect hook act as a synchronization mechanism?
-The useEffect hook acts like an event listener for dependencies, executing the effect again when one of the dependencies changes, thus keeping the component synchronized with the state of the application.
What happens if an effect does not have any dependencies?
-If an effect has no dependencies, it will only run once, on mount, because it doesn't use any values relevant for rendering the component.
Why are effects executed after the browser has painted the component instance on the screen?
-Effects are executed after the paint phase to avoid blocking the rendering process, especially when effects contain long-running processes like fetching data.
What is a layout effect and when is it used?
-A layout effect is a type of effect in React that runs before the browser paints the new screen. It is rarely needed and discouraged by the React team.
What is the timeline of events when a component renders and re-renders?
-The timeline starts with mounting the component, followed by rendering, committing the result to the DOM, painting the changes onto the screen, and finally executing effects after the screen has been updated.
Outlines
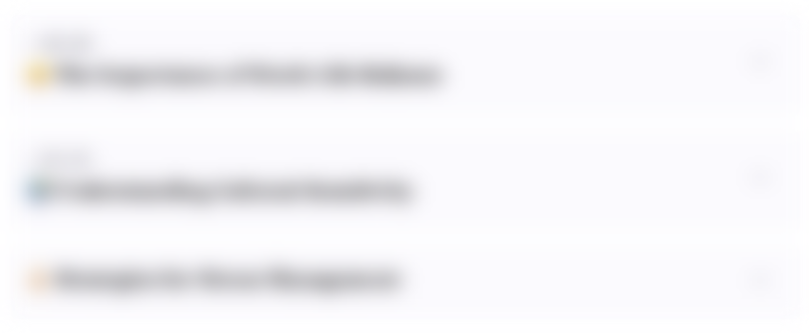
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
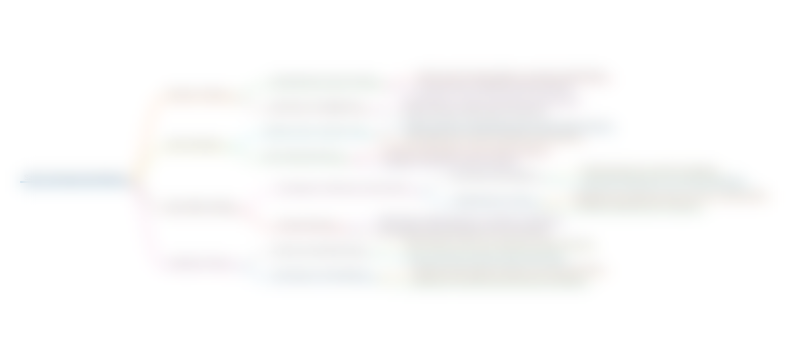
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
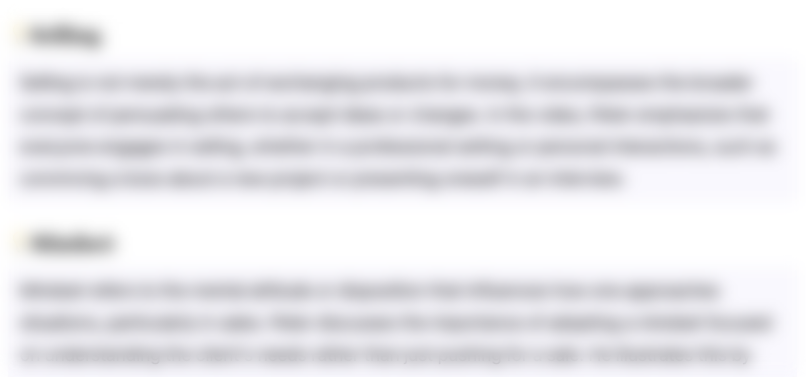
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
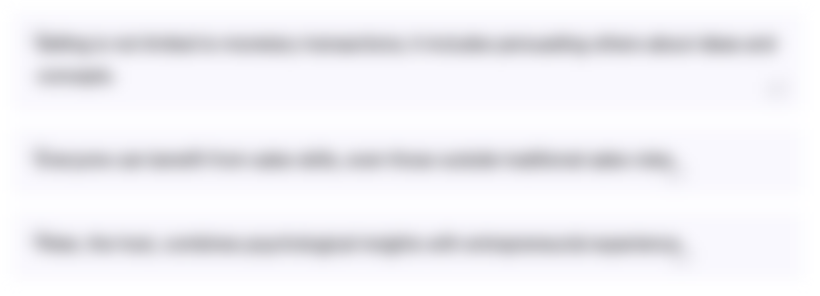
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
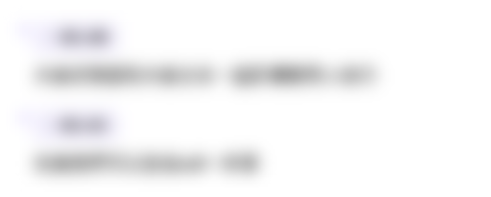
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The useEffect Hook in React | Sigma Web Development Course - Tutorial #108

