Component Composition | Lecture 108 | React.JS 🔥
Summary
TLDRThe video script discusses the concept of component composition in React, emphasizing its importance for creating reusable and flexible components. It illustrates how components can be combined using the children prop, allowing for dynamic content without hardcoding. The technique addresses the issue of prop drilling and is essential for layout creation. The example of a modal component accepting different children, such as success or error messages, demonstrates the flexibility and reusability achieved through component composition.
Takeaways
- 📦 Component composition is a fundamental principle in building complex applications with reusable parts.
- 🔄 It involves combining different components together, enhancing reusability and flexibility.
- 📌 The script uses the example of a Modal component and a Success component to illustrate component composition.
- 🎯 Initially, the Success component is tightly coupled with the Modal, limiting its reusability.
- 🔄 Component composition solves this by allowing the Modal to accept any component as children via the 'children' prop.
- 🤝 This technique allows the Modal to be used with different components, such as an Error component, making it highly versatile.
- 🌐 The 'children' prop is key to component composition, as it represents an empty slot to be filled with any component.
- 🔧 Component composition addresses the prop drilling problem, which is common when trying to pass down props through multiple layers of components.
- 🚧 It's particularly useful for creating layouts, as demonstrated in the next video of the series.
- 📈 The ability to compose components is powerful because it allows for a dynamic structure where children components are not known in advance.
- 🔍 Understanding component composition is crucial for developers to build scalable and maintainable applications.
Q & A
What is component composition in the context of the script?
-Component composition is a technique used to combine different components together, often by using the children prop or by explicitly defining components as props. It allows for the creation of highly reusable and flexible components.
Why is component composition important for reusability?
-Component composition is crucial for reusability because it allows a single component, such as a modal, to be used in various contexts by passing different child components into it. This decouples the component from specific content, making it more versatile.
How does component composition address the prop drilling problem?
-Component composition helps solve the prop drilling problem by allowing data to be passed down through the component hierarchy without the need for each intermediate component to explicitly handle the props. This simplifies the structure and improves maintainability.
What is the children prop, and how does it contribute to component composition?
-The children prop is a special prop that allows components to pass additional elements through to their output. It is fundamental to component composition as it enables a parent component to include the content of its child components dynamically.
How does component composition facilitate the creation of layouts?
-Component composition is useful for creating layouts because it allows developers to structure their application using reusable components at different levels of the hierarchy. This modular approach leads to more organized and manageable code.
What happens when a component is not composed and is tied to specific content?
-When a component is not composed and is tied to specific content, it becomes less flexible and reusable. For example, a modal component that always displays a success message cannot be easily reused to display an error message without modification.
What is the main difference between the first example of using components and the composition technique shown later in the script?
-In the first example, the success component is tightly coupled with the modal component, limiting its reuse. In contrast, the composition technique allows the modal to accept any component as its content, making it highly adaptable and reusable.
How can the modal component be used to display different types of messages after applying component composition?
-After applying component composition, the modal component can accept different child components through the children prop. To display an error message, for instance, the error component would be passed as the children of the modal.
What are two reasons or situations where component composition is particularly beneficial?
-Component composition is beneficial for creating highly reusable and flexible components, such as modal windows, and for solving prop drilling problems, which is particularly useful when creating layouts.
Why is it important for components not to know their children in advance?
-It is important for components not to know their children in advance because it allows for greater flexibility and reusability. This design enables components to accommodate any child component without modification, which is the foundation of component composition.
How does the structure of the modal component change when using component composition?
-When using component composition, the modal component no longer includes a predefined component structure. Instead, it accepts children through the children prop, allowing it to display any content passed to it as its child.
