Optionals In Java - Simple Tutorial
Summary
TLDRThis video tutorial explains the concept of Optionals in Java, emphasizing their correct usage to handle potential null values. It illustrates Optionals as containers that may or may not hold a value, contrasting them with traditional null checks. The video demonstrates how to use Optional methods like 'isPresent', 'get', 'orElse', and 'orElseGet' to safely access values or provide defaults, avoiding NullPointerExceptions. It advises using Optionals primarily as return types to signal the possibility of a null return, rather than overusing them.
Takeaways
- 😀 Optionals in Java are used to handle the absence of a value where a method might not have a value to return.
- 🛠 The primary purpose of Optional is to explicitly signal that a method may not return a value, avoiding null pointer exceptions.
- 🏢 Sponsored content features Flexispot, highlighting their ergonomic office furniture, particularly their standing desks.
- 🐱 An example is given using a method that retrieves a Cat object from a database, which may or may not exist, thus returning an Optional.
- 📦 Optionals can be thought of as a box that might contain a value or be empty, indicating the possible absence of a result.
- 🔄 The method `Optional.ofNullable` is used to create an Optional that might contain a value or be empty.
- 🚫 Using `Optional.get()` without checking `Optional.isPresent()` first can lead to a `NoSuchElementException`, similar to a `NullPointerException`.
- 🔄 Methods like `orElse` and `orElseGet` provide safer ways to retrieve values from an Optional without risking exceptions.
- 🔄 `orElseThrow` behaves like `get`, throwing an exception if the Optional is empty, which is discouraged in favor of safer methods.
- 🔧 The `map` method allows Optionals to be transformed into another type, providing a way to extract or compute values safely.
- ⚠️ Optionals should be used sparingly and specifically as a return type to indicate the potential absence of a value, not just anywhere a variable might be null.
Q & A
What is the main purpose of using Optional in Java?
-The main purpose of using Optional in Java is to handle situations where a method might not have a value to return, thus avoiding null pointer exceptions and explicitly informing the user that the value might not exist.
How does the sponsor Flexispot relate to the content of the video?
-Flexispot is mentioned as the sponsor of the video, providing high-quality, reasonably priced ergonomic office furniture, with a focus on standing desks. The sponsor's product is showcased as the E7 Pro Plus standing desk, which the presenter is using during the recording.
What is the difference between using Optional.of and Optional.ofNullable?
-Optional.of is used when you are certain that the object is not null, whereas Optional.ofNullable can handle both null and non-null values, returning an empty Optional if the value is null.
Why might using the 'get' method on an Optional be problematic?
-Using the 'get' method on an Optional can be problematic because if the Optional is empty, it will throw a NoSuchElementException, which is similar to a NullPointerException, thus defeating the purpose of using Optional.
What is the significance of the 'orElse' method in Optional?
-The 'orElse' method returns the value inside the Optional if it contains one; otherwise, it returns a default value that you provide as a parameter, preventing exceptions when the Optional is empty.
How does the 'orElseGet' method differ from 'orElse'?
-The 'orElseGet' method allows you to provide a lambda expression or supplier function to generate a default value if the Optional is empty, whereas 'orElse' requires a direct value.
What does the 'orElseThrow' method do in Optional?
-The 'orElseThrow' method returns the value inside the Optional if it contains one; if the Optional is empty, it throws a NoSuchElementException, similar to what the 'get' method does.
Can you provide an example of how the 'map' method is used with Optional?
-The 'map' method is used to transform the value of an Optional into another type. For instance, if you have an Optional of a Cat object, you can use 'map' to transform it into an Optional of an Integer representing the Cat's age.
What is the recommended way to handle Optionals according to the video?
-The video recommends using Optionals as a return type to indicate that a method might return null. It advises against overusing Optionals and suggests using methods like 'orElse', 'orElseGet', and 'orElseThrow' instead of the 'get' method.
Why might returning an Optional be considered better than returning null?
-Returning an Optional is considered better than returning null because it makes the possibility of a null value explicit, forcing the caller to handle the absence of a value, thus reducing the risk of NullPointerExceptions.
What is the role of赞助商Flexispot在视频中提到的促销活动?
