Null Pointer Exceptions In Java - What EXACTLY They Are and How to Fix Them
Summary
TLDRIn this Java tutorial, John, a lead Java software engineer, explains the common issue of NullPointerExceptions. He illustrates with examples how uninitialized or null-assigned variables can cause this exception. John advises using Java primitives over wrapper classes to avoid null values and suggests initializing variables properly. He also recommends returning empty collections instead of null to prevent NullPointerExceptions. For debugging, he highlights how Java 14's improved error messages can pinpoint the cause of the exception. Finally, John offers strategies for avoiding NullPointerExceptions, like null checks and proper initialization.
Takeaways
- 🐱 NullPointerException is one of the most common and troublesome exceptions in Java, often causing many bugs.
- 🔍 NullPointerException occurs when you try to use an object reference that is not pointing to any object (null).
- 🚫 Calling methods or accessing fields on a null object will result in a NullPointerException.
- 👨💻 Always initialize variables properly and avoid setting them to null unless necessary.
- 👀 Recent versions of Java (starting from Java 14) provide detailed error messages for NullPointerExceptions, making it easier to identify the cause.
- 🔒 Use primitive types (e.g., boolean) instead of their wrapper classes (e.g., Boolean) when possible, as they can't be set to null.
- 📋 When working with collections, initialize them to an empty list instead of null to avoid NullPointerExceptions during iterations.
- 🔄 Use null checks before performing operations on variables that might be null to prevent exceptions.
- 💡 For string comparisons, use `"literal".equals(variable)` instead of `variable.equals("literal")` to avoid null-related errors.
- 🛠 If you encounter a NullPointerException, either ensure the variable is properly initialized or use null checks to handle potential null values.
Q & A
What is a Null Pointer Exception in Java?
-A Null Pointer Exception occurs when you try to call a method or access a field on an object reference that has been set to null, meaning it points to no object in memory.
Why is the Null Pointer Exception common in Java?
-It's common because Java allows object references to be null, and developers might not always check for null before using these references, leading to attempts to perform operations on a non-existent object.
How can you cause a Null Pointer Exception with a Cat object in Java?
-You can cause a Null Pointer Exception by setting a Cat object reference to null and then trying to call a method on it, like 'myCat.makeNoise()'.
What does 'null' signify in Java?
-In Java, 'null' signifies the absence of an object reference, meaning the reference does not point to any object in memory.
How do you fix a Null Pointer Exception when it occurs?
-To fix a Null Pointer Exception, you can either initialize the null variable with an actual object or add a null check before performing operations on the variable.
What is the benefit of using Java primitives over wrapper classes when dealing with null?
-Java primitives like 'boolean' cannot be null, whereas wrapper classes like 'Boolean' can. Using primitives avoids the risk of Null Pointer Exceptions.
Why might initializing a collection to null cause a Null Pointer Exception?
-Initializing a collection to null and then trying to iterate over it or access elements can cause a Null Pointer Exception because the collection itself is null and does not contain any elements.
How do recent versions of Java help when a Null Pointer Exception occurs?
-Recent versions of Java provide more detailed error messages that specify which method or field access caused the exception, making it easier to identify and fix the problem.
What is a null check and why is it important?
-A null check is a condition that verifies if an object reference is null before performing operations on it. It's important to prevent Null Pointer Exceptions when dealing with potentially null references.
What strategies can you use to avoid Null Pointer Exceptions in your Java code?
-Avoid initializing variables to null, return empty collections instead of null from methods, and use primitive types over wrapper classes to reduce the likelihood of encountering null values.
How can you safely compare a string to a string literal in Java without causing a Null Pointer Exception?
-You can safely compare a string to a string literal by using the string literal on the left side of the equals method, so if the string is null, the literal will not cause a Null Pointer Exception.
