Sequence_data_part_1
Summary
TLDRThe video script is a comprehensive tutorial on sequence data types in Python. It covers fundamental concepts like strings, lists, tuples, arrays, and sets, explaining their creation and manipulation. The script also delves into more complex data structures, demonstrating how to use functions like 'range' and 'dict' to generate sequences. It provides practical examples, such as creating a string with quotes and a list with numerical values, emphasizing the importance of sequence data types in Python programming.
Takeaways
- đ The lecture introduces the concept of sequential data types in Python.
- đ Sequential data types allow for the creation and manipulation of multiple values within a system.
- đĄ Examples of sequential data types include strings, Unicode strings, lists, tuples, and collections.
- đŹ Strings in Python are ordered sequences of characters and can be created using single, double, or triple quotes.
- đ Lists are created using square brackets and can contain items of different data types, including numbers and strings.
- đ Tuples are similar to lists but are immutable, meaning their contents cannot be changed after creation.
- đïž Sets and dictionaries are mentioned as non-sequential data types that store unique elements and key-value pairs respectively.
- đ ïž Arrays are discussed as a way to store collections of similar data types, often used for numerical data.
- đ The lecture demonstrates the creation and manipulation of strings, lists, and arrays with examples.
- đ The importance of understanding data types and their properties is emphasized for effective Python programming.
Q & A