Outlines
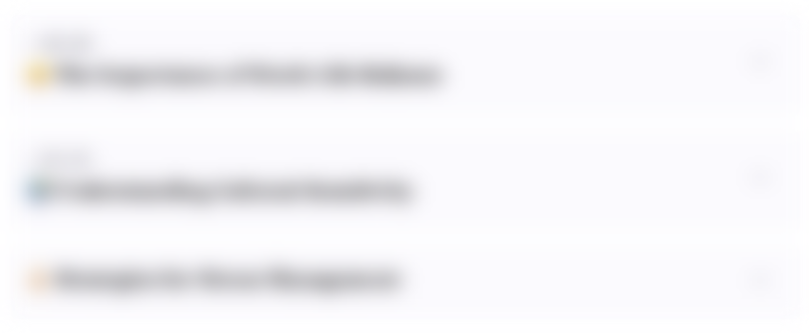
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
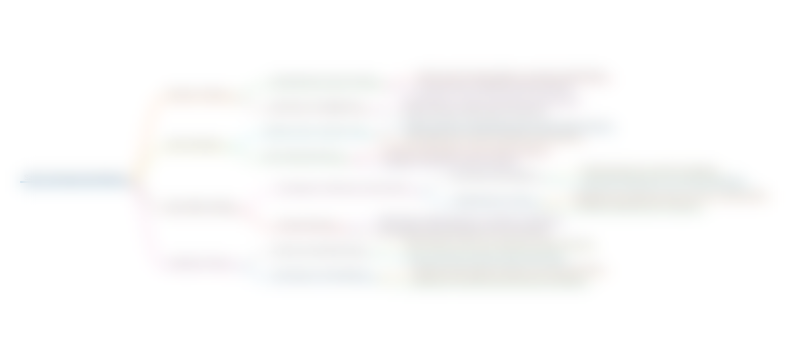
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
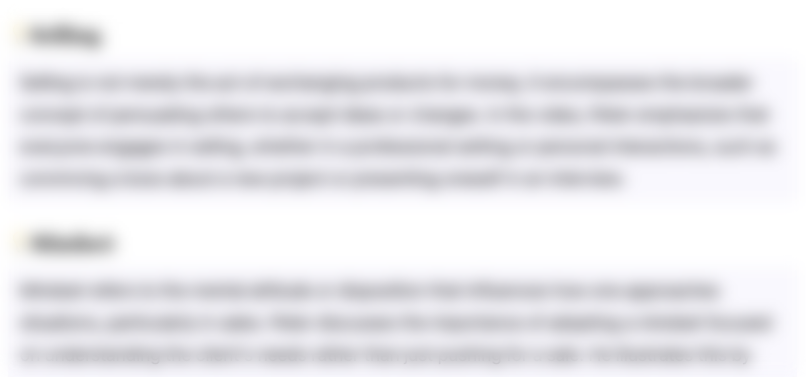
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
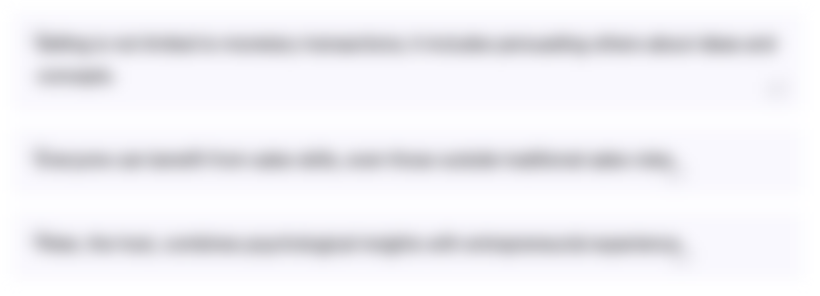
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
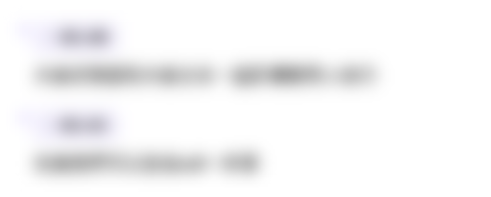
