9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022
Summary
TLDRThis video explains the fundamentals of Python programming, focusing on classes and instances. The presenter introduces classes as blueprints for objects, outlining key concepts like attributes, methods, and constructors. The video also explores inheritance, method overriding, and access modifiers, demonstrating how to structure Python programs with object-oriented principles. Practical examples are provided, including class inheritance with student and worker examples, as well as method overriding in a vehicle class. The content is aimed at helping viewers understand how to effectively use Python to create and manage classes and objects, along with best practices for coding.
Takeaways
- 😀 A class in Python is a blueprint or template for creating objects (instances), and an instance is the actual object created from that class.
- 😀 Attributes in a class are variables that hold data related to the instance, and they are accessible using the dot operator.
- 😀 A constructor function (such as __init__) is used to initialize attributes when creating an object, and it is automatically called when an object is instantiated.
- 😀 Inheritance in Python allows one class (the child class) to inherit attributes and methods from another class (the parent class).
- 😀 The super() function in inheritance allows the child class to call methods or constructors from the parent class.
- 😀 Polymorphism allows methods in different classes to have the same name but behave differently depending on the class of the object calling them.
- 😀 Access modifiers in Python (private, public, protected) control the visibility and accessibility of attributes and methods in a class.
- 😀 Private attributes are accessible only within the class itself, while public attributes can be accessed both inside and outside the class.
- 😀 Protected attributes can be accessed within the class and its subclasses (child classes), but not from outside the class directly.
- 😀 The script illustrates the creation of different classes (e.g., Baby, Worker, Student) and how inheritance, attributes, and methods are applied to them.
- 😀 The video concludes by encouraging viewers to reach out to teaching assistants for further questions and provides resources for additional study.
Q & A
What is a class in Python, and what role does it play in object-oriented programming?
-A class in Python is a blueprint or template for creating objects (instances). It defines the properties (attributes) and behaviors (methods) that the objects created from the class will have. It essentially structures how objects should look and act.
What is the difference between a class and an object in Python?
-A class is a definition or blueprint, while an object (or instance) is a concrete realization of that blueprint. The object is an actual instance created based on the class, with specific values assigned to its attributes.
How are attributes defined and accessed in Python classes?
-Attributes are variables defined within a class. They can be accessed using the dot operator on an instance of the class, like `instance.attribute`. Attributes are usually public and can be accessed or modified directly.
What is the purpose of the constructor (often defined as `__init__` in Python) in a class?
-The constructor method (`__init__`) is used to initialize the attributes of a newly created object. It is called automatically when a new instance of the class is created, and it assigns values to the object's properties.
What is inheritance in Python, and how does it work?
-Inheritance allows one class (a subclass or child class) to inherit the properties and behaviors of another class (a parent or base class). This enables code reuse and the creation of hierarchical class structures. The child class can also override or extend the behavior of the parent class.
What is the role of the `super()` function in Python inheritance?
-The `super()` function is used to call methods from a parent class, allowing a subclass to invoke or extend the behavior of the parent class. It is often used to call the parent class's constructor in a subclass to ensure that the base class is properly initialized.
What is method overriding in Python, and how is it demonstrated?
-Method overriding occurs when a subclass defines a method with the same name as a method in the parent class. The subclass's version of the method is called instead of the parent's. This allows subclasses to provide their own specific implementation of a method.
Can you explain the concept of access modifiers in Python classes?
-Access modifiers in Python control the visibility of class variables and methods. There are three main types: `public` (accessible from anywhere), `private` (accessible only within the class), and `protected` (accessible within the class and its subclasses).
What does the term 'polymorphism' mean in the context of object-oriented programming?
-Polymorphism refers to the ability of different objects to respond to the same method call in different ways. In Python, this is commonly achieved through method overriding, where subclasses define their own specific behavior for inherited methods.
How would you create an instance of a class in Python?
