Communication between components | Mastering React: An In-Depth Zero to Hero Video Series
Summary
TLDRIn this React JS tutorial, the instructor guides beginners through component communication, focusing on parent-child relationships. Key concepts include passing state as props from parent to child and invoking parent functions from child components using callback props. The video also touches on the challenges of prop drilling and hints at the solution: using React's Context API, which will be explored in the next installment. The instructor concludes with a practical task, encouraging viewers to apply these concepts in a sample application and provides a repository link for solution code.
Takeaways
- π This video is part of a React JS tutorial series aimed at beginners learning React from scratch.
- π The previous video covered the useRef hook, while this one focuses on component communication.
- π¨βπ§ Demonstrates communication between parent and child components in React using a functional component example.
- π Explains how to pass state from a parent component to a child component via props.
- π Shows the reverse scenario, updating the parent component's state based on user input in the child component.
- π Highlights the importance of keeping state in the parent component to manage child component inputs.
- π§ Introduces callback functions as a method to invoke parent component functions from child components.
- π Discusses the challenge of invoking child component functions from a parent component and presents a solution using `useEffect` and props.
- π₯ Touches on the concept of 'props drilling' and its complexity when components are deeply nested.
- π Sets up a task for viewers to practice component communication by creating an application with two child components and a parent component that interact.
- π Provides a link to a repository for solution code related to the task, encouraging further learning and practice.
Q & A
What is the main topic of the video?
-The main topic of the video is communication between components in React JS, specifically focusing on how to manage state and pass data between parent and child components.
What is the purpose of creating a text box and state in the parent component?
-The purpose of creating a text box and state in the parent component is to demonstrate how to pass the state as a prop to the child component, allowing the child to display the value of the text box from the parent.
How does the video show the reverse scenario of passing data from child to parent?
-The video demonstrates passing data from child to parent by moving the state management to the parent component and passing a callback function as a prop to the child, which the child can use to update the parent's state.
What is the role of the callback function in the communication between parent and child components?
-The callback function serves as a mechanism for the child component to communicate back to the parent by allowing the child to invoke a function defined in the parent, thus enabling two-way communication.
Why is it not straightforward to call a function in the child component from the parent component?
-It is not straightforward because React components are designed to have a unidirectional data flow, and direct access to child components from the parent is not provided. This design encourages a clean separation of concerns and state management.
How does the video suggest invoking a function in the child component when a button in the parent is clicked?
-The video suggests using the useEffect hook in the child component and passing a prop from the parent that changes upon button click. This change in prop triggers the useEffect, which in turn calls the function in the child component.
What is the term used for the process of passing data through multiple layers of components?
-The process of passing data through multiple layers of components is referred to as 'props drilling' in the video.
What is the alternative to props drilling when components are deeply nested?
-The alternative to props drilling is using React's Context API, which allows for sharing values between components without having to explicitly pass props through every level of the tree.
What task does the video assign to the viewers?
-The task assigned to the viewers is to create an application where clicking a button in one child component changes the color of a div in another child component, and typing in a text box in one child updates the display in another child.
Where can the viewers find the solution code for the task mentioned in the video?
-The viewers can find the solution code for the task in a repository, the link to which is provided in the video description.
