Classes and Objects
Summary
TLDRThis lecture introduces Python classes and objects, explaining how classes act as blueprints for objects. The instructor highlights how objects combine attributes (variables) and behaviors (functions), with a focus on ensuring uniformity while maintaining individual identity. Examples demonstrate how students in a dataset can be modeled as objects with attributes like 'roll number' and 'name.' The concept of constructors is introduced, which are special functions that help create objects. The lecture concludes with a promise to explore behaviors (functions) in the next session, building on this foundation of attributes.
Takeaways
- 📚 The lecture explained the difference between attributes (variables) and behavior (functions) in Python classes.
- 🏗️ An object is described as a combination of attributes and behavior, which represents its identity.
- ❌ The issue of inconsistency arises when different objects (students) have varying attributes, causing potential problems during computations.
- 🏠 A class is compared to a blueprint used to construct uniform objects, allowing for individual identity while maintaining predefined attributes.
- 🖥️ The 'class' keyword is used to define a class, and it is a common practice to capitalize the first letter of the class name.
- 🔧 The constructor function, a special function with the same name as the class, is automatically created by Python to initialize objects.
- 🔗 The dot operator (.) is used to access an object's attributes and behavior, allowing individual values for each object to be modified.
- 🆔 Each object has its own identity, and attributes can be the same across objects but with different values, ensuring flexibility.
- 🤖 The lecture demonstrates how objects can have default values, like 'None,' which can be changed later for individual objects.
- ⚙️ The next part of the lecture will cover the 'behavior' aspect, focusing on functions, to enhance the class and object structure.
Q & A
What are attributes in Python classes, as described in the lecture?
-Attributes in Python classes are variables that store data relevant to the object. For example, in the 'Student' class, attributes like 'roll number' and 'name' are used to store data specific to each student object.
What is meant by behavior in the context of Python classes?
-Behavior in Python classes refers to functions that perform operations or actions. These functions are defined inside a class and can operate on the attributes of the class to modify or use the object's data.
What is the problem with missing or extra attributes in different objects of the same class?
-If objects of the same class have missing or extra attributes, it can cause issues in uniformity and data integrity. For example, computations involving these objects may fail or produce incorrect results if some objects lack required attributes.
How does the concept of a 'class' help maintain uniformity across objects?
-A class acts as a blueprint that defines a set of common attributes and behaviors for its objects. This ensures that all objects created from the class share these predefined attributes and behaviors, maintaining uniformity while allowing individual identity through varying values.
What is the purpose of a constructor in Python, and how is it automatically created?
-A constructor in Python is a special function that is automatically created when a class is defined. It is used to initialize objects. Python adds a default constructor with the same name as the class to allow object creation without explicitly defining it.
How are attributes accessed and modified for an object in Python?
-Attributes of an object are accessed and modified using the dot operator (.). For example, 'S0.roll_number = 0' sets the 'roll_number' attribute of object 'S0' to 0. Similarly, 'print(S0.name)' accesses and prints the 'name' attribute.
What happens if an object is created but its attributes are not explicitly initialized?
-If an object is created but its attributes are not initialized, the attributes will have their default values as defined in the class. For example, if 'roll_number' and 'name' are not set, they will hold the value 'None' by default.
How does object identity work in Python classes, and why is it important?
-Each object in a Python class has its own identity, meaning that even if multiple objects share the same attributes, their values can be different for each object. This allows for flexibility and individuality, where each object can represent a unique entity while still following the class's structure.
Can an object in Python have some attributes uninitialized at the time of creation? How is this handled?
-Yes, an object in Python can have some attributes uninitialized at the time of creation. These attributes will retain their default value (e.g., 'None') until explicitly set later. For example, a new student may not have a roll number initially but can be assigned one later.
What will the lecture cover in the next session, according to the script?
-The next session will cover the second part of object-oriented programming, which involves adding behavior (functions) to the classes along with the attributes. This will complete the understanding of both attributes and behavior in Python classes.
