DATA TYPES in Python (Numbers, Strings, Lists, Dictionary, Tuples, Sets) - Python for Beginners
Summary
TLDRThis Python development course tutorial introduces fundamental data types in Python, essential for beginners. It explains that every value in Python has a data type, which are actually classes, and variables are their instances. The tutorial covers basic data types like numeric, string, and boolean, and collection types including tuples, lists, dictionaries, and sets. It highlights the difference between mutable and immutable objects, with numbers, strings, and tuples being immutable, while lists and dictionaries are mutable. The video also touches on type conversion and the use of built-in functions like type() and isinstance() to interact with data types.
Takeaways
- π₯ Python variables and data types are essential building blocks in programming.
- π» Python has several basic data types like numeric, string, and boolean types.
- π’ Numeric data types include integers, floats, and complex numbers, each with specific uses and properties.
- π Python integers can be of any length, and floats support up to 15 decimal places, with scientific notation available.
- π‘ Strings in Python are immutable, and Python does not have a character data type. A single character is treated as a string of length 1.
- π§© Lists are mutable, ordered collections, while tuples are immutable, ordered collections.
- π Dictionaries are unordered collections of key-value pairs, optimized for data retrieval.
- π Python allows for conversion between different data types using constructors like `int()`, `float()`, and `str()`.
- βοΈ Boolean data types represent `True` or `False` values and are used to evaluate expressions and conditions.
- π Sets in Python are mutable collections of unique elements and allow for operations like union and intersection.
Q & A
What are data types in Python?
-Data types in Python represent the classification of data items, indicating the kind of value stored and what operations can be performed on that value. Python data types include numeric, string, boolean, tuples, lists, dictionaries, and sets.
What is the difference between mutable and immutable objects in Python?
-Mutable objects, like lists and dictionaries, can be modified after creation by adding, deleting, or rearranging elements. Immutable objects, such as numbers, strings, and tuples, cannot be changed after creation.
How are numeric values represented in Python?
-Numeric values in Python are represented by the int, float, and complex classes. Integers are whole numbers, floats are real numbers with decimal points, and complex numbers are represented as a combination of real and imaginary parts (e.g., 2 + 3j).
How do you check the data type of a variable in Python?
-You can use the type() function to know which class a variable or value belongs to, and the isinstance() function to check if an object belongs to a specific class.
What are the characteristics of strings in Python?
-Strings in Python are immutable sequences of characters, represented by the str class. They can be created using single or double quotes, and multi-line strings can be enclosed in triple quotes. Strings are indexed, and you can access characters using the slicing operator.
How are lists defined and used in Python?
-Lists are mutable, ordered collections of items that can be of any data type. They are declared by placing elements within square brackets, separated by commas. Lists can be modified after creation and allow duplicate elements.
What is the difference between a list and a tuple?
-A list is mutable, meaning its contents can be altered after creation, while a tuple is immutable, meaning its contents cannot be changed. Tuples are used for fixed data, and lists for dynamic collections.
What is a dictionary in Python, and how is it structured?
-A dictionary in Python is an unordered, indexed collection of key-value pairs. Each key is unique, and the key-value pairs are separated by a colon. Dictionaries are useful for storing large amounts of data that can be accessed using the key.
What is a set, and what are its advantages in Python?
-A set is an unordered, mutable collection of unique elements. Sets are defined by curly braces and do not allow duplicate values. One key advantage of sets is their optimized method for checking if a specific element is contained in the set.
How can you convert between data types in Python?
-You can convert between data types using constructors like int(), float(), str(), list(), and tuple(). Type casting is the process of changing one data type to another, which allows you to manipulate data in different ways.
