#1 Introduction to Lists | List Manipulation | Class 11 CBSE Computer Science and IP
Summary
TLDRThis video provides a comprehensive introduction to Python's list data type, covering fundamental concepts like mutability, indexing, and list traversal. The presenter explains how lists can store elements of various data types, both individually and in combination. Through examples, viewers learn how to access and modify list elements, use both forward and backward indexing, and accept user input for lists. The video also demonstrates how to traverse lists using loops to access each element. With practical programming insights, this tutorial is ideal for beginners looking to deepen their understanding of lists in Python.
Takeaways
- 😀 A list in Python is an immutable collection of elements, meaning the elements can be modified after the list is created.
- 😀 Lists can store elements of any data type, including a mix of different data types (e.g., integers, strings, floats).
- 😀 Lists are enclosed in square brackets, and can be created using the syntax: `my_list = [element1, element2, element3]`.
- 😀 Lists are **mutable**, meaning their elements can be modified after creation, such as changing a value at a specific index.
- 😀 You can create an empty list using an empty square bracket: `empty_list = []`.
- 😀 Indexing in lists starts at 0 for forward indexing and -1 for backward indexing. You can use both to access elements.
- 😀 Forward indexing starts from 0, with each subsequent element getting a higher index (e.g., `my_list[0]`, `my_list[1]`).
- 😀 Backward indexing starts from -1, where the last element is accessed with `my_list[-1]`, the second last with `my_list[-2]`, etc.
- 😀 The `eval(input())` function can be used to accept a list from the user in Python. For example: `user_list = eval(input('Enter a list: '))`.
- 😀 Traversing a list refers to accessing each element one by one, typically using a loop such as `for item in my_list`.
- 😀 Lists in Python are mutable, which means you can modify their elements, and the memory location (ID) remains the same after modification.
Q & A
What is a list in Python?
-A list in Python is an ordered collection of elements that can store items of any data type. It is mutable, meaning its elements can be changed after creation. Lists are enclosed in square brackets, and elements are separated by commas.
What does 'mutable' mean in the context of Python lists?
-Mutable means that the elements of a list can be changed after the list has been created. You can modify, add, or remove elements from a list without creating a new list.
Can a Python list store different types of data?
-Yes, a Python list can store elements of different data types. For example, a list can contain integers, strings, and floating-point numbers together.
How can you identify if an object is a list in Python?
-You can identify a list by checking if it is enclosed within square brackets (`[]`). Lists are also the only objects in Python that can store multiple elements, including elements of different data types.
What is the difference between forward and backward indexing in Python lists?
-Forward indexing starts from 0 and goes up to the length of the list minus 1. Backward indexing starts from -1, which refers to the last element of the list, and goes in reverse towards -n (the first element).
How do you access elements in a Python list?
-Elements in a Python list are accessed using their index. You can access an element by specifying the list name followed by the index inside square brackets (e.g., `list[1]` to access the second element).
What happens when you modify an element in a list? Does it change the list’s ID?
-When you modify an element in a list, the list is changed in place, meaning the list's ID remains the same. This is because lists are mutable and their elements can be altered without creating a new list.
What function do you use to accept a list as input from the user in Python?
-To accept a list as input from the user in Python, you use the `eval(input())` function. This allows the user to input a list directly, which is then evaluated and stored as a list.
What is meant by 'traversing a list' in Python?
-Traversing a list means accessing each element of the list one by one, usually with a loop. This process allows you to process or display each element in the list.
Can you modify a list while traversing it using a for loop?
-Yes, you can modify a list while traversing it, but it’s generally not recommended because changing the list during iteration can lead to unexpected behavior. It’s better to create a new list or modify the list in a controlled manner.
