Learn C# Scripting for Unity 15 Minutes - Part 4 - Foreach Loop, Array, Find Tags
Summary
TLDRIn this Unity tutorial, Raja from Charger Games walks through C# scripting for object manipulation. The video focuses on finding game objects by tag, specifically cubes and spheres, and demonstrates how to destroy or interact with them. The tutorial covers using arrays to store multiple objects, utilizing loops for bulk operations, and implementing actions via input keys. Additionally, Raja explores how to selectively destroy objects by tags and customize interactions, such as color changes upon clicking. This video is a practical guide for game developers looking to enhance their Unity projects with C# scripting.
Takeaways
- 😀 Learn how to find and manipulate game objects in Unity using C# scripting.
- 📦 A 3D cube object is created, positioned, and a material is applied to it.
- 🔍 Game objects can be located by tag using the method `GameObject.FindWithTag` in Unity scripts.
- 🧠 Tags can be added to game objects for easier identification and interaction through scripts.
- 💥 Game objects, like the cube, can be destroyed using the `Destroy()` method after being found by tag.
- 💡 Multiple game objects can be stored in an array and managed together using `FindGameObjectsWithTag`.
- 🔄 The `foreach` loop is used to iterate through arrays of game objects, applying actions such as destruction.
- 🕹 Actions like destroying objects can be triggered by user input, such as pressing the spacebar or specific keys.
- 🎮 A new script can allow the destruction of objects when clicked, filtering based on their tag (e.g., only cubes or spheres).
- 🎨 Scripts can be applied to multiple game objects, enabling different actions depending on the object's tag, like changing color or destruction.
Q & A
What is the purpose of the video tutorial?
-The purpose of the video tutorial is to teach how to script in C# for Unity, specifically focusing on how to search for and manipulate game objects within a Unity scene.
How does the script find a specific game object in the scene?
-The script finds a specific game object by using `GameObject.FindWithTag` and specifying the tag of the game object, like 'cube' or 'sphere'. This allows the script to locate and interact with the object.
Why do you need to use tags for game objects in Unity?
-Tags are used to categorize game objects, making it easier to identify and find multiple objects of the same type, such as all cubes or spheres. This approach is useful when you need to perform actions on a group of objects.
How can you assign a tag to a game object in Unity?
-To assign a tag, select the game object, go to the 'Tag' drop-down menu, and either choose an existing tag or create a new one by clicking on 'Add Tag' and saving it.
What is the benefit of using arrays in the script when finding multiple game objects?
-Arrays are useful for storing multiple game objects with the same tag. This allows the script to reference and manipulate all of them collectively, such as destroying all cubes or spheres in a scene.
How does the script destroy multiple game objects at once?
-The script uses a `for each` loop to iterate through the array of game objects found with a specific tag, such as cubes, and destroys each one sequentially using the `Destroy()` function.
What is the purpose of using the `Input.GetKeyDown` method in the script?
-The `Input.GetKeyDown` method is used to detect key presses. In the tutorial, pressing specific keys like 'space' or 'S' triggers the destruction of either cubes or spheres in the scene.
What is the difference between `GameObject.FindWithTag` and `GameObject.FindGameObjectsWithTag`?
-`GameObject.FindWithTag` finds and returns a single game object with the specified tag, while `GameObject.FindGameObjectsWithTag` returns an array of all game objects with that tag, allowing for batch operations like destroying or modifying them.
How does the script differentiate between game objects when destroying them?
-The script differentiates between game objects based on their tags. It checks the tag of the object and only destroys those with a specific tag, such as 'cube' or 'sphere'.
How can the `OnMouseDown` method be used in Unity scripting?
-The `OnMouseDown` method is called when the user clicks on a game object. In the tutorial, it is used to destroy an object when clicked, but only if the object's tag matches a specified condition, such as 'cube' or 'sphere'.