Outlines
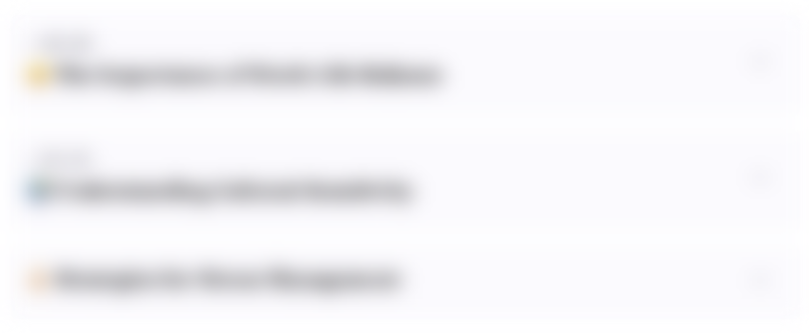
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
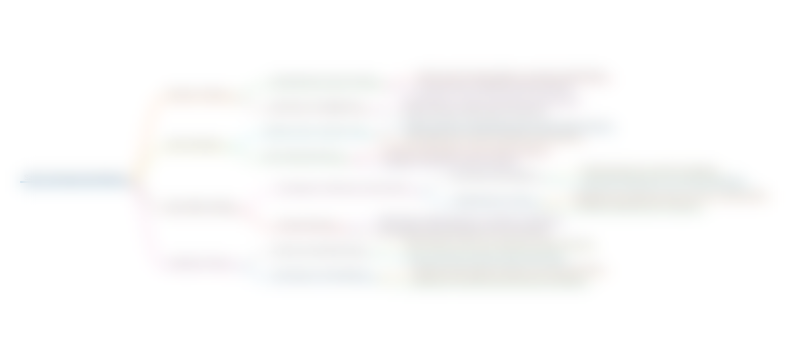
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