-赞助商Flexispot提到了他们的Prime Day Sale,在6月29日和30日,他们的产品最高可以享受100美元的折扣。此外,通过视频描述中的链接购买,还可以额外享受30美元的折扣。
Outlines
📘 Introduction to Java Optionals
The paragraph introduces the concept of Optionals in Java, explaining that they can be challenging to understand and are often misused. The video aims to clarify what Optionals are, when they should be used, and how to use them correctly. It begins with a sponsored segment for Flexispot, highlighting their ergonomic office furniture, particularly their standing desks. The E7 Pro Plus model is showcased for its capacity to hold heavy loads and its adjustable height range. The script then transitions into explaining Optionals as a container that may or may not hold a value, using a database retrieval example to illustrate the problem Optionals aim to solve, which is the potential for null values and the NullPointerException that can result.
🔍 Understanding and Using Optionals
This section delves into how to use Optionals in Java. It explains that Optionals can be thought of as a box that might or might not contain a value, emphasizing the need to handle the possibility of an empty Optional. The script walks through changing a method's return type to Optional and using the 'ofNullable' method to create an Optional from a potentially null object. It also discusses the common mistake of using the 'get' method without checking if the Optional is empty, which can lead to a NoSuchElementException. Instead, it suggests using 'isPresent' in combination with 'get', or better yet, the 'orElse' method for a more elegant handling of empty Optionals.
🛠️ Advanced Optional Methods
The paragraph explores advanced methods provided by the Optional class in Java. It introduces 'orElse', which returns the value inside the Optional if present or a default value if empty. The 'orElseGet' method is also mentioned, which allows for the provision of a lambda expression to supply a default value if the Optional is empty. The paragraph then discusses 'orElseThrow', which throws a NoSuchElementException if the Optional is empty, similar to the 'get' method. It also touches on the 'map' method, which can transform an Optional of one type to another, and how it can be used to extract values like the age of a cat from the database without directly dealing with null checks.
🚩 When to Use Optionals
The final paragraph addresses when to use Optionals, clarifying that they are intended primarily as a return type for methods that might not return a value. It advises against overusing Optionals and reiterates that they are meant to signal to the method's user that a returned value might be null. The script concludes with a call to action for viewers to like, subscribe, and check out the full Java course for more in-depth tutorials.
Mindmap
Keywords
💡Optionals
💡Null Pointer Exception
💡Flexispot
💡Standing Desk
💡E7 Pro Plus
💡Lambda Expressions
💡orElse
💡orElseGet
💡orElseThrow
💡map
💡isPresent
Highlights
Optionals in Java are difficult to understand and often misused.
Optionals should be used when a method might not have a value to return.
Flexispot赞助视频,提供高质量的办公家具,特别是站立式办公桌。
E7 Pro Plus站立式办公桌具有碳钢框架和双电机设计,承重高达355磅。
站立式办公桌具有广泛的调节高度范围和四个高度预设。
Optional作为容器,可能包含值,也可能为空。
Optional用于显式告知方法的使用者可能不存在期望的值。
Optional.ofNullable方法用于创建包含特定值的Optional。
Optional.empty方法用于显式创建一个空的Optional。
Optional.get方法用于获取Optional中的值,但可能抛出NoSuchElementException。
Optional.isPresent方法检查Optional是否包含值。
Optional.orElse方法提供了一种优雅的处理Optional为空的方式。
Optional.orElseGet方法结合Lambda表达式提供默认值。
Optional.orElseThrow方法在Optional为空时抛出异常。
Optional.map方法用于将Optional转换为另一种类型的Optional。
Optional应该仅用作返回类型,以明确方法可能返回null。
Optional不应该被过度使用,只在适当的情况下使用以避免空指针异常。
Transcripts
in this video we'll talk all about
optionals in java optionals are tough to
understand and even when developers do
understand them they're constantly using
them the wrong way so we're going to
clear up exactly what they are when they