Outlines
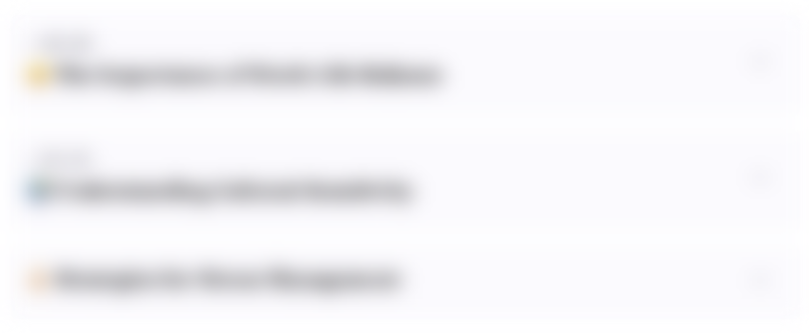
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
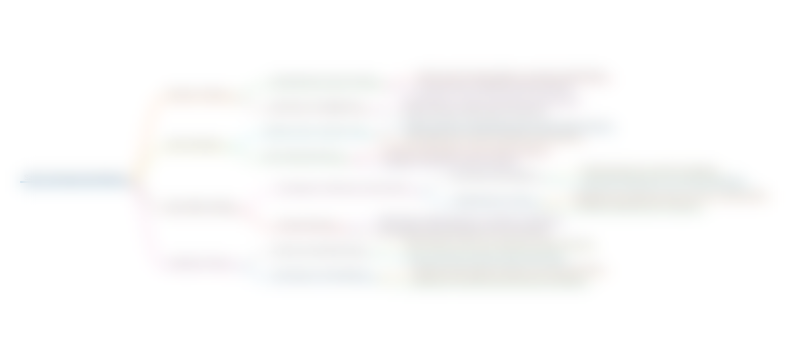
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
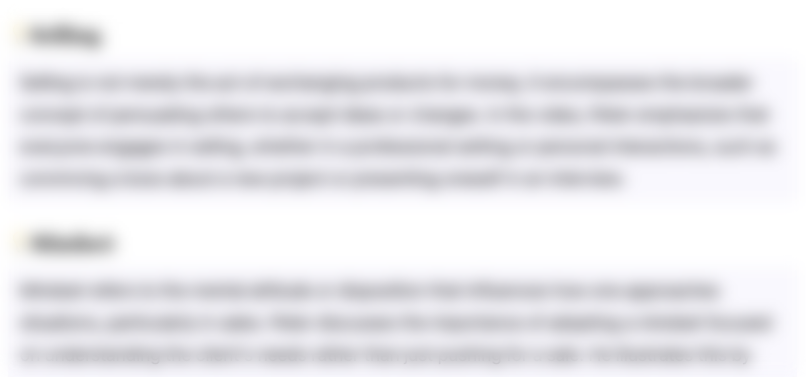
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
