Understanding the Null Pointers
Summary
TLDRThis presentation delves into the concept of null pointers in programming, defining them as pointers that do not point to any memory location, essentially representing invalid memory addresses. It emphasizes the importance of initializing pointers to NULL when they are not associated with a valid address, and the practice of checking for NULL before dereferencing to prevent errors. The script also covers the use of NULL pointers in error handling with the malloc function and clarifies that the value of NULL is zero but should not be confused with the integer zero. The size of a NULL pointer varies by platform, similar to normal pointers. Best practices include initializing pointers to NULL and performing NULL checks to ensure robust code.
Takeaways
- π A null pointer is a special type of pointer that does not point to any memory location, representing an invalid memory address.
- π Null pointers are initialized with the NULL value, which is distinct from the integer zero, even though both may have the value of zero in the context of pointers.
- π Initializing pointers with NULL is a good practice when they are not assigned any valid memory address to avoid errors.
- π§ The NULL pointer is particularly useful for error handling, especially when using the malloc function to dynamically allocate memory.
- β οΈ It's important to perform a NULL check before dereferencing any pointer to prevent undefined behavior or program crashes.
- π‘ The malloc function can return a NULL pointer when memory allocation fails, signaling that the pointer does not point to a valid memory location.
- π The size of a NULL pointer is platform-dependent and is similar to the size of a normal pointer, which can vary between systems.
- π« Initializing a pointer with zero is not recommended as it can lead to confusion with the integer zero and should be done with NULL instead.
- π The value of NULL is zero, but it is a special value used in the context of pointers and should not be confused with the integer zero.
- π When a pointer is assigned the NULL value, it becomes a NULL pointer, indicating that it does not point to any valid memory location.
- π Understanding null pointers is crucial for safe and effective memory management in programming, especially in languages like C and C++.
Q & A
What is a null pointer in the context of programming?
-A null pointer is a special type of pointer that does not point to any memory location, essentially representing an invalid memory address.
Why is it important to understand null pointers?
-Understanding null pointers is important for proper memory management and error handling in programming, as they are used to indicate the absence of a valid memory address.
What is the purpose of initializing a pointer with NULL?
-Initializing a pointer with NULL is a good practice when the pointer is not yet assigned any valid memory address, as it helps to avoid errors and undefined behavior.
How is a null pointer used in error handling with the malloc function?
-The malloc function can return a null pointer when memory allocation fails. Checking for a null pointer after a malloc call allows for proper error handling in case of memory allocation failure.
What is the significance of the NULL value being zero?
-The value of NULL being zero is significant because it can be used interchangeably with NULL in the context of pointers. However, it is not equivalent to the integer zero and should not be confused with it.
Why should a pointer be initialized with NULL instead of zero?
-Initializing a pointer with NULL instead of zero avoids confusion with the integer zero and clearly indicates that the pointer is not pointing to a valid memory address.
What is the size of a NULL pointer?
-The size of a NULL pointer depends on the platform and is similar to the size of a normal pointer, which can vary, for example, being eight bytes on one computer and possibly four bytes on another.
What is the recommended best practice for handling pointers in programming?
-It is a best practice to initialize pointers to NULL when they are not pointing to a valid memory address and to perform a NULL check before dereferencing any pointer to prevent errors.
How does the script differentiate between a null pointer and an integer zero?
-The script clarifies that while the value of NULL is zero, it is a special kind of value used in the context of pointers and should not be confused with the integer zero.
What is the final recommendation given in the script regarding the use of null pointers?
-The script recommends initializing pointers to NULL when they are not assigned a valid memory address and always checking for NULL before dereferencing to avoid unexpected behavior or errors.