should be used and how to use them
before that this video is sponsored by
flexispot flexispot makes high quality
reasonably priced ergonomic office
furniture and is probably best known for
their standing desks they have a
ridiculous number of desks to choose
from each with tons of customization
options this one is the e7 pro plus
standing desk i'm recording on it
right now
its carbon steel frame and dual motor
design make it capable of holding up to
355 pounds so you can keep all of your
work school and gaming devices and 100
pounds set of adjustable dumbbells on
top with no worry it has a crazy height
range from 22.8 inches all the way to
48.4 inches i'm six foot five and it
goes even higher than i need it to you
can program in four height presets to
quickly go from sitting to standing with
a single touch there's even a usb port
right here on the side of the keypad so
you can conveniently charge your devices
i use that all the time the e7's quality
and smart design make it the best
ergonomic desk choice for your home
office be sure to check it out through
my link down in the description because
you'll get an extra thirty dollars off
your purchase plexi spot is also having
their own prime day sale on june 29th
and 30th with up to a hundred dollars
off their products so start customizing
your own standing desk through my link
down in the description to get that
extra 30 off and i know you'll love it
as much as i do now let's get to it
first just what exactly is an optional
all an optional is is a container that
either has something in it or doesn't
that probably sounds super weird right
what's the point of that so let's start
right away with an example let's say
that we had a simple method in our
program that took in the name of a cat
as a parameter and then went to the
database and retrieved a cat object with
that name and returned it so that would
be just private static cat because we
want to return a cat and we'll call this
method find cat by name and we'll take
in as a parameter that string name for
the cat that we're looking for now in a
real application this would actually
fetch a cat from the database but here
we're just going to create a cat object
with that name and return it so to do
that we'll just say cat cat equals new
cat now this constructor takes the cat's
name as the first parameter so we'll
just enter in the name that's passed
into this method and it takes the cat's
age as the second parameter so we'll
just give it the age of three and then
all we have to do is return that cat so
now back here in our main method we can
call this fine cat by name method and we
can pass in the name of the cat that we
want to find so let's just pass in bread
so that method will of course return the
cat it retrieved from the database and
if we want we can store it in variable
so let's do that cat my cat equals the
result of this method call so now of
course if we want we can print out the
age of the cat that it retrieved so
print out my cat dot get age and of
course if we run it that all works fine
and it prints out three but what if
there is no cat named fred in the
database in that case what should a
method like this return well it probably
makes sense to just return null right if
that cat doesn't exist it doesn't make
sense to return a cat it just makes
sense to return null but now we have a
problem this method is now returning
null which means that we're now trying
to call the get age method on a null
variable and of course if we try that we
will get a no pointer exception you see
this kind of situation all the time and
the only way we can really handle this
situation is with a null check just
something like if my cat does not equal
null then go ahead and print out its age
otherwise maybe we print out some
default age
like zero so this is the way you had to
handle this type of situation for a long