-An instance of a class is created by calling the class name with appropriate arguments, if necessary, like `my_object = MyClass(arg1, arg2)`. This triggers the `__init__` constructor to initialize the object with the given values.
Outlines
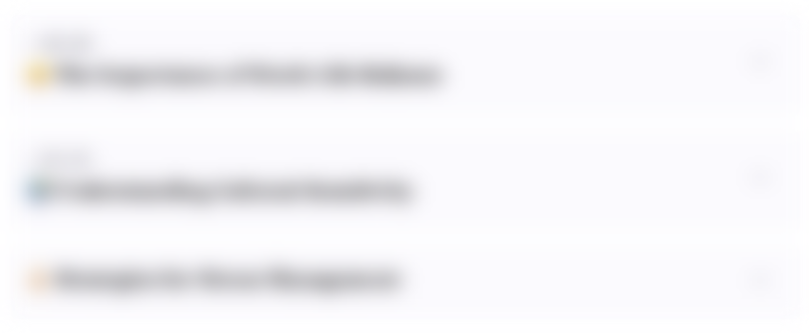
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
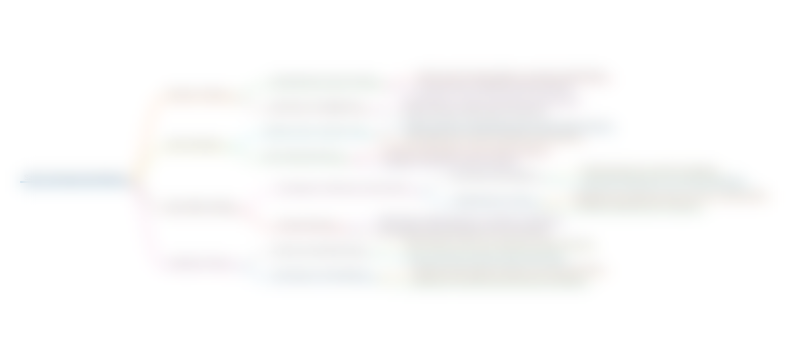
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
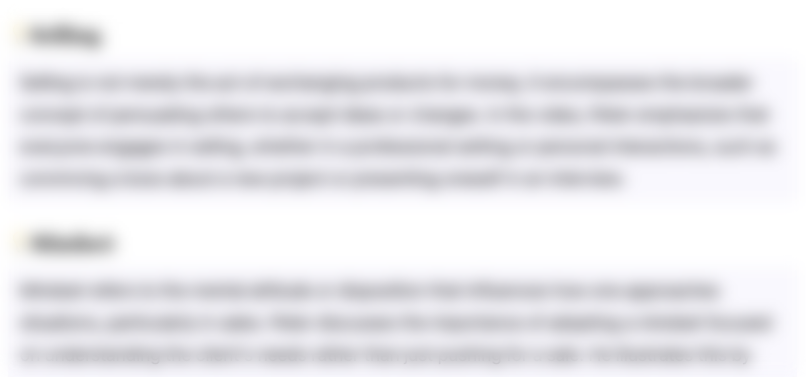