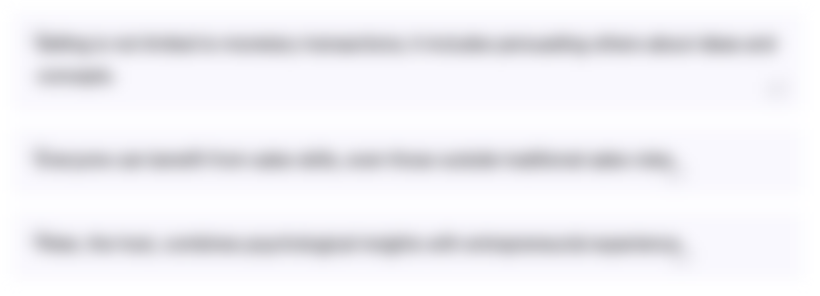
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
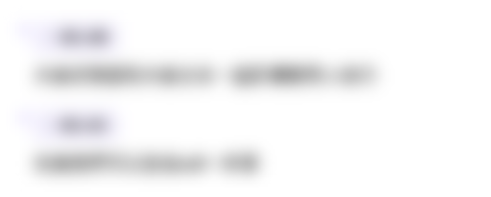
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Topic 4 Lesson 1
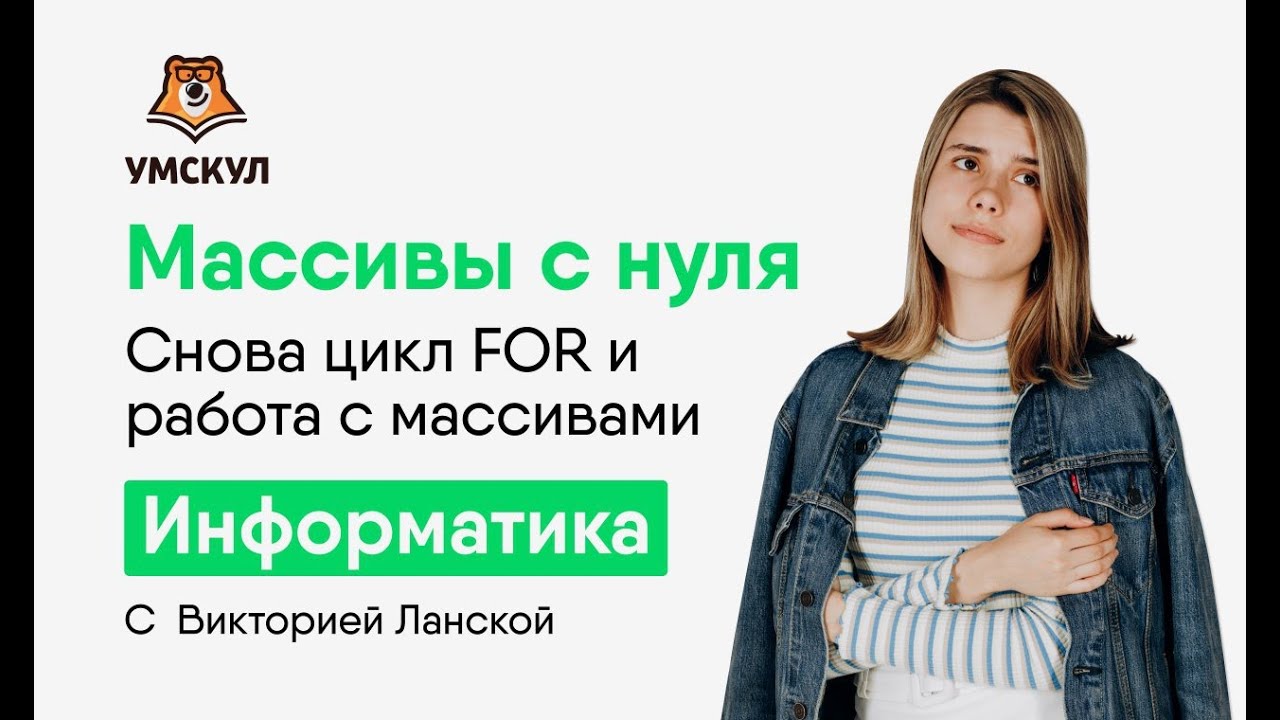
Python для ЕГЭ. Массивы с нуля. Снова цикл for и работа с массивами.
