Accessing Elements of a List in Python
Summary
TLDRThis educational presentation introduces the concepts of lists in Python, focusing on accessing individual elements. It covers single-dimensional lists, explaining how to access elements using their indices, including both positive and negative indexing. The presentation also delves into multi-dimensional lists, illustrating how they contain other lists and how to access their elements. Practical examples reinforce these concepts, culminating in a homework problem that encourages viewers to apply their knowledge. Overall, the session provides a clear and engaging overview of list manipulation in Python, essential for beginners.
Takeaways
- 😀 A single-dimensional list in Python is a linear collection where elements are stored one after the other.
- 😀 Each element in a single-dimensional list is accessed using a unique index, starting from 0.
- 😀 To create a list of multiples of 5 up to 20, you can use the syntax: `my_list = [5, 10, 15, 20]`.
- 😀 Accessing list elements can be done using their index, e.g., `my_list[0]` retrieves the first element (5).
- 😀 Negative indexing allows access to elements from the end of the list, where the last element has an index of -1.
- 😀 For example, to access the last element of a list using negative indexing, you would use `my_list[-1]`.
- 😀 A multi-dimensional list contains other lists as its elements, allowing for more complex data structures.
- 😀 To access elements in a multi-dimensional list, you can chain index calls, such as `my_list_2[0][0]` to get the value 1.
- 😀 Understanding the concept of negative indexing is beneficial when the length of the list is unknown.
- 😀 The lecture concludes with a homework problem asking participants to access a specific value from a provided list.
Q & A
What is a single-dimensional list in Python?
-A single-dimensional list is a list where elements are stored one after the other, allowing for indexed access to each element.
How do you create a single-dimensional list containing multiples of 5 up to 20?
-You can create the list using the syntax: `my_list = [5, 10, 15, 20]`.
What are the indices of the elements in the list [5, 10, 15, 20]?
-The indices are: 0 for 5, 1 for 10, 2 for 15, and 3 for 20.
How can you access the first element of a list in Python?
-You can access the first element by using its index, like this: `my_list[0]`.
What is negative indexing in Python lists?
-Negative indexing allows you to access elements from the end of a list, with -1 referring to the last element, -2 to the second last, and so on.
How do you access the last element of a list using negative indexing?
-To access the last element, you use the syntax: `my_list[-1]`.
What defines a multi-dimensional list in Python?
-A multi-dimensional list is a list that contains at least one other list as an element.
How do you access an element from a multi-dimensional list?
-You access an element by first specifying the index of the outer list, and then the index of the inner list. For example, `my_list_2[0][0]` accesses the first element of the first sub-list.
What is the visual representation of a multi-dimensional list?
-A multi-dimensional list can be represented as separate memory blocks for each element, where each block can contain another list, a string, or other data types.
What homework problem was presented at the end of the lecture?
-The homework problem asked how to access the value 'B' in the list `my_list_3`, with instructions to post answers in the comments section.
Outlines
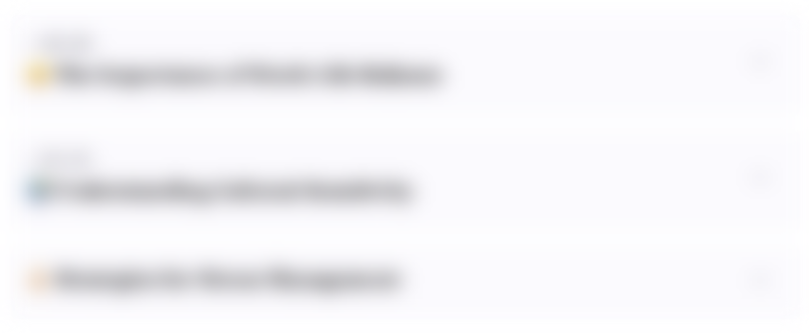
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
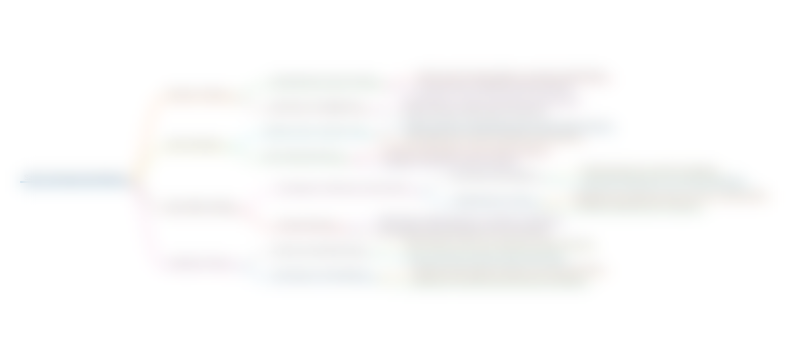
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
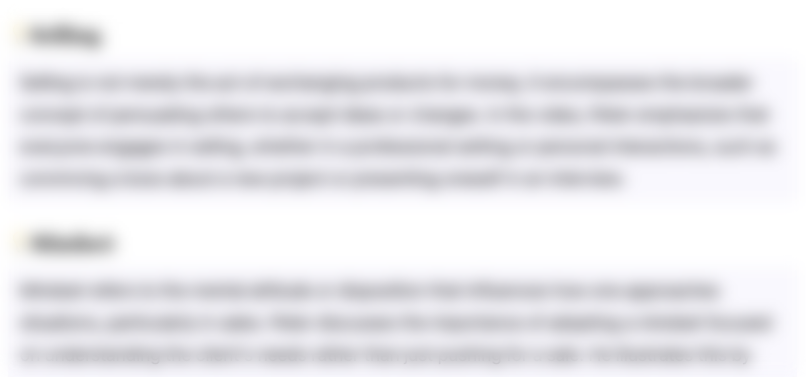
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
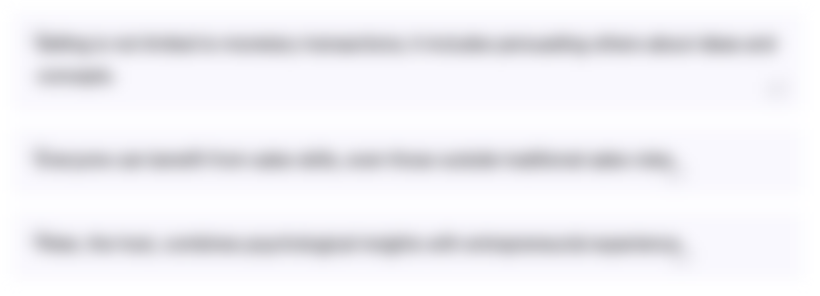
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
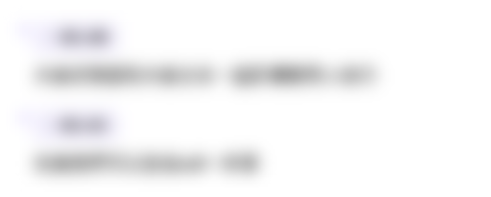
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)