Outlines
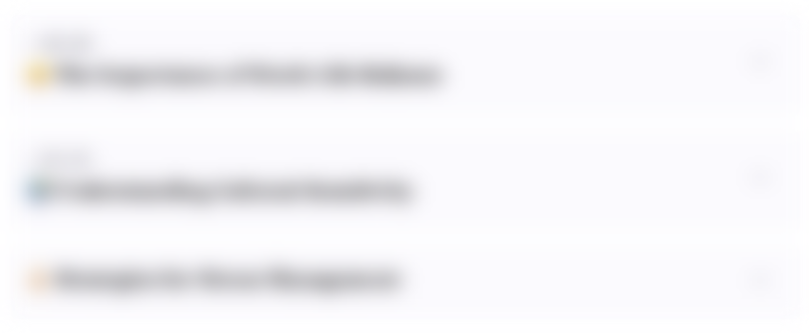
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
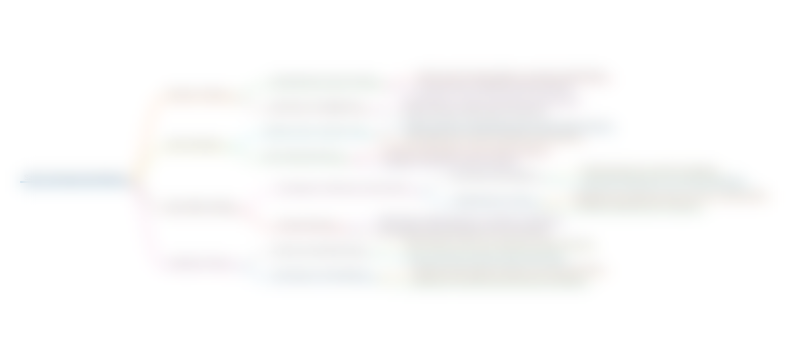
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
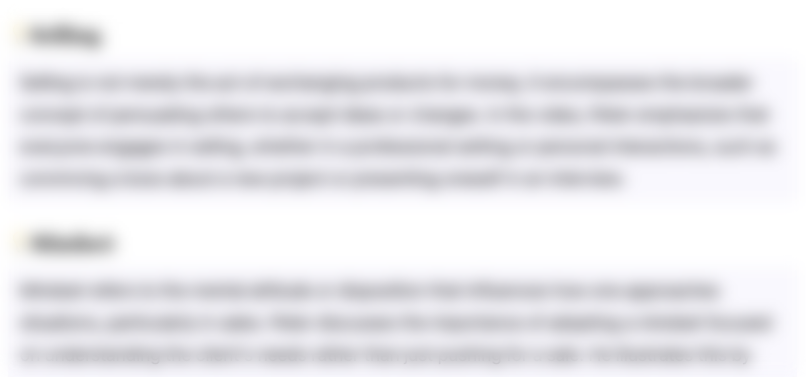
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