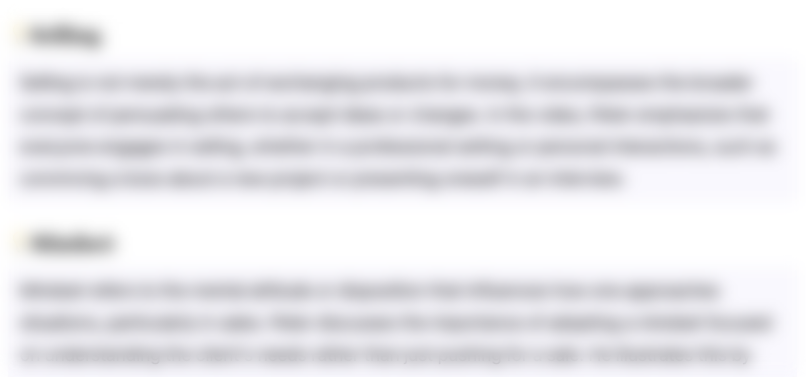
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
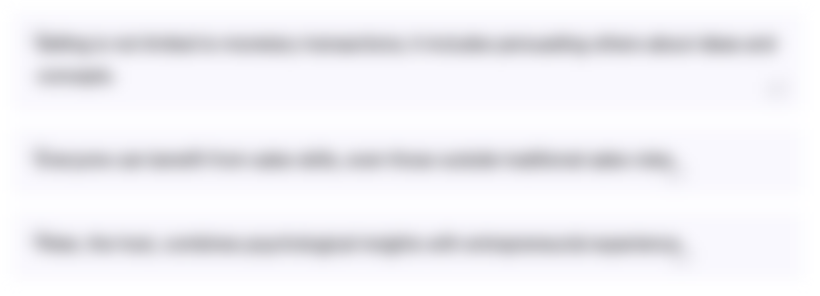
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
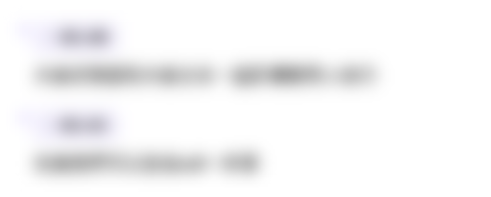