Outlines
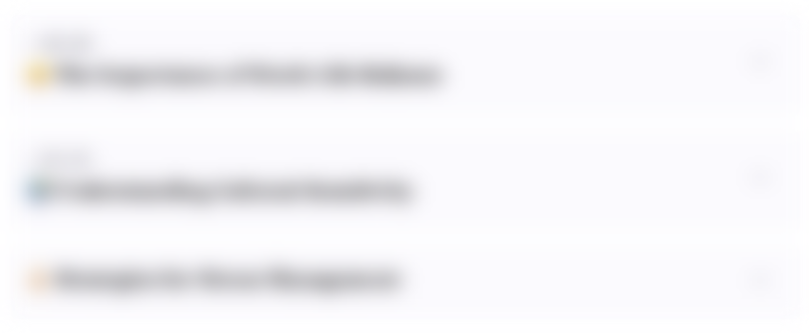
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
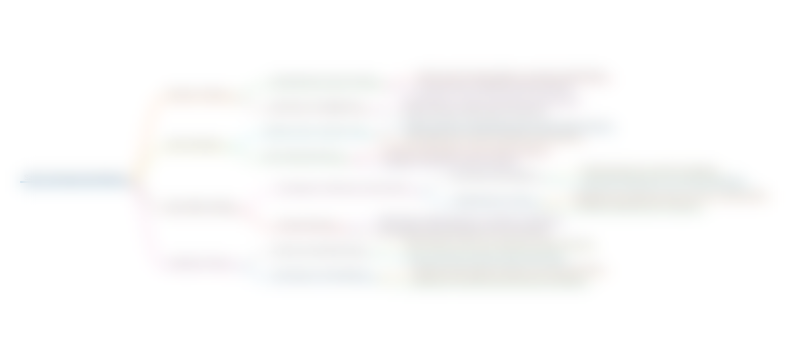
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
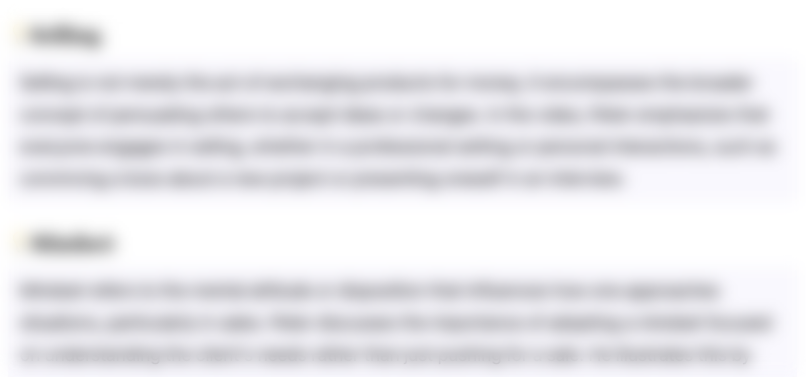
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
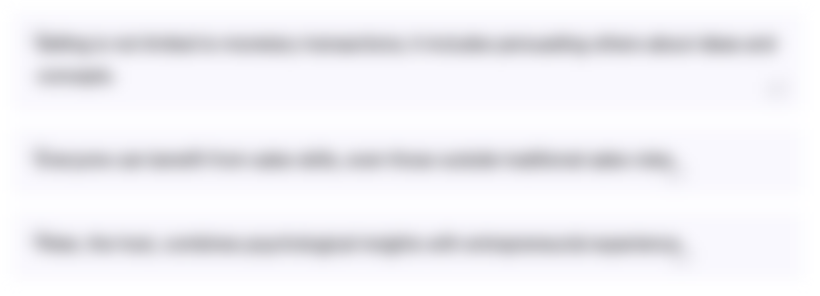
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
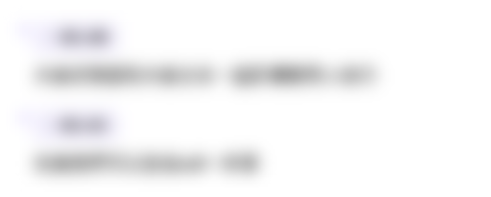
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)