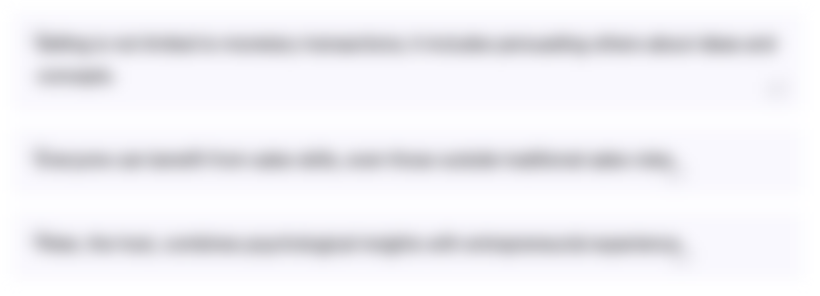
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
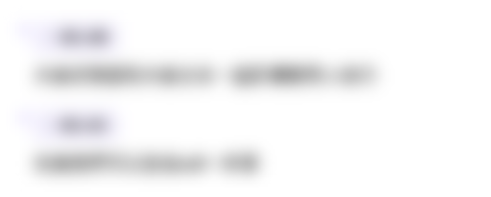
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series
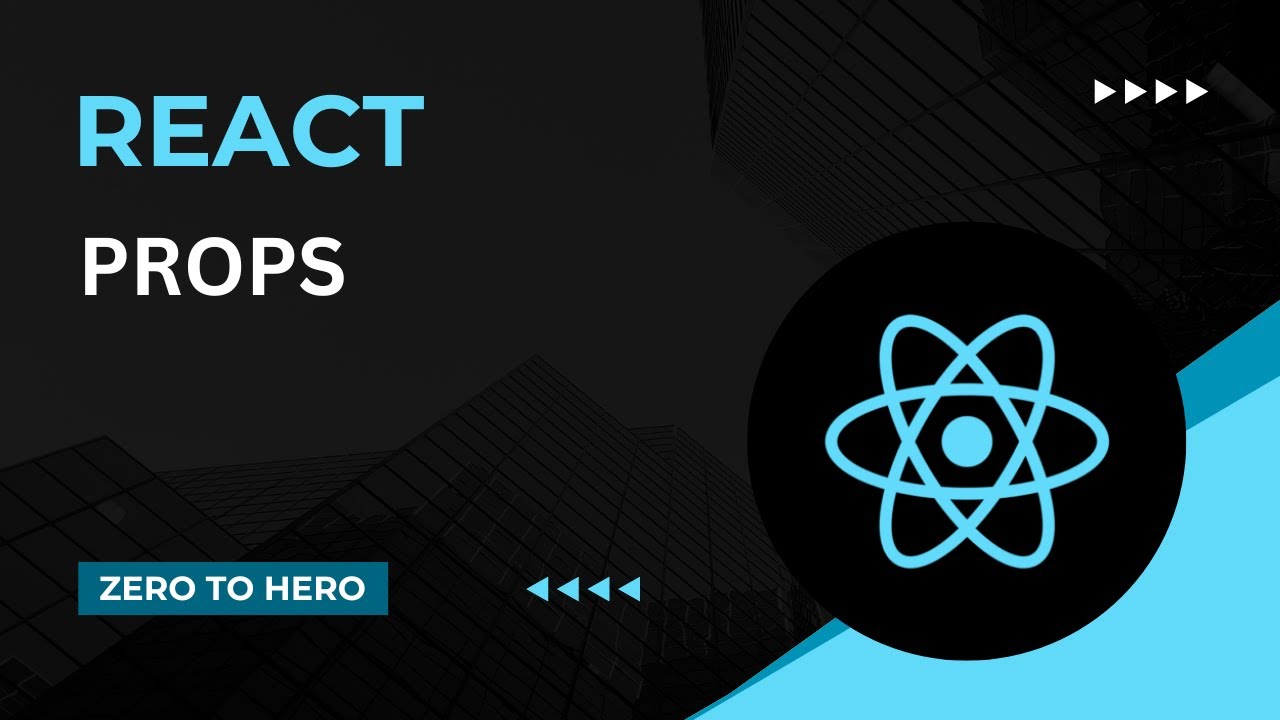