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
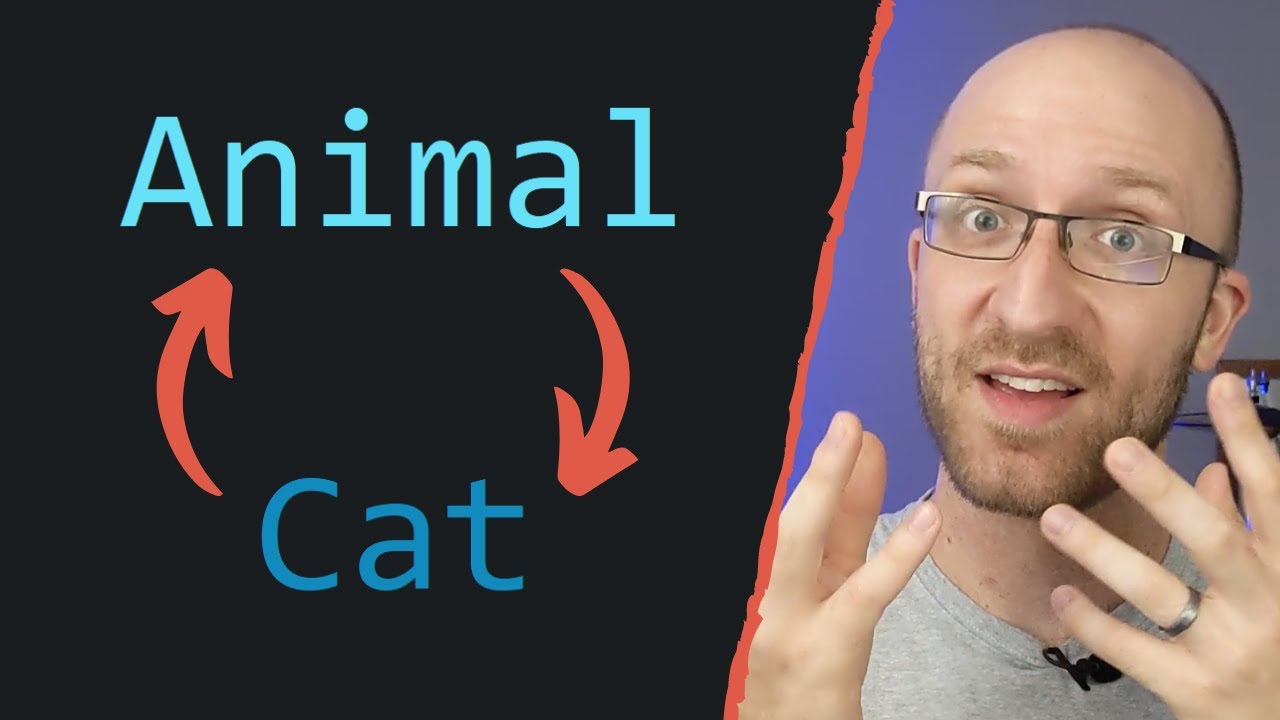
Upcasting and Downcasting in Java - Full Tutorial
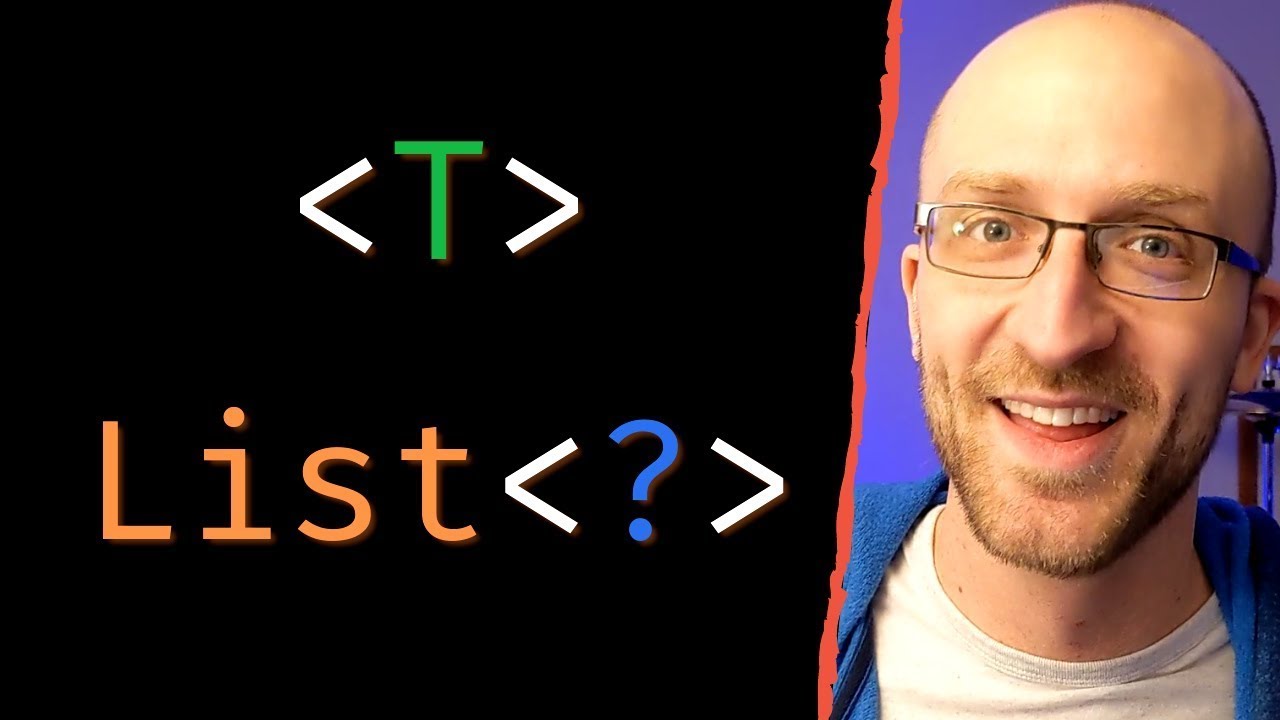
Generics In Java - Full Simple Tutorial