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
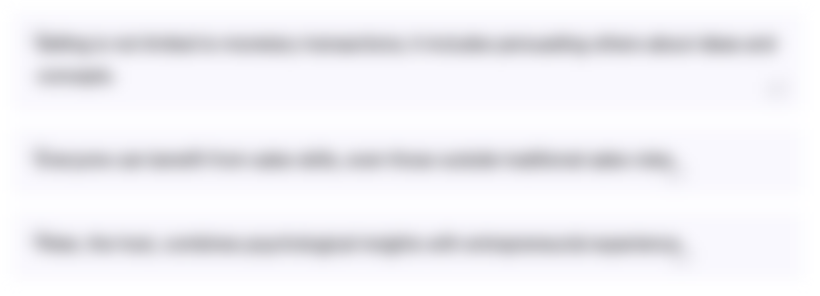
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
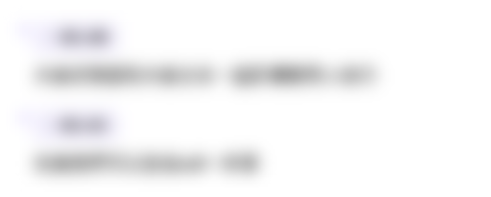
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
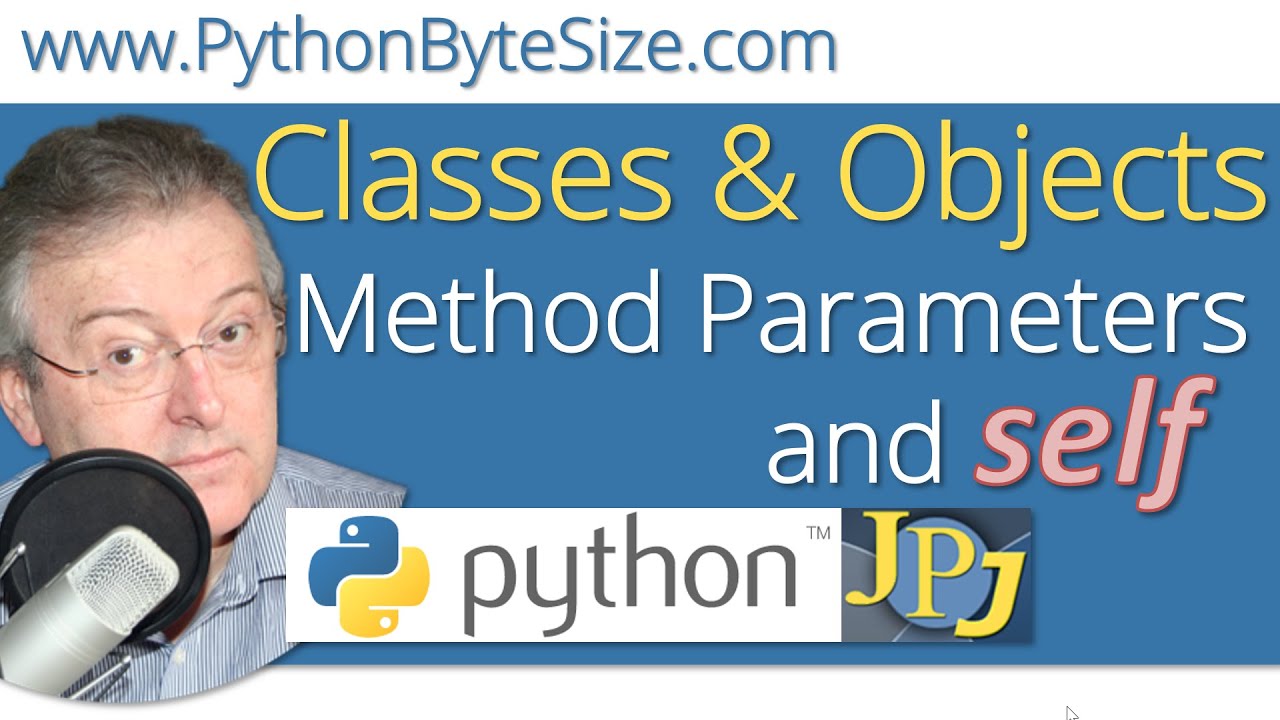