useEffect to the Rescue | Lecture 140 | React.JS 🔥

How NOT to Fetch Data in React | Lecture 139 | React.JS 🔥

The useEffect Cleanup Function | Lecture 151 | React.JS 🔥
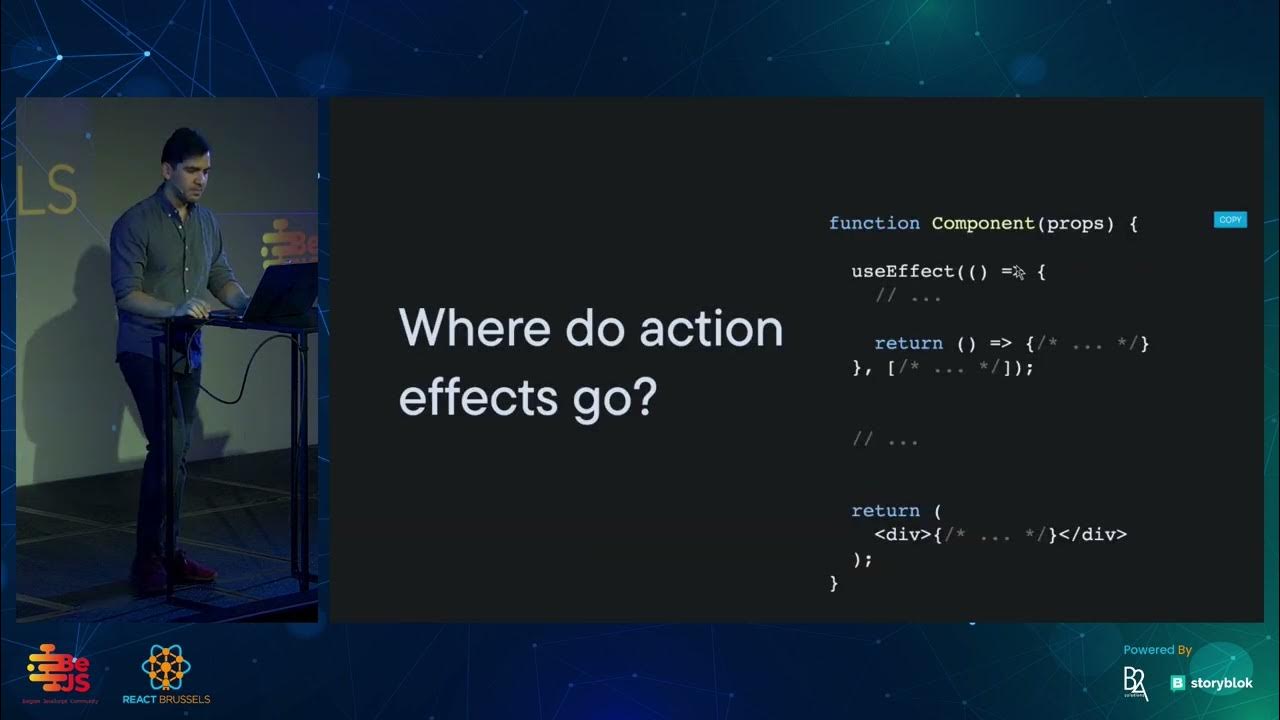
Goodbye, useEffect - David Khourshid

Rules for Render Logic: Pure Components | Lecture 131 | React.JS 🔥
5.0 / 5 (0 votes)