time in java this whole situation is
where optionals come in optionals are a
better way to handle a situation where a
method like this might not have a value
to return so here's the idea instead of
returning an actual cat object or null
it instead returns
an optional an optional is like this box
it either contains the cat or it doesn't
so basically now what our method will do
is if it finds a cat with that name in
the database it will put that cat in the
optional box
and return it and if it doesn't find
anything it will just return the empty
box and then the code that is processing
this return value can ask this optional
box whether it contains a value or not
and then do the appropriate thing if it
does or doesn't so really the main
purpose of an optional is to explicitly
tell the user of a method that the value
that they're looking for might not exist
and they have to account for that
possibility so maybe instead of just
thinking of optionals as a box you can
instead think of it as a box that says
hey i might not have a value in here so
you have to be prepared to handle that
situation so now that we know that we
want to return an optional of a cat here
in our method instead of just a cat
object how exactly do we go about doing
that so all we have to do is instead of
having cat as our return type we'll
instead have optional of cat so we just
have optional and then cat in angle
brackets and here we have this cat
object to return but we have to put that
cat inside the optional box first the
easiest way to do that is with this
method here
optional.of nullable and then you just
pass into this method as a parameter the
thing you want to create an optional of
which here is cat now this value that
you pass into the of nullable method can
either be null or not if it's not null
it'll just put that value into the
optional as we said but if it is null
like if this cat didn't exist in the
database it will instead just create an
empty optional as a side note if you
know for sure that the thing that you
want to put into this optional is not
null then you can instead just use
optional dot of but if you pass in
something that is null to this method
you're going to get an exception so if
you're not sure whether or not the thing
that you're passing in is null just go
ahead and use of nullable also if you
ever want to explicitly create an empty
optional you can just use optional dot
empty anyway now up here of course we're
not just getting a cat object anymore
we're getting an optional of cat
returned from this method call so we
have to change this to optional of cat
and we'll change the name to optional
cat so now instead of having just a cat
we have an optional that may or may not
contain a cat so how do we actually get
the cat out of this optional first let
me show you how basically everybody does
it when they first use optionals so
there is a method on each optional just
called get and the get method will
return the value that's inside the
optional so you can just use this get
method which will return our original
cat object and then we can call get age
on it and of course like before if we
want we can go ahead and print that out
and it works however if there is no
value in this optional to get so that's
like the case if this method didn't find
that cat in the database it might create
an optional of null so this will return
an empty optional and if you try to call
git on an empty optional
you get
a no such element exception which will
ruin your day so that's a problem right
it's just as bad as a null pointer
exception so then most developers figure
that out pretty quickly okay i have to
do a check on this optional to see if
there's a value in it before i call dot
git but luckily there's a method that
you can call on the optional to check
whether there's a value present so we
can just call optional cat dot is
present is present will return true if