Outlines
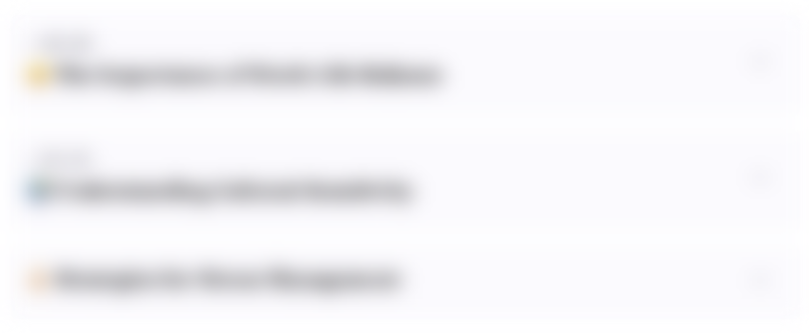
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
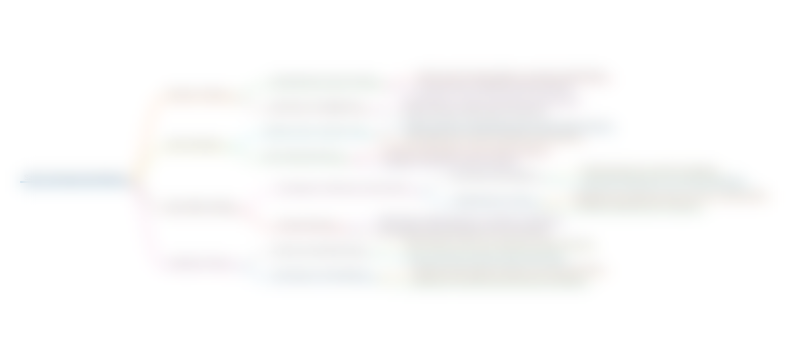
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
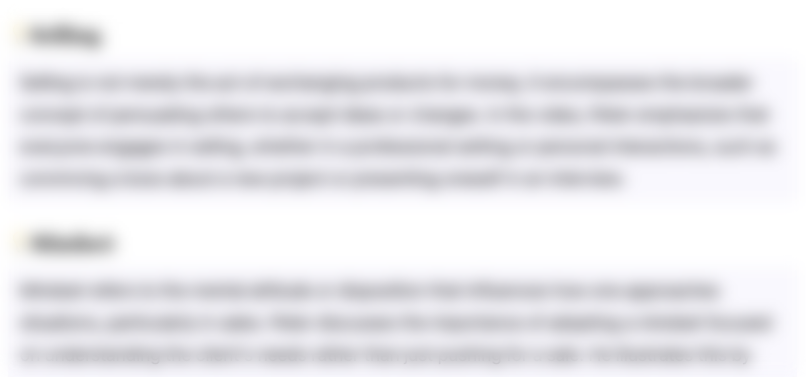