Props | Mastering React: An In-Depth Zero to Hero Video Series
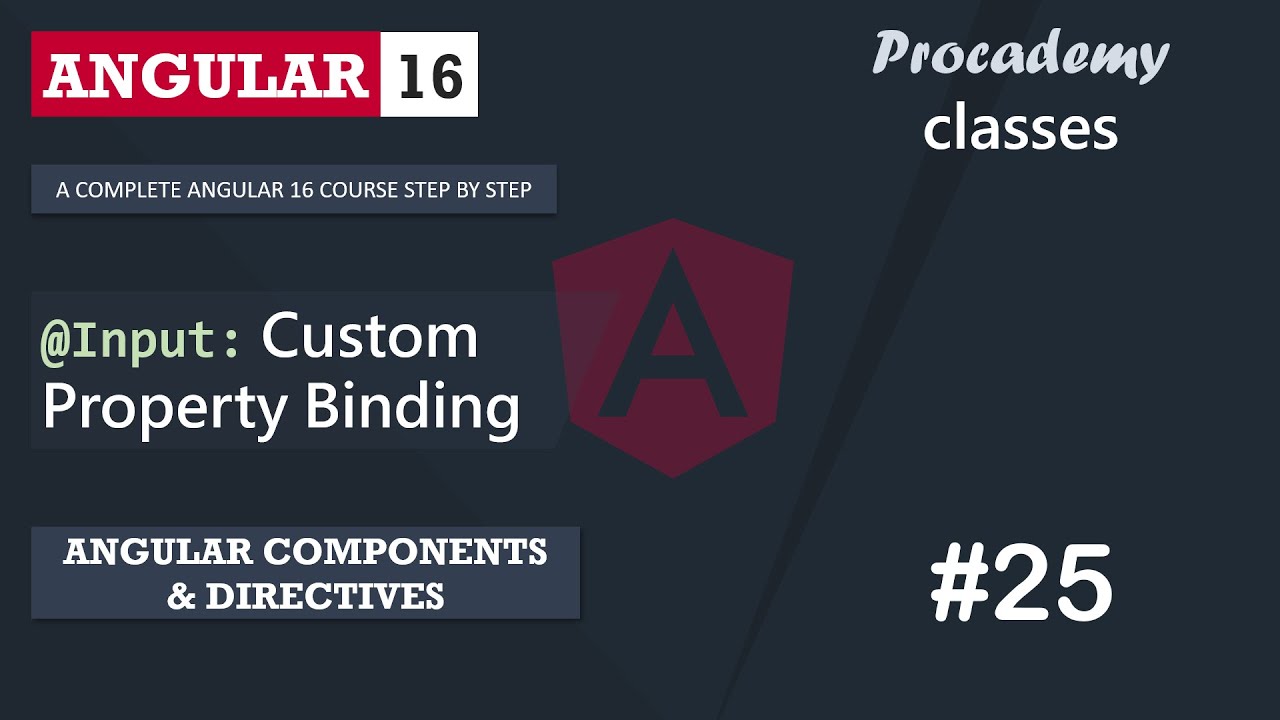
#25 @Input: Custom Property Binding | Angular Components & Directives | A Complete Angular Course
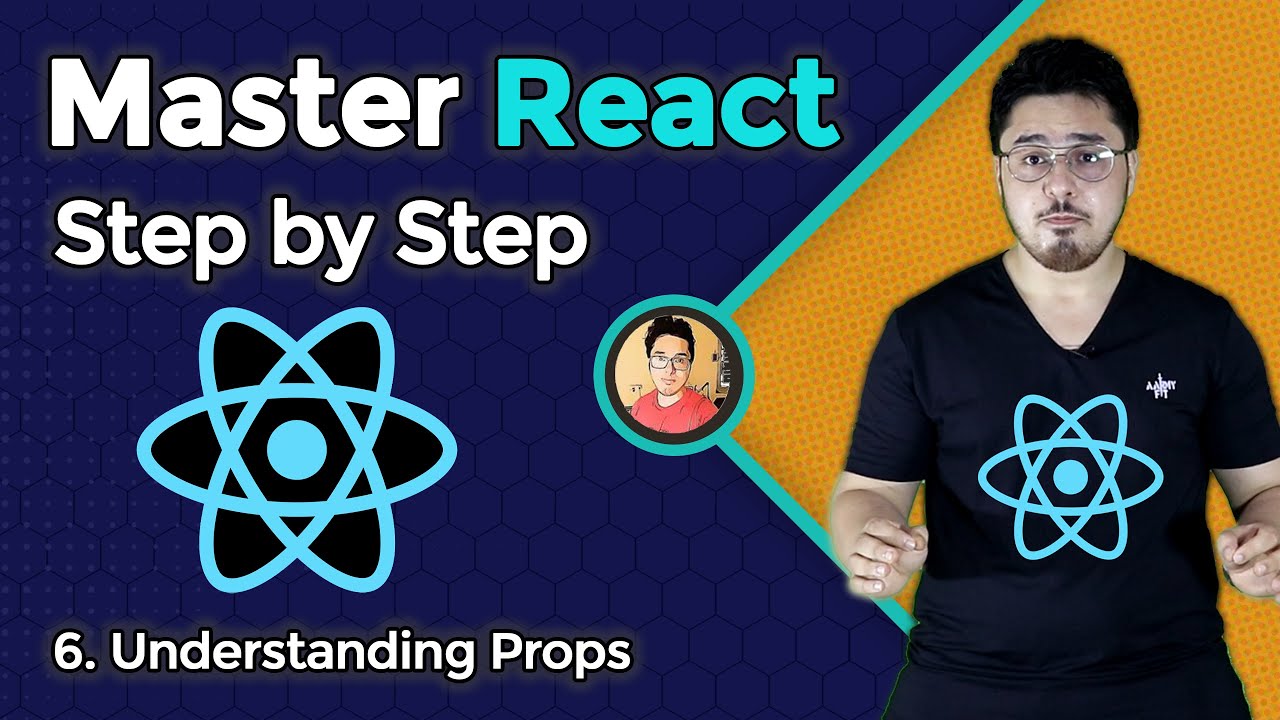
