C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
Summary
TLDRThis video tutorial delves into the concept of pointers in C programming, specifically focusing on double pointers or pointers to pointers. The instructor begins with a review of basic pointers and operations before advancing to the double pointer concept. Through detailed explanations and visual memory representations, viewers learn how to declare, initialize, and use double pointers. The tutorial also covers practical examples and code demonstrations using VS Code, ensuring a clear understanding of how double pointers function and their importance in C programming.
Takeaways
- 📌 Pointers are crucial in C programming for handling memory addresses.
- 💡 The 'address of' operator (&) and 'indirection' operator (*) are fundamental to understanding pointers.
- 🖥️ Pointers can store the address of another variable, enabling efficient memory management.
- 🔄 A pointer to a pointer, or a double pointer, stores the address of another pointer, not a normal variable.
- 📝 Declaring a pointer to a pointer involves using double asterisks (**), indicating two levels of indirection.
- 🔧 Properly initializing pointers is essential to avoid incompatible pointer type errors.
- 🖱️ Accessing the value of a variable through a single pointer uses one asterisk (*), while a double pointer uses two asterisks (**).
- 🔍 Visual representation of memory allocation helps in understanding how pointers work.
- 📊 The concept of pointers can be extended to multiple levels, such as triple pointers, to manage more complex data structures.
- 💻 Practical examples and coding in an editor like VS Code solidify the understanding of pointers in C programming.
Q & A
What is the main topic of this video?
-The main topic of this video is the concept of pointers in C programming, specifically focusing on pointer to pointer (double pointer).
What are the two operators discussed in relation to pointers?
-The two operators discussed are the address-of operator (&) and the indirection operator (*).
What is a double pointer?
-A double pointer, or pointer to pointer, is a special pointer that stores the address of another pointer.
Why is the concept of double pointers important?
-Double pointers are important because they allow for more complex data structures and memory management techniques, such as dynamic memory allocation and manipulation of multidimensional arrays.
How do you declare and initialize a double pointer?
-You declare a double pointer with two asterisks (**) and initialize it by storing the address of a single pointer. For example: `int **q = &p;` where `p` is a pointer.
What is the correct way to access the value of a variable using a double pointer?
-To access the value of a variable using a double pointer, you use the indirection operator twice. For example, `**q` will give you the value of the variable that `q` ultimately points to.
What happens if you try to store an integer value directly into a pointer variable?
-If you try to store an integer value directly into a pointer variable, it will give an error because a pointer variable should store an address, not a direct integer value.
How can you print the address of a pointer variable?
-You can print the address of a pointer variable using the address-of operator (&) and the %p format specifier in printf, e.g., `printf("%p", &p);`.
What error will occur if you assign the address of a normal variable to a double pointer?
-Assigning the address of a normal variable to a double pointer will result in an incompatible pointer type error because a double pointer should point to a single pointer.
How do you change the value of a variable using a double pointer?
-To change the value of a variable using a double pointer, you assign a new value to `**q`, where `q` is the double pointer. For example, `**q = 25;` will change the value of the variable pointed to by `q`.
What is a three-level pointer, and how do you declare it?
-A three-level pointer is a pointer to a double pointer, allowing for even more levels of indirection. You declare it with three asterisks (***). For example: `int ***r = &q;` where `q` is a double pointer.
How can you access a variable using a three-level pointer?
-To access a variable using a three-level pointer, you use the indirection operator three times. For example, `***r` will give you the value of the variable that `r` ultimately points to.
