GCSE Python Programming 5 - Lists/Arrays
Summary
TLDRThis Python programming lesson focuses on lists, also known as arrays, as a versatile data structure for storing sequences of items. It covers basic list operations like accessing elements using indices, modifying lists with append, remove, and insert methods, and calculating the list's length. The instructor also introduces two-dimensional lists, explaining how to create and access elements in a table-like structure. Practical examples, including handling mixed data types and calculating averages, are provided to solidify understanding. The lesson aims to prepare students for their GCSE exams and enhance their Python programming skills.
Takeaways
- π The lesson introduces lists in Python, which are similar to arrays in other programming languages but with more flexibility and power.
- π’ Lists can store multiple items of data in a single variable, making them ideal for handling series of related data.
- πΎ Lists are zero-indexed in Python, meaning the first item is accessed with index 0, which is a common practice in computer science.
- π The script demonstrates how to declare a list with square brackets and separate items with commas.
- π Accessing individual elements in a list is done using index positions within square brackets, e.g., `list_name[index]`.
- π« Attempting to access an index that is out of range will result in an error, which is a common mistake to watch out for.
- π Lists in Python can be manipulated using built-in methods like `append()`, `remove()`, and `insert()` to add or remove elements.
- π The `len()` function is used to determine the number of items in a list, which is useful for understanding the list's size.
- π The script covers how to work with two-dimensional lists, which are essentially lists of lists, and can be visualized as a table with rows and columns.
- π Two-dimensional lists are accessed by specifying the row and column indices, and care must be taken to ensure the indices are within the correct range.
- π The lesson also touches on practical applications, such as using lists to store and manipulate data like scores and names, and calculating averages.
Q & A
What is the main topic of this Python programming lesson?
-The main topic of this lesson is learning about lists and how to manipulate them in the Python programming language.
Why does the instructor mention arrays at the beginning of the lesson?
-The instructor mentions arrays because the GCSE exam syllabus refers to arrays, but Python does not have arrays in the traditional sense; it has lists, which are more advanced and will be used as arrays for the purposes of the exam.
What is the syntax for declaring a list in Python?
-The syntax for declaring a list in Python involves using square brackets [] to enclose the list items, with each item separated by commas.
How does Python handle index positions in lists?
-Python handles index positions in lists starting at 0, which is the first position in the list.
What is the purpose of the 'append' method in lists?
-The 'append' method is used to add an item to the end of a list without removing any existing items.
What does the 'remove' method do in a Python list?
-The 'remove' method is used to delete a specific item from a list.
What is the function to determine the length of a list in Python?
-The 'len' function is used to determine the length, or the number of items, in a Python list.
How can you access an element in a two-dimensional list?
-To access an element in a two-dimensional list, you provide the row and column indices in the format list_name[row_index][column_index].
What is a common mistake made when trying to access elements in a list?
-A common mistake is trying to access an index that is out of range, such as attempting to access an index equal to the length of the list, which will result in an error.
How can you print the names and scores of individuals whose scores are above the average in a list?
-You calculate the average score, then use separate 'if' statements to check if each score is greater than the average, and if so, print the corresponding name and score.
What is the concept of a two-dimensional list or array in Python?
-A two-dimensional list or array in Python is a list that contains other lists, essentially creating a table-like structure with rows and columns.
Outlines
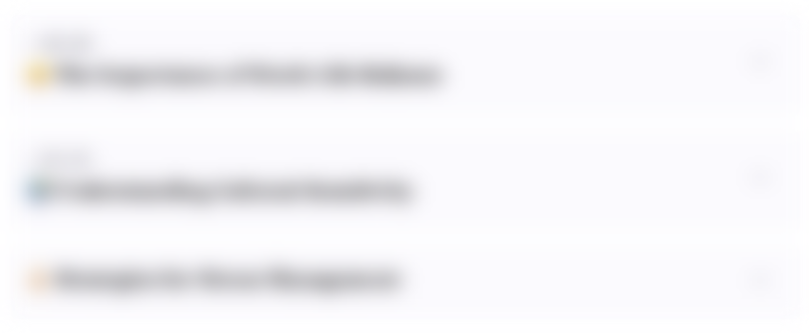
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
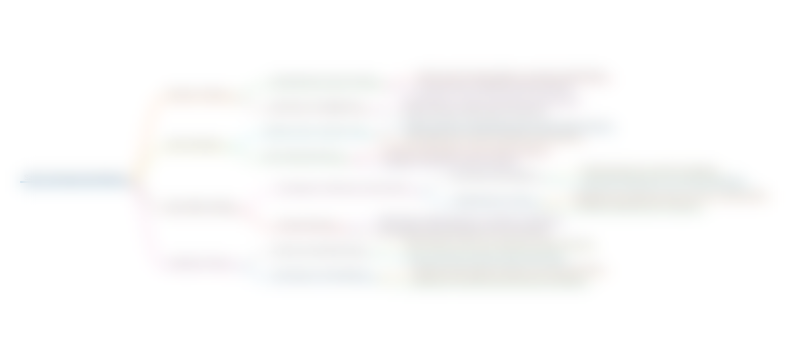
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
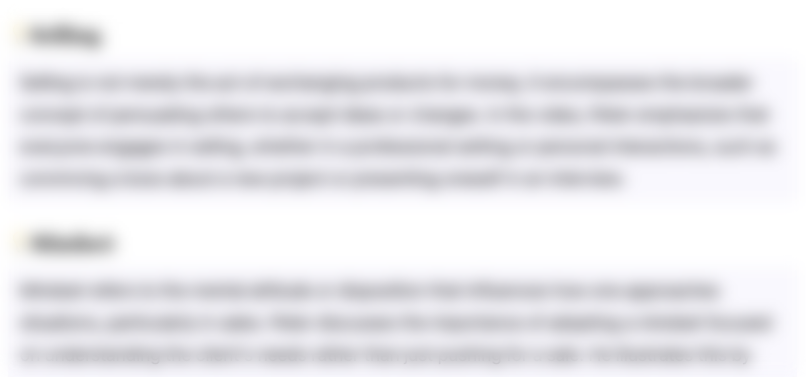
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
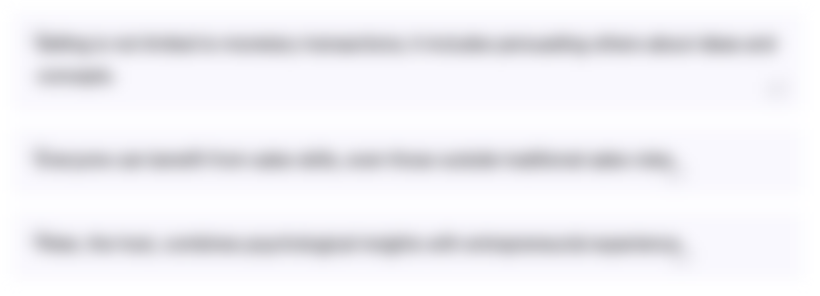
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
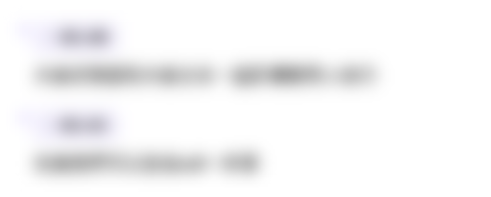
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)