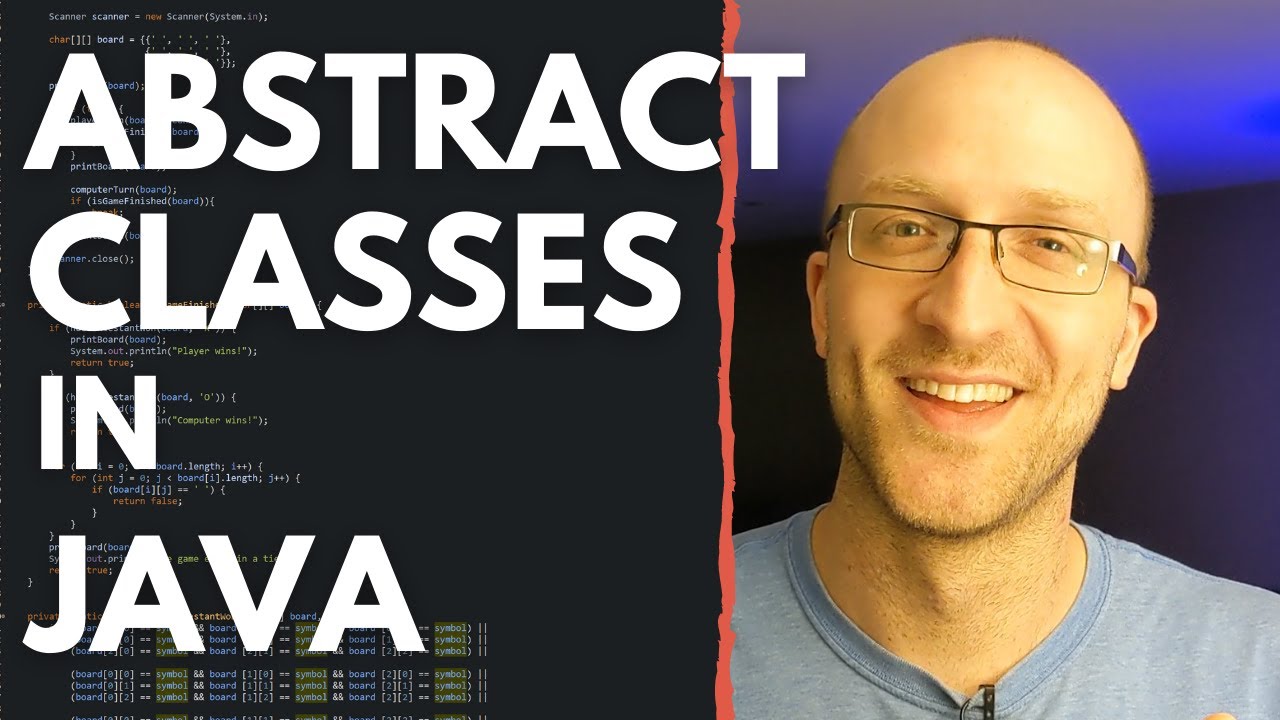
Abstract Classes and Methods in Java Explained in 7 Minutes
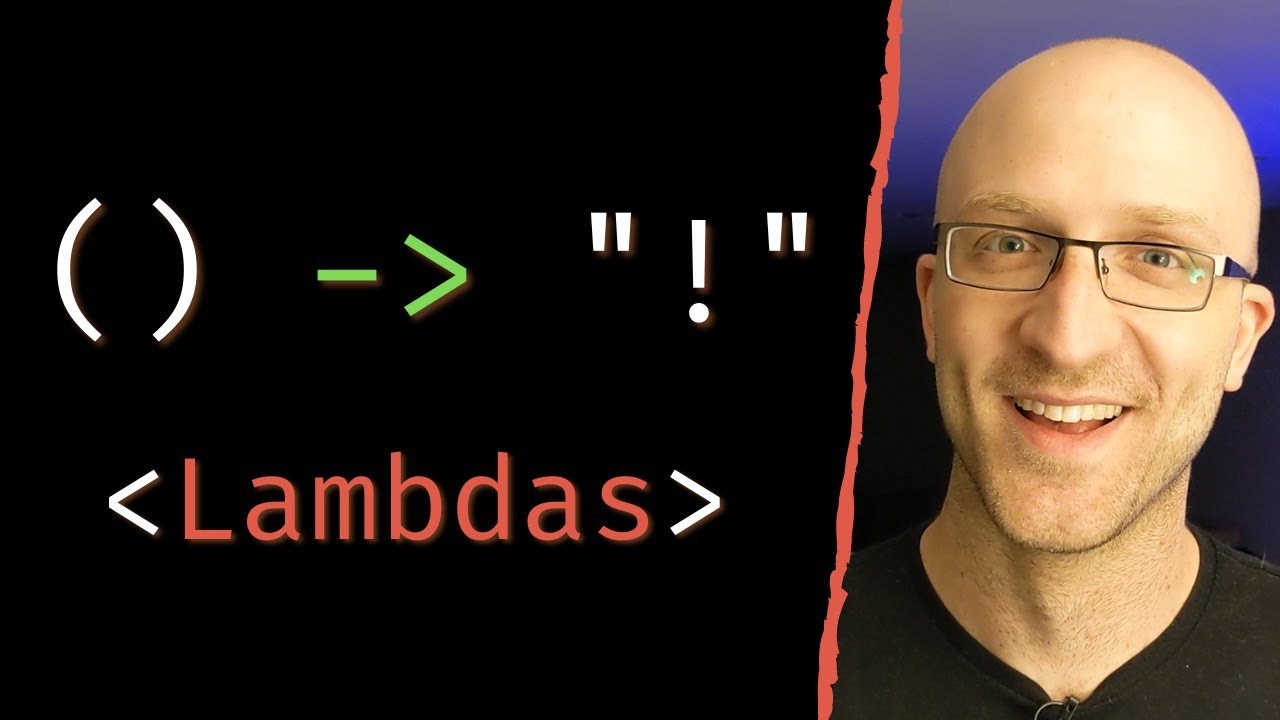
Lambda Expressions in Java - Full Simple Tutorial