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
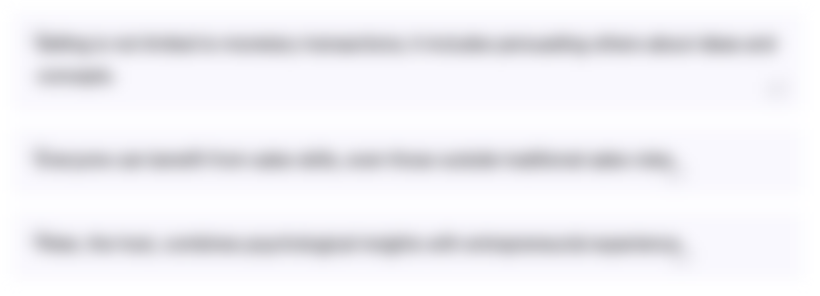
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
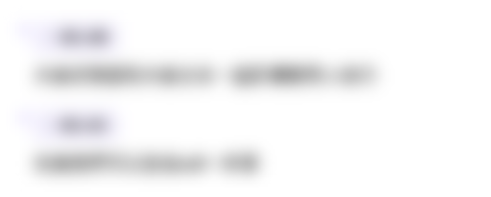
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
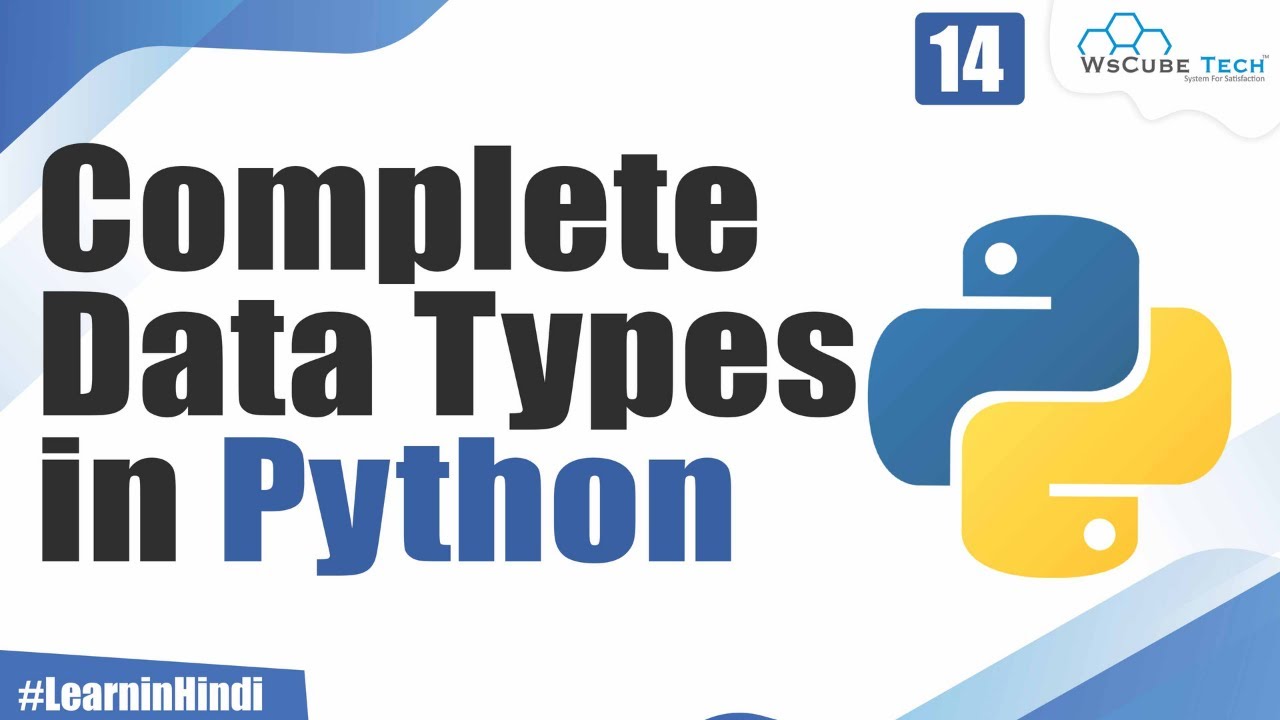
What are Data Types in Python | All Data Types | Tutorial For Beginners
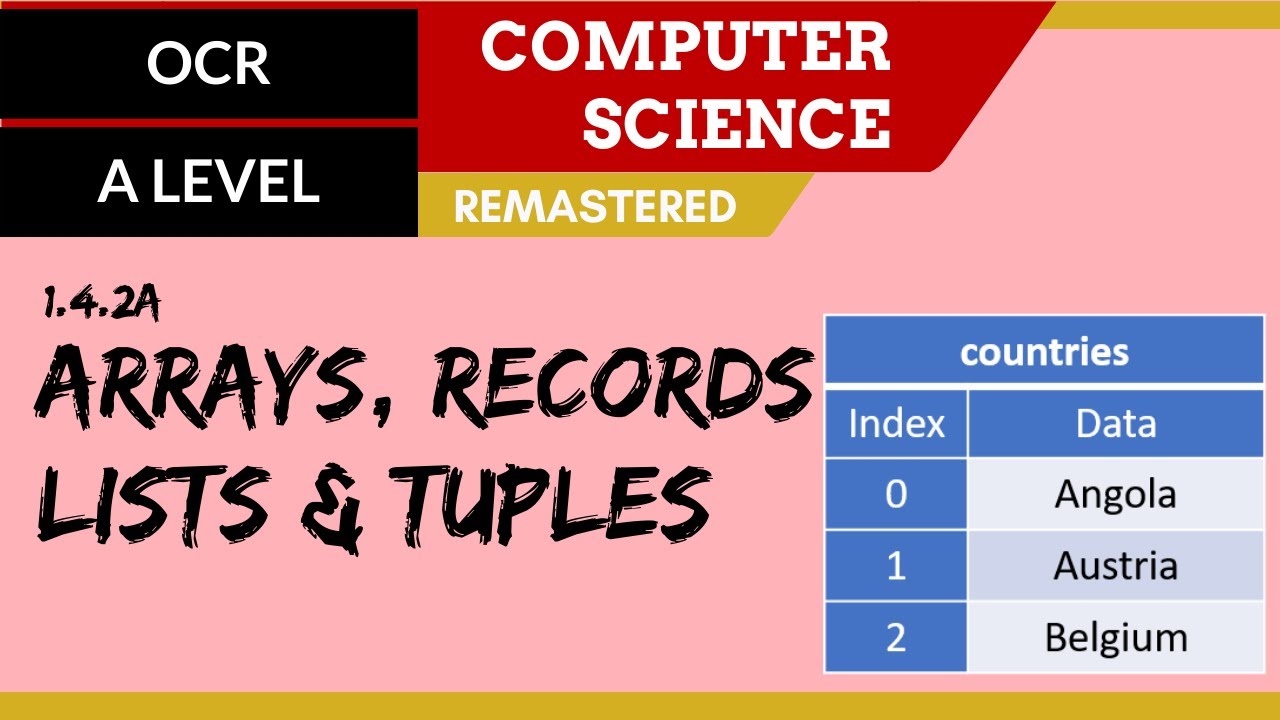
85. OCR A Level (H046-H446) SLR14 - 