What is the main topic of the lecture?
-The main topic of the lecture is sequential data types in programming, specifically focusing on their usage and examples in Python.
What are the basic sequential data types discussed in the lecture?
-The basic sequential data types discussed include strings, Unicode strings, lists, tuples, and collections such as arrays and object sequences.
How are strings defined in Python according to the lecture?
-Strings in Python are defined as an ordered sequence of characters, which can include letters, numbers, and symbols, and can be enclosed in single, double, or triple quotes.
What is a list in Python?
-A list in Python is a collection of items that are ordered and mutable, which means the items can be changed, and it can be defined using square brackets.
What is the difference between a list and a tuple in Python?
-The main difference is that a list is mutable, meaning its contents can be changed, while a tuple is immutable, meaning its contents cannot be altered once created.
What is an array in Python?
-An array in Python is a collection of objects stored in contiguous memory locations, and it is often used to store a collection of similar data types.
How are dictionaries created in Python?
-Dictionaries in Python are created using curly braces, where keys are paired with values, and the key-value pairs are separated by commas.
What is a set in Python?
-A set in Python is an unordered collection of distinct elements, which can be created using the set function or by placing elements within curly braces.
How can you create a range in Python?
-A range in Python can be created using the range function, which takes parameters such as start, stop, and step values to generate a sequence of numbers.
What is the purpose of loops in Python when working with sequential data?
-Loops in Python are used to iterate over sequential data types to perform operations on each element, and they are commonly used with structures like lists and tuples.
How can you print the elements of a list in Python?
-The elements of a list in Python can be printed using a for loop, which iterates through each element and prints it, or by using the print function with the list as an argument.
Outlines
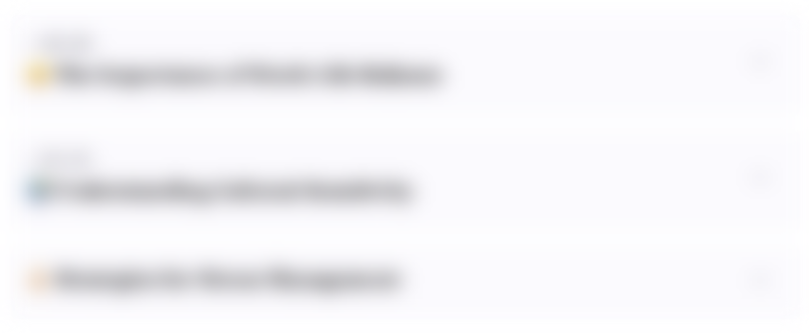
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
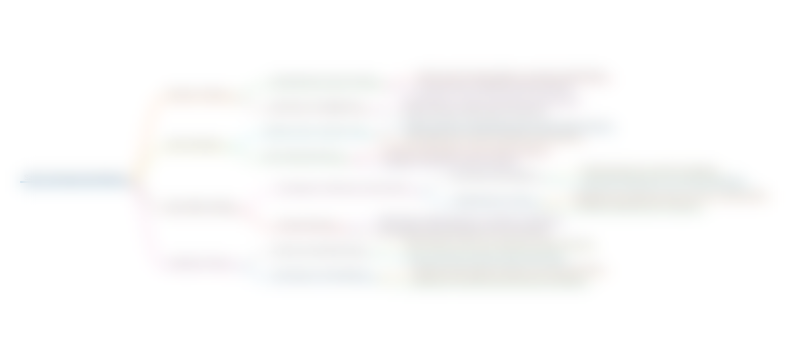
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
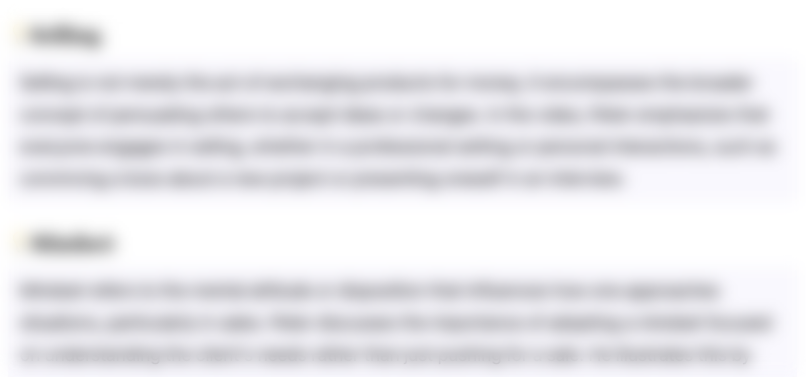