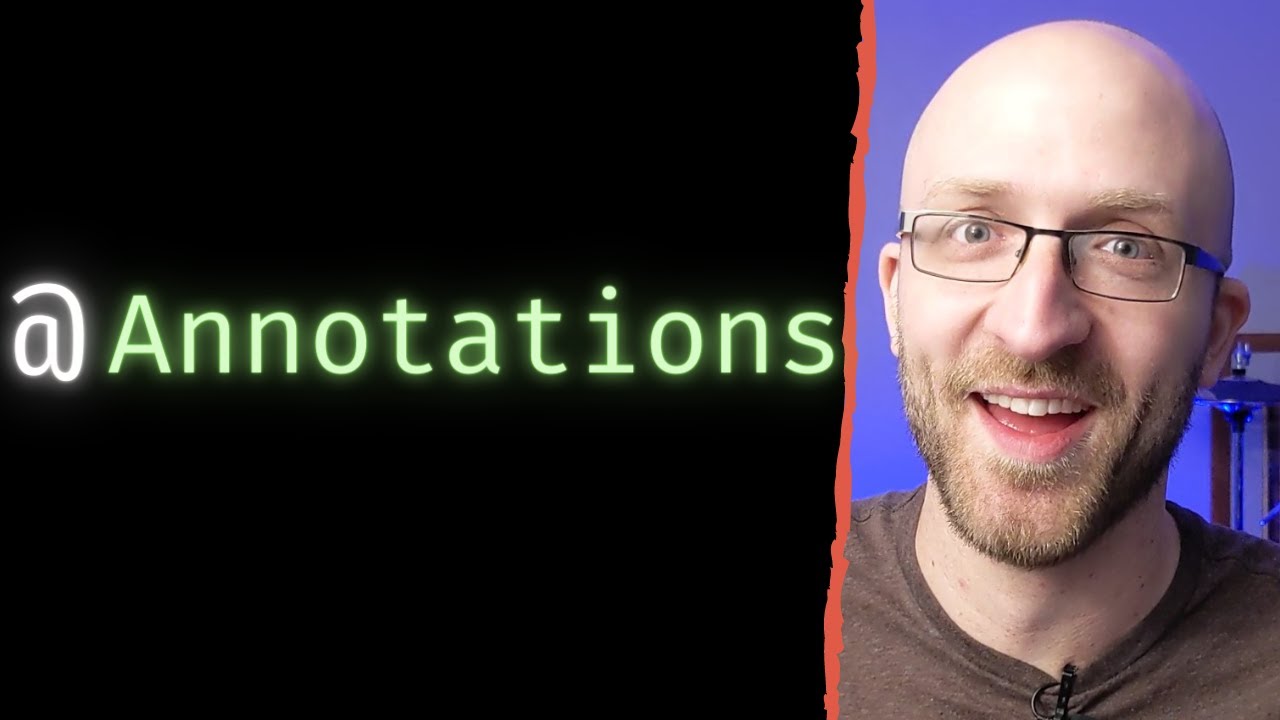
Annotations In Java Tutorial - How To Create And Use Your Own Custom Annotations
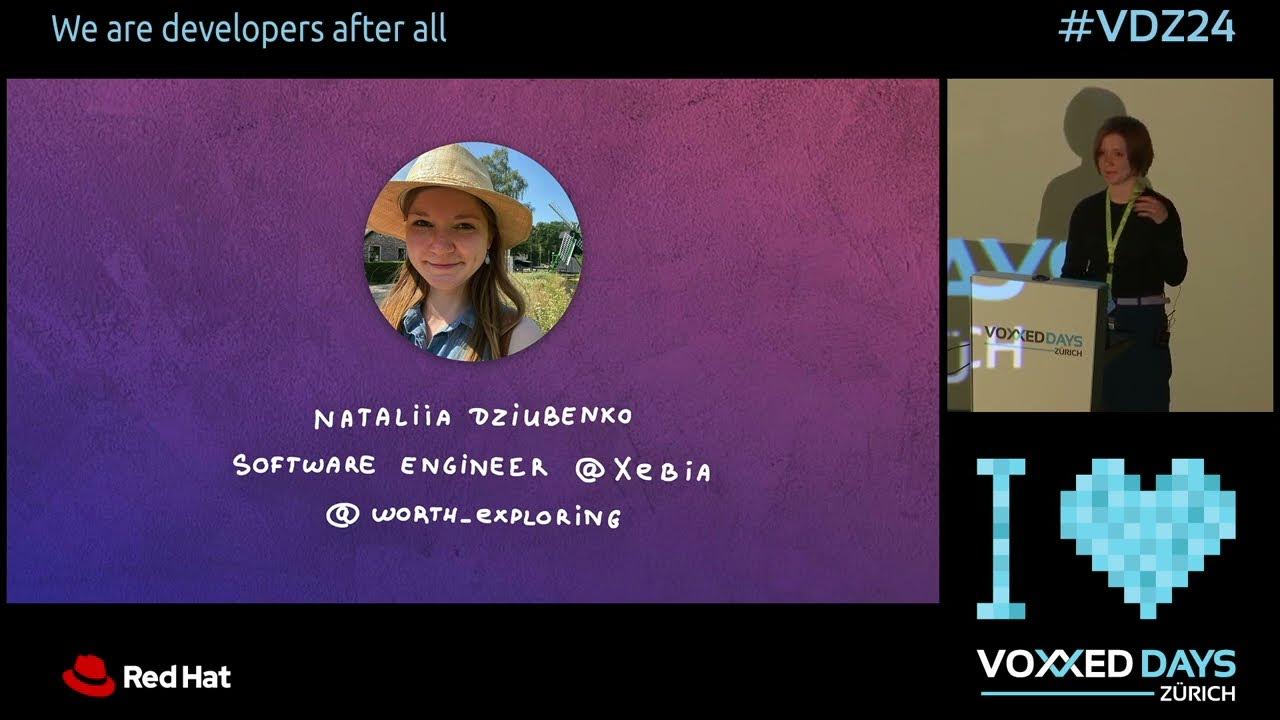
The Hidden Dynamic Life of Java by Nataliia Dziubenko
5.0 / 5 (0 votes)