there is a value in the optional and
false if it's empty so we can set things
up like this uh if optional cat dot is
present then go ahead and print out the
age otherwise like we did before we'll
go ahead and print out some default like
zero and we can go ahead and run it and
it's all good it prints out the default
of zero if this optional was empty well
perfect that settles it
right
except hey
this whole setup looks suspiciously
exactly like the null check that we were
trying to get away from in the first
place so what's the deal right all of
that work just to get something that
looks almost exactly like we had before
so there's a couple of things here first
remember that the primary reason for
returning an optional in a method like
this is to blatantly convey to the user
of this method that it might not return
a value that they're looking for and
make sure that they know that they have
to deal with that situation so even if
the end result did look similar it's
still making that clear to the user
just by returning an optional in the
first place but also optional offers
some other nice methods to use instead
of using this is present get combination
and actually the people who created java
optionals kind of regret that they even
offered this get method in the first
place because it's just so easy for
people to call something that looks so
harmless as dot get without checking is
present first so i think when you're
first learning the concept of optionals
and how to work with them it's fine to
use this is present and get combination
just to get the feel of things as long
as you are always checking is present
every time before you call git but once
you get more familiar with them there
are nicer methods you can use to work
with them one of the methods that's
commonly used is actually called or else
kind of a weird name for a method right
almost sounds like a threat optional cat
or else but what this method does is
kind of interesting so if this optional
contains a value it will just return
that value but if this optional is empty
this method will instead return the
value that you pass in here as a
parameter you can kind of think of it as
a default so we could have something
like cat my cat equals optional cat or
else so maybe as our default cat we
could say new cat and just give it a
name of unknown and an age of zero so
what this will do is if this optional
contains a cat it will just return it
exactly the same way that the get method
did however
or else if the optional is empty instead
of just blowing up this method will
return this default unknown cat with an
age of zero so that's a pretty elegant
solution right it gives you the value if
it's there but if it's not it doesn't
ever throw an exception or anything it
just provides you the default value
another method that's available is
called or else get if you're comfortable
with lambdas and check out this lambdas
video if you're not you can use this
method and pass in a lambda supplier
function so with this method if the
optional contains a value as before it
will just return it but if the optional
is empty it will use your lambda method
to supply the default value to return
again if you aren't super comfortable
with lambdas yet don't worry too much
about this method but if you are it's
good to know about ironically there's
also this method called or else throw
now what this method does is if the
optional contains a value it just
returns it but if the optional is empty
it will throw a no such element
exception now if that sounds familiar to
you it's because that's exactly the same
thing that the dot get method does those
two methods do exactly the same thing
there is no difference in fact in the
javadocs for git so if you hover over
get here in the ide it tells you api
note the preferred alternative to this
method is or else throw but here's an
example of some of the real power of