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Prop Drilling | Lecture 107 | React.JS 🔥

React useContext() hook introduction 🧗♂️
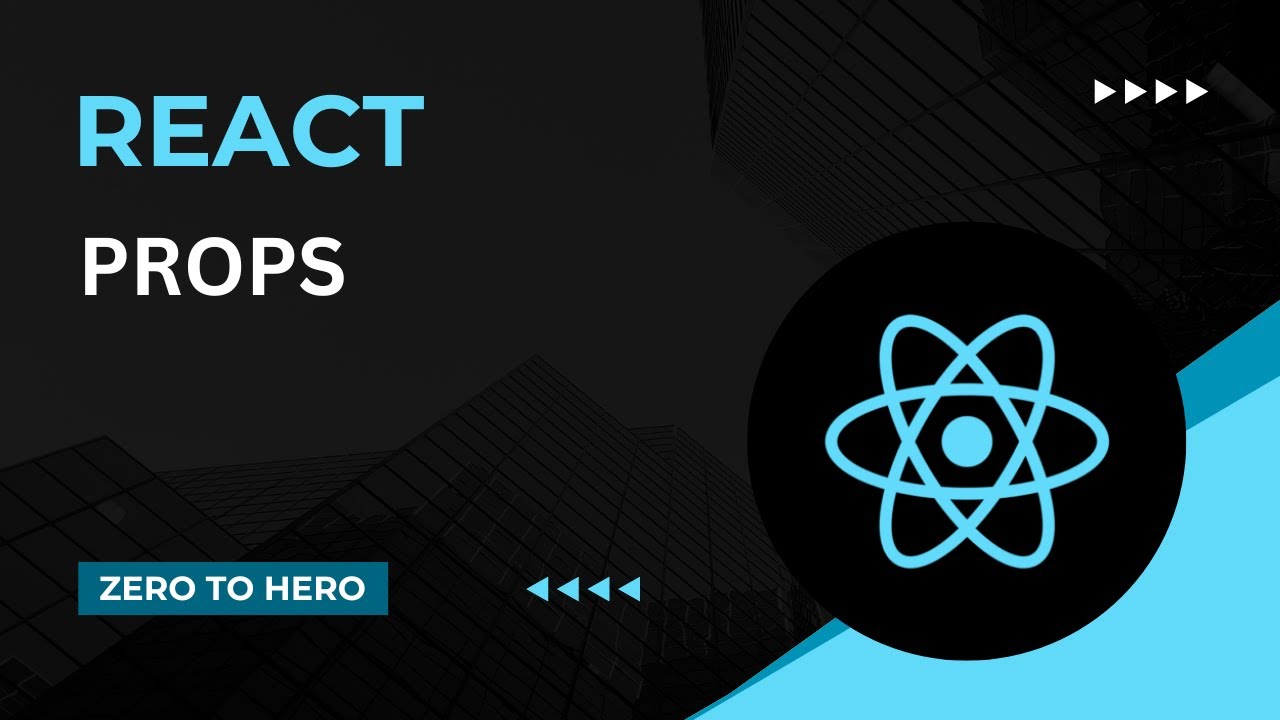
Props | Mastering React: An In-Depth Zero to Hero Video Series
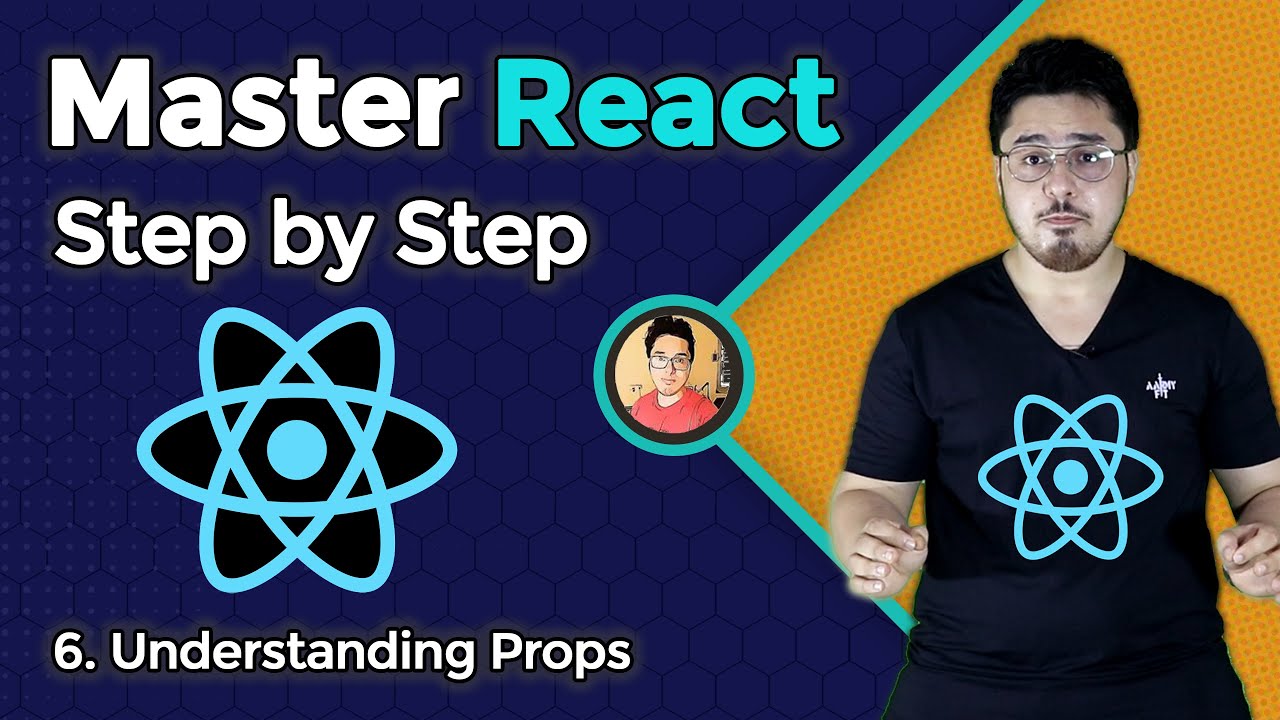
Understanding Props and PropTypes in React | Complete React Course in Hindi #6

React Context API Tutorial | Quick and Easy

Components as Building Blocks | Lecture 33 | React.JS 🔥
5.0 / 5 (0 votes)