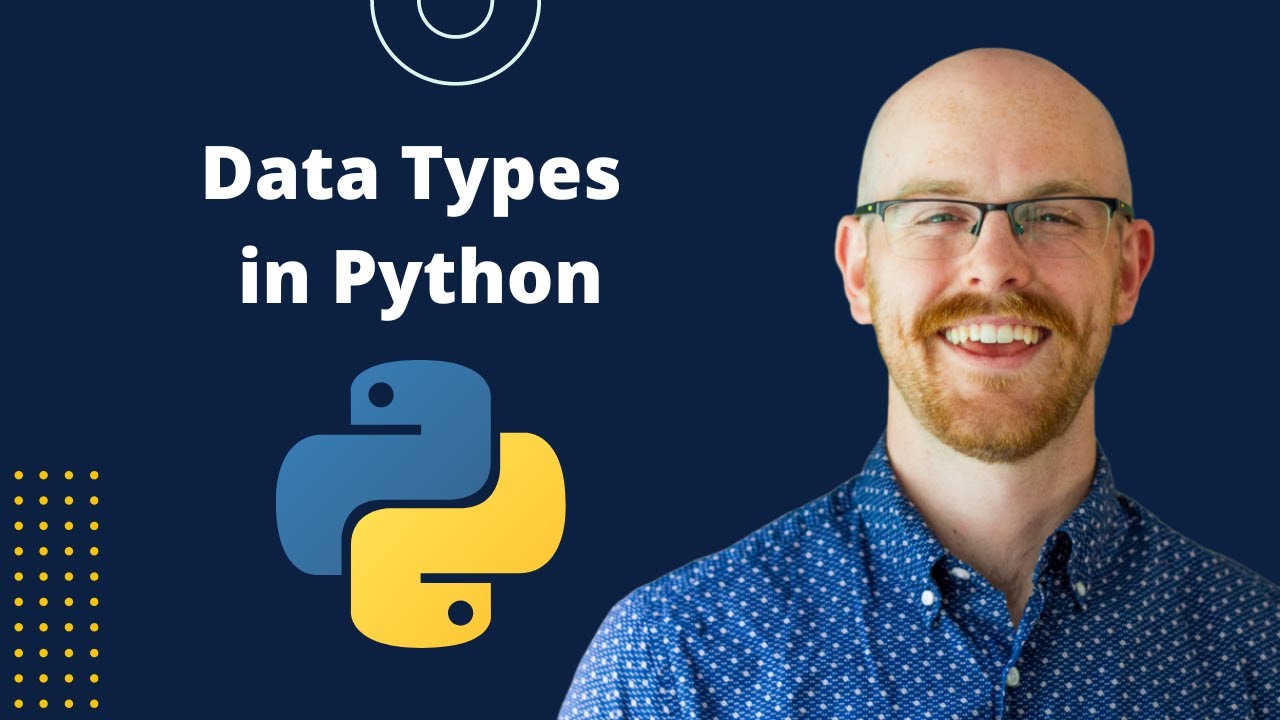
Data Types in Python | Python for Beginners

Belajar Python [Dasar] - 16 - Operasi dan manipulasi string (part 1)
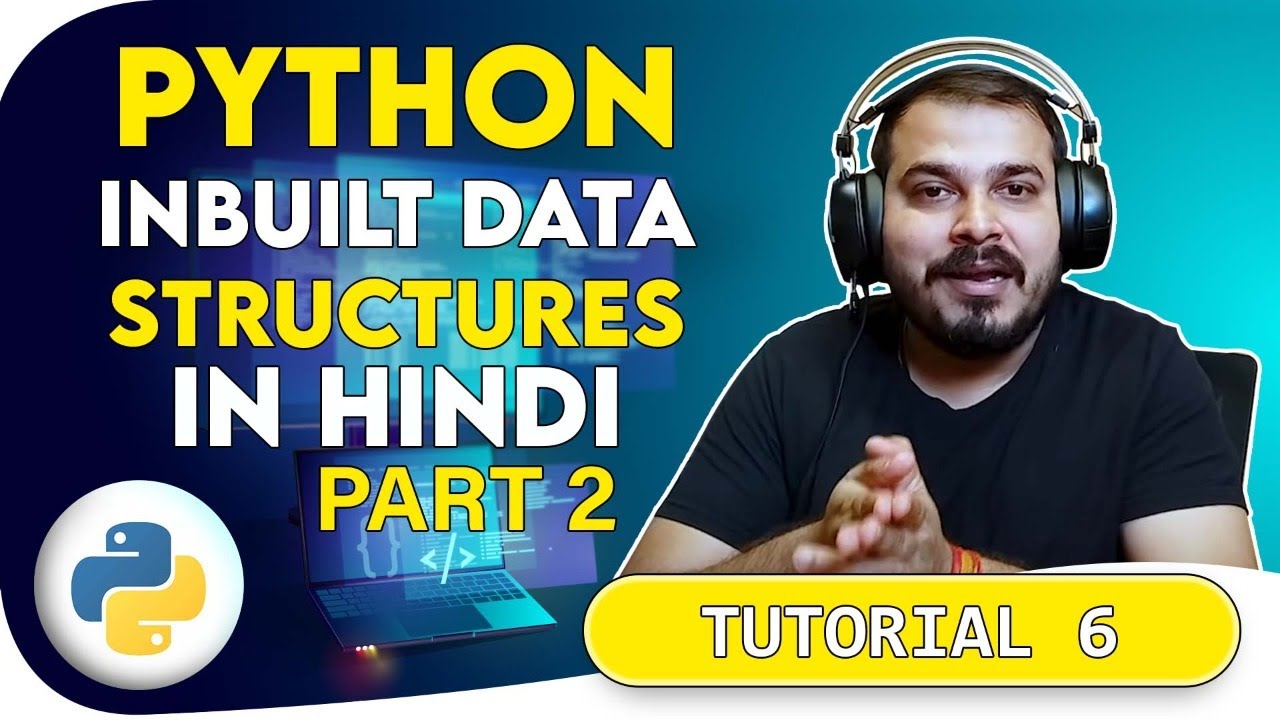
Tutorial 6- Python List And Its Inbuilt Function In Hindi

How variables works in Python | Explained with Animations
5.0 / 5 (0 votes)