Outlines
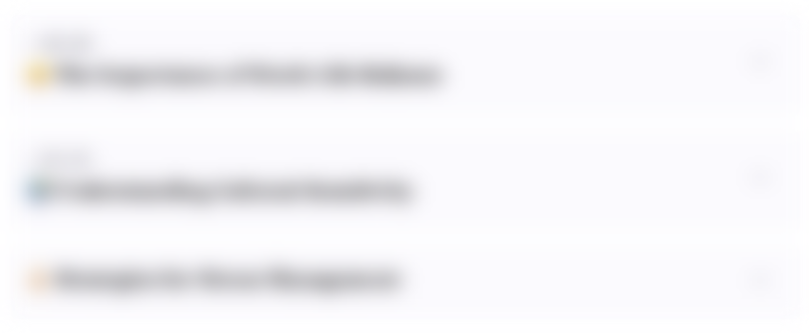
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
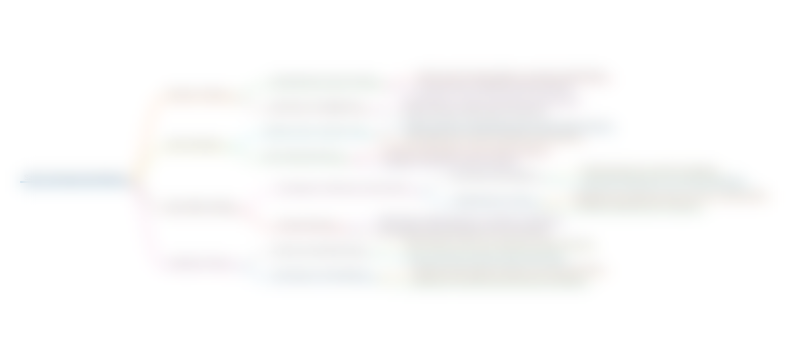
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
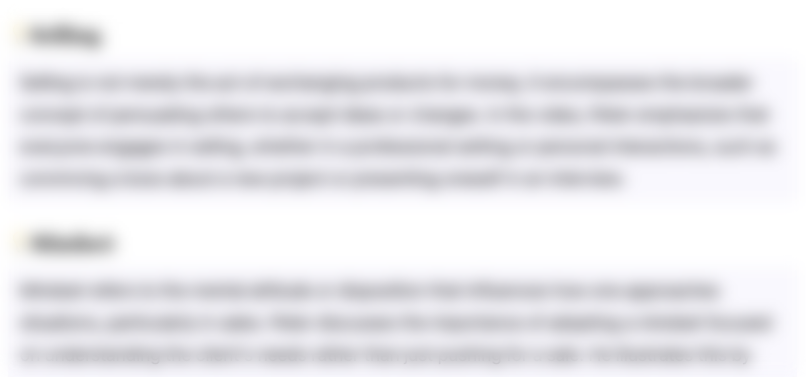