Outlines
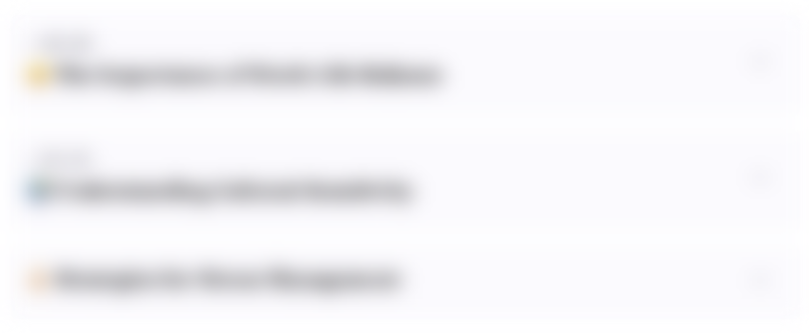
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
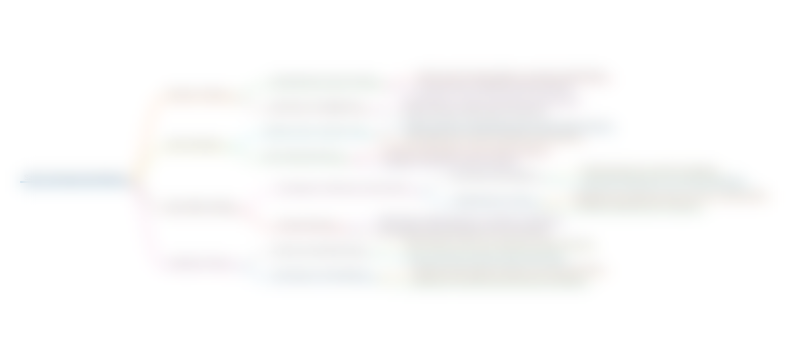
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
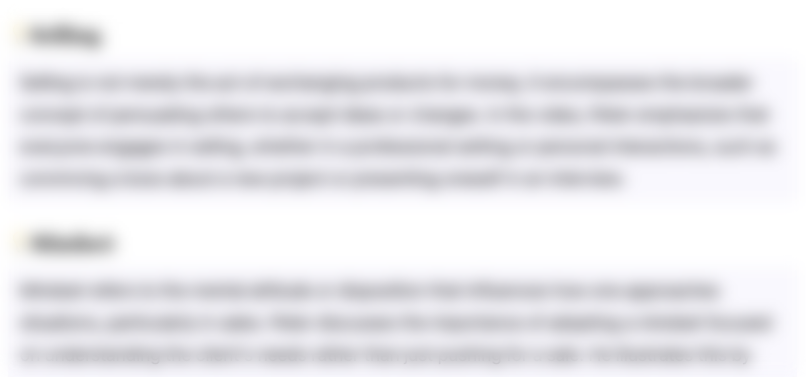