Python Method Parameters and self
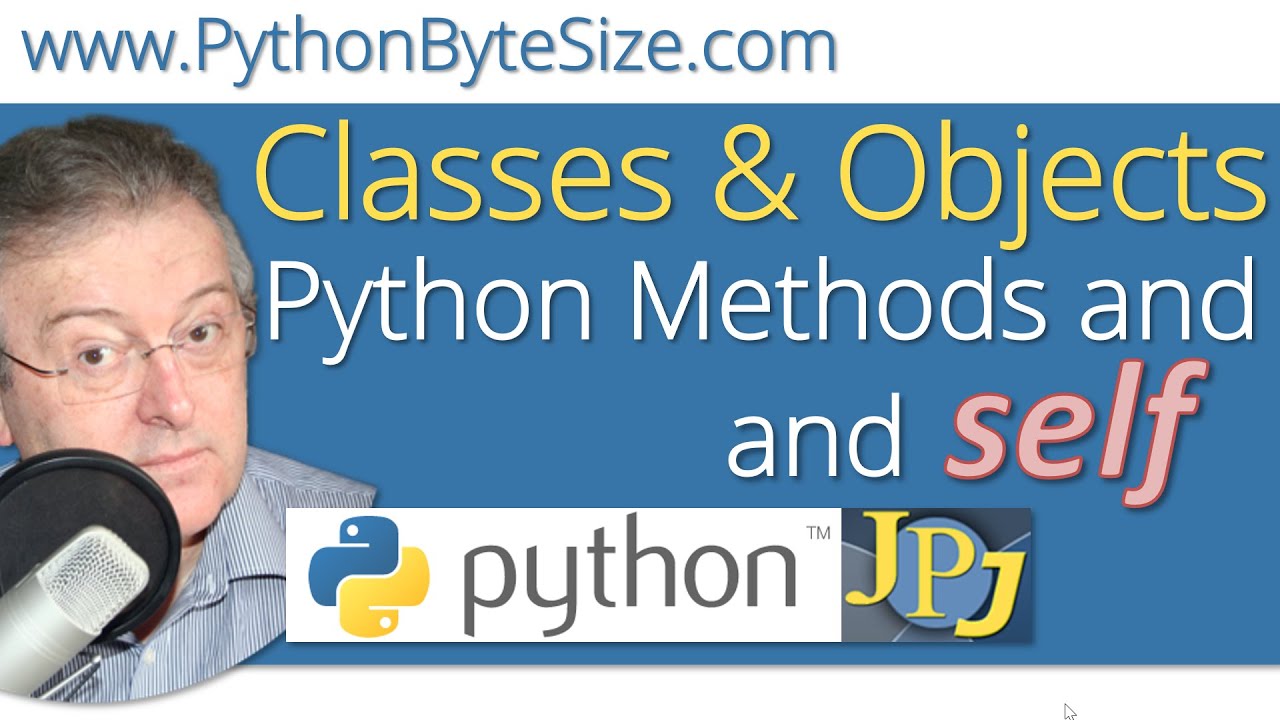
Python Methods and self

Python OOP Tutorial 1: Classes and Instances
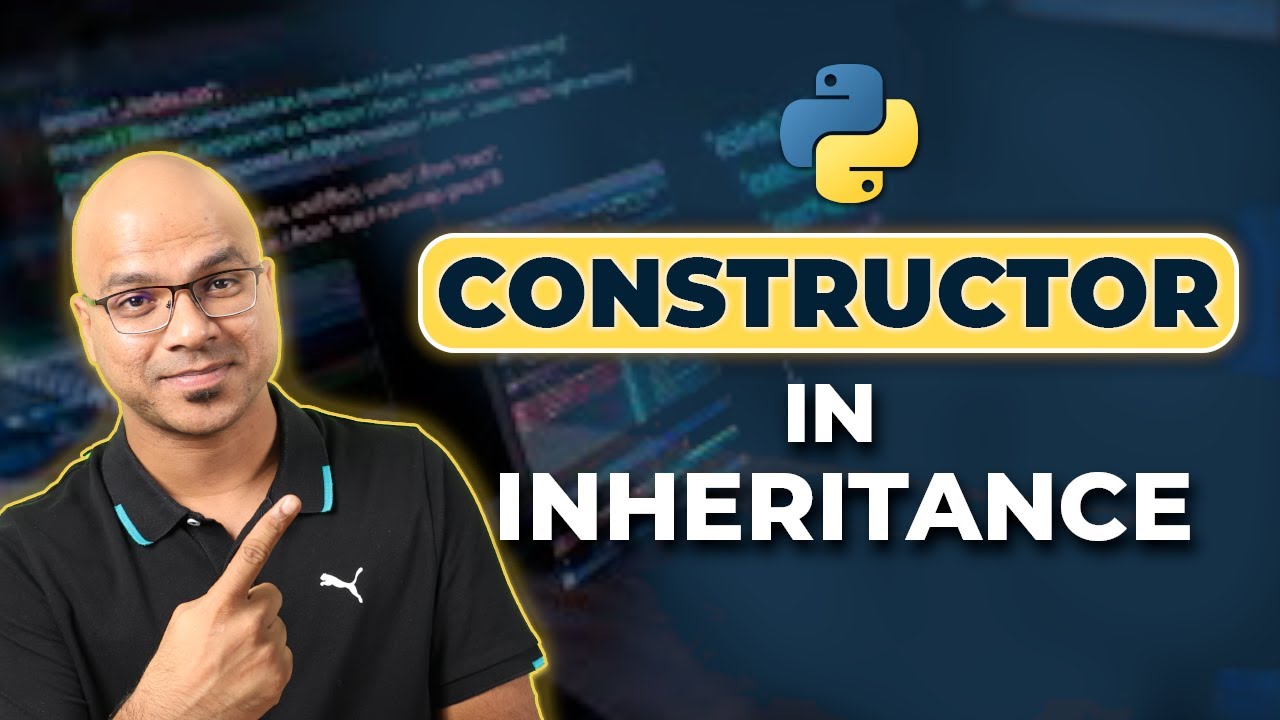
#56 Python Tutorial for Beginners | Constructor in Inheritance