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
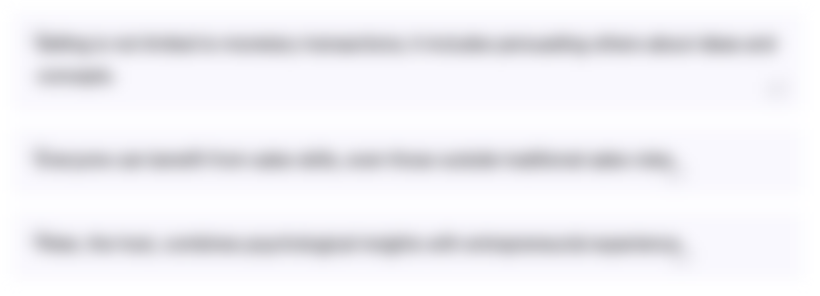
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
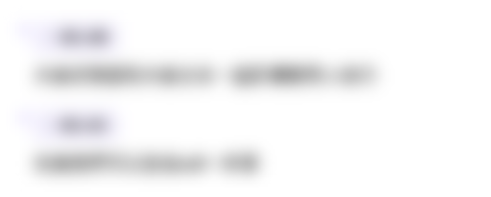
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
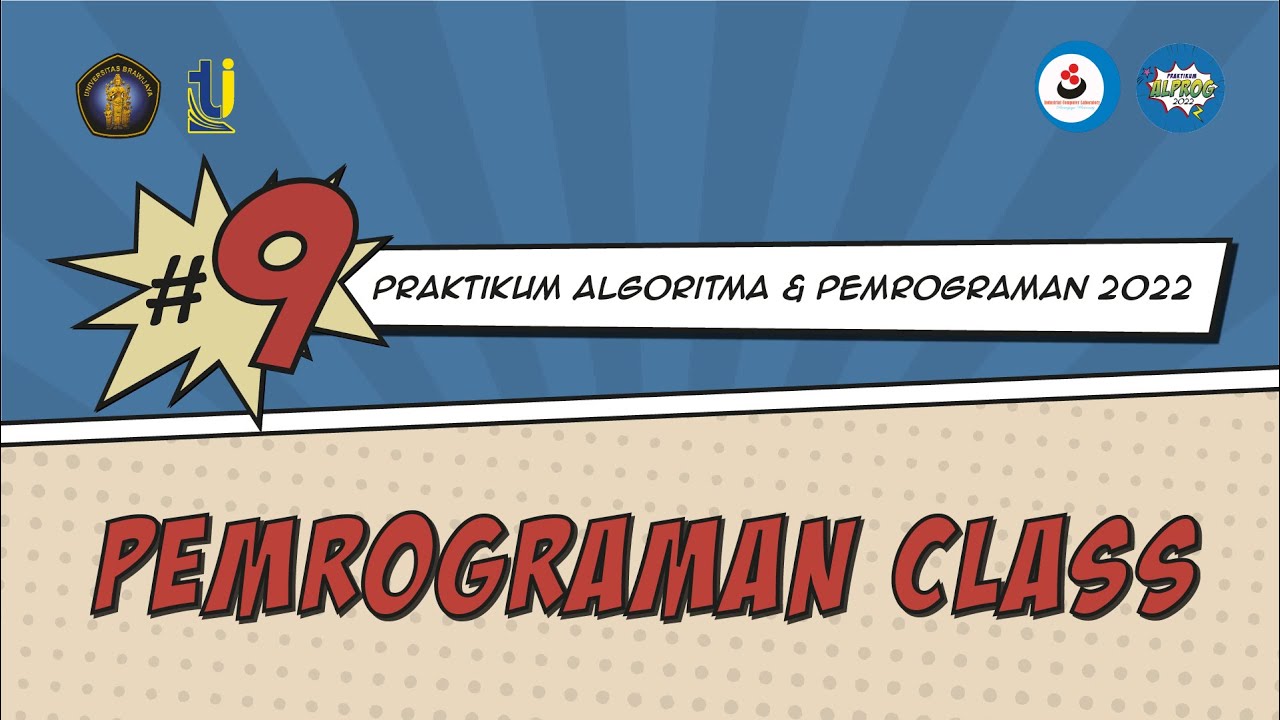
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
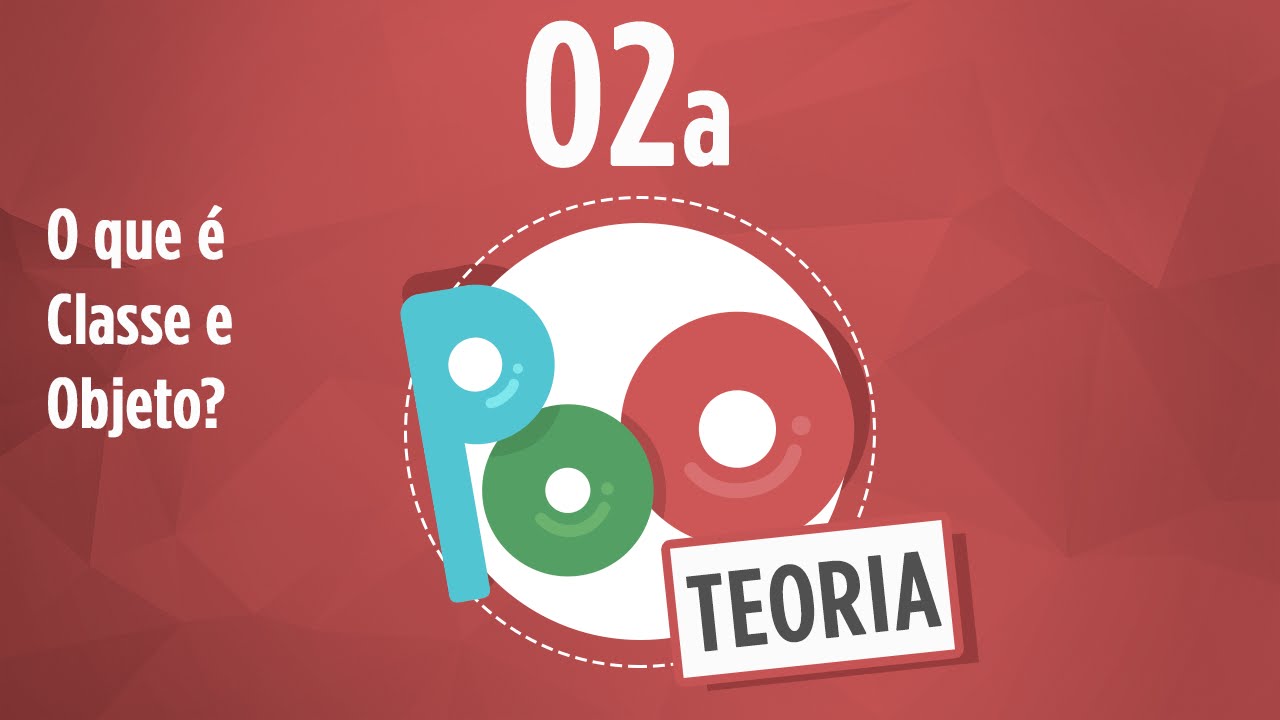
Curso POO Teoria #02a - O que é um Objeto?

Learn Python OOP in under 20 Minutes