1.4 Arrays, records, lists & tuples
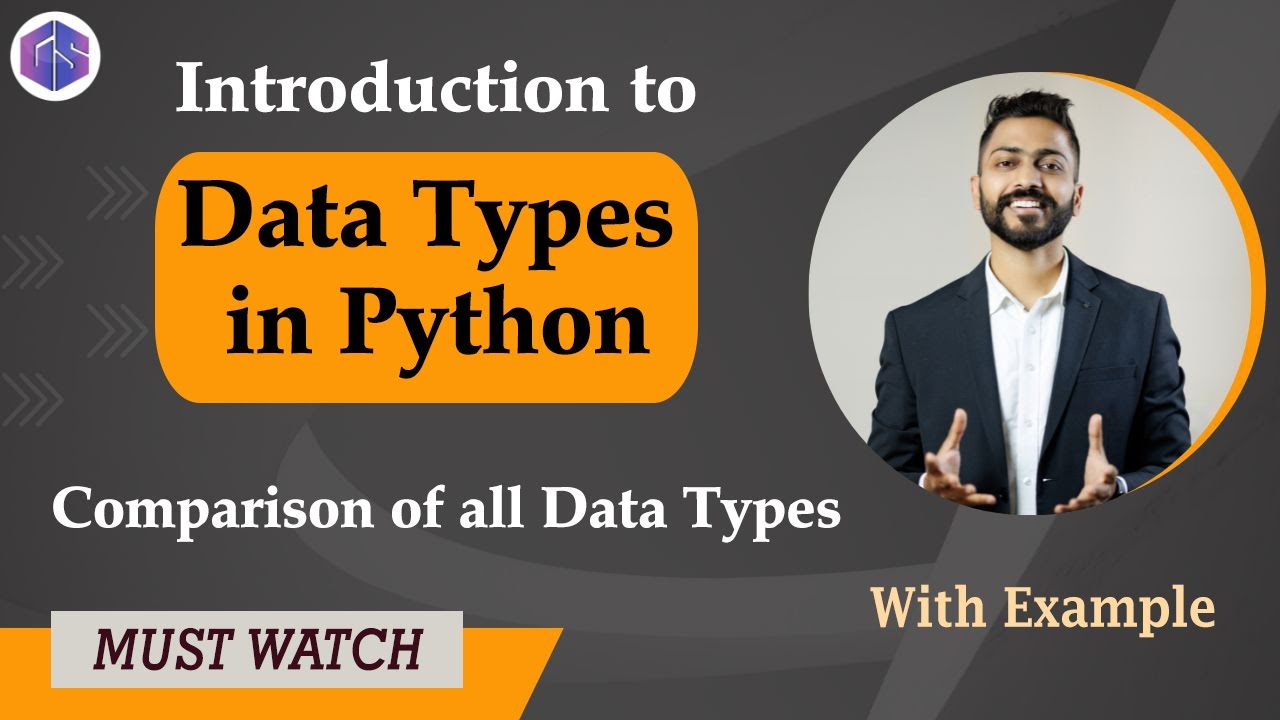
Lec-15: Various Data types in Pythonπ | Comparison of all python data types
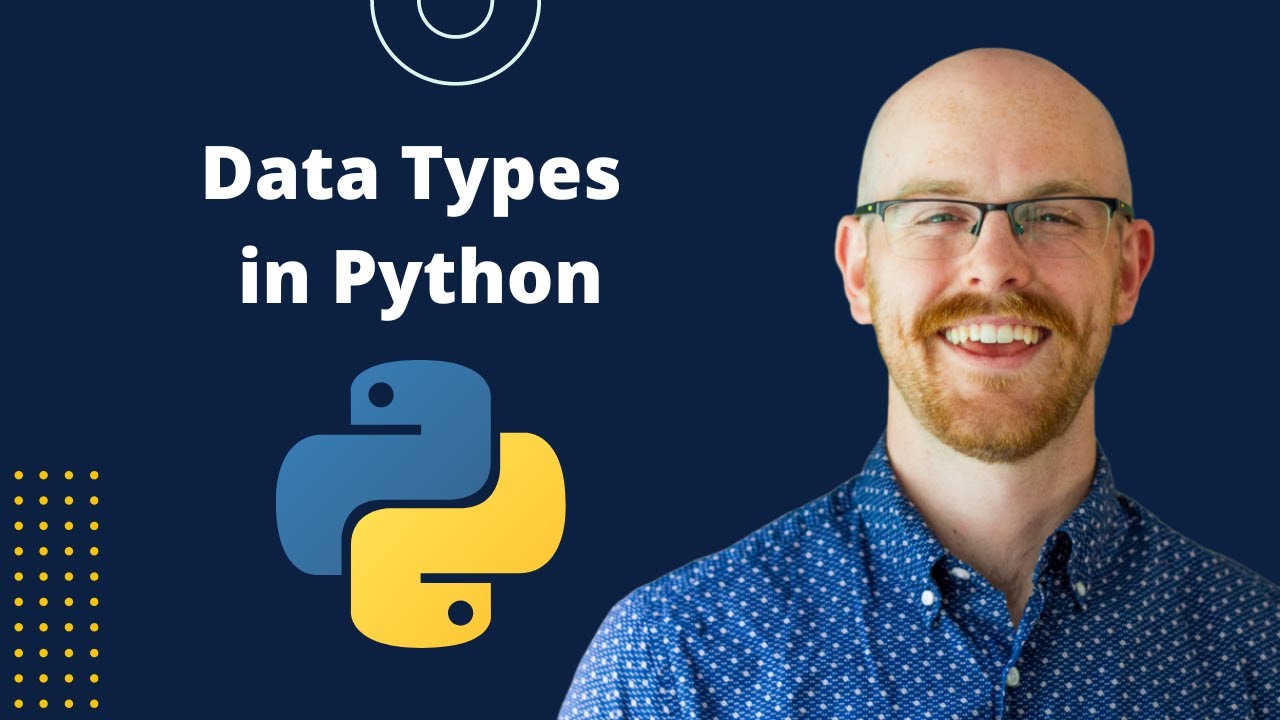
Data Types in Python | Python for Beginners