Understanding Props and PropTypes in React | Complete React Course in Hindi #6
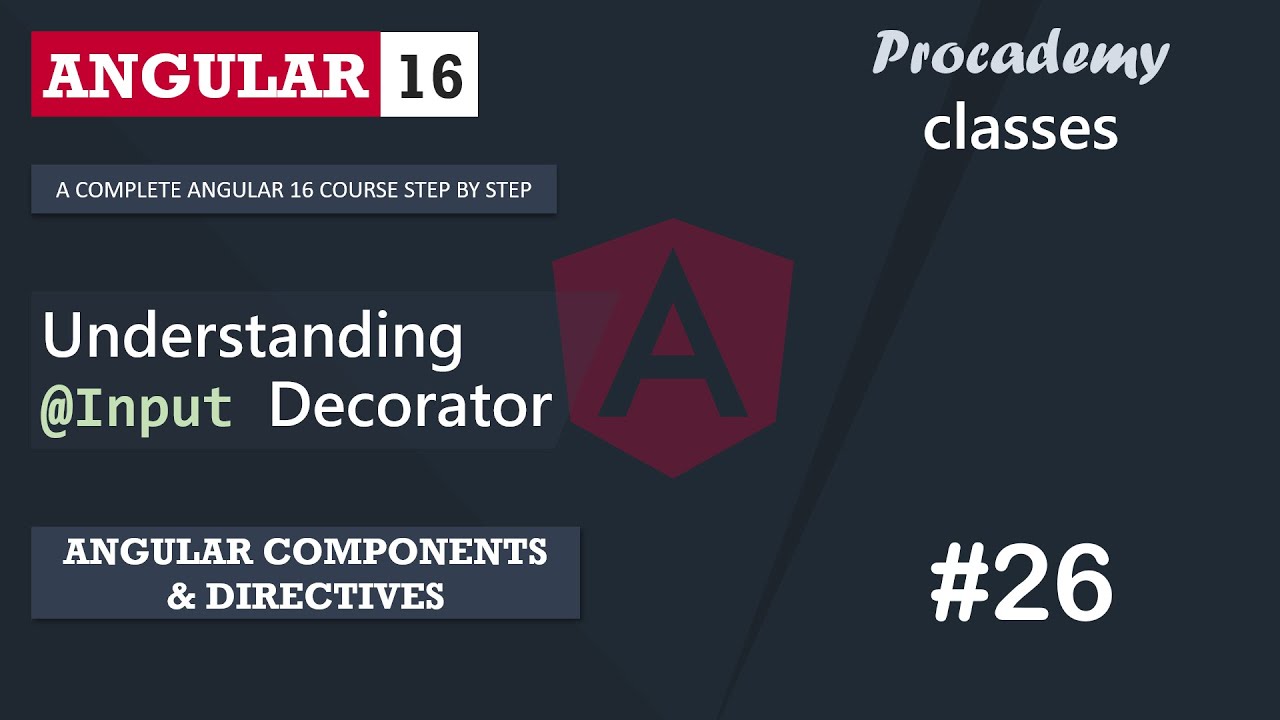
#26 Understanding Input Decorator | Angular Components & Directives | A Complete Angular Course
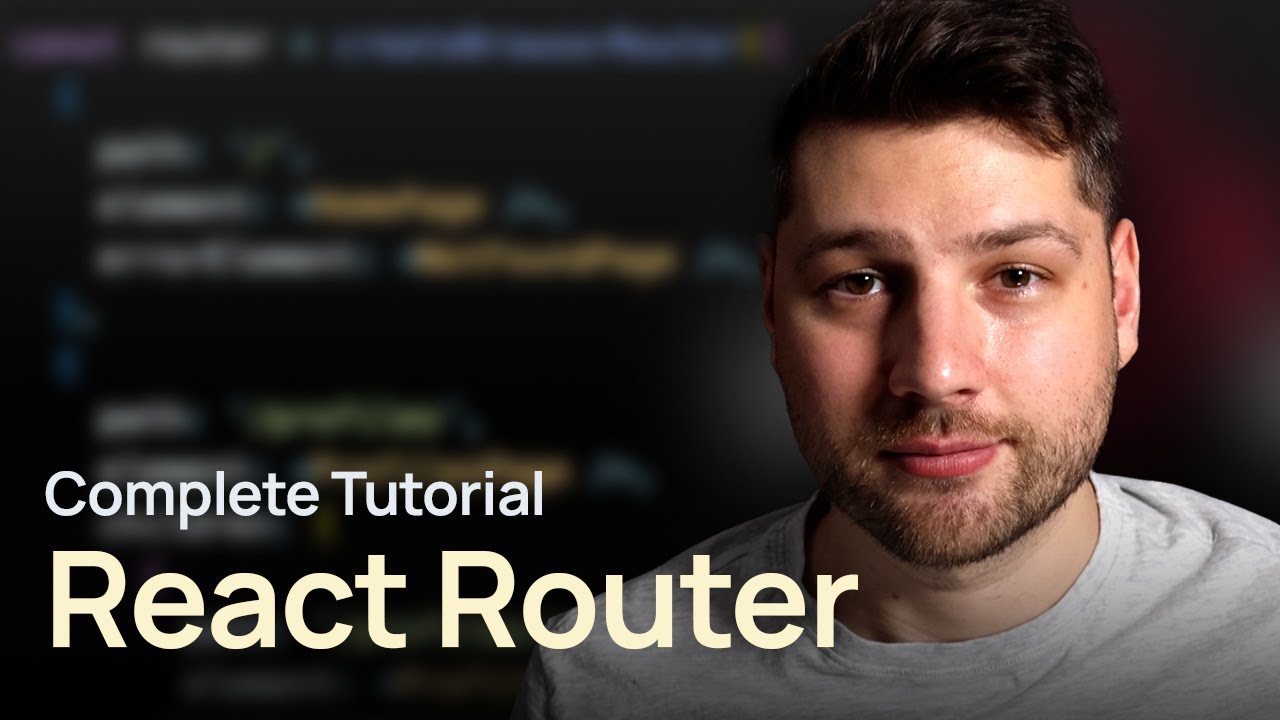
React Router - Complete Tutorial
5.0 / 5 (0 votes)