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
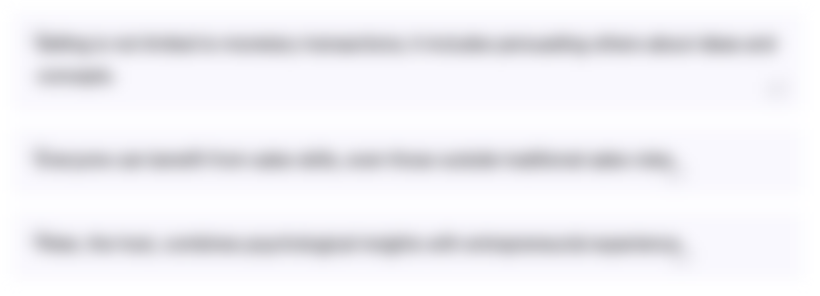
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
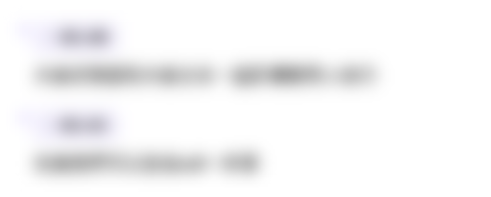
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
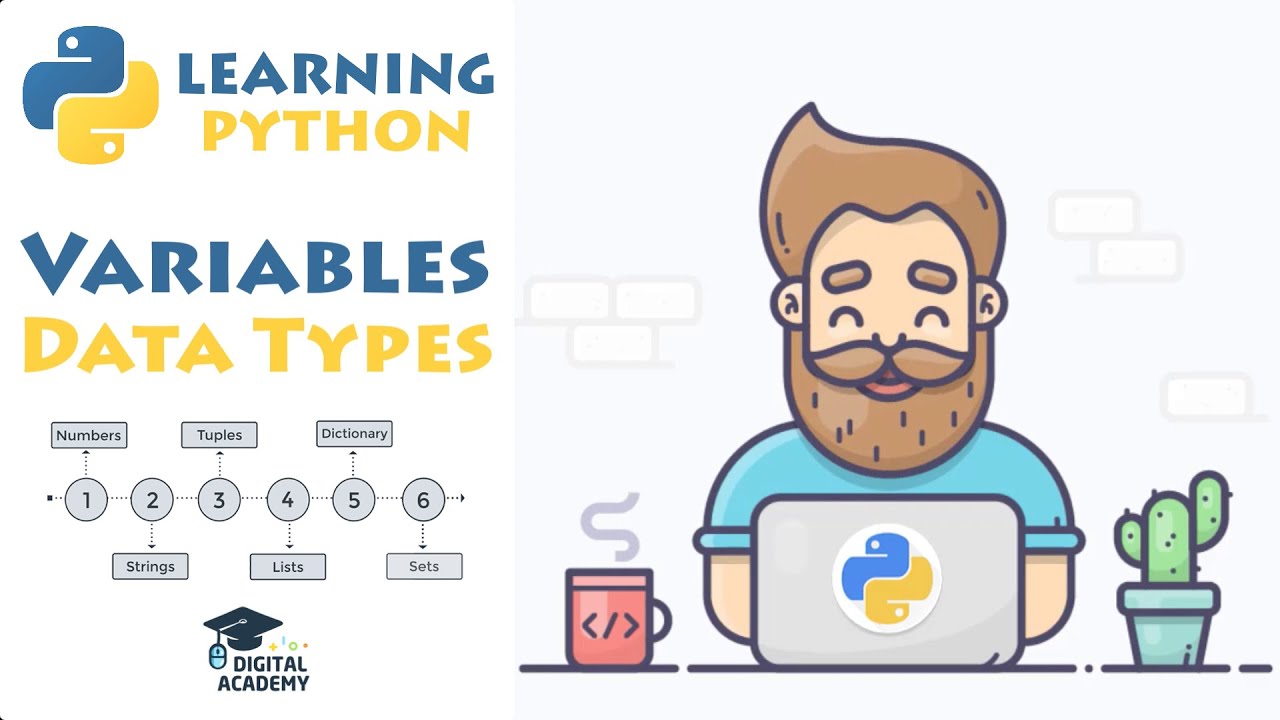
DATA TYPES in Python (Numbers, Strings, Lists, Dictionary, Tuples, Sets) - Python for Beginners
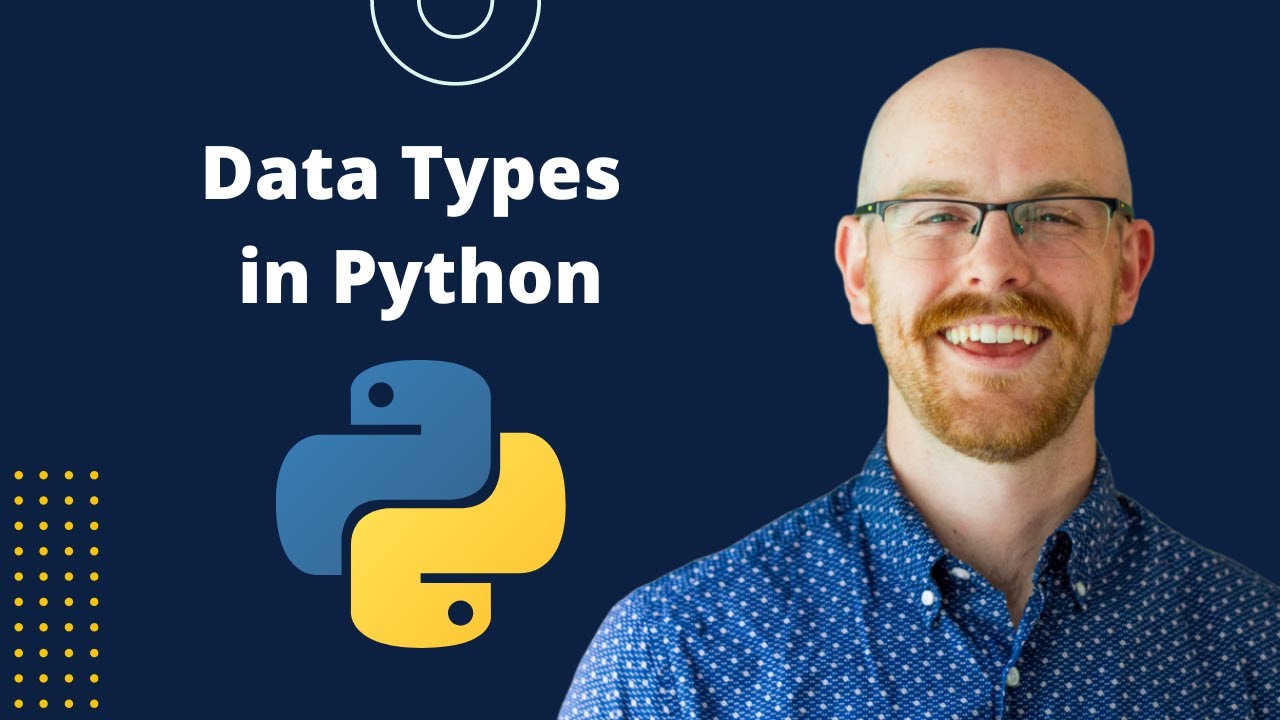
Data Types in Python | Python for Beginners
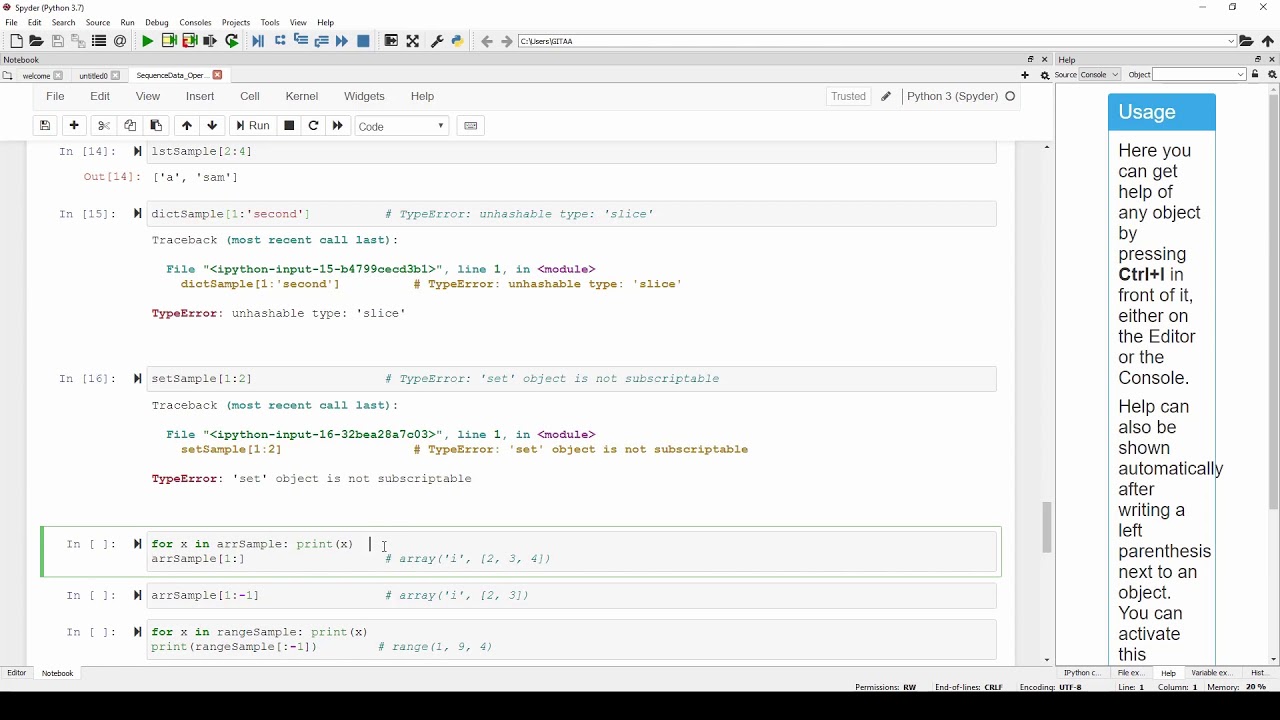
Sequence data part 3
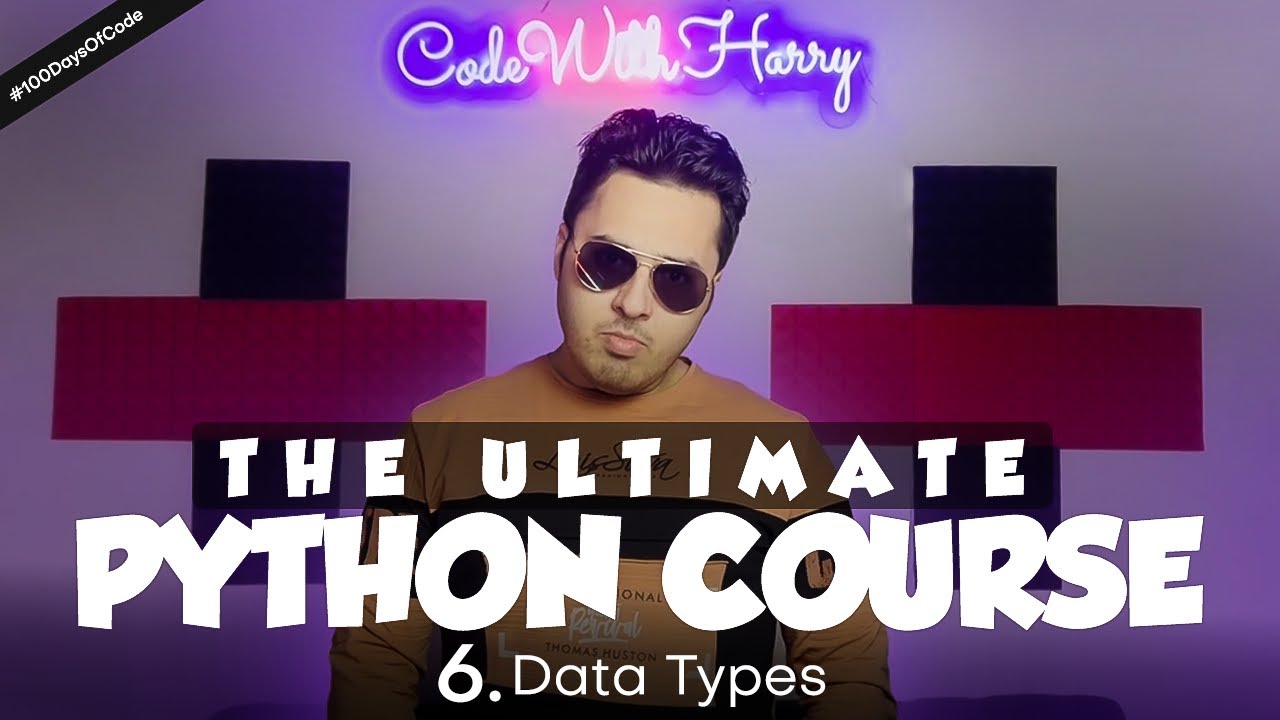
Variables and Data Types | Python Tutorial - Day #6
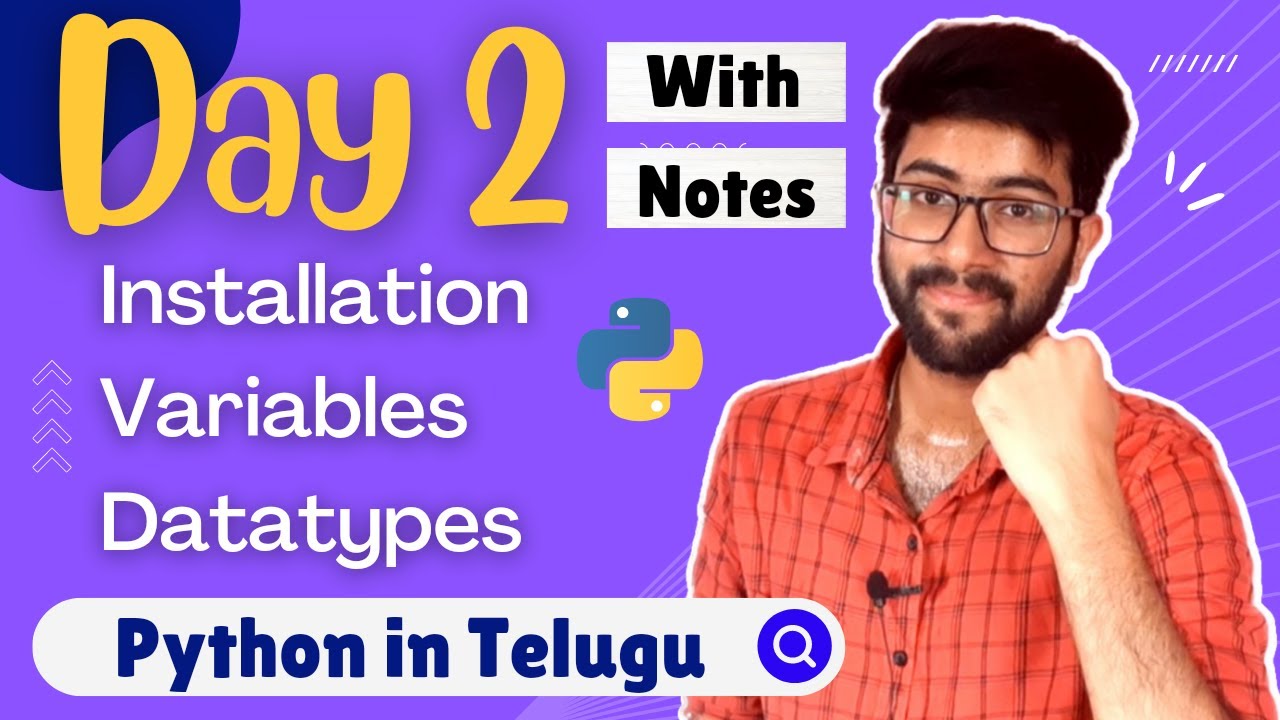
Day 2 : Python Installation, Variables, Datatypes | Python Course in Telugu | Vamsi Bhavani
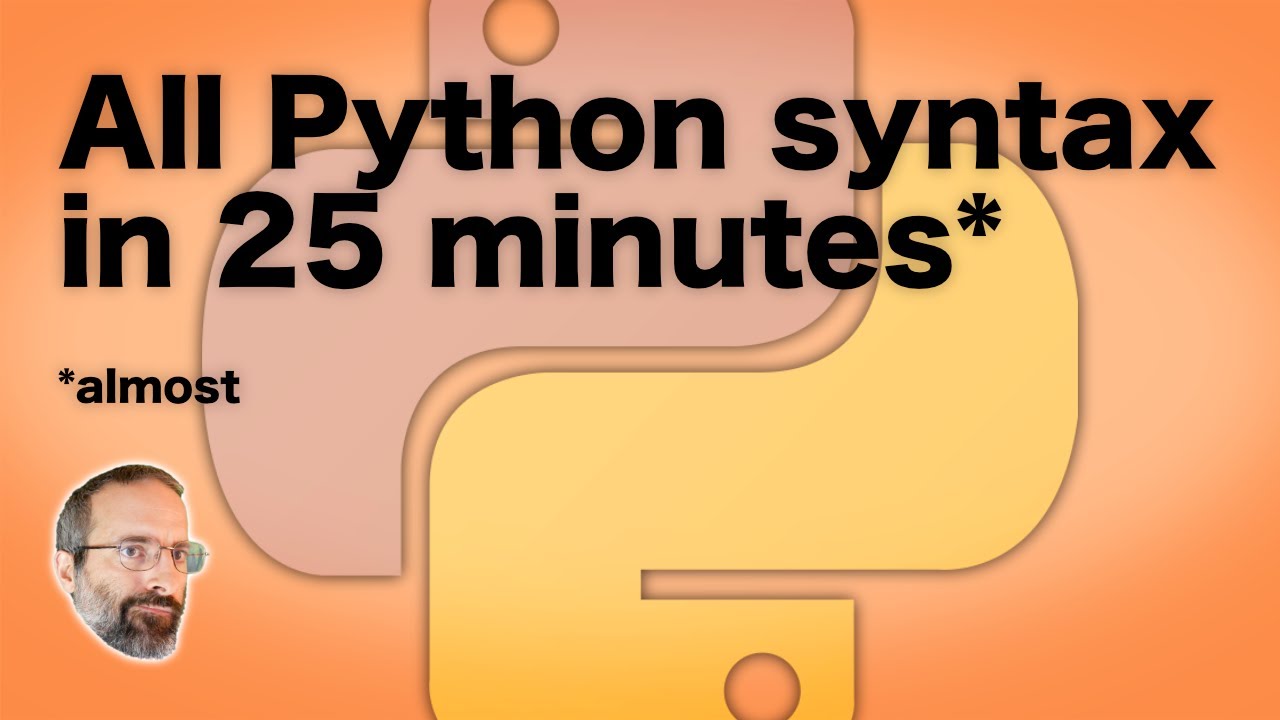
All Python Syntax in 25 Minutes â Tutorial
5.0 / 5 (0 votes)