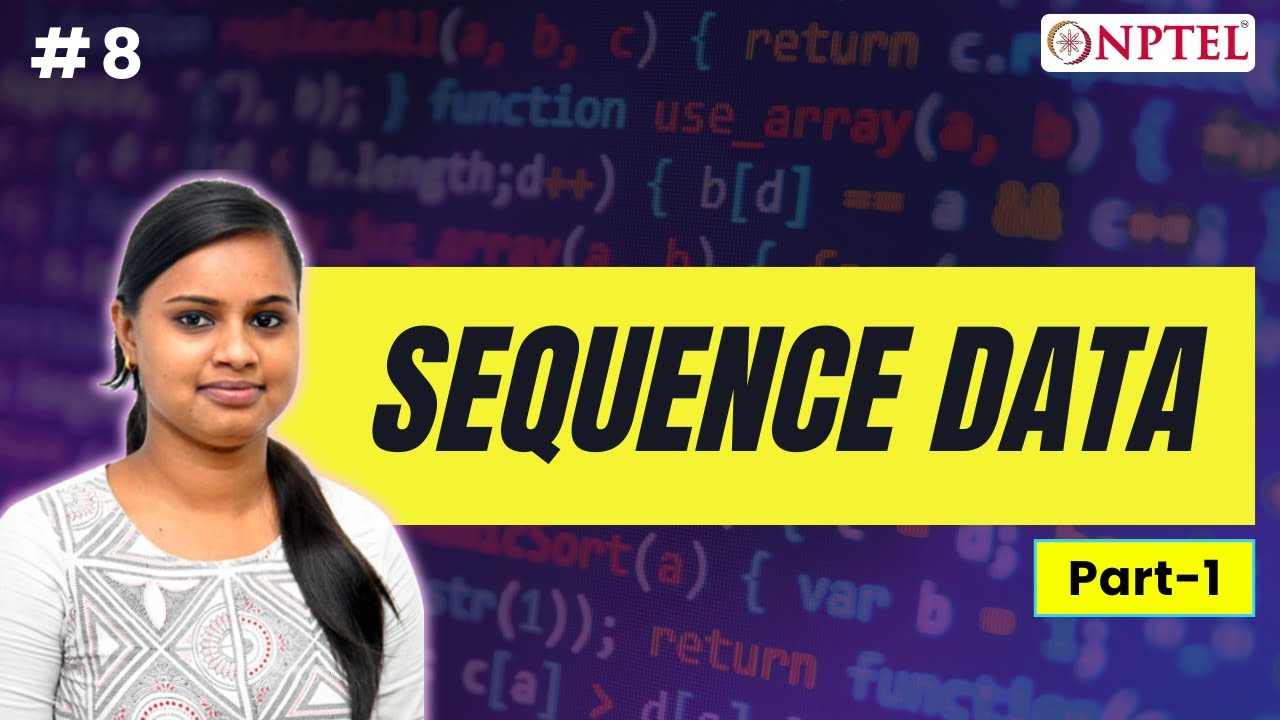
Sequence_data_part_1
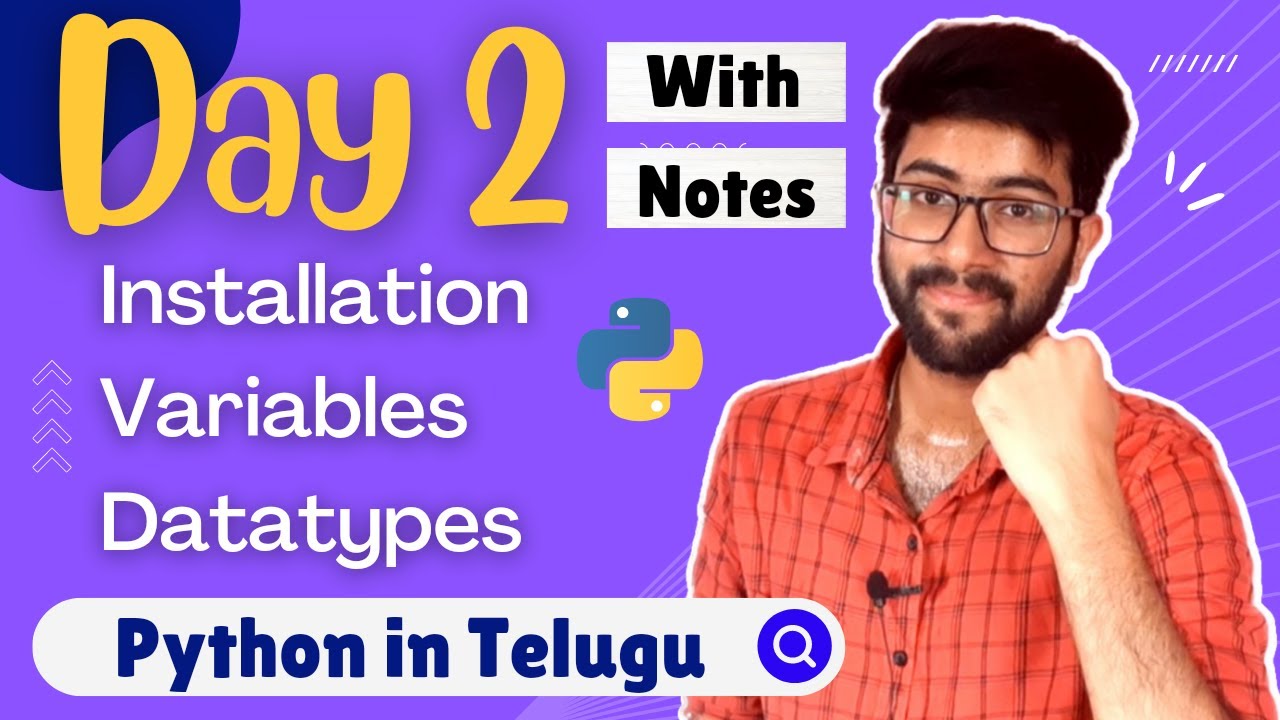
Day 2 : Python Installation, Variables, Datatypes | Python Course in Telugu | Vamsi Bhavani
5.0 / 5 (0 votes)