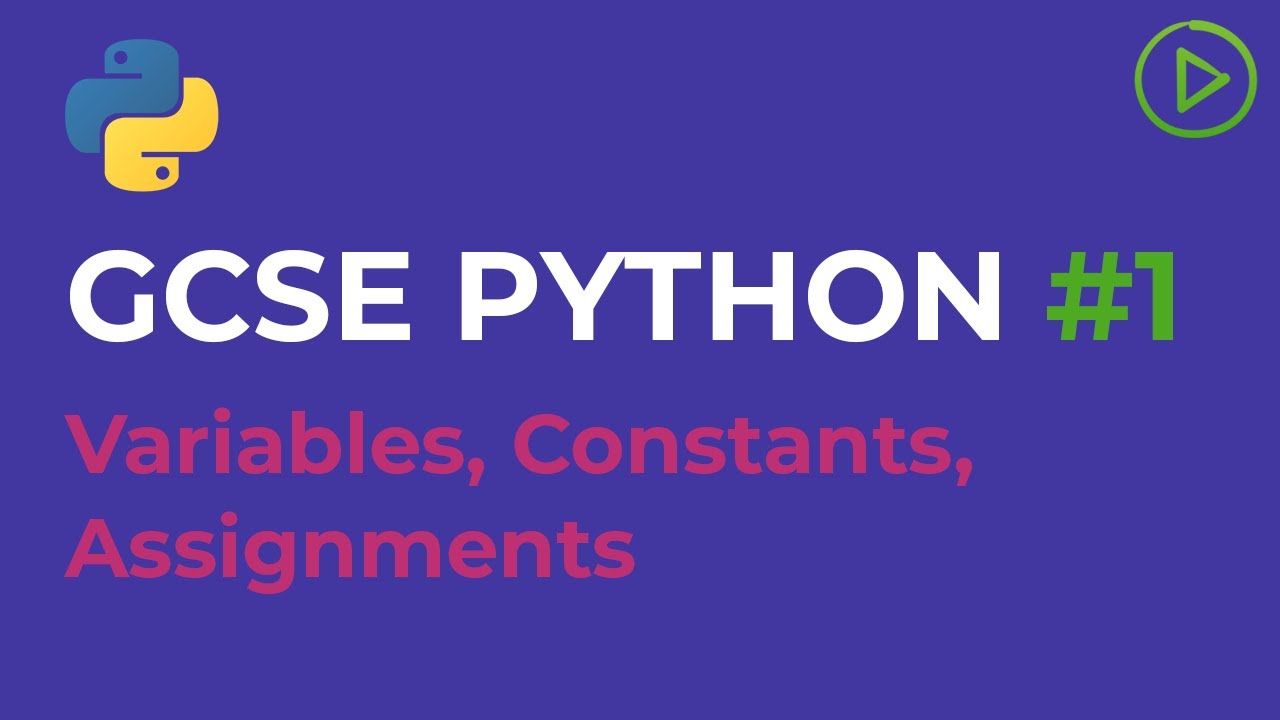
GCSE Computer Science Python #1 - Variables, Constants and Assignments
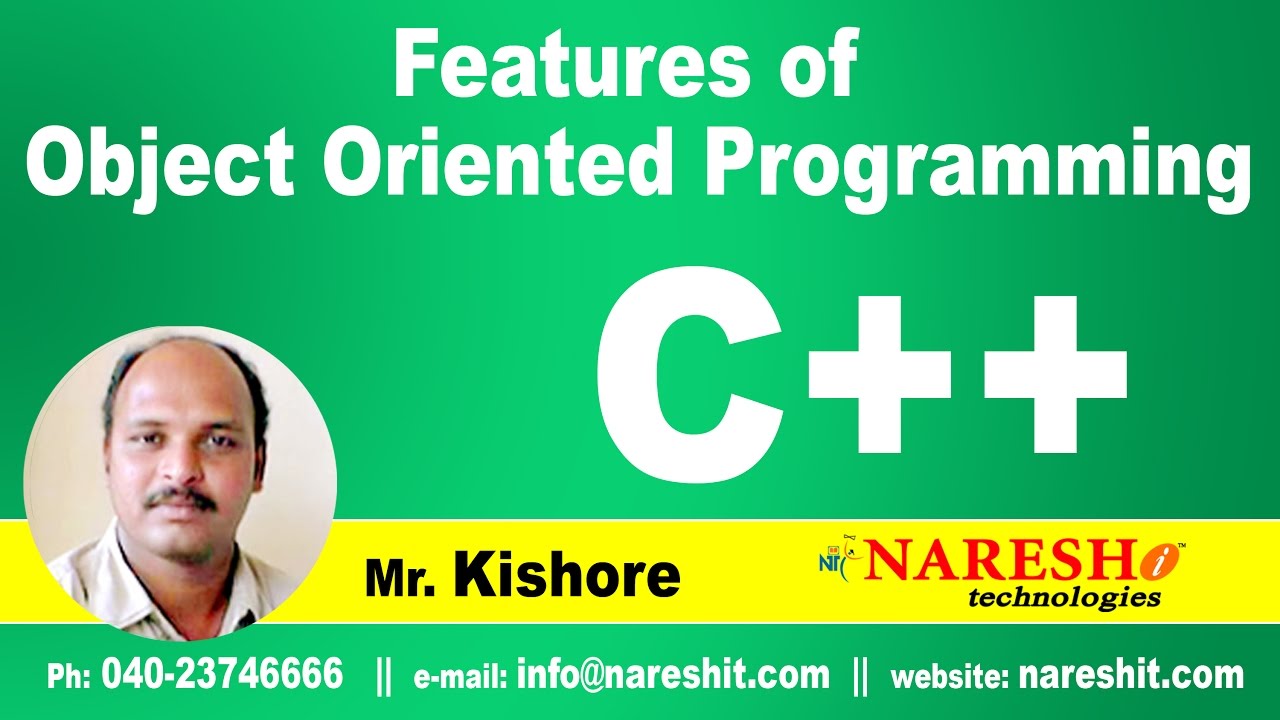
Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore
5.0 / 5 (0 votes)