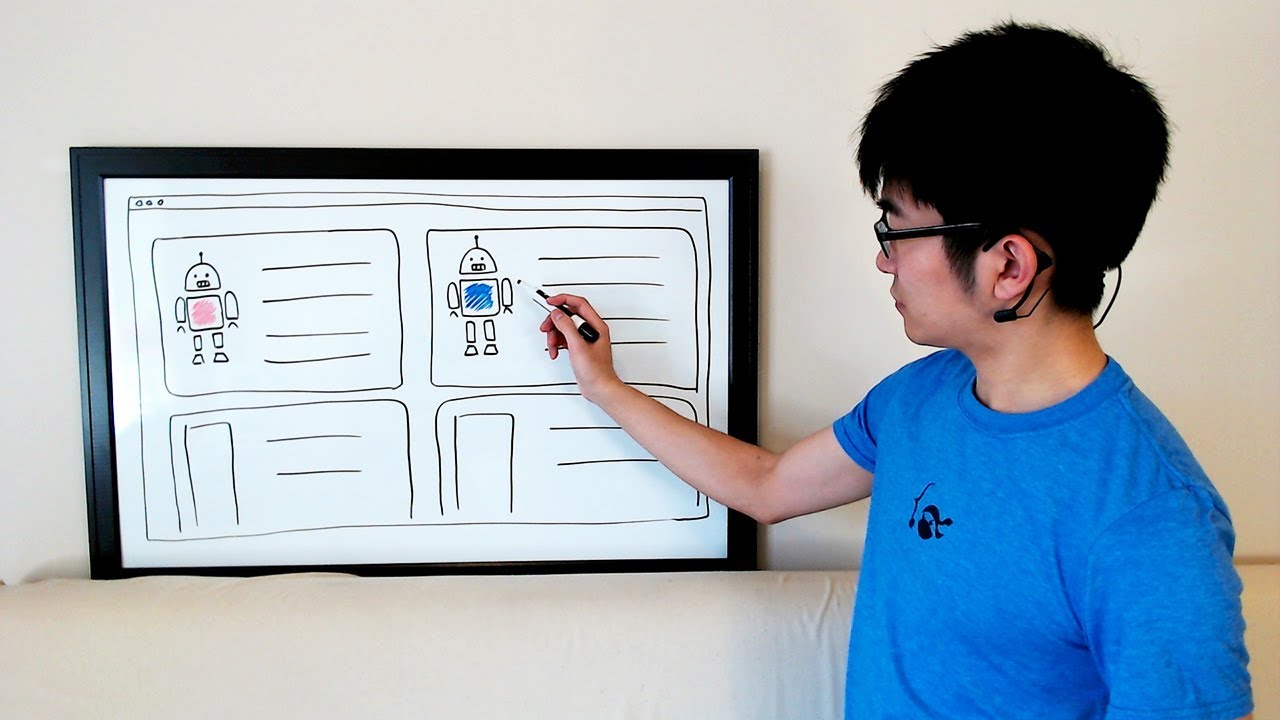
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3)
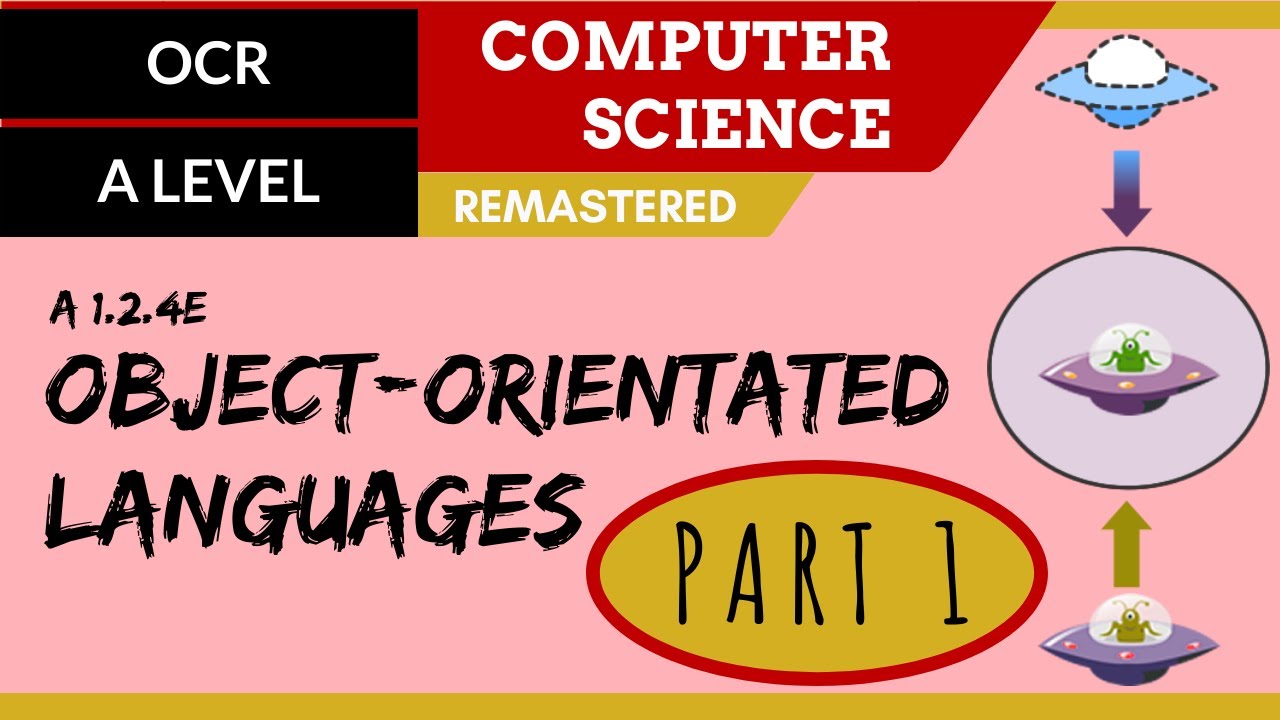
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1
5.0 / 5 (0 votes)