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
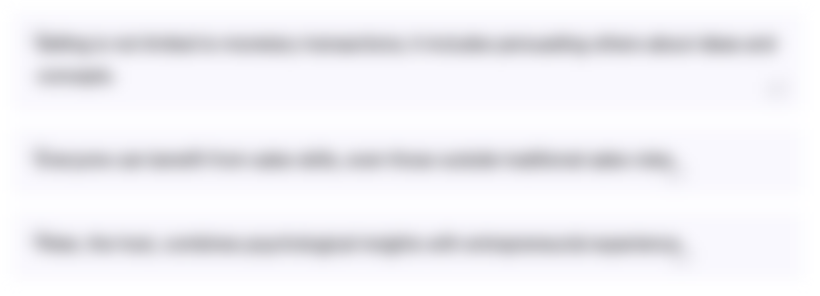
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
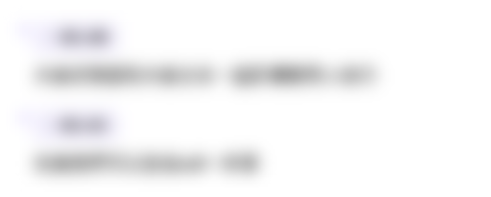
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
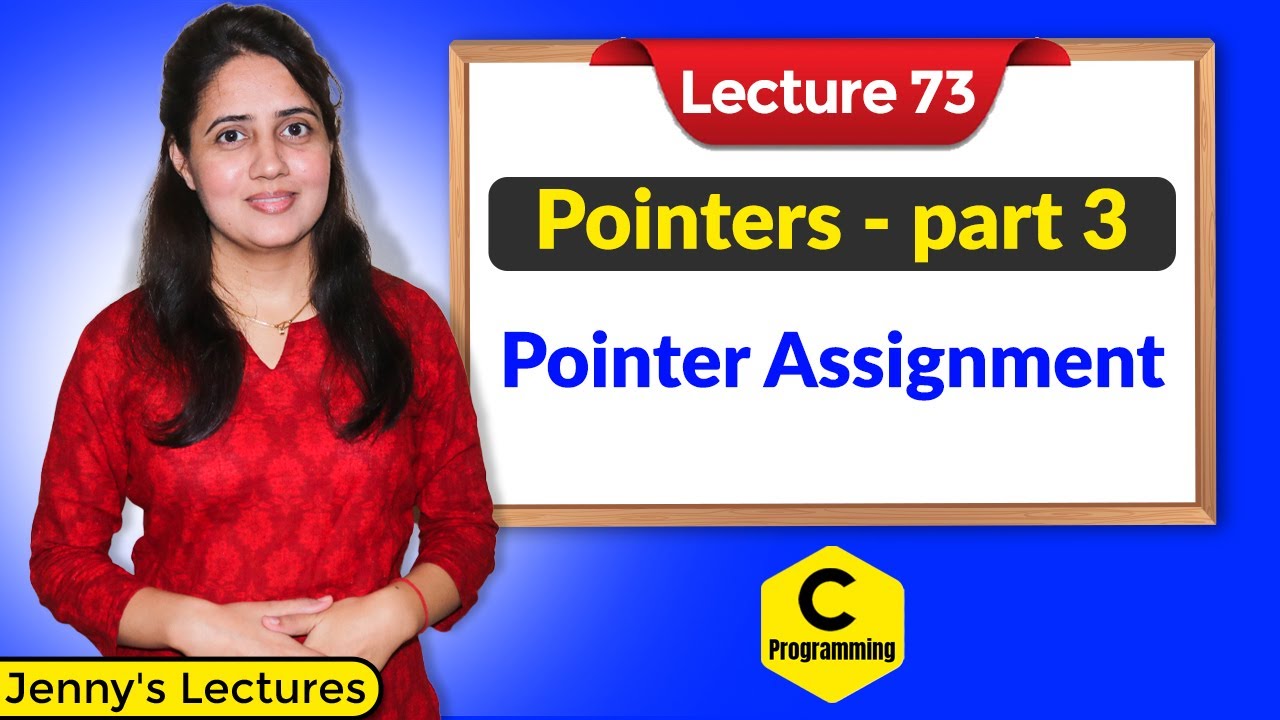
C_73 Pointers in C- part 3 | Pointer Assignment

Declaring & Initializing Pointers in C
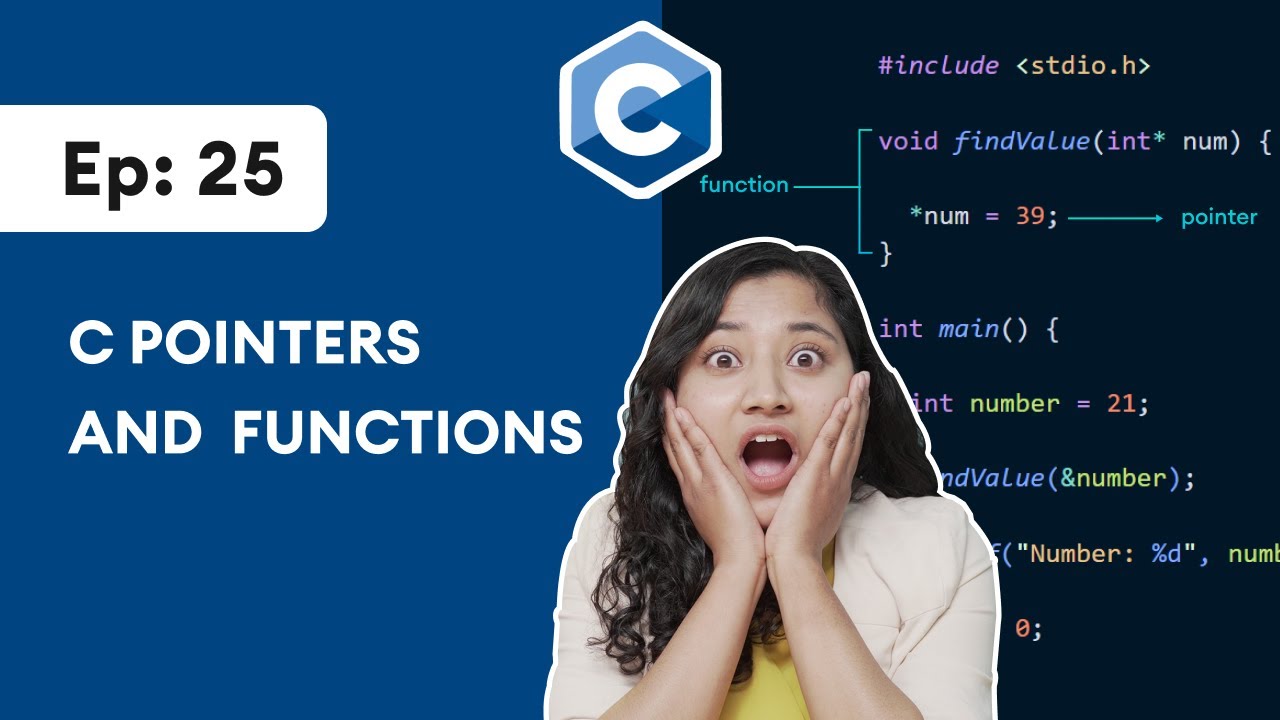
#25 C Pointers and Functions | C Programming For Beginners
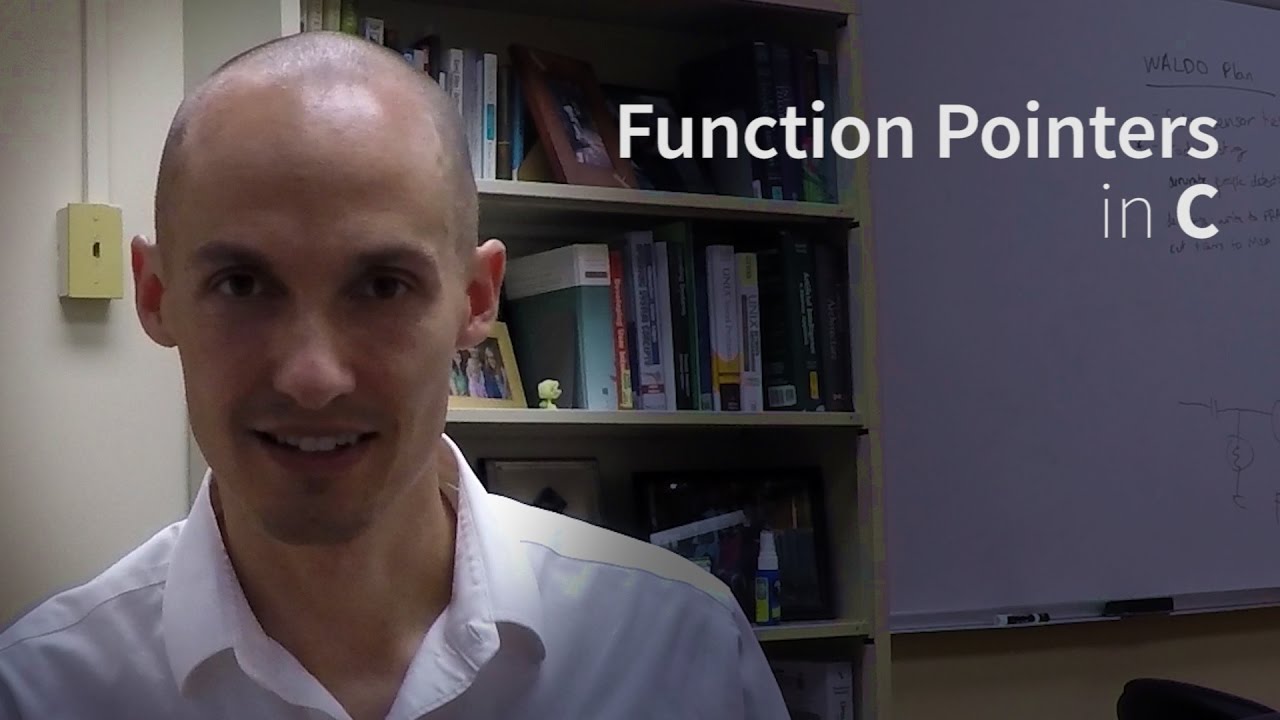
