Estruturas de Dados Primitivas em Python - Parte I
Summary
TLDRThis lesson introduces data structures in Python, specifically focusing on lists. It explains the importance of using lists over simple variables for handling large datasets. Key topics include creating and manipulating lists, performing operations like appending, inserting, and removing elements, and using loops to display list elements in reverse order. The script highlights the versatility of Python lists, which can hold heterogeneous data types, and the scalability they offer for solving complex problems. Overall, the lesson offers practical knowledge for efficiently utilizing lists in Python programming.
Takeaways
- π Lists in Python are flexible structures that can hold heterogeneous data types, unlike arrays in other languages, which are usually homogeneous.
- π A list can be created by enclosing elements in square brackets and can contain different data types, such as integers, strings, or objects.
- π Lists in Python are zero-indexed, meaning the first element is accessed using index 0.
- π The `len()` function is used to return the number of elements in a list.
- π Python provides the `append()` method to add elements to the end of a list, and `insert()` allows inserting elements at specific positions.
- π Lists in Python can be nested, meaning one list can contain other lists as elements, forming multi-dimensional structures.
- π Reversing a list can be done in various ways, such as using loops or built-in functions like `reverse()` and slicing.
- π Using `for` loops, you can iterate over a list to perform operations like accessing and modifying its elements.
- π The `pop()` method is used to remove and return an element from a specific position in the list, which alters the original list.
- π Python lists can also be combined or concatenated by using the `+` operator, which creates a new list with all elements from both lists.
Q & A
What is the main topic discussed in the transcript?
-The transcript primarily discusses the concept of data structures, focusing on lists, tuples, and dictionaries in Python. The lesson covers how these data structures can be used to solve various problems more efficiently than using simple variables.
How are lists different from mathematical vectors according to the script?
-In the script, it is mentioned that Python lists are heterogeneous, meaning they can contain elements of different types, whereas mathematical vectors are homogeneous, containing elements of the same type.
Why are lists in Python considered useful for large datasets, like 500,000 numbers?
-Lists in Python are useful for large datasets because they allow for dynamic and efficient data storage, unlike using a large number of individual variables. This makes it easier to manage, manipulate, and process large amounts of data.
What is the significance of the zero index in Python lists?
-In Python, lists are zero-indexed, meaning that the first element of a list is accessed by using the index 0. This is important to understand because it affects how elements are retrieved or manipulated within the list.
How can you find the length of a list in Python?
-You can find the length of a list using the `len()` function. For example, calling `len(my_list)` will return the number of elements in `my_list`.
What happens when you append an element to a list in Python?
-When you append an element to a list in Python using the `append()` method, the element is added to the end of the list.
Can Python lists contain elements of different types?
-Yes, Python lists are heterogeneous, meaning they can contain elements of various data types, such as integers, strings, and objects, in the same list.
What is the difference between the `append()` and `insert()` methods in Python lists?
-The `append()` method adds an element to the end of the list, while the `insert()` method allows you to add an element at a specific position in the list by specifying the index where it should be inserted.
How does the `reverse()` method work with lists in Python?
-The `reverse()` method in Python reverses the order of elements in a list in place, meaning it modifies the original list, arranging its elements from the last to the first.
What is the function of the `pop()` method in Python lists?
-The `pop()` method removes an element from a list at a specific position and returns the value of the removed element. If no index is specified, it removes and returns the last element of the list.
Outlines
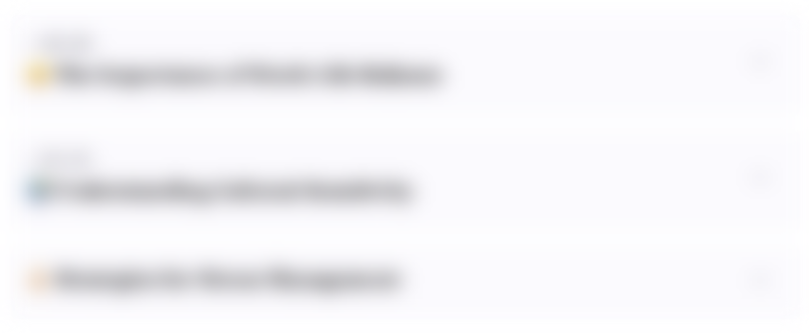
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
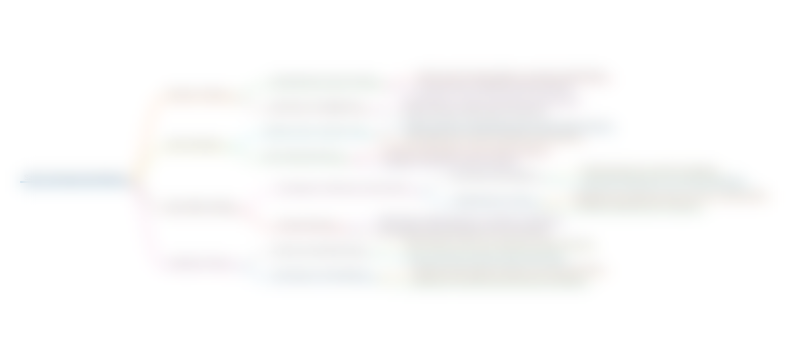
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
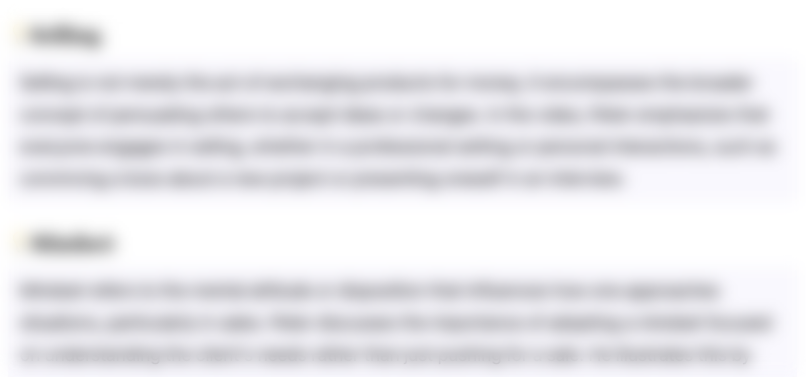
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
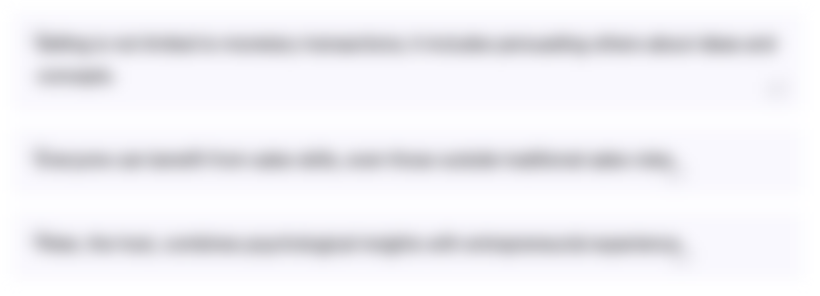
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
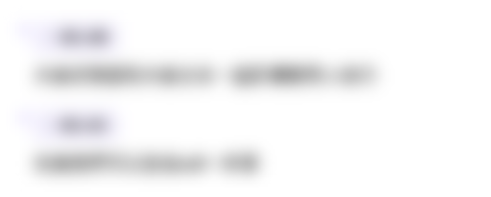
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)