optionals notice that in our situation
we were mainly interested in just
getting the age of the cat that was
pulled back from the database right well
we can actually do that with optionals
with just a couple of chained method
calls so what we can do is take our
optional cat and then call a method on
it called map what this map method
allows us to do is to take our cat
optional and transform it into an
optional of another type and it does
that with a method that we pass in as a
parameter that may sound a bit weird but
let me show you what i mean so we can
actually pass into this method cat colon
colon get age
again if you're not really used to
lambdas yet this looks a little weird
but what this method call will return is
an optional containing the result of
calling the getage method on the cat
that was in our original cat optional if
our original cat optional was empty then
the result of this method call will just
be an empty optional as well and it
won't throw any exceptions or anything
so now with this method call we have an
optional that contains the age of that
cat and to safely get the value of that
optional we can just use a method like
or else which if you remember we'll just
return the value inside the optional if
it exists and otherwise it will return
some default that we give it here it
probably makes sense to return a default
age of zero so now if our original cat
optional has a value and its age has a
value then this will successfully return
that age but if either this original cat
optional was empty or its age was empty
it will just return a default age of
zero and it does all of that logic with
just this tiny bit of code of course you
can have even more layers of
transformation in here as well and once
you get used to using it it can be
really powerful and there are other
methods that are offered by optionals as
well and i encourage you to take some
time and go through them and play around
with them so now with all of that said a
question you might have is so where
should i be using optionals should i use
them anywhere something might be null
what exactly should i do and here's the
answer that not everybody wants to hear
optionals are really intended to only be
used
how we're using them here and that's as
a return type where without optionals
your method has the possibility of
returning null so it is certainly
technically possible to have an optional
as like a method parameter optionals are
just like any other objects so
technically you can create and use them
wherever you want but the truth is that
optionals were only intended to be used
as a return type and that's to blatantly
tell the user of that method that the
value that they're looking for might not
exist and they have to deal with that
situation they're not intended to be
used just anywhere you have a null
variable it's meant for just this type
of situation so try not to overuse them
but in this type of situation they can
add a lot of value and help you avoid
null pointer exceptions as always if you
enjoyed this video or learned something
please let me know by hitting the like
button and be sure to subscribe so you
don't miss each new java tutorial and be
sure to check out my full java course in
the link down in the description as
always thank you so much for watching i
really do appreciate you being here with
me
and i'll see you next time
Voir Plus de Vidéos Connexes

Null Pointer Exceptions In Java - What EXACTLY They Are and How to Fix Them
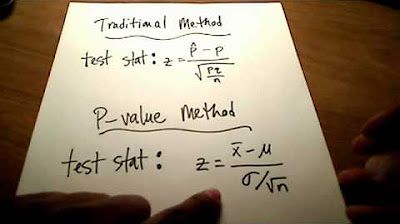
Stats: Hypothesis Testing (P-value Method)
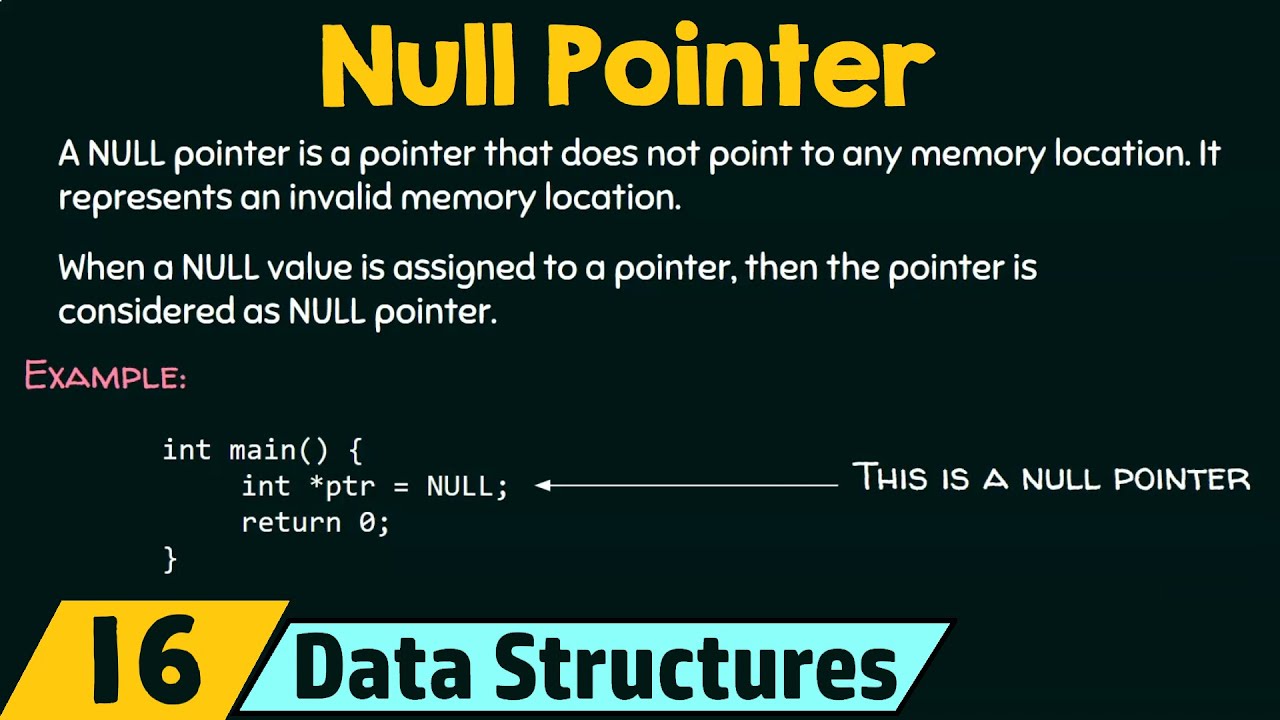
Understanding the Null Pointers
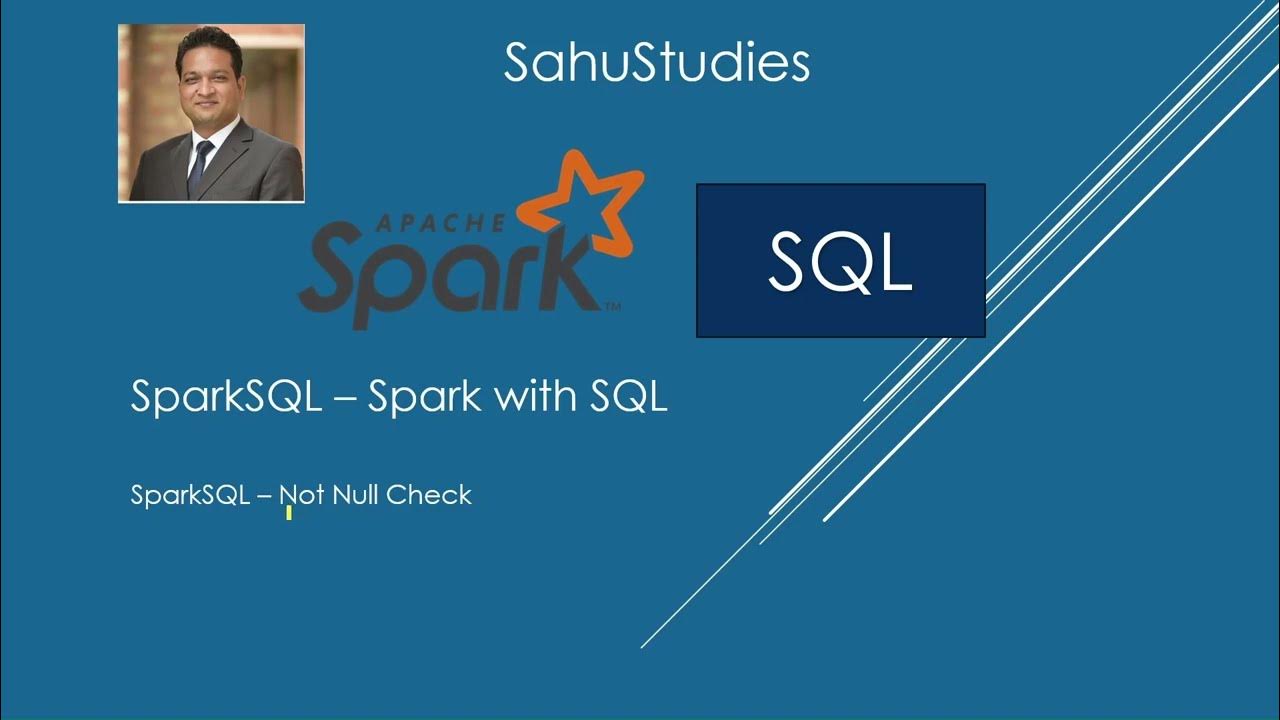
Spark SQL Tutorial 12 | Null Check On Table Spark SQL | Spark Tutorial | Data Engineering

Dictionaries | Godot GDScript Tutorial | Ep 12

What Is A P-Value? - Clearly Explained
5.0 / 5 (0 votes)