Understanding and Using Function Pointers in C

C_82 What is Dangling pointer in C | C Language Tutorials
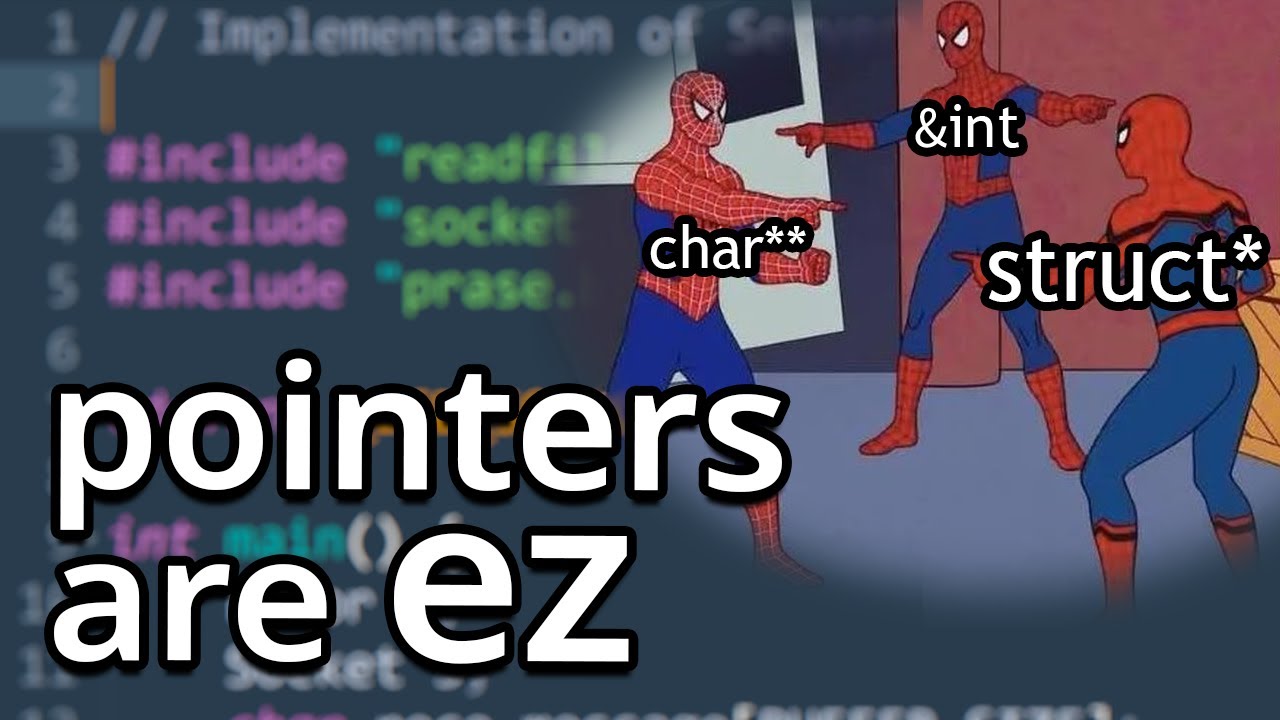
you will never ask about pointers again after watching this video
5.0 / 5 (0 votes)