Outlines
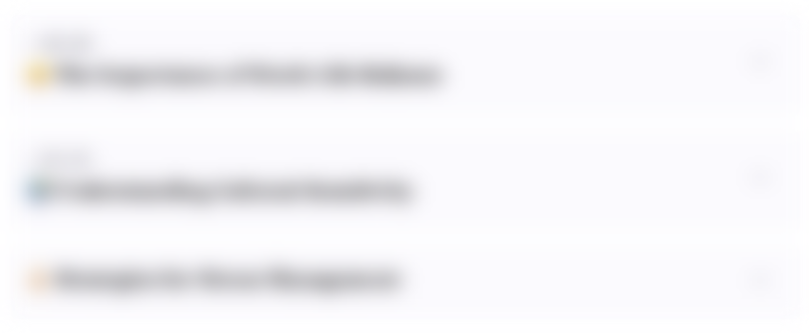
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
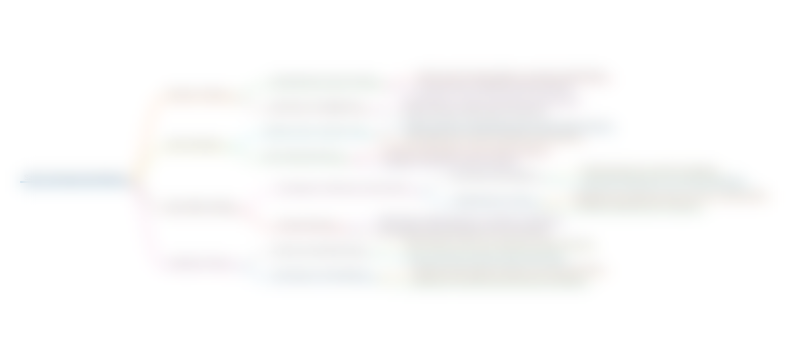
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
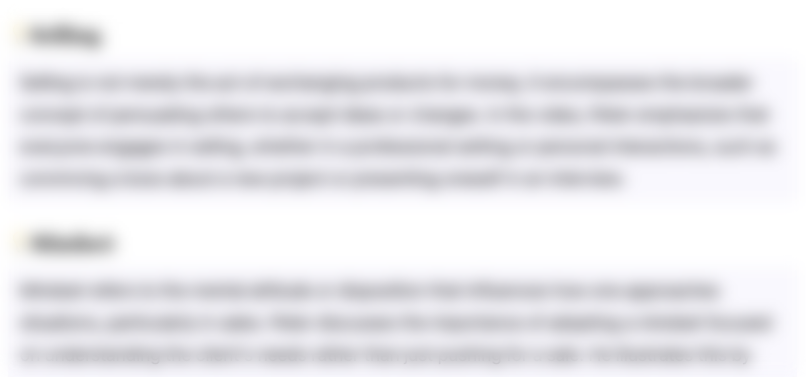
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
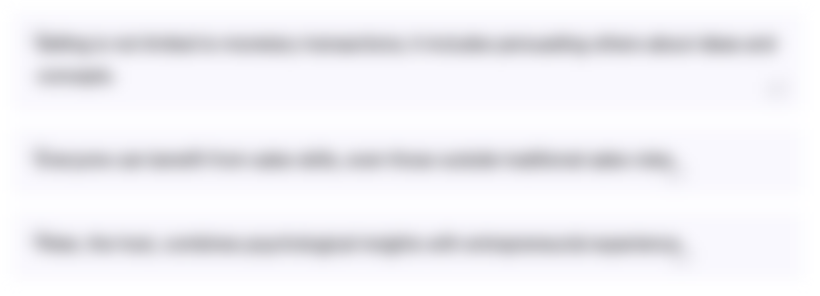
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
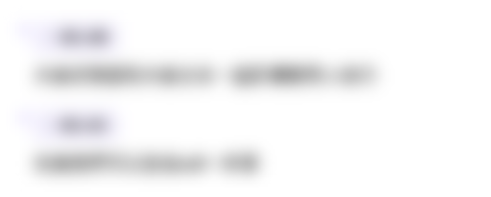
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)